关于一个刷题软件的简易功能实现
自己建项目名GeoQuiz , 不多说了,直接上代码了。。。
1. activity_quiz.xml布局及代码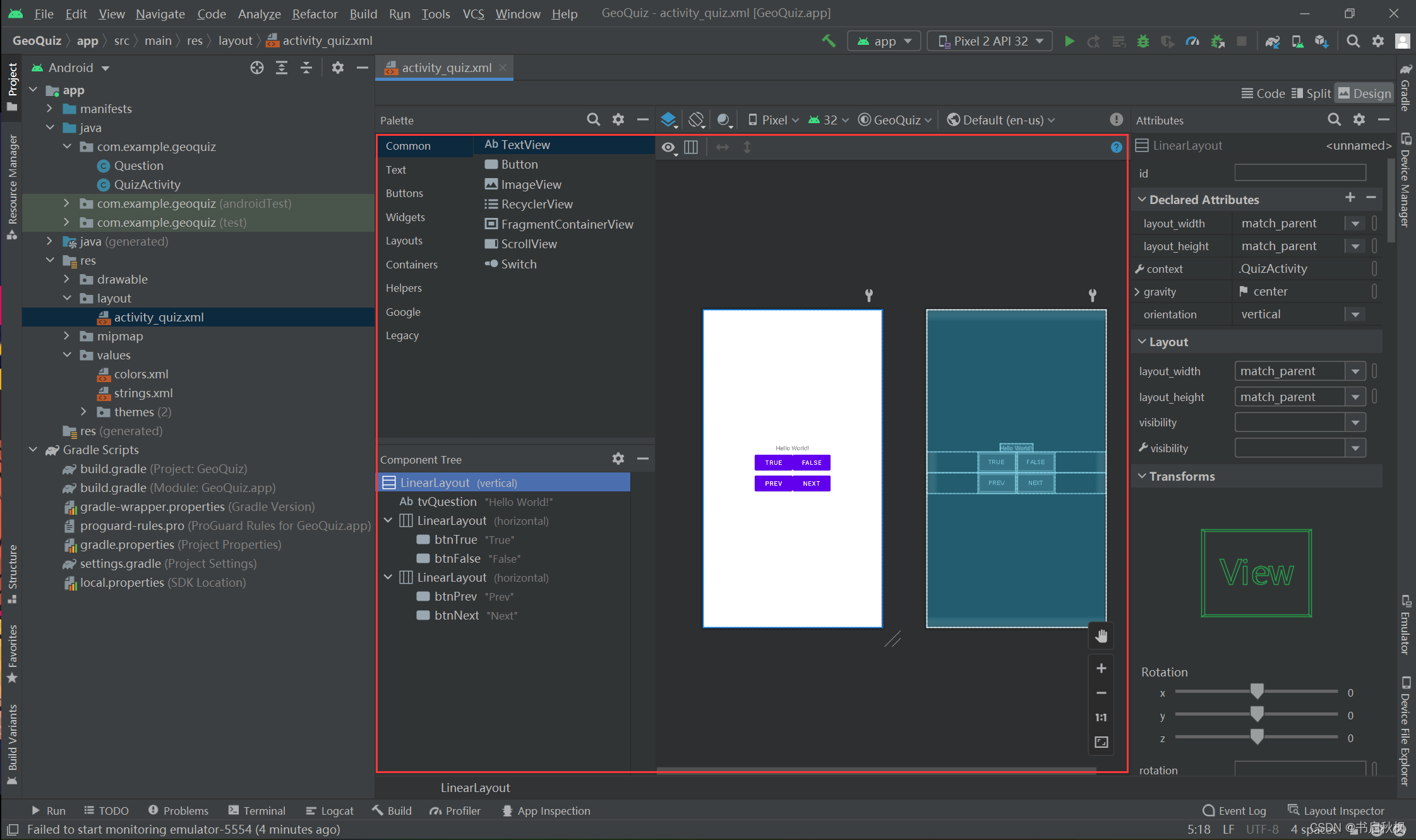
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
tools:context=".QuizActivity">
<TextView
android:id="@+id/tvQuestion"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal">
<Button
android:id="@+id/btnTrue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="True" />
<Button
android:id="@+id/btnFalse"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="False" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal">
<Button
android:id="@+id/btnPrev"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Prev" />
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next" />
