List 有序集合,只读List,可变MutableList。分别通过listOf()和mutableListOf()创建
val systemUsers: MutableList<Int> = mutableListOf(1, 2, 3)
val sudoers: List<Int> = systemUsers
fun addSystemUser(newUser: Int) {
systemUsers.add(newUser)
}
fun getSysSudoers(): List<Int> {
return sudoers
}
fun main() {
addSystemUser(4)
println("Tot sudoers: ${getSysSudoers().size}")
getSysSudoers().forEach {
i -> println("Some useful info on user $i")
}
}
Set 无序集合,只读Set,可变MutableSet。分别通过setOf()和mutableSetOf()创建
val openIssues: MutableSet<String> = mutableSetOf("uniqueDescr1", "uniqueDescr2", "uniqueDescr3")
fun addIssue(uniqueDesc: String): Boolean {
return openIssues.add(uniqueDesc)
}
fun getStatusLog(isAdded: Boolean): String {
return if (isAdded) "registered correctly." else "marked as duplicate and rejected."
}
fun main() {
val aNewIssue: String = "uniqueDescr4"
val anIssueAlreadyIn: String = "uniqueDescr2"
println("Issue $aNewIssue ${getStatusLog(addIssue(aNewIssue))}")
println("Issue $anIssueAlreadyIn ${getStatusLog(addIssue(anIssueAlreadyIn))}")
}
Map key value 通过 mapOf() 和 mutableMapOf() 创建
val EZPassAccounts: MutableMap<Int, Int> = mutableMapOf(Pair(1,100), Pair(2,100), Pair(3,100)) 简便方式: A to B
val EZPassAccounts: MutableMap<Int, Int> = mutableMapOf(1 to 100, 2 to 100, 3 to 100)
to 的内部实现是中缀函数
public infix fun <A, B> A.to(that: B): Pair<A, B> = Pair(this, that)
const val POINTS_X_PASS: Int = 15
val EZPassAccounts: MutableMap<Int, Int> = mutableMapOf(1 to 100, 2 to 100, 3 to 100)
val EZPassReport: Map<Int, Int> = EZPassAccounts
fun updatePointsCredit(accountId: Int) {
if (EZPassAccounts.containsKey(accountId)) {
println("Updating $accountId...")
EZPassAccounts[accountId] = EZPassAccounts.getValue(accountId) + POINTS_X_PASS
} else {
println("Error: Trying to update a non-existing account (id: $accountId)")
}
}
fun accountsReport() {
println("EZ-Pass report:")
EZPassReport.forEach {
k, v -> println("ID $k: credit $v")
}
}
fun main() {
accountsReport()
updatePointsCredit(1)
updatePointsCredit(1)
updatePointsCredit(5)
accountsReport()
}
只读集合没有add方法,可变集合有add方法 继承关系
List Set
public interface Iterable<out T> {
public operator fun iterator(): Iterator<T>
}
public interface MutableIterable<out T> : Iterable<T>
public interface Collection<out E> : Iterable<E>
public interface MutableCollection<E> : Collection<E>, MutableIterable<E>
public interface List<out E> : Collection<E>
public interface MutableList<E> : List<E>, MutableCollection<E>
