-
private?LruCache?mMemoryCache;
-
@Override
-
protected?void?onCreate(Bundle?savedInstanceState)?{
-
…
-
//?Get?memory?class?of?this?device,?exceeding?this?amount?will?throw?an
-
//?OutOfMemory?exception.
-
final?int?memClass?=?((ActivityManager)?context.getSystemService(
-
Context.ACTIVITY_SERVICE)).getMemoryClass();
-
//?Use?1/8th?of?the?available?memory?for?this?memory?cache.
-
final?int?cacheSize?=?1024?*?1024?*?memClass?/?8;
-
mMemoryCache?=?new?LruCache(cacheSize)?{
-
@Override
-
protected?int?sizeOf(String?key,?Bitmap?bitmap)?{
-
//?The?cache?size?will?be?measured?in?bytes?rather?than?number?of?items.
-
return?bitmap.getByteCount();
-
}
-
};
-
…
-
}
-
public?void?addBitmapToMemoryCache(String?key,?Bitmap?bitmap)?{
-
if?(getBitmapFromMemCache(key)?==?null)?{
-
mMemoryCache.put(key,?bitmap);
-
}
-
}
-
public?Bitmap?getBitmapFromMemCache(String?key)?{
-
return?mMemoryCache.get(key);
-
}
-
private?DiskLruCache?mDiskCache;
-
private?static?final?int?DISK_CACHE_SIZE?=?1024?*?1024?*?10;?//?10MB
-
private?static?final?String?DISK_CACHE_SUBDIR?=?“thumbnails”;
-
@Override
-
protected?void?onCreate(Bundle?savedInstanceState)?{
-
…
-
//?Initialize?memory?cache
-
…
-
File?cacheDir?=?getCacheDir(this,?DISK_CACHE_SUBDIR);
-
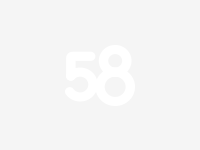
????mDiskCache?=?DiskLruCache.openCache(this,?cacheDir,?DISK_CACHE_SIZE);
-
…
-
}
-
class?BitmapWorkerTask?extends?AsyncTask?{
-
…
-
//?Decode?image?in?background.
-
@Override
-
protected?Bitmap?doInBackground(Integer…?params)?{
-
final?String?imageKey?=?String.valueOf(params[0]);
-
//?Check?disk?cache?in?background?thread
-
Bitmap?bitmap?=?getBitmapFromDiskCache(imageKey);
-
if?(bitmap?==?null)?{?//?Not?found?in?disk?cache
-
//?Process?as?normal
-
final?Bitmap?bitmap?=?decodeSampledBitmapFromResource(
-
getResources(),?params[0],?100,?100));
-
}
-
//?Add?final?bitmap?to?caches
-
addBitmapToCache(String.valueOf(imageKey,?bitmap);
-
return?bitmap;
-
}
-
…
-
}
-
public?void?addBitmapToCache(String?key,?Bitmap?bitmap)?{
-
//?Add?to?memory?cache?as?before
-
if?(getBitmapFromMemCache(key)?==?null)?{
-
mMemoryCache.put(key,?bitmap);
-
}
-
//?Also?add?to?disk?cache
-
if?(!mDiskCache.containsKey(key))?{