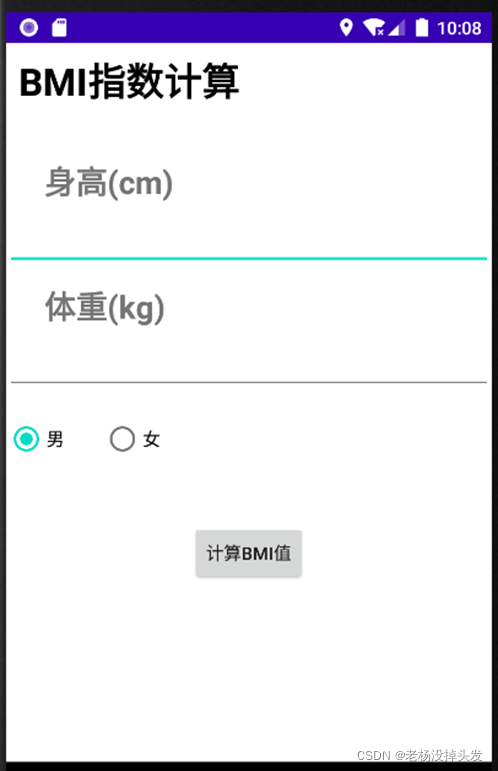
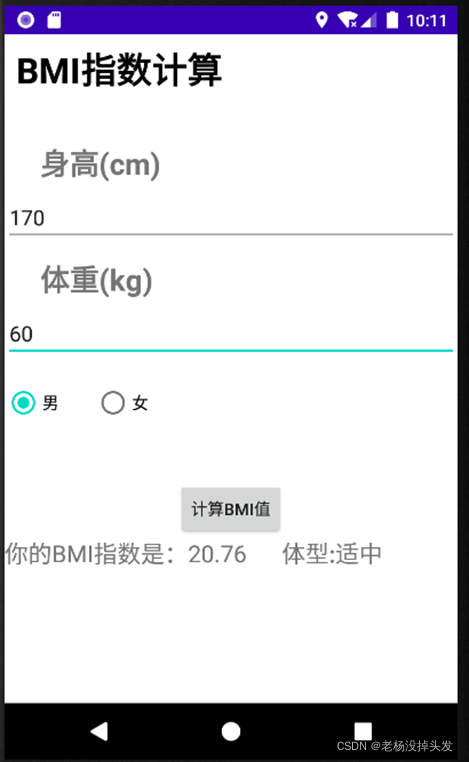
运行文件:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="fill_parent"
android:layout_height="70dp"
android:layout_marginTop="10dp"
android:layout_marginLeft="10dp"
android:text="BMI指数计算"
android:textColor="@color/black"
android:textSize="30sp"
android:textStyle="bold"/>
<TextView
android:layout_width="fill_parent"
android:layout_height="40dp"
android:layout_marginTop="13dp"
android:layout_marginLeft="30dp"
android:text="身高(cm)"
android:textSize="25sp"
android:textStyle="bold"
android:id="@+id/txt1"/>
<EditText
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/edX"/>
<TextView
android:layout_width="fill_parent"
android:layout_height="40dp"
android:layout_marginTop="13dp"
android:layout_marginLeft="30dp"
android:text="体重(kg)"
android:textSize="25sp"
android:textStyle="bold"
android:id="@+id/txt2"/>
<EditText
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/edY"/>
<RadioGroup
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_marginTop="20dp"
android:id="@+id/rg">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="男"
android:id="@+id/rb1"
android:layout_marginRight="30dp"
android:checked="true"/>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="女"
android:id="@+id/rb2"
/>
</RadioGroup>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btn"
android:layout_marginTop="50dp"
android:layout_gravity="center"
android:text="计算BMI值"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/tvResult"
android:textSize="20sp"/>
</LinearLayout>
package com.example.pro1;
import androidx.appcompat.app.AppCompatActivity;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.TextView;
import org.w3c.dom.Text;
public class bmi extends Activity implements View.OnClickListener {
RadioButton rb1;
RadioButton rb2;
TextView tvResult;
EditText txt1;
EditText txt2;
Button btn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.bmi);
setViews();
}
public void setViews() {
rb1 = (RadioButton) findViewById(R.id.rb1);
rb2 = (RadioButton) findViewById(R.id.rb2);//判断男女
tvResult = (TextView) findViewById(R.id.tvResult);
txt1 = (EditText)findViewById(R.id.edX);
txt2 = (EditText)findViewById(R.id.edY);
btn = (Button)findViewById(R.id.btn);
btn.setOnClickListener(this);
}
@Override
public void onClick(View v) {
double x = Double.parseDouble(txt1.getText().toString());//身高
double y = Double.parseDouble(txt2.getText().toString());//体重//体重除以身高的平方
double res;
String str = "你的BMI指数是:";
if(x<=0 || y<=0) {
tvResult.setText("值异常,不计算");
return ;
}
x = x/100;
res = y / (x*x);
String str1 = String.format("%.2f",res);
str = str + str1;
if(rb1.isChecked())
res -= 1;
str += " 体型:";
if(res < 19)
str += "过轻";
else if(res < 24)
str += "适中";
else if(res<29)
str += "超重";
else if(res<34)
str += "肥胖";
else
str += "严重肥胖";
tvResult.setText(str);
}
}
|