最近在写项目的时候,首页打算加一个地图SDK,并且在地图上标记出附近的球馆。由于附近球馆比较少,示例用电影院。
第一步:导入第三方库
首先要完成第三方库的导入:在这个实现中,主要需要导入下面三个库: AMap3DMap (用于显示地图) AMapLocation 定位SDK AMapSearch 搜索SDK
导入的时候可以通过CocoaPods ,可以看这篇博客去配置:【iOS开发】——CocoaPods的基本使用
第二步:设置info.plist
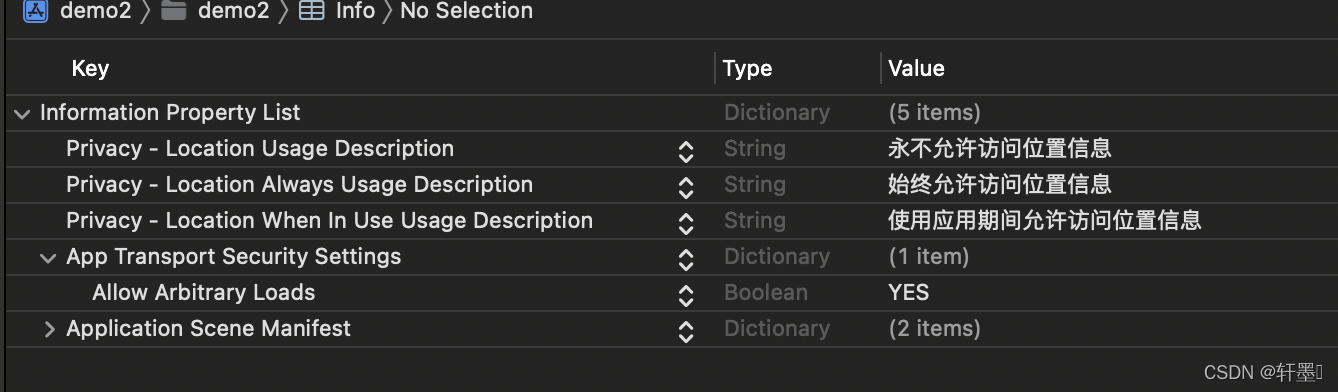
第三步:添加地图
_mapView = [[MAMapView alloc] initWithFrame:CGRectMake(0, 0, W, H/2)];
_mapView.showsUserLocation = YES;
_mapView.userTrackingMode = MAUserTrackingModeFollow;
[self.view addSubview:_mapView];
第四步:设置定位蓝点
MAUserLocationRepresentation *r = [[MAUserLocationRepresentation alloc] init];
r.showsHeadingIndicator = YES;
r.image = [UIImage imageNamed:@"daohang.png"];
[_mapView updateUserLocationRepresentation:r];
定位当前位置
self.locManager = [[CLLocationManager alloc] init];
self.locManager.delegate = self;
self.locManager.distanceFilter = 10.0f;
self.locManager.desiredAccuracy = kCLLocationAccuracyKilometer;
[self.locManager startUpdatingLocation];
代理方法:
-(void)locationManager:(CLLocationManager *)manager didUpdateLocations:(NSArray<CLLocation *> *)locations {
CLLocation *newLocation = locations[0];
CLGeocoder *geocoder = [[CLGeocoder alloc] init];
[geocoder reverseGeocodeLocation:newLocation completionHandler:^(NSArray *array, NSError *error){
if (array.count > 0){
CLPlacemark *placemark = [array objectAtIndex:0];
NSString *city = placemark.locality;
if (!city) {
city = placemark.administrativeArea;
}
[self searchPOI:city];
NSLog(@"city = %@", city);
}
else if (error == nil && [array count] == 0)
{
NSLog(@"No results were returned.");
}
else if (error != nil)
{
NSLog(@"An error occurred = %@", error);
}
}];
}
第五步:搜索方法
我这里用的是搜索所在城市的电影院,如果有需要搜索附近或者多边形内的地点等可以看高德官方开发文档。
- (void)searchPOI:(NSString*)city {
self.search = [[AMapSearchAPI alloc] init];
self.search.delegate = self;
AMapPOIKeywordsSearchRequest *request = [[AMapPOIKeywordsSearchRequest alloc] init];
request.keywords = @"电影院";
request.city = city;
request.types = @"电影院";
request.requireExtension = YES;
request.cityLimit = YES;
request.requireSubPOIs = YES;
[self.search AMapPOIKeywordsSearch:request];
}
第六步:设置搜索的代理方法
- (void)onPOISearchDone:(AMapPOISearchBaseRequest *)request response:(AMapPOISearchResponse *)response
{
if (response.pois.count == 0)
{
NSLog(@"没有查询到相关场所");
} else {
NSLog(@"%ld", response.pois.count);
[response.pois enumerateObjectsUsingBlock:^(AMapPOI *obj, NSUInteger idx, BOOL *stop) {
MAPointAnnotation *pointAnnotation = [[MAPointAnnotation alloc] init];
