1、效果
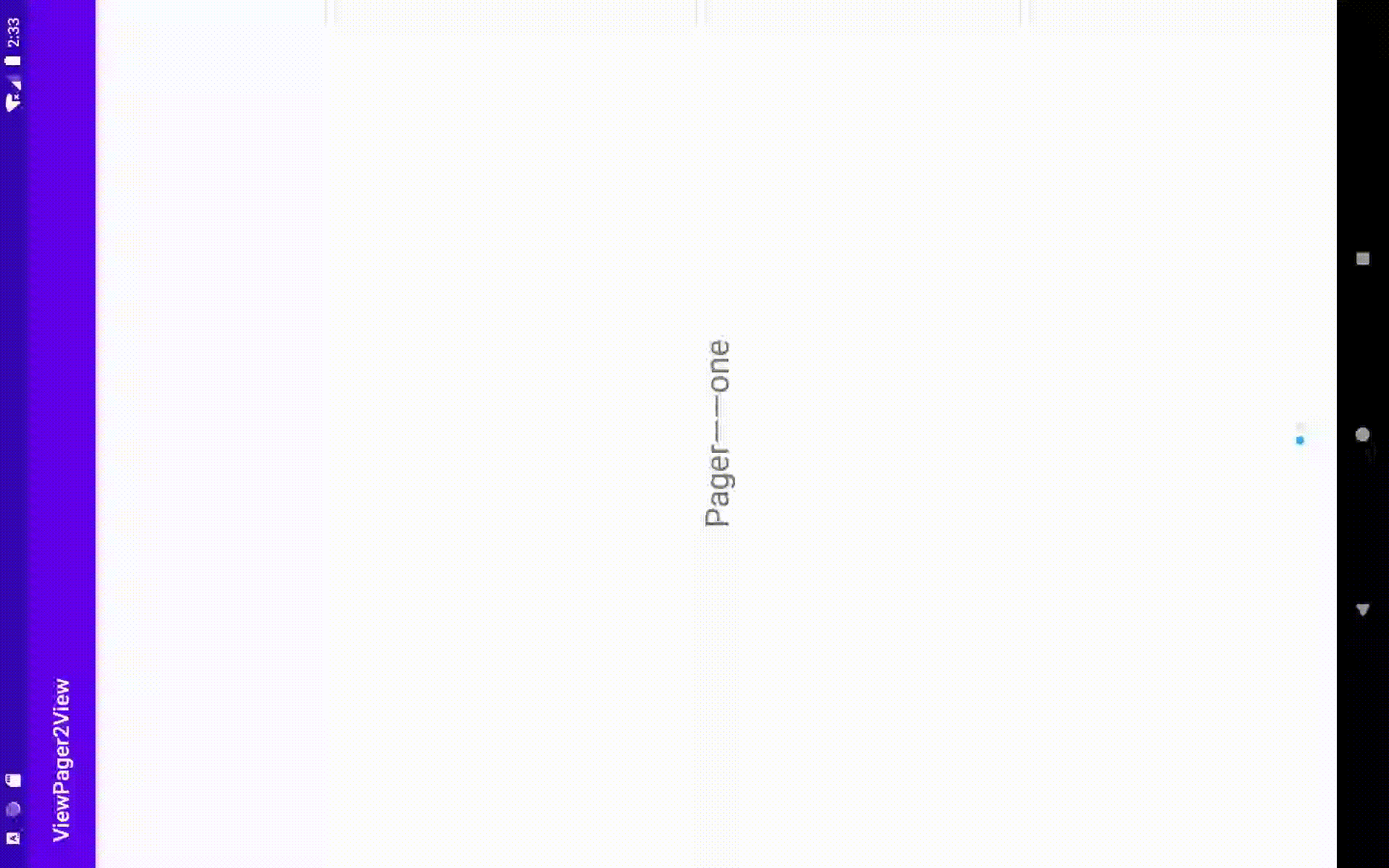
2、使用
2.1 Activity的XML文件
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.viewpager.widget.ViewPager
android:id="@+id/viewpager"
android:layout_width="match_parent"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
<LinearLayout
android:id="@+id/ll_dot"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_marginBottom="30dp"
android:gravity="center"
android:orientation="horizontal"
app:layout_constraintBottom_toBottomOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
?? ll_dot为指示器的布局文件
2.2 Activity文件
/**
* ViewPager中包含View
*/
public class MainActivity extends AppCompatActivity {
/**
* ViewPager控件
*/
private ViewPager mViewPager;
/**
* 底部的指示器控件
*/
private LinearLayout mDotLL;
/**
* ViewPager的数据源
* 其中存放的为ViewPager的布局View
*/
private List<View> views;
/**
* ViewPager中的布局
*/
private View viewOne,viewTwo;
/**
* ViewPager 的适配器
*/
private ViewPagerAdapter mAdpter;
/**
* 当前显示的是第几页
*/
private int curIndex = 0;
/**
* 布局加载起
*/
private LayoutInflater mInflater;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mInflater = LayoutInflater.from(this);
mViewPager = (ViewPager) findViewById(R.id.viewpager);
mDotLL = (LinearLayout) findViewById(R.id.ll_dot);
views = new ArrayList<>();
viewOne = mInflater.inflate(R.layout.layout_one, null);
viewTwo = mInflater.inflate(R.layout.layout_two, null);
views.add(viewOne);
views.add(viewTwo);
mAdpter = new ViewPagerAdapter(views);
mViewPager.setAdapter(mAdpter);
mViewPager.setCurrentItem(curIndex);
mViewPager.setOffscreenPageLimit(2);
setOvalLayout();
mViewPager.addOnPageChangeListener(new ViewPager.OnPageChangeListener() {
@Override
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) {
}
@Override
public void onPageSelected(int position) {
// 取消圆点选中
mDotLL.getChildAt(curIndex)
.findViewById(R.id.v_dot)
.setBackgroundResource(R.drawable.dot_normal);
// 圆点选中
mDotLL.getChildAt(position)
.findViewById(R.id.v_dot)
.setBackgroundResource(R.drawable.dot_selected);
