Blazor框架的表单验证方便快捷:EditForm+模型绑定+数据注解(Data Annotations)
自定义表单验证可以支持更加复杂的输入验证规则,学习体验如下:
实现的效果如下图所示:
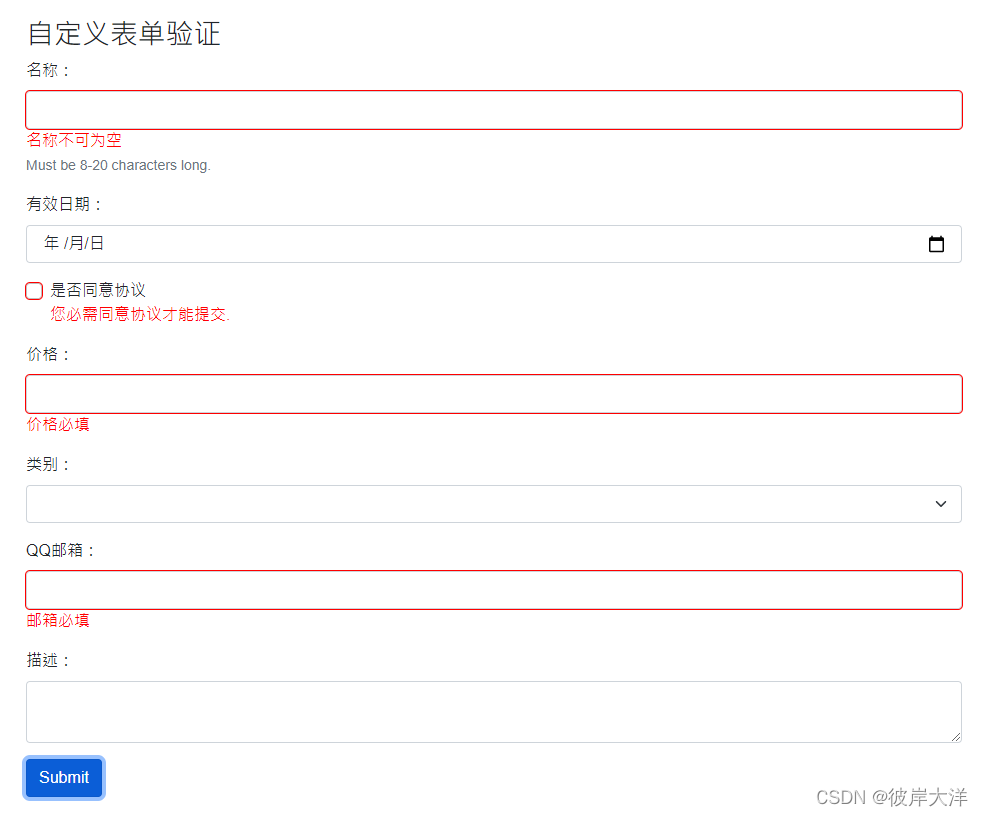
如果输入的不是qq邮箱,或者 选择某个类型时,描述必填的业务验证:
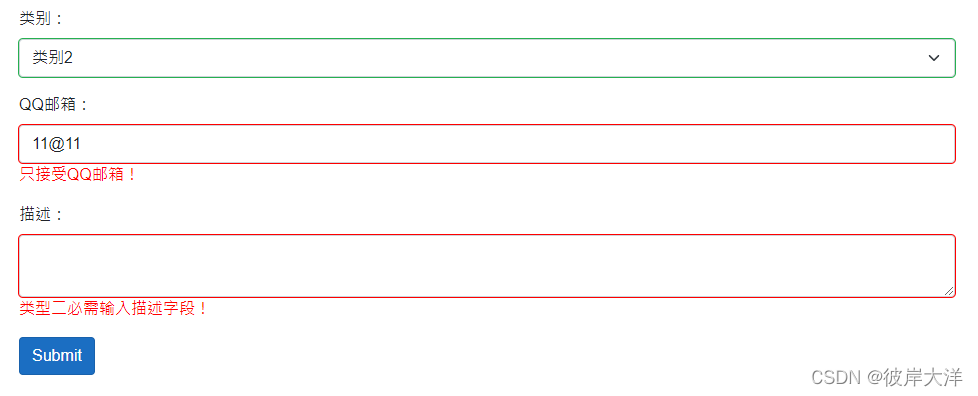
?实现的代码如下:
1:模型数据注解添加:
public class DemoDataModel
{
[Required(ErrorMessage ="名称不可为空")]
[StringLength(maximumLength:20, ErrorMessage ="名称太长,可输入最大20个字符长度")]
public string ModelName { get; set; }
[Required(ErrorMessage = "价格必填")]
public float? Price { get; set; }
[Required(ErrorMessage = "邮箱必填")]
public string Email { get; set; }
public DateTime? ValidDataTime { get; set; }
[Required]
[Range(typeof(bool), "true", "true",ErrorMessage = "您必需同意协议才能提交.")]
