开发当中,很多地方都需要红点气泡,红点内部显示数量
思路:
1.自定义view,先绘制一个圆形,在绘制一个text文本内容
2.根据view的宽高,取最小值,因为圆形的特点,必须具备正方形区域才能绘制圆形,所以在宽高当中取最小值来绘制圆形
3.内部的text,居中显示,文本的默认显示方法是基于文本的左下角为基点,所以为了让文本居中,要计算偏移量。
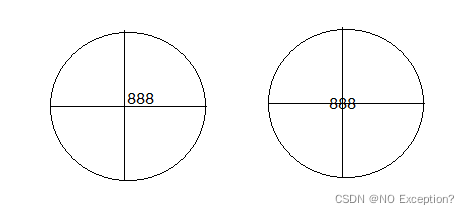
4.如何进行偏移呢?
先说X轴,只有知道了文本的宽度,向左偏移一半距离,就可以了
同理,Y轴也是这样的逻辑,向下偏移一半距离,就可以居中了。
val rect = Rect()
paintText.getTextBounds(numberStr, 0, numberStr!!.length, rect)
rect.getWidth()
rect.getHeight()
5.全部代码
class RedPoint constructor(
context: Context,
attrs: AttributeSet,
defStyleAttr: Int = 0,
defStyleRes: Int
) :
View(context, attrs, defStyleAttr, defStyleRes) {
constructor(context: Context, attrs: AttributeSet) : this(context, attrs, 0, 0)
private val paintBg = Paint()
private val paintText = Paint()
private val textSize =
TypedValue.applyDimension(COMPLEX_UNIT_SP, 14f, context.resources.displayMetrics)
private var numberStr: String? = ""
private var radius: Float = 0f
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec)
val width = MeasureSpec.getSize(widthMeasureSpec)
val height = MeasureSpec.getSize(heightMeasureSpec)
radius = width.coerceAtMost(height).toFloat() / 2
}
override fun onDraw(canvas: Canvas?) {
super.onDraw(canvas)
paintBg.color = Color.RED
canvas?.drawCircle(radius, radius, radius, paintBg)
paintText.color = Color.WHITE
paintText.style = Paint.Style.FILL
paintText.textSize = textSize
Log.d(TAG, "textsize:" + paintText.textSize);
val rect = Rect()
paintText.getTextBounds(numberStr, 0, numberStr!!.length, rect)
val x = radius - rect.width() / 2
val y = radius + rect.height() / 2
canvas?.drawText(numberStr!!, x, y, paintText)
}
fun setText(number: String) {
this.numberStr = number
invalidate()
}
private val TAG = "RedPoint"
}
|