实验内容:
设计实现一个判断题题库Android APP,其基本功能包括:
- 启动后显示第一个题目的界面,如图1-1所示,界面中包含了题目内容、“正确”、“错误”、“查看答案”和“下一个”按钮。点击“正确”或“错误”表示用于给出的答案,点击“下一个”进入下一个判断题;
- 用户点击“查看”答案后,显示如图1-2所示的界面,其中显示提示信息“确定看答案?”和“答案”按钮。用户点击“答案”后,界面显示该题目的标准答案,如图1-3所示;
- 用户如果在题目界面未选择答案而直接进入答案界面并查看了答案,再次回到题目界面(图1)点击“正确”或“错误”按钮,界面提示信息,如图1-4所示的“作弊是不对的”。
页面展示:
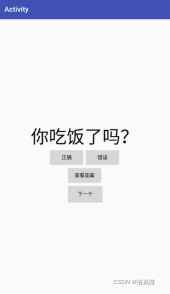 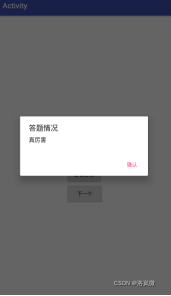 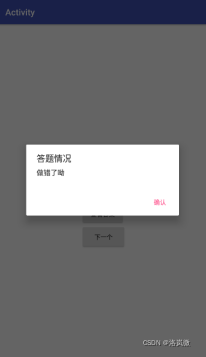
?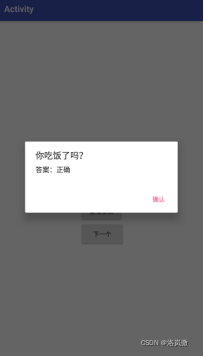 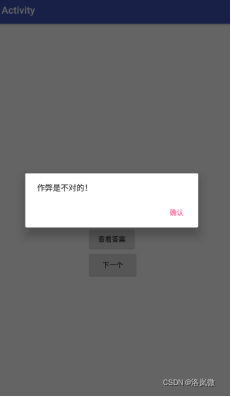 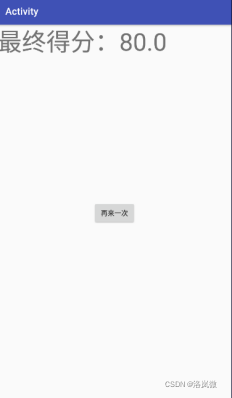
?代码如下:
?布局文件:activity_main.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/question"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:layout_above="@+id/wrapUp"
android:textSize="50sp"
android:textColor="#FF101113"
android:text="question"
/>
<LinearLayout
android:id="@+id/wrapUp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true">
<Button
android:id="@+id/Yes"
android:layout_width="100dp"
android:layout_height="50dp"
android:layout_toLeftOf="@+id/No"
android:text="正确" />
<Button
android:id="@+id/No"
android:layout_width="100dp"
android:layout_height="50dp"
android:layout_toRightOf="@+id/Yes"
android:text="错误" />
</LinearLayout>
<Button
android:id="@+id/Next"
android:layout_width="103dp"
android:layout_height="55dp"
android:text="下一个"
android:layout_below="@+id/showAnswer"
android:layout_alignLeft="@+id/showAnswer"
android:layout_alignStart="@+id/showAnswer" />
<Button
android:id="@+id/showAnswer"
android:layout_width="100dp"
android:layout_height="50dp"
android:text="查看答案"
android:layout_below="@+id/wrapUp"
android:layout_centerHorizontal="true" />
</RelativeLayout>
问题类Question:
public class Question {
public String question;
public boolean answer;
public boolean isCheated;
public Question() {
}
public boolean isAnswer() {
return answer;
}
public void setAnswer(boolean answer) {
this.answer = answer;
}
public String getQuestion() {
return question;
}
public void setQuestion(String question) {
this.question = question;
}
public boolean isCheated() {
return isCheated;
}
public void setCheated(boolean cheated) {
isCheated = cheated;
}
}
MainActivity:
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private List<Question> questions;
private TextView ques;
private int idofQuestions;
private double score;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initQuestions();
Button yes=(Button) findViewById(R.id.Yes);
Button no=(Button) findViewById(R.id.No);
Button showAnswer=(Button) findViewById(R.id.showAnswer);
Button next=(Button) findViewById(R.id.Next);
yes.setOnClickListener(this);
no.setOnClickListener(this);
showAnswer.setOnClickListener(this);
next.setOnClickListener(this);
}
/**
* 初始化问题列表
*/
public void initQuestions(){
ques=(TextView) findViewById(R.id.question);
this.questions=new ArrayList<>();
this.idofQuestions=0;
Question q1=new Question();
q1.setQuestion("你吃饭了吗?");q1.setAnswer(true);q1.setCheated(false);
this.questions.add(q1);
Question q2=new Question();
q2.setQuestion("你喜欢移动应用吗?");q2.setAnswer(true);q2.setCheated(false);
this.questions.add(q2);
Question q3=new Question();
q3.setQuestion("社会主义核心价值观");q3.setAnswer(true);q3.setCheated(false);
this.questions.add(q3);
Question q4=new Question();
q4.setQuestion("Activity");q4.setAnswer(true);q4.setCheated(false);
this.questions.add(q4);
