Android 5.0 新增 CardView,CardView 继承自 FrameLayout 类,可以设置圆角和阴影等。
使用示例
配置build.gradle
app\build.gradle
implementation 'androidx.cardview:cardview:1.0.0'
xml布局
app\src\main\res\layout\activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical"
>
<androidx.cardview.widget.CardView
android:id="@+id/test_cd"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_gravity="center"
app:cardBackgroundColor="#FFC0CB"
app:cardCornerRadius="10dp"
app:contentPadding="10dp"
app:cardElevation="10dp"
>
<RelativeLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/test_iv"
android:layout_width="200dp"
android:layout_height="200dp"
android:src="@drawable/pic_16"
/>
<TextView
android:id="@+id/test_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="hello world"
android:layout_marginTop="10dp"
android:layout_below="@+id/test_iv"
android:layout_centerInParent="true"
/>
</RelativeLayout>
</androidx.cardview.widget.CardView>
<LinearLayout
android:layout_marginTop="100dp"
android:layout_marginLeft="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="radius"
/>
<SeekBar
android:id="@+id/radius_sb"
android:layout_width="200dp"
android:layout_height="wrap_content"
/>
</LinearLayout>
<LinearLayout
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="elevation"
/>
<SeekBar
android:id="@+id/elevation_sb"
android:layout_width="200dp"
android:layout_height="wrap_content"
/>
</LinearLayout>
<LinearLayout
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="padding"
/>
<SeekBar
android:id="@+id/padding_sb"
android:layout_width="200dp"
android:layout_height="wrap_content"
/>
</LinearLayout>
</LinearLayout>
MainActivity
app\src\main\java\com\example\mycardviewtest\MainActivity.java
import androidx.appcompat.app.AppCompatActivity;
import androidx.cardview.widget.CardView;
import android.os.Bundle;
import android.util.Log;
import android.view.Window;
import android.widget.SeekBar;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "AAAAAA";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
supportRequestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
CardView cardView = findViewById(R.id.test_cd);
SeekBar radius_sb = findViewById(R.id.radius_sb);
radius_sb.setMax(200);
SeekBar elevation_sb = findViewById(R.id.elevation_sb);
elevation_sb.setMax(200);
SeekBar padding_sb = findViewById(R.id.padding_sb);
padding_sb.setMax(200);
radius_sb.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
Log.d(TAG, "onProgressChanged: " + progress);
cardView.setRadius(progress);
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) { }
@Override
public void onStopTrackingTouch(SeekBar seekBar) { }
});
elevation_sb.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
Log.d(TAG, "onProgressChanged: " + progress);
cardView.setCardElevation(progress);
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) { }
@Override
public void onStopTrackingTouch(SeekBar seekBar) { }
});
padding_sb.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
Log.d(TAG, "onProgressChanged: " + progress);
cardView.setContentPadding(progress, progress, progress, progress);
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) { }
@Override
public void onStopTrackingTouch(SeekBar seekBar) { }
});
}
}
效果
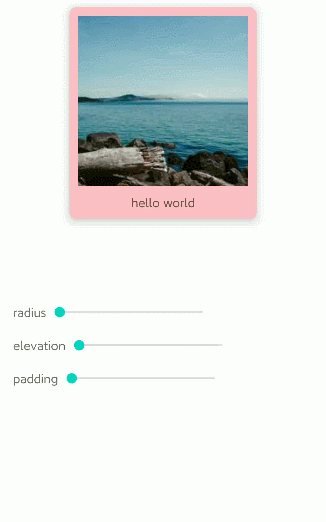
示例代码地址
示例代码:地址
|