最近做首页新手引导需要显示一个在控件下面的弹框,由于之前的同事使用的是FramLayout以addview的方式实现的,发现很不好扩展,修改控件位置及其他需求比较麻烦,于是决定用PopupWindow重新写,直接上代码:
1.浮窗显示在控件底部:
调用showAsDropDown()方法,源码翻译出来大致意思就是显示固定位置的视图
2.初始化
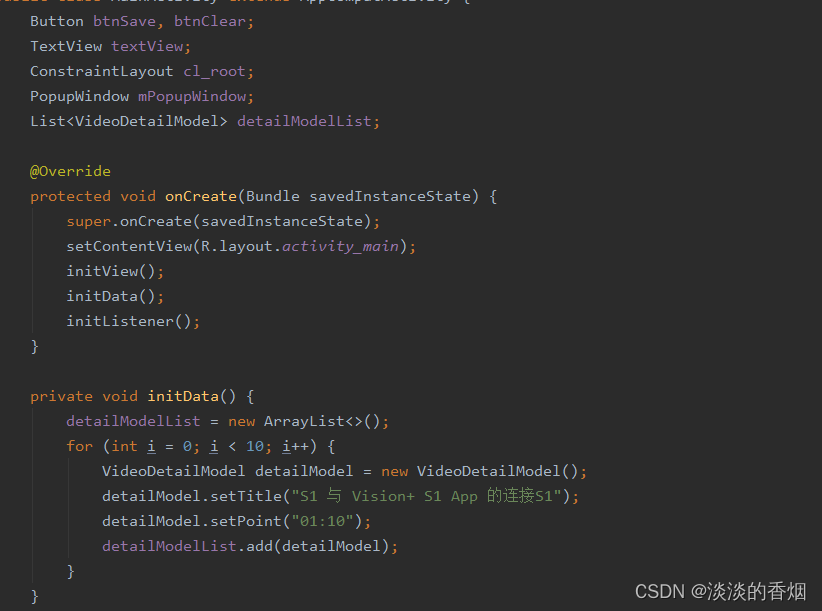
Button btnSave, btnClear;
TextView textView;
ConstraintLayout cl_root;
PopupWindow mPopupWindow;
List<VideoDetailModel> detailModelList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
initData();
initListener();
}
private void initData() {
detailModelList = new ArrayList<>();
for (int i = 0; i < 10; i++) {
VideoDetailModel detailModel = new VideoDetailModel();
detailModel.setTitle("S1 与 Vision+ S1 App 的连接S1");
detailModel.setPoint("01:10");
detailModelList.add(detailModel);
}
}
private void initView() {
btnSave = findViewById(R.id.btn_save);
btnClear = findViewById(R.id.btn_clear);
cl_root = findViewById(R.id.cl_root);
}
?3.浮窗在按钮下面弹出:
btnBottom.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mPopupWindow = new TipPopupWinDow(MainActivity.this, ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.WRAP_CONTENT, true);
//浮框在按钮下面
showPopUpWindow(v);
setBackgroundAlpha(MainActivity.this, 0.6f);
}
});

private void showPopUpWindow(View v) {
mPopupWindow.showAsDropDown(v);
mPopupWindow.setOnDismissListener(new PopupWindow.OnDismissListener() {
@Override
public void onDismiss() {
setBackgroundAlpha(MainActivity.this, 1.0f);
}
});
}
4、浮窗在按钮右边弹出
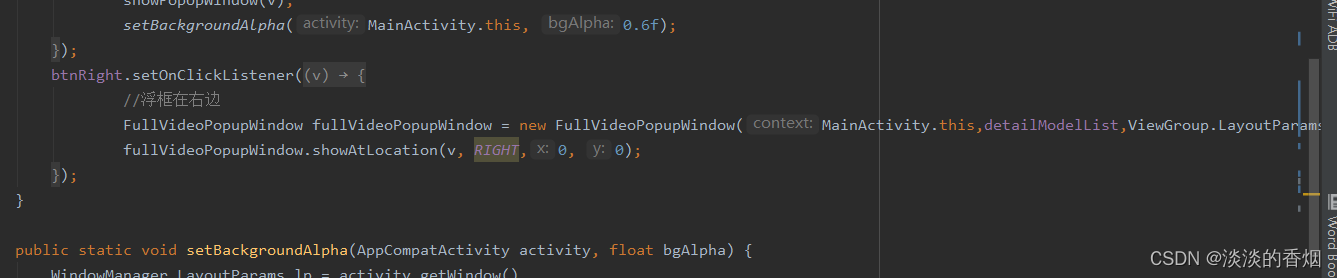
btnRight.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//浮框在右边
FullVideoPopupWindow fullVideoPopupWindow = new FullVideoPopupWindow(MainActivity.this,detailModelList,ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.MATCH_PARENT);
fullVideoPopupWindow.showAtLocation(v, RIGHT,0, 0);
}
});
5.浮窗从按钮左边弹出:
btnLeft.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//浮框在左边