</LinearLayout>
</LinearLayout>
?2. strings.xml代码实现
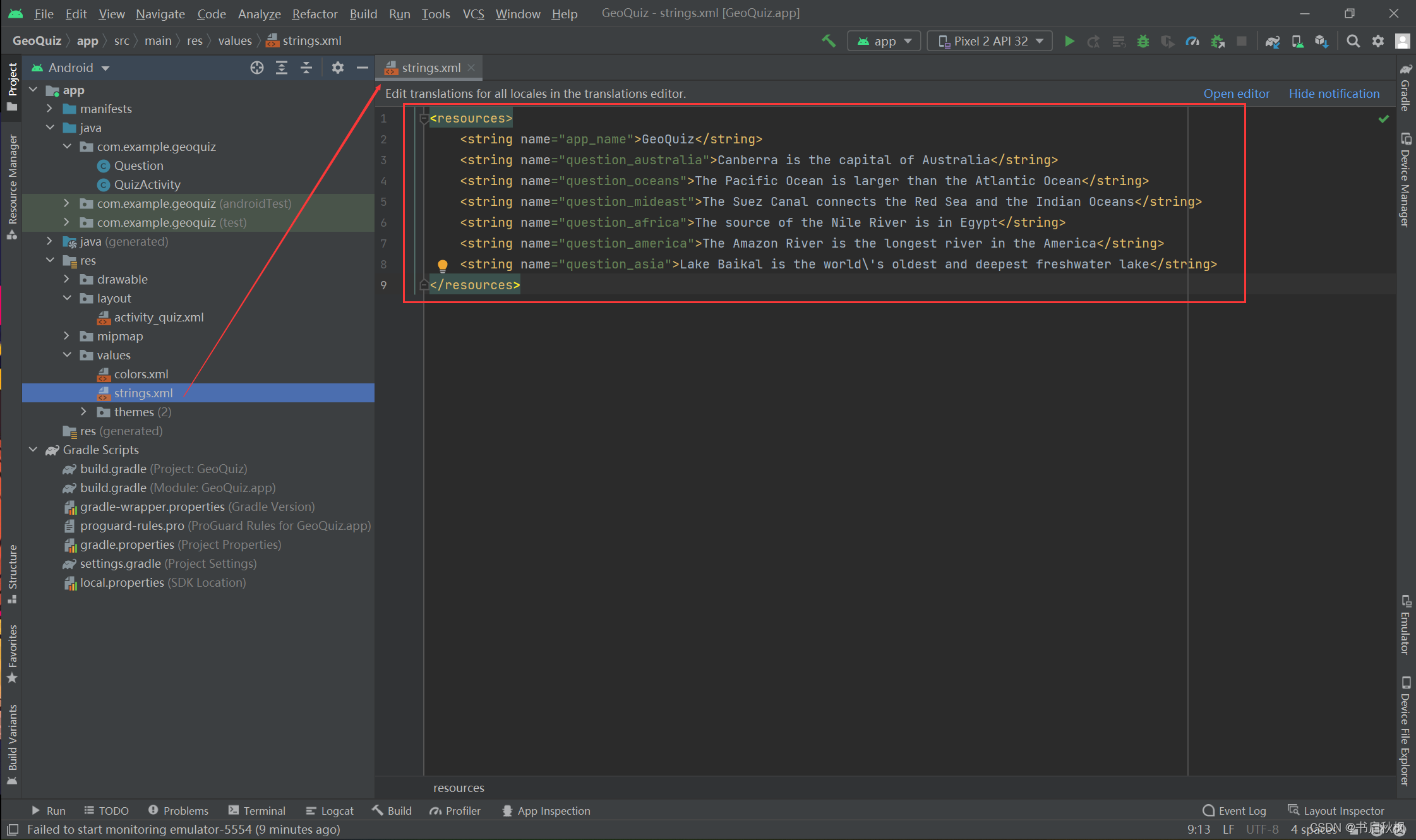
<resources>
<string name="app_name">GeoQuiz</string>
<string name="question_australia">Canberra is the capital of Australia</string>
<string name="question_oceans">The Pacific Ocean is larger than the Atlantic Ocean</string>
<string name="question_mideast">The Suez Canal connects the Red Sea and the Indian Oceans</string>
<string name="question_africa">The source of the Nile River is in Egypt</string>
<string name="question_america">The Amazon River is the longest river in the America</string>
<string name="question_asia">Lake Baikal is the world\'s oldest and deepest freshwater lake</string>
</resources>
