Java代码部分
生成验证码
import javax.imageio.ImageIO;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.util.Random;
@WebServlet("/login")
public class CheckCode extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
int width=100;
int height=50;
BufferedImage image=new BufferedImage(width,height,BufferedImage.TYPE_INT_RGB);
Graphics g= image.getGraphics();
g.setColor(Color.PINK);
g.fillRect(0,0,width,height);
g.setColor(Color.black);
g.drawRect(0,0,width-1,height-1);
String str="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
Random ran=new Random();
StringBuilder sb=new StringBuilder();
for (int i = 1; i <= 4; i++) {
int index=ran.nextInt(str.length());
char c=str.charAt(index);
sb.append(c);
g.drawString(c+"",width/5*i,height/2);
}
String checkCode_session=sb.toString();
req.getSession().setAttribute("checkCode_session",checkCode_session);
g.setColor(Color.GREEN);
for (int i = 0; i < 10; i++) {
int x1= ran.nextInt(width);
int x2= ran.nextInt(width);
int y1= ran.nextInt(height);
int y2= ran.nextInt(height);
g.drawLine(x1,y1,x2,y2);
}
System.out.println(image+"123");
ImageIO.write(image,"jpg",resp.getOutputStream());
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
this.doPost(req, resp);
}
}
判断用户信息
先获取用户信息,然后判断验证码,用户名密码是否正确.(局限,未实现从数据库中获取信息,仅一位用户信息能实现登录)
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
@WebServlet("/login2")
public class Login extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("utf-8");
String username = req.getParameter("username");
String password = req.getParameter("password");
String checkCode = req.getParameter("checkCode");
HttpSession session = req.getSession();
String checkCode_session = (String) session.getAttribute("checkCode_session");
if (checkCode.equalsIgnoreCase(checkCode_session)) {
if ("张三".equals(username) && "123".equals(password)) {
session.setAttribute("user","张三");
resp.sendRedirect(req.getContextPath()+"/success.jsp");
} else {
req.setAttribute("login_error", "用户名或密码错误!");
req.getRequestDispatcher("/login.jsp").forward(req,resp);
}
} else {
req.setAttribute("error", "验证码错误!");
req.getRequestDispatcher("/login.jsp").forward(req, resp);
}
}
}
jsp部分
页面样式
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>login</title>
<style>
div {
color: red;
}
</style>
<script>
window.onload=function () {
document.getElementById("img").onclick=function (){
this.src="/untitled1_war_exploded/login?time"+new Date().getTime();
}
}
</script>
</head>
<body>
<form action="/untitled1_war_exploded/login2" method="post">
<table>
<tr>
<th>用户名</th>
<td><input type="text" name="username"></td>
</tr>
<tr>
<th>密码</th>
<td><input type="password" name="password"></td>
</tr>
<tr>
<th>验证码</th>
<td><input type="text" name="checkCode"></td>
</tr>
<tr>
<td colspan="2"><img id="img" src="/untitled1_war_exploded/login"></td>
</tr>
<tr>
<td colspan="2"><input type="submit" value="登录"></td>
</tr>
</table>
</form>
${requestScope.error}
${requestScope.login_error}
<%--<div><%=request.getAttribute("error")==null?"":request.getAttribute("error")%></div>--%>
<%--<div><%=request.getAttribute("login_error")==null?"":request.getAttribute("login_error")%></div>--%>
</body>
</html>
登录成功后页面
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1><%=request.getSession().getAttribute("user")%>,欢迎您!</h1>
</body>
</html>
效果
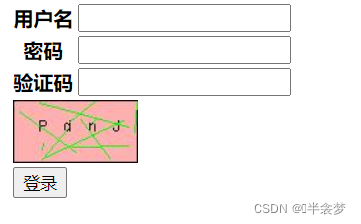 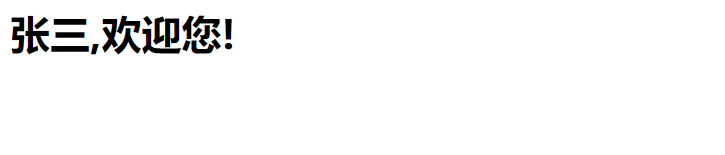 该部分从B站上学习
|