翻牌游戏
老师主要运用的是storyboard来编写程序,但说实话,真的用起来很不习惯。
还是用回纯代码编写吧。
主要知识点:
class ViewController: UIViewController {
var count = 0 {
didSet{
scoreLabel.text = "消耗步数:\(count)"
}
}
let fruitKindArray = ["🍎","🍉","🍊","🍓","🍈","🍇","🥝","🥑","🌰","🍑","🍌","🍒","🍏","🍍","🍐","🍋","🥬","🌶?"]
let animalKindArray = ["🐒","🦁?","🐯","🐔","🐶","🐍","🐷","🐎","🐑","🐫","🐭","🐲","🐰","🐱","🐘","🐂","🦎","🦔"]
var hang:Int = 7
var lie:Int = 4
lazy var mysteriousDataSource = animalKindArray
lazy var mysteriousArray = Array.init(repeating: "", count: hang*lie)
var matchingArray:Array<UIButton> = Array.init()
var matchingCount = 0 {
didSet{
if(matchingCount == hang*lie){
let fenmu = Double(count)
let fenzi = Double(count - hang*lie)
let score = 1.0 - fenzi/fenmu
let scoreString = String(format: "%.2f", score*100)
scoreLabel.text = "得分:\(scoreString)"
}
}
}
var scoreLabel:UILabel = UILabel.init()
override func viewDidLoad() {
super.viewDidLoad()
initMysteriousArray()
createScoreInterface()
createNewGameButton()
createCardsInterface()
}
func initMysteriousArray(){
mysteriousArray = Array.init(repeating: "", count: hang*lie)
for _ in 0 ..< Int(hang*lie/2) {
let kindIndex = Int(arc4random_uniform(UInt32(mysteriousDataSource.count)))
let kindString = mysteriousDataSource[kindIndex]
var times = 2
repeat{
let inputIndex = Int(arc4random_uniform(UInt32(mysteriousArray.count)))
if(mysteriousArray[inputIndex] == ""){
mysteriousArray[inputIndex] = kindString
times -= 1
}
}while(times>0)
}
}
func createScoreInterface(){
let screenSize = UIScreen.main.bounds.size
scoreLabel.frame = CGRect.init(x: 0, y: 20, width: screenSize.width*0.65, height: 90-20)
scoreLabel.font = UIFont.systemFont(ofSize: 25)
scoreLabel.textColor = UIColor.label
scoreLabel.textAlignment = NSTextAlignment.center
self.view.addSubview(scoreLabel)
count = 0
}
func createNewGameButton(){
let screenSize = UIScreen.main.bounds.size
let newGameButtonFrame = CGRect.init(x: screenSize.width*0.65, y: 35, width: screenSize.width*0.30, height: 90-50)
let newGameButton = UIButton.init(frame:newGameButtonFrame)
newGameButton.setTitle("New Game", for: UIControl.State.normal)
newGameButton.backgroundColor = UIColor.blue
newGameButton.titleLabel?.textColor = UIColor.white
newGameButton.layer.cornerRadius = 7
newGameButton.layer.masksToBounds = true
newGameButton.addTarget(self, action: #selector(newGame), for: UIControl.Event.touchUpInside)