Question q5=new Question();
q5.setQuestion("你追星吗");q5.setAnswer(false);q5.setCheated(false);
this.questions.add(q5);
ques.setText(this.questions.get(this.idofQuestions).getQuestion());
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.Yes:
AlertDialog.Builder dialog=new AlertDialog.Builder(MainActivity.this);
if(this.idofQuestions<this.questions.size()){
if(this.questions.get(this.idofQuestions).isCheated()==true){
dialog.setMessage("作弊是不对的!");
}
else{
if(this.questions.get(this.idofQuestions).isAnswer()==true){
this.score+=20;
dialog.setTitle("答题情况");
dialog.setMessage("真厉害");
}
else{
dialog.setTitle("答题情况");
dialog.setMessage("做错了呦");
}
}
dialog.setCancelable(true);
dialog.setPositiveButton("确认", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
/* dialog.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});*/
dialog.show();
}
else{
String data=""+this.score;
Intent intent=new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("extra_data",data);
startActivity(intent);
}
break;
case R.id.No:
AlertDialog.Builder dialog1=new AlertDialog.Builder(MainActivity.this);
if(this.idofQuestions<this.questions.size()){
if(this.questions.get(this.idofQuestions).isCheated()==true){
dialog1.setMessage("作弊是不对的!");
}
else{
if(this.questions.get(this.idofQuestions).isAnswer()==false){
this.score+=20;
dialog1.setTitle("答题情况");
dialog1.setMessage("厉害了");
}
else{
dialog1.setTitle("答题情况");
dialog1.setMessage("做错了呦");
}
}
dialog1.setCancelable(true);
dialog1.setPositiveButton("确认", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
dialog1.show();
}
else{
String data=""+this.score;
Intent intent=new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("extra_data",data);
startActivity(intent);
}
break;
case R.id.showAnswer:
AlertDialog.Builder dialog2=new AlertDialog.Builder(MainActivity.this);
this.questions.get(this.idofQuestions).setCheated(true);//作弊了
if(this.idofQuestions<this.questions.size()) {
dialog2.setTitle(this.questions.get(this.idofQuestions).getQuestion());
if(this.questions.get(this.idofQuestions).isAnswer()==true){
dialog2.setMessage("答案:正确");
}
else{
dialog2.setMessage("答案:错误");
}
dialog2.setCancelable(true);
dialog2.setPositiveButton("确认", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
dialog2.show();
}
break;
case R.id.Next:
this.idofQuestions++;
if(this.idofQuestions<this.questions.size()){
this.ques.setText(this.questions.get(this.idofQuestions).getQuestion());
}
else{
String data=""+this.score;
Intent intent=new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("extra_data",data);
startActivity(intent);
}
break;
}
}
}
?布局文件activity_second.xml :展示得分
得分SecondActivity:
public class SecondActivity extends AppCompatActivity {
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
Intent intent=getIntent();
String data=intent.getStringExtra("extra_data");
textView=(TextView) findViewById(R.id.score);
textView.setText("最终得分:"+data);
Button button2 = (Button) findViewById(R.id.button_2);
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent();
intent.putExtra("data_return", "Hello FirstActivity");
setResult(RESULT_OK, intent);
startActivity(intent);
}
});
}
@Override
public void onBackPressed() {
Intent intent=new Intent();
intent.putExtra("data_return","Hello FirstActivity");
setResult(RESULT_OK,intent);
finish();
}
}
?
?
?
|