3. Question代码实现
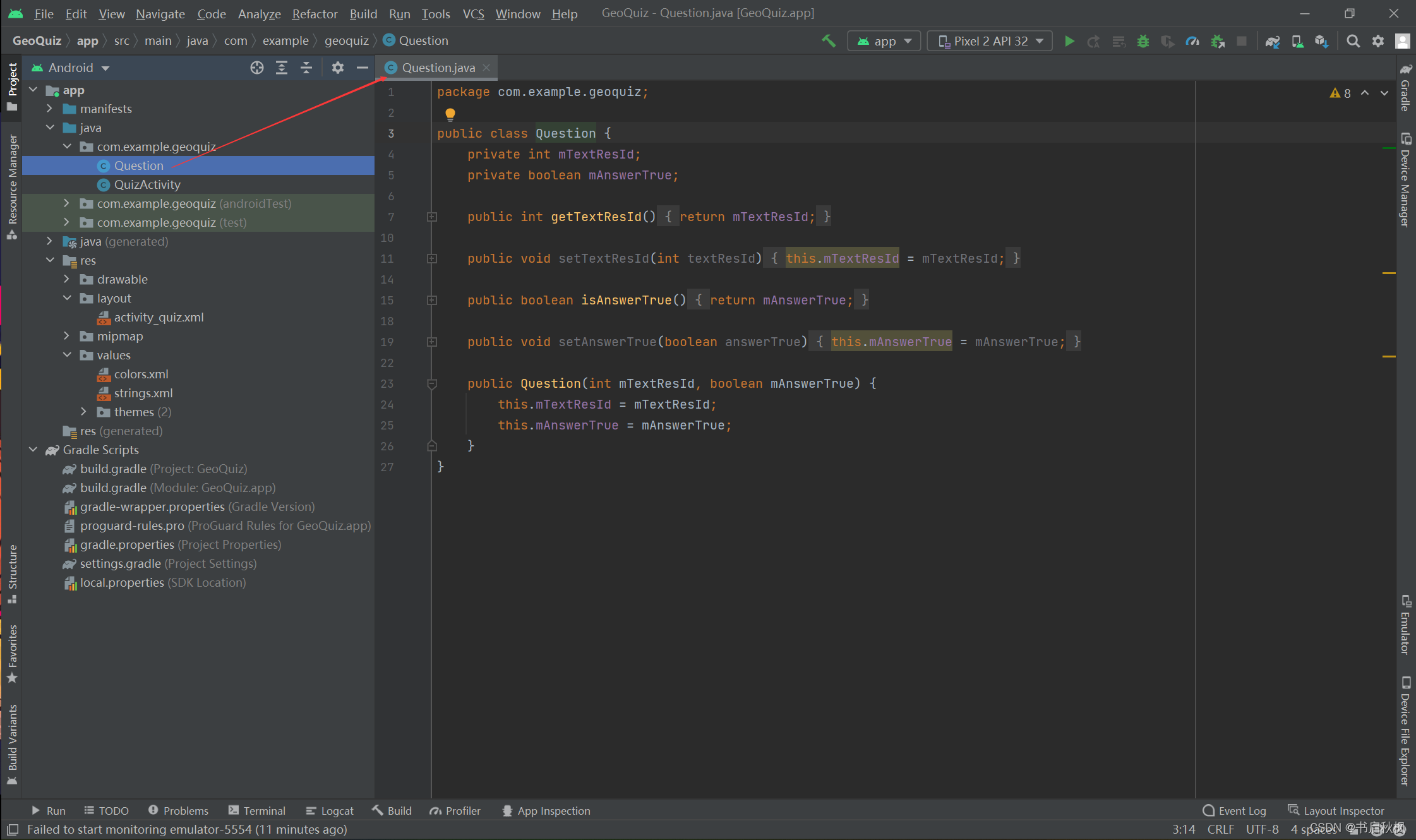
package com.example.geoquiz;
public class Question {
private int mTextResId;
private boolean mAnswerTrue;
public int getTextResId() {
return mTextResId;
}
public void setTextResId(int textResId) {
this.mTextResId = mTextResId;
}
public boolean isAnswerTrue() {
return mAnswerTrue;
}
public void setAnswerTrue(boolean answerTrue) {
this.mAnswerTrue = mAnswerTrue;
}
public Question(int mTextResId, boolean mAnswerTrue) {
this.mTextResId = mTextResId;
this.mAnswerTrue = mAnswerTrue;
}
}
4. QuizActivity代码实现
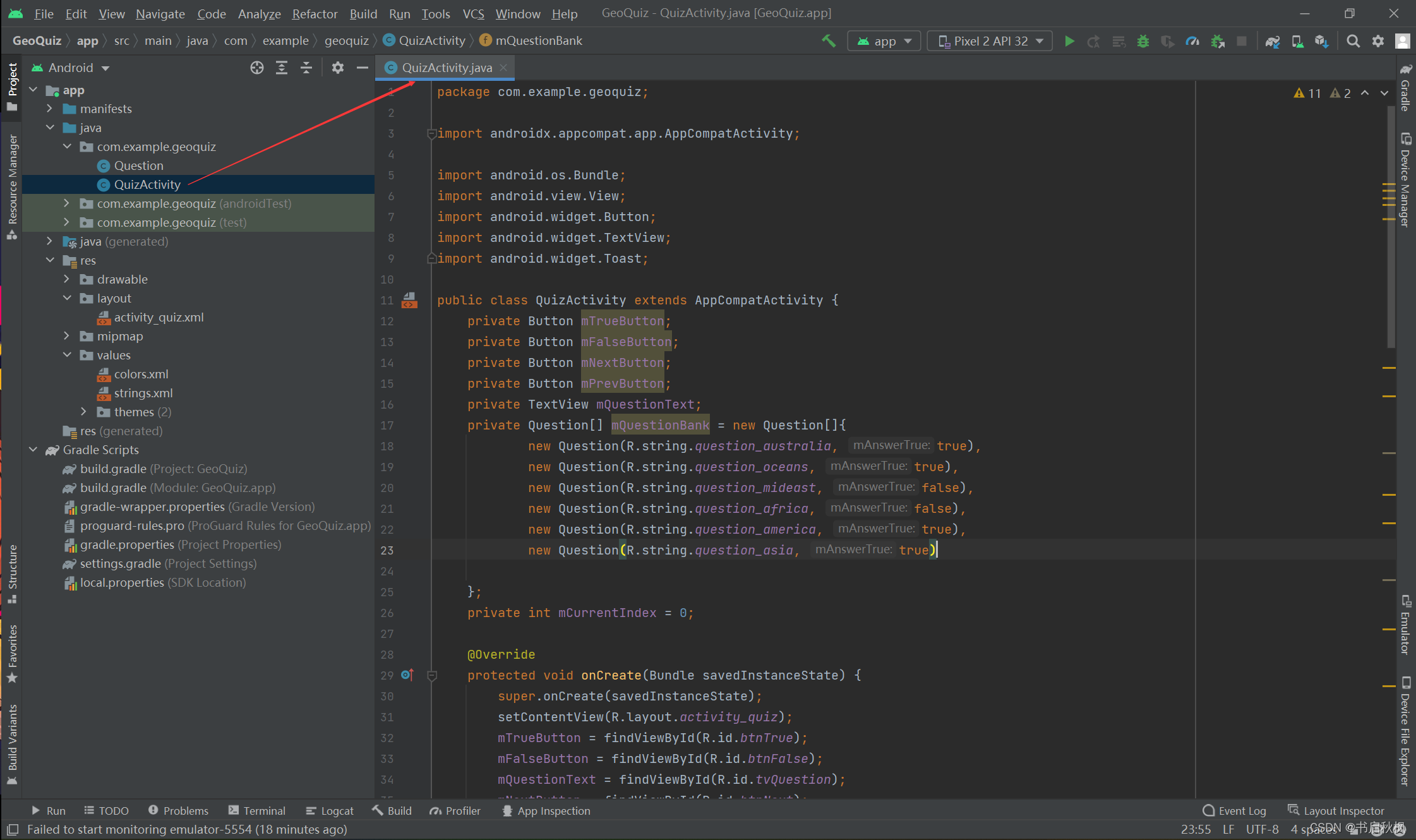
package com.example.geoquiz;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