public interface Set<out E> : Collection<E>
public interface MutableSet<E> : Set<E>, MutableCollection<E>
Map:
public interface Map<K, out V>
public interface MutableMap<K, V> : Map<K, V>
集合方法:
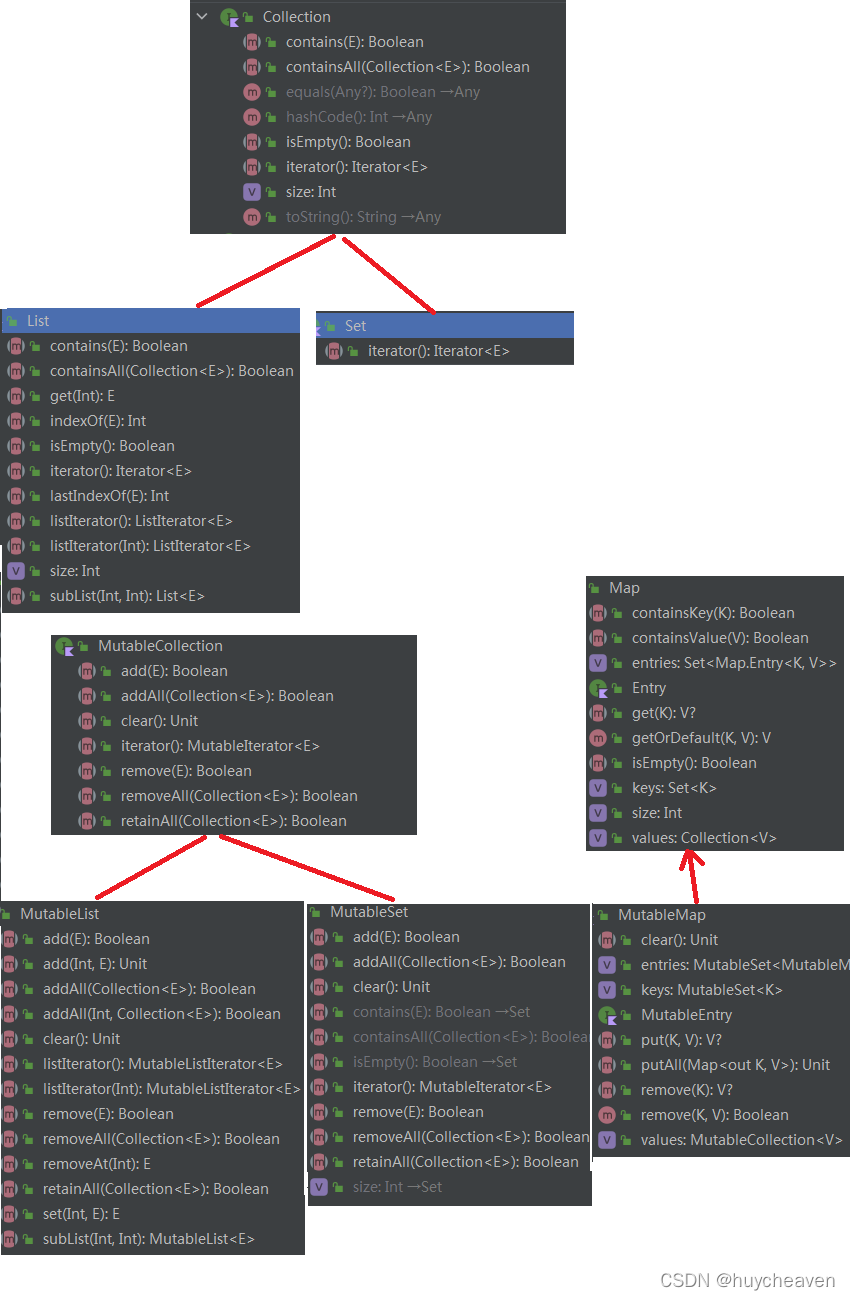
操作函数 filter map
val numbers = listOf(1, -2, 3, -4, 5, -6)
val positives = numbers.filter { x -> x > 0 }
val negatives = numbers.filter { it < 0 }
println("positives = $positives negatives = $negatives")
val doubled = numbers.map { x -> x * 2 }
val tripled = numbers.map { it * 3 }
println("doubled = $doubled tripled = $tripled")
操作函数 any, all, none any 集合中至少有一个满足判断条件 all 集合中每一个元素都满足判断条件 none 集合中没有一个元素满足判断条件
al numbers = listOf(1, -2, 3, -4, 5, -6)
val anyNegative = numbers.any { it < 0 }
val anyGT6 = numbers.any { it > 6 }
val allEven = numbers.all { it % 2 == 0 }
val allLess6 = numbers.all { it < 6 }
val allEven = numbers.none { it % 2 == 1 }
val allLess6 = numbers.none { it > 6 }
操作函数 find, findLast
find 返回满足条件的第一个元素 没有返回null findLast 返回满足条件的最后一个元素,没有返回null
val words = listOf("Lets", "find", "something", "in", "collection", "somehow")
val first = words.find { it.startsWith("some") }
val last = words.findLast { it.startsWith("some") }
val nothing = words.find { it.contains("nothing") }
操作函数 first, last first() 返回集合中的第一个元素 last() 返回集合中的最后一个元素
first{} 返回集合中满足条件的第一个元素 last{} 返回集合中满足条件的最后一个元素
val numbers = listOf(1, -2, 3, -4, 5, -6)
val first = numbers.first()
val last = numbers.last()
val firstEven = numbers.first { it % 2 == 0 }
val lastOdd = numbers.last { it % 2 != 0 }
操作函数 firstOrNull, lastOrNull firstOrNull() 返回集合中的第一个元素 集合为空返回null lastOrNull() 返回集合中的最后一个元素 集合为空返回null
firstOrNull{} 返回满足条件的集合中的第一个元素 lastOrNull{} 返回满足条件的集合中的最后一个元素
val words = listOf("foo", "bar", "baz", "faz")
val empty = emptyList<String>()
val first = words.firstOrNull()
val last = empty.lastOrNull()
val firstF = words.firstOrNull { it.startsWith('f') }
val firstZ = words.firstOrNull { it.startsWith('z') }
val lastF = words.lastOrNull { it.endsWith('f') }
val lastZ = words.lastOrNull { it.endsWith('z') }
操作函数 count() 返回集合数,count{}或者满足条件的集合数 associateBy 将list转化为Map<K,V>类型的集合 value为匹配集合中最后一个 groupBy 将list转化为Map<K, List>类型的集合 value为匹配集合
data class Person(val name: String, val city: String, val phone: String)
val people = listOf(
Person("John", "Boston", "+1-888-123456"),
Person("Svyatoslav", "Saint-Petersburg", "+7-999-456789"),
Person("Sarah", "Munich", "+49-777-789123"),
Person("Vasilisa", "Saint-Petersburg", "+7-999-123456"))
