一、Java锁机制
1.自旋转锁与互斥锁区别
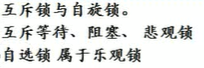
互斥锁:线程会从sleep(加锁)?running(解锁),过程中有上下文的切换,CPU的抢占,信号的发送等开销。 自旋锁:线程一直是running(加锁?解锁),死循环检测锁的标志位,机制不复杂。
2.公平与非公平锁
 
二、Disruptor
1.disruptor原理
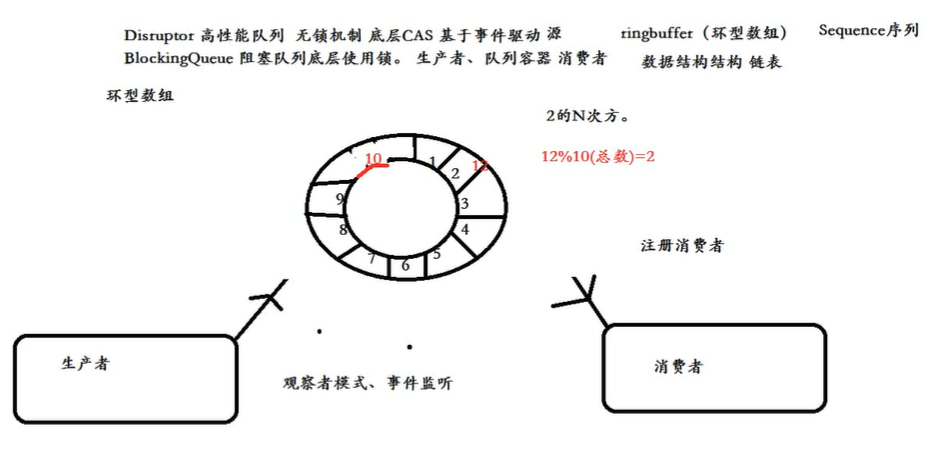
2.创建disruptor消费端与生产者
Pom Maven依赖信息
<dependencies>
<dependency>
<groupId>com.lmax</groupId>
<artifactId>disruptor</artifactId>
<version>3.2.1</version>
</dependency>
</dependencies>
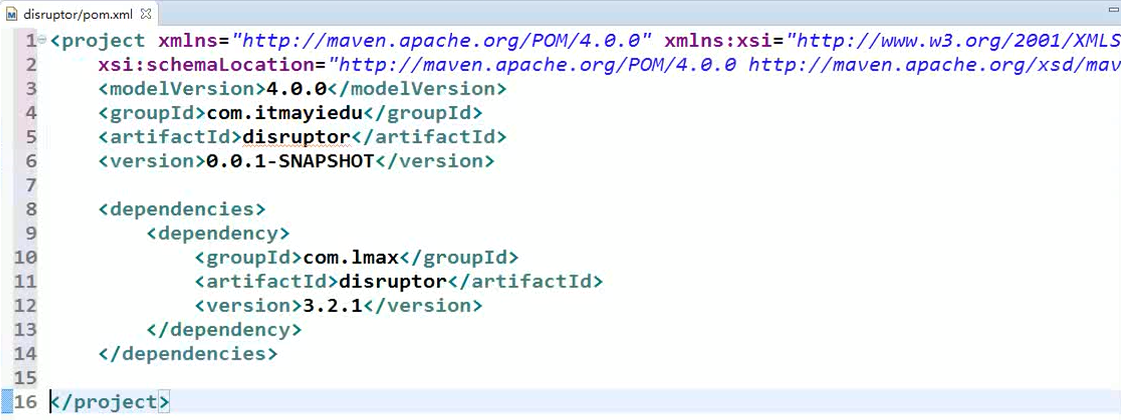
package com.itmayiedu.entity;
public class LongEvent {
private Long value;
public Long getValue() {
return value;
}
public void setValue(Long value) {
this.value = value;
}
}
package com.itmayiedu.factory;
import com.itmayiedu.entity.LongEvent;
import com.lmax.disruptor.EventFactory;
public class LongEventFactory implements EventFactory<LongEvent> {
public LongEvent newInstance() {
return new LongEvent();
}
}
package com.itmayiedu.consumer;
import com.itmayiedu.entity.LongEvent;
import com.lmax.disruptor.EventHandler;
public class LongEventHandler implements EventHandler<LongEvent> {
public void onEvent(LongEvent event, long sequence, boolean endOfBatch) throws Exception {
System.out.println("消费者1 获取生产者数据..event:" + event.getValue());
}
}
package com.itmayiedu.consumer;
import com.itmayiedu.entity.LongEvent;
import com.lmax.disruptor.EventHandler;
public class LongEventHandler2 implements EventHandler<LongEvent> {
public void onEvent(LongEvent event, long sequence, boolean endOfBatch) throws Exception {
System.out.println("消费者2 获取生产者数据..event:" + event.getValue());
}
}
package com.itmayiedu.producer;
import java.nio.ByteBuffer;
import com.itmayiedu.entity.LongEvent;
import com.lmax.disruptor.RingBuffer;
public class LongEventProducer {
private RingBuffer<LongEvent> ringBuffer;
public LongEventProducer(RingBuffer<LongEvent> ringBuffer) {
this.ringBuffer = ringBuffer;
}
public void onData(ByteBuffer byteBuffer) {
long sequence = ringBuffer.next();
try {
LongEvent longEvent = ringBuffer.get(sequence);
longEvent.setValue(byteBuffer.getLong(0));
} catch (Exception e) {
} finally {
System.out.println("生产者发送数据...");
ringBuffer.publish(sequence);
}
}
}
package com.itmayiedu.producer;
import java.nio.ByteBuffer;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import com.itmayiedu.consumer.LongEventHandler;
import com.itmayiedu.consumer.LongEventHandler2;
import com.itmayiedu.entity.LongEvent;
import com.itmayiedu.factory.LongEventFactory;
import com.lmax.disruptor.EventFactory;
import com.lmax.disruptor.RingBuffer;
import com.lmax.disruptor.YieldingWaitStrategy;
import com.lmax.disruptor.dsl.Disruptor;
import com.lmax.disruptor.dsl.ProducerType;
public class Main {
public static void main(String[] args) {
ExecutorService executor = Executors.newCachedThreadPool();
EventFactory<LongEvent> eventFactory = new LongEventFactory();
int ringbuffer = 1024 * 1024;
Disruptor<LongEvent> disruptor = new Disruptor<LongEvent>(eventFactory, ringbuffer, executor,
ProducerType.MULTI, new YieldingWaitStrategy());
disruptor.handleEventsWith(new LongEventHandler());
disruptor.handleEventsWith(new LongEventHandler2());
disruptor.start();
RingBuffer<LongEvent> ringBuffer = disruptor.getRingBuffer();
LongEventProducer producer = new LongEventProducer(ringBuffer);
ByteBuffer byteBuffer = ByteBuffer.allocate(8);
for (int i = 1; i < 100; i++) {
byteBuffer.putLong(0, i);
producer.onData(byteBuffer);
}
executor.shutdown();
disruptor.shutdown();
}
}
|