1.创建画板?CustomPaint
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: SizedBox(
width: 400,
height: 400,
child: CustomPaint(painter: TestPaint(),),
),
),
);
}
2.创建绘画工具?CustomPainter
class TestPaint extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
canvas.drawLine(Offset(size.width / 2, size.height / 2 - (size.height * 0.3)), Offset(size.width / 2, 0), circlePaint);
}
@override
bool shouldRepaint(covariant TestPaint oldDelegate) {
//和刷新有关
return true;
}
}
3.一些常用样式绘制示例
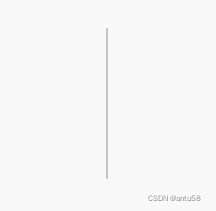
canvas.drawLine(Offset(size.width / 2, size.height / 2 - size.height), Offset(size.width / 2, 0), Paint());
?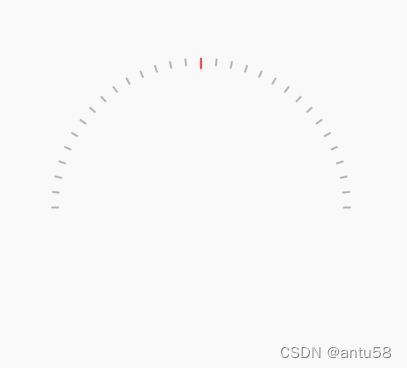
void drawScale(Canvas canvas, Size size) {
final Paint circlePaint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 2.5;
double angleRange = 180;
double progress = 0.5;
double rootAngle = (360 - angleRange) / 2 + 90;
final radius = min(size.width, size.height) / 2 - circlePaint.strokeWidth / 2;
circlePaint.color = Colors.black26;
Offset center = Offset(size.width / 2, size.height / 2);
for (int i = 0; i <= angleRange; i++) {
if (i % 6 == 0) {
Offset start = degreesToCoordinates(center, i + rootAngle, radius - 10);
Offset end = degreesToCoordinates(center, i + rootAngle, radius);
canvas.drawLine(start, end, circlePaint);
}
}
circlePaint.color = Colors.red;
Offset start = degreesToCoordinates(center, angleRange * progress + rootAngle, radius - 15);
Offset handler = degreesToCoordinates(center, angleRange * progress + rootAngle, radius);
canvas.drawLine(start, handler, circlePaint);
}
Offset degreesToCoordinates(Offset center, double degrees, double radius) {
return radiansToCoordinates(center, degreeToRadians(degrees), radius);
}
double degreeToRadians(double degree) {
return (pi / 180) * degree;
}
Offset radiansToCoordinates(Offset center, double radians, double radius) {
var dx = center.dx + radius * cos(radians);
var dy = center.dy + radius * sin(radians);
return Offset(dx, dy);
}
?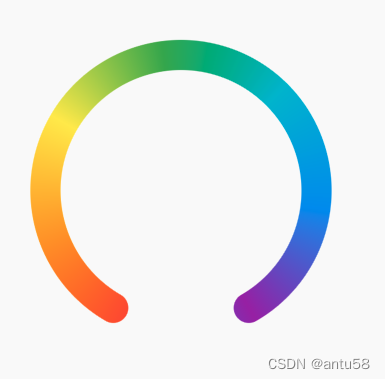
void drawCircle(Canvas canvas, Size size) {
final Paint circlePaint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 40
..strokeCap = StrokeCap.round;
double angleRange = 300;
List<Color> colors = [Colors.red, Colors.orange, Colors.yellow, Colors.green, Colors.cyan, Colors.blue, Colors.purple];
double a = pi / 180;
double rootAngle = (360 - angleRange) / 2 + 90;
double start = a * rootAngle;
double end = a * angleRange;
double redress = 15;
final radius = min(size.width, size.height) / 2 - circlePaint.strokeWidth / 2;
final rect = Rect.fromCircle(radius: radius, center: Offset(size.width / 2, size.height / 2));
circlePaint.shader = SweepGradient(
transform: GradientRotation(a * (rootAngle - redress)),
tileMode: TileMode.mirror,
colors: colors,
startAngle: 0,
endAngle: end + a * redress
).createShader(rect);
canvas.drawArc(rect, start, end, false, circlePaint);
}
?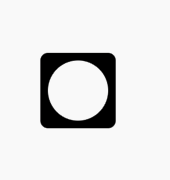
canvas.drawDRRect(RRect.fromLTRBR(0, 0, 100, 100, const Radius.circular(10)), RRect.fromLTRBR(10, 10, 90, 90, const Radius.circular(45)), Paint());
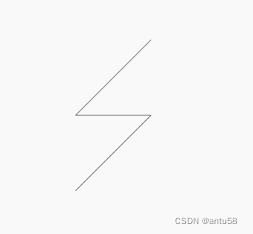
Path path = Path();
path.moveTo(200, 0);
path.lineTo(100, 100);
path.lineTo(200, 100);
path.lineTo(100, 200);
canvas.drawPath(path, Paint()..style = PaintingStyle.stroke);
?
|