pointAnnotation.coordinate = CLLocationCoordinate2DMake(obj.location.latitude, obj.location.longitude);
[_mapView addAnnotation:pointAnnotation];
}];
}
}
第七步:自定义绘制点
- (MAAnnotationView *)mapView:(MAMapView *)mapView viewForAnnotation:(id <MAAnnotation>)annotation {
if ([annotation isKindOfClass:[MAPointAnnotation class]])
{
static NSString *pointReuseIndentifier = @"pointReuseIndentifier";
MAPinAnnotationView*annotationView = (MAPinAnnotationView*)[mapView dequeueReusableAnnotationViewWithIdentifier:pointReuseIndentifier];
if (annotationView == nil)
{
annotationView = [[MAPinAnnotationView alloc] initWithAnnotation:annotation reuseIdentifier:pointReuseIndentifier];
}
annotationView.canShowCallout= YES;
annotationView.animatesDrop = YES;
annotationView.draggable = YES;
annotationView.pinColor = MAPinAnnotationColorPurple;
return annotationView;
}
return nil;
}
完整代码:
#import <UIKit/UIKit.h>
#import <AMapSearchKit/AMapSearchKit.h>
#import <CoreLocation/CoreLocation.h>
#import <MAMapKit/MAMapKit.h>
#import <AMapFoundationKit/AMapFoundationKit.h>
@interface ViewController : UIViewController
<AMapSearchDelegate,
CLLocationManagerDelegate,
MAMapViewDelegate>
@property (nonatomic) AMapSearchAPI* search;
@property (nonatomic, strong) MAMapView *mapView;
@property (strong, nonatomic) CLLocationManager *locManager;
@property (strong, nonatomic) CLLocation *checkinLocation;
@end
#import "ViewController.h"
#define W [UIScreen mainScreen].bounds.size.width
#define H [UIScreen mainScreen].bounds.size.height
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
_mapView = [[MAMapView alloc] initWithFrame:CGRectMake(0, 0, W, H/2)];
_mapView.showsUserLocation = YES;
_mapView.userTrackingMode = MAUserTrackingModeFollow;
_mapView.showsUserLocation = YES;
_mapView.userTrackingMode = MAUserTrackingModeFollow;
MAUserLocationRepresentation *r = [[MAUserLocationRepresentation alloc] init];
r.showsHeadingIndicator = YES;
r.image = [UIImage imageNamed:@"daohang.png"];
[_mapView updateUserLocationRepresentation:r];
[self.view addSubview:_mapView];
self.locManager = [[CLLocationManager alloc] init];
self.locManager.delegate = self;
self.locManager.distanceFilter = 10.0f;
self.locManager.desiredAccuracy = kCLLocationAccuracyKilometer;
[self.locManager startUpdatingLocation];
}
- (void)onPOISearchDone:(AMapPOISearchBaseRequest *)request response:(AMapPOISearchResponse *)response
{
if (response.pois.count == 0)
{
NSLog(@"没有查询到相关场所");