public class QuizActivity extends AppCompatActivity {
private Button mTrueButton;
private Button mFalseButton;
private Button mNextButton;
private Button mPrevButton;
private TextView mQuestionText;
private Question[] mQuestionBank = new Question[]{
new Question(R.string.question_australia, true),
new Question(R.string.question_oceans, true),
new Question(R.string.question_mideast, false),
new Question(R.string.question_africa, false),
new Question(R.string.question_america, true),
new Question(R.string.question_asia, true)
};
private int mCurrentIndex = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_quiz);
mTrueButton = findViewById(R.id.btnTrue);
mFalseButton = findViewById(R.id.btnFalse);
mQuestionText = findViewById(R.id.tvQuestion);
mNextButton = findViewById(R.id.btnNext);
mPrevButton = findViewById(R.id.btnPrev);
mTrueButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
boolean answer = mQuestionBank[mCurrentIndex].isAnswerTrue();
if (answer == true)
Toast.makeText(QuizActivity.this,
"回答正确",
Toast.LENGTH_SHORT).show();
else
Toast.makeText(QuizActivity.this,
"回答错误",
Toast.LENGTH_SHORT).show();
// Toast.makeText(MainActivity.this,
// "Click True",
// Toast.LENGTH_SHORT).show();
}
});
mFalseButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
boolean answer = mQuestionBank[mCurrentIndex].isAnswerTrue();
if (answer == true)
Toast.makeText(QuizActivity.this,
"回答错误",
Toast.LENGTH_SHORT).show();