self.view.addSubview(newGameButton)
}
func createCardsInterface(){
let outsideMargin = 20.0
let insideMargin = 10.0
let screenSize = UIScreen.main.bounds.size
let buttonWidth = (screenSize.width - outsideMargin*2 - insideMargin*Double(lie-1))/Double(lie)
let upMargin = 90.0
let downMargin = 35.0
let buttonHeight = (screenSize.height - upMargin - downMargin - insideMargin*Double(hang-1))/Double(hang)
for index in 0 ..< hang*lie {
let buttonX = outsideMargin + (Double)(index % lie)*(buttonWidth + insideMargin)
let buttonY = upMargin + Double(index / lie)*(buttonHeight + insideMargin)
let button = UIButton.init(frame: CGRect.init(x: buttonX, y: buttonY, width: buttonWidth, height: buttonHeight))
button.setTitle("👻", for: UIControl.State.normal)
button.backgroundColor = UIColor.black
button.titleLabel?.font = UIFont.systemFont(ofSize: 50)
button.layer.cornerRadius = 7
button.layer.masksToBounds = true
button.tag = index
button.addTarget(self, action: #selector(filpCard), for: UIControl.Event.touchUpInside)
self.view.addSubview(button)
}
}
@objc func filpCard(_ sender:UIButton){
if(matchingArray.count>=2){
return
}
count += 1
print("count : \(count)")
let tag = sender.tag
sender.isEnabled = false
matchingArray.append(sender)
let buttonString = mysteriousArray[tag] as String
sender.setTitle(buttonString, for: UIControl.State.normal)
sender.backgroundColor = UIColor.orange
if(matchingArray.count == 2){
self.perform(#selector(matching), with: nil, afterDelay: 0.5)
}
}
@objc func matching(){
let firstButton:UIButton = matchingArray[0] as UIButton
let secButton:UIButton = matchingArray[1] as UIButton
if (firstButton.titleLabel?.text == secButton.titleLabel?.text){
firstButton.removeFromSuperview()
secButton.removeFromSuperview()
matchingArray.removeAll()
matchingCount += 2
}else{
turnOffTheCard(firstButton)
turnOffTheCard(secButton)
matchingArray.removeAll()
}
}
func turnOffTheCard(_ button:UIButton){
button.setTitle("👻", for: UIControl.State.normal)
button.backgroundColor = UIColor.black
button.isEnabled = true
}
@objc func newGame(){
newGameHandle(withHang: 5, lie: 4)
}
func newGameHandle(withHang hang:Int,lie:Int){
self.hang = hang
self.lie = lie
count = 0
matchingCount = 0
initMysteriousArray()
createCardsInterface()
}
运行结果: 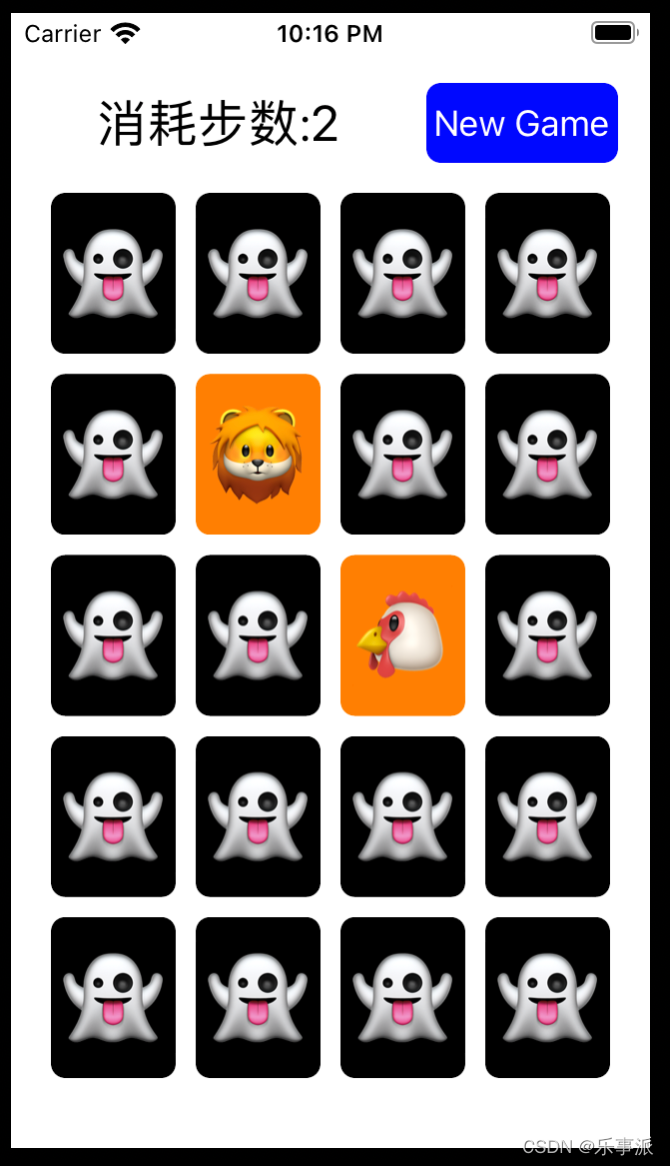
|