FullVideoPopupWindow fullVideoPopupWindow = new FullVideoPopupWindow(MainActivity.this, detailModelList, ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.MATCH_PARENT);
fullVideoPopupWindow.showAtLocation(v, Gravity.START,0, 0);
}
});
6.MainActivity:完整代码:
public class MainActivity extends AppCompatActivity {
Button btnBottom,btnLeft, btnRight;
ConstraintLayout cl_root;
PopupWindow mPopupWindow;
List<VideoDetailModel> detailModelList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
initData();
initListener();
}
private void initData() {
detailModelList = new ArrayList<>();
for (int i = 0; i < 10; i++) {
VideoDetailModel detailModel = new VideoDetailModel();
detailModel.setTitle("S1 与 Vision+ S1 App 的连接S1");
detailModel.setPoint("01:10");
detailModelList.add(detailModel);
}
}
private void initView() {
btnBottom = findViewById(R.id.btn_bottom);
btnLeft = findViewById(R.id.btn_left);
btnRight = findViewById(R.id.btn_right);
cl_root = findViewById(R.id.cl_root);
}
private void initListener() {
btnLeft.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//浮框在左边
FullVideoPopupWindow fullVideoPopupWindow = new FullVideoPopupWindow(MainActivity.this, detailModelList, ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.MATCH_PARENT);
fullVideoPopupWindow.showAtLocation(v, Gravity.START,0, 0);
}
});
btnBottom.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mPopupWindow = new TipPopupWinDow(MainActivity.this, ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.WRAP_CONTENT, true);
//浮框在按钮下面
showPopUpWindow(v);
setBackgroundAlpha(MainActivity.this, 0.6f);
}
});
btnRight.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//浮框在右边
FullVideoPopupWindow fullVideoPopupWindow = new FullVideoPopupWindow(MainActivity.this,detailModelList,ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.MATCH_PARENT);
fullVideoPopupWindow.showAtLocation(v, RIGHT,0, 0);
}
});
}