else
Toast.makeText(QuizActivity.this,
"回答正确",
Toast.LENGTH_SHORT).show();
// Toast.makeText(MainActivity.this,
// "Click False",
// Toast.LENGTH_SHORT).show();
}
});
mQuestionText.setText(mQuestionBank[mCurrentIndex].getTextResId());
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mCurrentIndex = (mCurrentIndex + 1) % mQuestionBank.length;
mQuestionText.setText(mQuestionBank[mCurrentIndex].getTextResId());
}
});
mPrevButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mCurrentIndex = (mCurrentIndex - 1);
if (mCurrentIndex < 0)
mCurrentIndex = 0;
mQuestionText.setText(mQuestionBank[mCurrentIndex].getTextResId());
}
});
}
}
5.效果展示 GeoQuiz
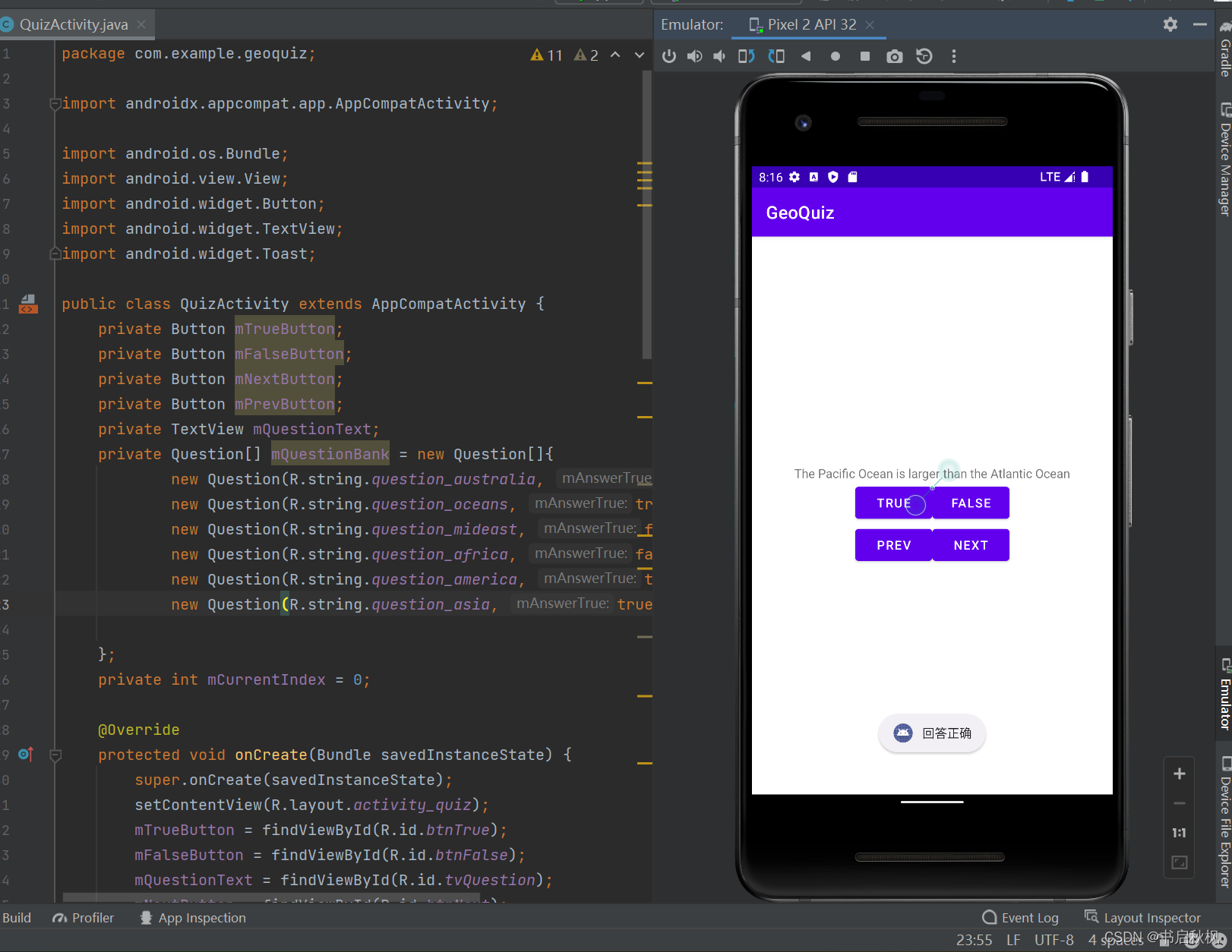
|