} else {
NSLog(@"%ld", response.pois.count);
[response.pois enumerateObjectsUsingBlock:^(AMapPOI *obj, NSUInteger idx, BOOL *stop) {
MAPointAnnotation *pointAnnotation = [[MAPointAnnotation alloc] init];
pointAnnotation.coordinate = CLLocationCoordinate2DMake(obj.location.latitude, obj.location.longitude);
[_mapView addAnnotation:pointAnnotation];
}];
}
}
- (void)AMapSearchRequest:(id)request didFailWithError:(NSError *)error
{
NSLog(@"Error: %@", error);
}
- (MAAnnotationView *)mapView:(MAMapView *)mapView viewForAnnotation:(id <MAAnnotation>)annotation {
if ([annotation isKindOfClass:[MAPointAnnotation class]])
{
static NSString *pointReuseIndentifier = @"pointReuseIndentifier";
MAPinAnnotationView*annotationView = (MAPinAnnotationView*)[mapView dequeueReusableAnnotationViewWithIdentifier:pointReuseIndentifier];
if (annotationView == nil)
{
annotationView = [[MAPinAnnotationView alloc] initWithAnnotation:annotation reuseIdentifier:pointReuseIndentifier];
}
annotationView.canShowCallout= YES;
annotationView.animatesDrop = YES;
annotationView.draggable = YES;
annotationView.pinColor = MAPinAnnotationColorPurple;
return annotationView;
}
return nil;
}
-(void)locationManager:(CLLocationManager *)manager didUpdateLocations:(NSArray<CLLocation *> *)locations {
CLLocation *newLocation = locations[0];
CLGeocoder *geocoder = [[CLGeocoder alloc] init];
[geocoder reverseGeocodeLocation:newLocation completionHandler:^(NSArray *array, NSError *error){
if (array.count > 0){
CLPlacemark *placemark = [array objectAtIndex:0];
NSString *city = placemark.locality;
if (!city) {
city = placemark.administrativeArea;
}
[self searchPOI:city];
NSLog(@"city = %@", city);
}
else if (error == nil && [array count] == 0)
{
NSLog(@"No results were returned.");
}
else if (error != nil)
{
NSLog(@"An error occurred = %@", error);
}
}];
}
- (void)searchPOI:(NSString*)city {
self.search = [[AMapSearchAPI alloc] init];
self.search.delegate = self;
AMapPOIKeywordsSearchRequest *request = [[AMapPOIKeywordsSearchRequest alloc] init];
request.keywords = @"电影院";
request.city = city;
request.types = @"电影院";
request.requireExtension = YES;
request.cityLimit = YES;
request.requireSubPOIs = YES;
[self.search AMapPOIKeywordsSearch:request];
}
@end
注意:别忘了在AppDelegate.m文件中加入自己的apiKey:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[AMapServices sharedServices].apiKey = @"yourKey";
return YES;
}
效果:
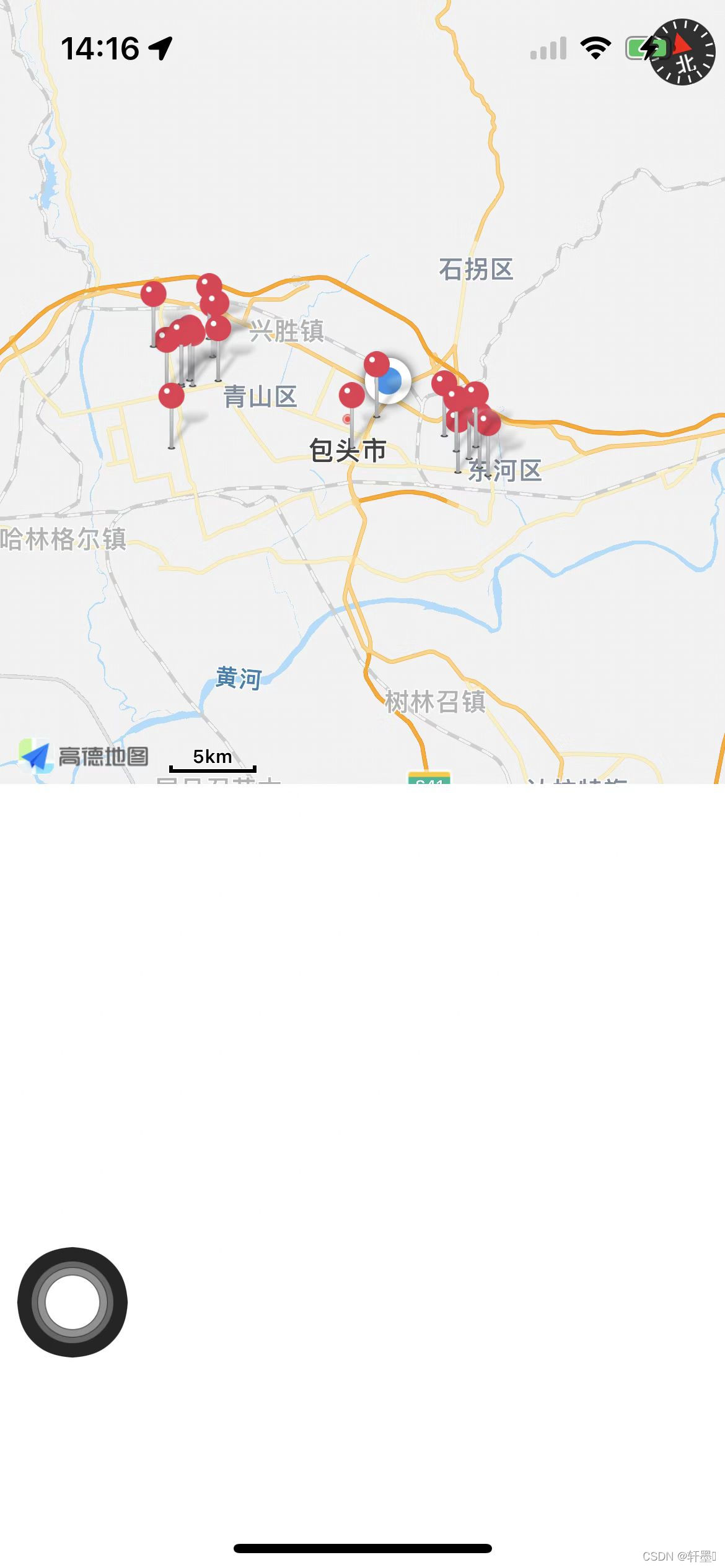
|