public static void setBackgroundAlpha(AppCompatActivity activity, float bgAlpha) {
WindowManager.LayoutParams lp = activity.getWindow()
.getAttributes();
lp.alpha = bgAlpha;
(activity).getWindow().setAttributes(lp);
}
private void showPopUpWindow(View v) {
mPopupWindow.showAsDropDown(v);
mPopupWindow.setOnDismissListener(new PopupWindow.OnDismissListener() {
@Override
public void onDismiss() {
setBackgroundAlpha(MainActivity.this, 1.0f);
}
});
}
}
?7.布局代码:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/cl_root"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_left"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="@dimen/dp_20"
android:layout_marginEnd="@dimen/dp_20"
android:background="@color/colorPrimary"
android:text="从按钮左边弹出"
android:textColor="@color/white"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintLeft_toRightOf="@id/btn_bottom"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/btn_bottom"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="@dimen/dp_20"
android:background="@color/colorPrimary"
android:text="从按钮下面弹出"
android:textColor="@color/white"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toRightOf="@id/btn_left"
app:layout_constraintRight_toLeftOf="@id/btn_right"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/btn_right"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="@dimen/dp_20"
android:layout_marginEnd="@dimen/dp_20"
android:background="@color/colorPrimary"
android:text="从按钮右边弹出"
android:textColor="@color/white"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toRightOf="@id/btn_bottom"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
8.底部弹框代码:
/**
* @author: njb
* @date: 2022/2/17 14:53
* @desc: 引导弹框
*/
public class TipPopupWinDow extends PopupWindow {
private View view;
FrameLayout flTip;
public TipPopupWinDow(Activity context,int width, int height, boolean focusable){
