????????1.1.5 拼接字符串
java代码:
String strA = “a”;
String strB = “b”;
Log.e(“TAG”, “print:” + strA + strB);
kotlin代码:
val strA = “a”
val strB = “b”
Log.e(“TAG”, “print:
s
t
r
A
strA
strAstrB”)
????????1.1.8 更方便的集合操作
java代码:
final List listOfNumber = Arrays.asList(1, 2, 3, 4);
final Map<Integer, String> keyValue = new HashMap<Integer, String>();
map.put(1, “Amit”);
map.put(2, “Ali”);
map.put(3, “Mindorks”);
// Java 9
final List listOfNumber = List.of(1, 2, 3, 4);
final Map<Integer, String> keyValue = Map.of(1, “Amit”,
2, “Ali”,
3, “Mindorks”);
kotlin代码:
val listOfNumber = listOf(1, 2, 3, 4)
val keyValue = mapOf(1 to “Amit”,
2 to “Ali”,
3 to “Mindorks”)
????????1.1.9 方法定义
java代码:
void doSomething() {
// todo here
}
void doSomething(int… numbers) {
// todo here
}
kotlin代码:
fun doSomething() {
// logic here
}
fun doSomething(vararg numbers: Int) {
// logic here
}
????????1.2.0 类型判断和转换(隐式)
java代码:
if (object instanceof Car) {
Car car = (Car) object;
}
kotlin代码:
if (object is Car) {
var car = object // 智能转换
}
??1.3 点击事件
java代码:
btnLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
}
});
kotlin代码:
btn_login.setOnClickListener(View.OnClickListener { })
??1.4 精简空判断
????????1.4.1 防空判断
java代码:
if(a != null && a.b != null && a.b.c != null) {
String user = a.b.c.d;
}
kotlin代码:
val user = a?.b?.c?.d
????????1.4.2 空判断
java代码:
if (str != null) {
int length = str.length();
}
kotlin代码:
str?.let {
val length = str.length
}
// 更简单的写法
val length = str?.length
// 为null赋予默认值
val length = str?.length?:0
????????????????1.5.4.1 带返回值的方法
java代码:
int getScore() {
// logic here
return score;
}
kotlin代码:
fun getScore(): Int {
// logic here
return score
}
// 单表达式函数
fun getScore(): Int = score
????????????????1.5.4.1 无结束符号
java代码:
int getScore(int value) {
// logic here
return 2 * value;
}
kotlin代码:
fun getScore(value: Int): Int {
// logic here
return 2 * value
}
// 单表达式函数
fun getScore(value: Int): Int = 2 * value
???????1.5.5 单表达式函数
java代码:
public double cube(double x) {
return x * x * x;
}
kotlin代码:
fun cube(x: Double) : Double = x * x * x
???????1.5.6 可变参数数量(vararg)
java代码:
public int sum(int… numbers) { }
kotlin代码:
fun sum(vararg x: Int) { }
???????1.5.7 Main函数
java代码:
public class MyClass {
public static void main(String[] args){
}
}
kotlin代码:
fun main(args: Array) {
}
???????1.5.8 参数命名
java代码:
public static void main(String[]args){
openFile(“file.txt”, true);
}
public static File openFile(String filename, boolean readOnly) { }
kotlin代码:
fun main(args: Array) {
openFile(“file.txt”, readOnly = true)
}
fun openFile(filename: String, readOnly: Boolean) : File { }
???????1.5.9 方法重载(可选参数)
java代码:
public static void main(String[]args){
createFile(“file.txt”);
createFile(“file.txt”, true);
createFile(“file.txt”, true, false);
createExecutableFile(“file.txt”);
}
public static File createFile(String filename) { }
public static File createFile(String filename, boolean appendDate) { }
public static File createFile(String filename, boolean appendDate,
boolean executable) { }
public static File createExecutableFile(String filename) { }
kotlin代码:
fun main(args: Array) {
createFile(“file.txt”)
createFile(“file.txt”, true)
createFile(“file.txt”, appendDate = true)
createFile(“file.txt”, true, false)
createFile(“file.txt”, appendDate = true, executable = true)
createFile(“file.txt”, executable = true)
}
fun createFile(filename: String, appendDate: Boolean = false,
executable: Boolean = false): File { }
???????1.6.0 泛型函数
java代码:
public void init() {
List moduleInferred = createList(“net”);
}
public List createList(T item) { }
kotlin代码:
fun init() {
val module = createList(“net”)
val moduleInferred = createList(“net”)
}
fun createList(item: T): List { }
???????1.6 代码精简
==============================================================================
???????1.5.1 精简Set/Get方法
java代码:
public class User {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
kotlin代码:
class User {
var name : String? = null
}
属性访问对比
java代码:
user.setName(“Java”);
String name = user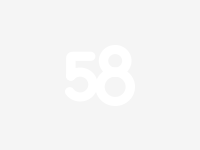 .getName();
kotlin代码:
user.name = “Kotlin”
String name = user.name
???????1.5.2 精简属性调用
java代码:
RequestBean requestBean = new RequestBean();
requestBean.method = AppService.METHOD_CASHIER;
requestBean.cartId = mCartId;
requestBean.merchantOrderInfo = “1234567890”;
requestBean.merchantOrderId = “1234567890”;
kotlin代码:
val requestBean = RequestBean().apply {
method = AppService.METHOD_CASHIER
cartId = mCartId
merchantOrderInfo = “1234567890”
merchantOrderId = “1234567890”
}
???????1.5.3 精简单例模式
java示例:
tName(“Java”);
String name = user[外链图片转存中…(img-S0puX0IK-1643359329256)] .getName();
kotlin代码:
user.name = “Kotlin”
String name = user.name
???????1.5.2 精简属性调用
java代码:
RequestBean requestBean = new RequestBean();
requestBean.method = AppService.METHOD_CASHIER;
requestBean.cartId = mCartId;
requestBean.merchantOrderInfo = “1234567890”;
requestBean.merchantOrderId = “1234567890”;
kotlin代码:
val requestBean = RequestBean().apply {
method = AppService.METHOD_CASHIER
cartId = mCartId
merchantOrderInfo = “1234567890”
merchantOrderId = “1234567890”
}
???????1.5.3 精简单例模式
java示例:
|