curIndex = position;
}
@Override
public void onPageScrollStateChanged(int state) {
}
});
}
/**
* 设置圆点
*/
public void setOvalLayout() {
for (int i = 0; i < views.size(); i++) {
mDotLL.addView(mInflater.inflate(R.layout.dot, null));
}
// 默认显示第一页
mDotLL.getChildAt(0).findViewById(R.id.v_dot)
.setBackgroundResource(R.drawable.dot_selected);
}
}
2.3 PagerAdapter
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.viewpager.widget.PagerAdapter;
import java.util.List;
public class ViewPagerAdapter extends PagerAdapter {
private List<View> mViewList;
public ViewPagerAdapter(List<View> mViewList) {
this.mViewList = mViewList;
}
@Override
public void destroyItem(@NonNull ViewGroup container, int position, @NonNull Object object) {
container.removeView(mViewList.get(position));
}
@NonNull
@Override
public Object instantiateItem(@NonNull ViewGroup container, int position) {
container.addView(mViewList.get(position));
return (mViewList.get(position));
}
@Override
public int getCount() {
return mViewList == null ? 0 : mViewList.size();
}
@Override
public boolean isViewFromObject(@NonNull View view, @NonNull Object object) {
return view == object;
}
}
2.4 dot.xml文件(用作指示器)
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<!-- 小圆点View -->
<View
android:id="@+id/v_dot"
android:layout_width="8dp"
android:layout_height="8dp"
android:layout_marginLeft="2dp"
android:layout_marginRight="2dp"
android:background="@drawable/dot_normal"/>
</RelativeLayout>
2.5 dot_normal.xml未选中状态
<?xml version="1.0" encoding="utf-8"?><!-- 圆点未选中 -->
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<solid android:color="#90C4C4C4" />
<corners android:radius="8dp" />
</shape>
2.6 dot_selected.xml选中状态
<?xml version="1.0" encoding="utf-8"?><!-- 圆点选中 -->
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<solid android:color="#24AAF6" />
<corners android:radius="8dp" />
</shape>
3、Git地址
https://github.com/muyexiaogui/DailyExercise/tree/master/viewpager2view
|