val phoneBook = people.associateBy { it.phone }
val cityBook = people.associateBy(Person::phone, Person::city)
val peopleCities = people.groupBy(Person::city, Person::name)
val lastPersonCity = people.associateBy(Person::city, Person::name)
结果:lastPersonCity = {Boston=John, Saint-Petersburg=Vasilisa, Munich=Sarah}
partition 根据条件将原集合拆分为2个集合。 第一个集合满足条件 第二个集合不满足条件
源码:
public inline fun <T> Iterable<T>.partition(predicate: (T) -> Boolean): Pair<List<T>, List<T>> {
val first = ArrayList<T>()
val second = ArrayList<T>()
for (element in this) {
if (predicate(element)) {
first.add(element)
} else {
second.add(element)
}
}
return Pair(first, second)
}
val numbers = listOf(1, -2, 3, -4, 5, -6)
val evenOdd = numbers.partition { it % 2 == 0 }
val (positives, negatives) = numbers.partition { it > 0 }
println("Numbers: $numbers")
println("Even numbers: ${evenOdd.first}")
println("Odd numbers: ${evenOdd.second}")
println("Positive numbers: $positives")
println("Negative numbers: $negatives")
flatMap 把原集合List<List>放到一个新集合List中并返回 主要是Iterable类型的集合
源码:
public inline fun <T, R> Iterable<T>.flatMap(transform: (T) -> Iterable<R>): List<R> {
return flatMapTo(ArrayList<R>(), transform)
}
public inline fun <T, R, C : MutableCollection<in R>> Iterable<T>.flatMapTo(destination: C, transform: (T) -> Iterable<R>): C {
for (element in this) {
val list = transform(element)
destination.addAll(list)
}
return destination
}
val fruitsBag = listOf("apple","orange","banana","grapes")
val clothesBag = listOf("shirts","pants","jeans")
val cart = listOf(fruitsBag, clothesBag)
val mapBag = cart.map { it }
val flatMapBag = cart.flatMap { it }
cart or mapBag : [[apple, orange, banana, grapes], [shirts, pants, jeans]]
flatMapBag : [apple, orange, banana, grapes, shirts, pants, jeans]
minOrNull, maxOrNull 返回最小值,最大值
sorted 按照自然顺序进行排序,默认是升序 sortedBy 按照给定条件进行排序 sortedDescending sortedByDescending 通过比较器进行排序
zip 中缀函数,将两个集合的每个元素拼接,然后组成一个新的集合,
public infix fun <T, R> Iterable<T>.zip(other: Iterable<R>): List<Pair<T, R>> {
return zip(other) { t1, t2 -> t1 to t2 }
}
val A = listOf("a", "b", "c")
val B = listOf(1, 2, 3, 4)
val resultPairs = A zip B
val resultReduce = A.zip(B) { a, b -> "$a$b" }
println("resultPairs: $resultPairs, resultReduce = $resultReduce")
resultPairs: [(a, 1), (b, 2), (c, 3)], resultReduce = [a1, b2, c3]
getOrElse 根据索引获取当前值,没有给一个默认值
val list = listOf(0, 10, 20)
println(list.getOrElse(1) { 42 })
println(list.getOrElse(10) { 42 })
结果:
10
42
|