view = LayoutInflater.from(context).inflate(R.layout.layout_tip_popup_window, null);
flTip = view.findViewById(R.id.fl_tip);
setContentView(view);
setTouchable(true);
setHeight(height);
setWidth(width);
setOutsideTouchable(true);
initView();
}
private void initView() {
flTip.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
TipPopupWinDow.this.dismiss();
}
});
}
}
9.左右弹框代码:
/**
* @author: njb
* @date: 2022/3/14 17:39
* @desc: 描述
*/
public class FullVideoPopupWindow extends PopupWindow {
private List<VideoDetailModel> videoDetails;
private RecyclerView recyclerView;
private FullVideoDetailAdapter fullVideoDetailAdapter;
private Activity context;
private View view;
private LinearLayoutManager centerLayoutManager;
private ConstraintLayout clVideo;
public FullVideoPopupWindow(@NonNull Activity context, List<VideoDetailModel> videoDetails,int width,int height) {
super(context);
this.context = context;
this.videoDetails = videoDetails;
view = LayoutInflater.from(context).inflate(R.layout.popup_full_video, null);
setContentView(view);
this.setBackgroundDrawable(null);
setWidth(width);
setHeight(height);
setOutsideTouchable(true);
setTouchable(true);
initView();
}
private void initView() {
recyclerView = view.findViewById(R.id.rv_full_video);
clVideo = view.findViewById(R.id.cl_full_root);
fullVideoDetailAdapter = new FullVideoDetailAdapter(context,videoDetails);
centerLayoutManager = new LinearLayoutManager(context,LinearLayoutManager.VERTICAL,false);
recyclerView.setLayoutManager(new LinearLayoutManager(context));
recyclerView.setAdapter(fullVideoDetailAdapter);
clVideo.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
FullVideoPopupWindow.this.dismiss();
}
});
}
}
10.实现的效果如下:
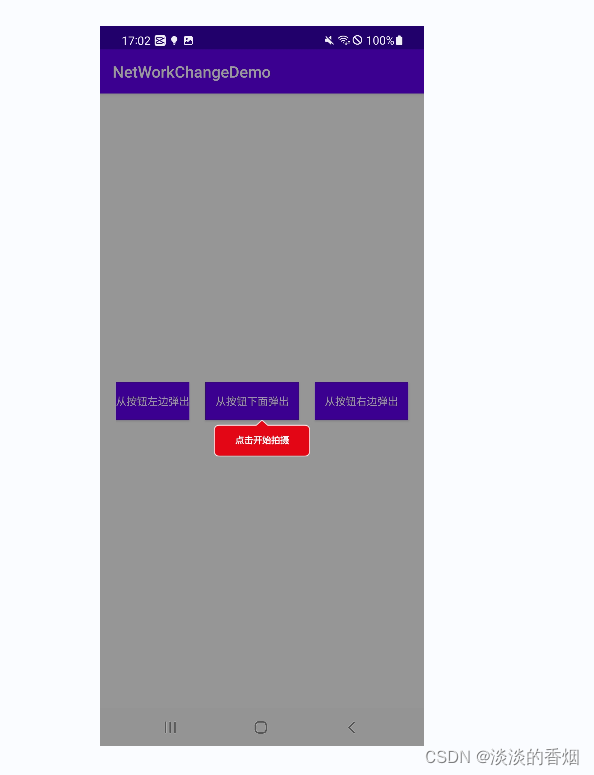
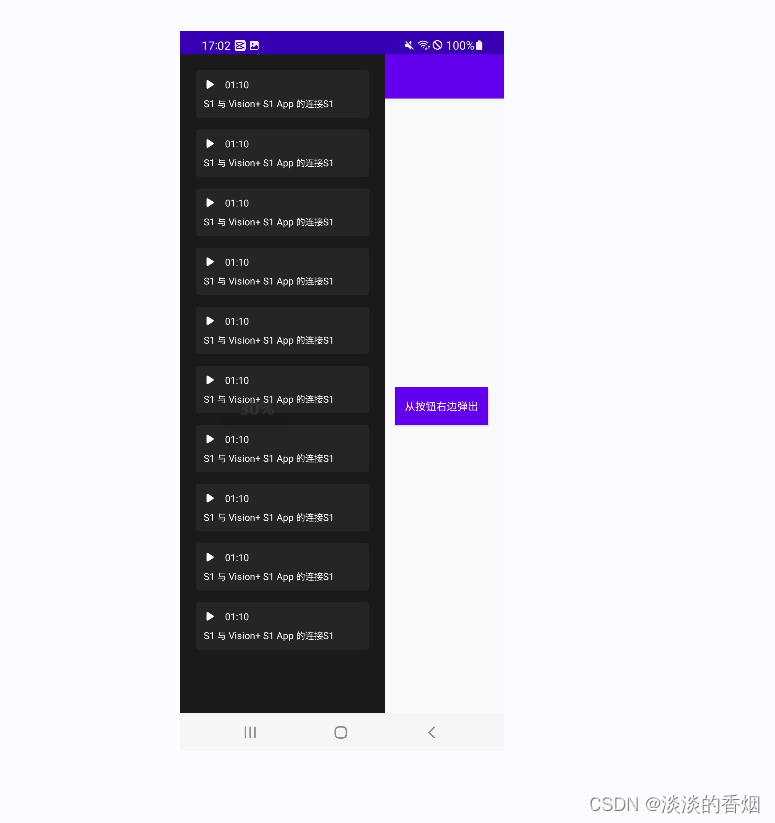
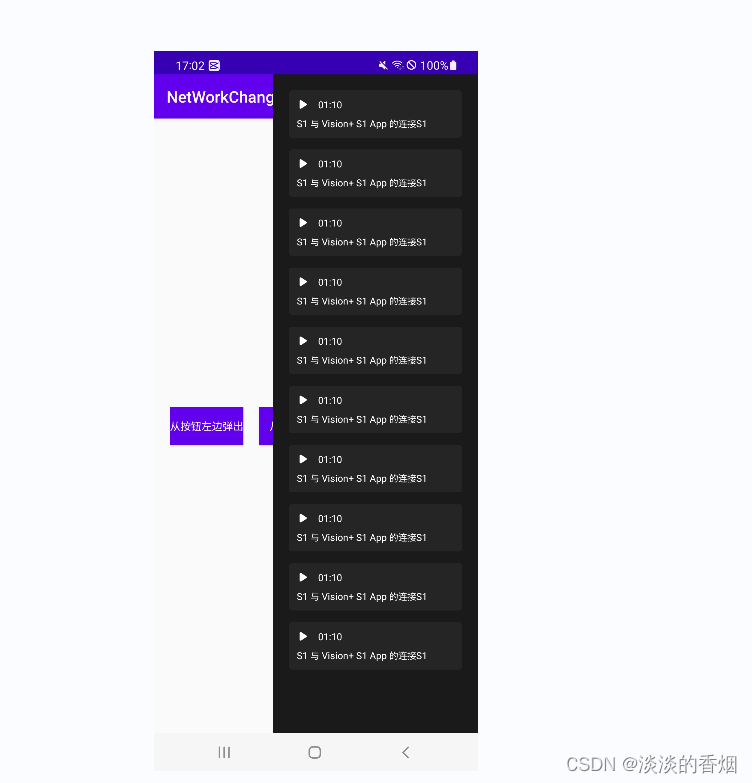
最后项目的源码如下:PopupWindowDemo: PopUpWindow的简单使用
|