public bool IsChecked { get; set; }
public int ModelCategory { get; set; }
public string Description { get; set; }
}
?2:自定义验证组件LearnCustomValidator:
public class LearnCustomValidator : ComponentBase
{
private ValidationMessageStore messageStore;
[CascadingParameter]
private EditContext CurrentEditContext { get; set; }
protected override void OnInitialized()
{
if (CurrentEditContext == null)
{
throw new InvalidOperationException($"{nameof(LearnCustomValidator)} requires a cascading " +
$"parameter of type {nameof(EditContext)}. ");
}
messageStore = new(CurrentEditContext);
CurrentEditContext.OnValidationRequested += (s, e) =>
messageStore.Clear();
CurrentEditContext.OnFieldChanged += (s, e) =>
messageStore.Clear(e.FieldIdentifier);
}
public void DisplayErrors(Dictionary<string, List<string>> errors=null)
{
if (errors != null)
{
foreach (var err in errors)
{
messageStore.Add(CurrentEditContext.Field(err.Key), err.Value);
}
}
CurrentEditContext.NotifyValidationStateChanged();
}
public void ClearErrors()
{
messageStore.Clear();
CurrentEditContext.NotifyValidationStateChanged();
}
public void AddTestError(string filedName,string error="这个是一条测试验证提示")
{
messageStore.Add(CurrentEditContext.Field(filedName), error);
}
}
3: 自定义验证组件的使用:
@page "/formdemo"
<h3>自定义表单验证</h3>
<EditForm Model="@dataModel" OnValidSubmit="@HandleValidSubmit">
<DataAnnotationsValidator></DataAnnotationsValidator>
<LearnCustomValidator @ref="customValidation" />
@*<ValidationSummary />*@
<div class="mb-3">
<div class="col-auto">
<label for="modelName" class="form-label">名称:</label>
</div>
<div class="col-auto">
<InputText id="modelName" class="form-control" @bind-Value="dataModel.ModelName" />
</div>
<div class="col-auto">
<ValidationMessage For="@(() => dataModel.ModelName)" />
<span id="modelNameHelpInline" class="form-text">
Must be 8-20 characters long.
</span>
</div>
</div>
<div class="mb-3">
<div class="col-auto">
<label for="modelDate" class="form-label">有效日期:</label>
</div>
<div class="col-auto">
<InputDate id="modelDate" class="form-control" @bind-Value="dataModel.ValidDataTime" @bind-Value:format="yyyy-MM-dd HH:mm:ss"/>
</div>
</div>
<div class="mb-3 form-check">
<InputCheckbox class="form-check-input" id="exampleCheck1" @bind-Value="dataModel.IsChecked" />
<label class="form-check-label" for="exampleCheck1">是否同意协议</label>
<ValidationMessage For="@(() => dataModel.IsChecked)" />
</div>
<div class="mb-3">
<label for="price" class="form-label">价格:</label>
<InputNumber id="price" class="form-control" @bind-Value="dataModel.Price" @bind-Value:format="{0:c2}" ParsingErrorMessage="价格不能为空" />
<ValidationMessage For="@(() => dataModel.Price)" />
</div>
<div class="mb-3">
<label for="category" class="form-label">类别:</label>
<InputSelect id="category" class="form-select" @bind-Value="dataModel.ModelCategory">
<option value="1">类别1</option>
<option value="2">类别2</option>
<option value="3">类别3</option>
<option value="4">类别4</option>
</InputSelect>
</div>
<div class="mb-3">
<label for="email" class="form-label">QQ邮箱:</label>
<InputText id="email" type="email" class="form-control" @bind-Value="dataModel.Email" />
<ValidationMessage For="@(() => dataModel.Email)" />
</div>
<div class="mb-3">
<label for="desc" class="form-label">描述:</label>
<InputTextArea id="desc" class="form-control" @bind-Value="dataModel.Description" />
<ValidationMessage For="@(() => dataModel.Description)" />
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</EditForm>
@code {
private LearnCustomValidator customValidation;
private DemoDataModel dataModel;
protected override void OnInitialized()
{
dataModel = new();
base.OnInitialized();
}
void HandleValidSubmit()
{
if (!dataModel.Email.Contains("qq.com"))
{
customValidation.AddTestError(nameof(dataModel.Email), "只接受QQ邮箱!");
}
if (dataModel.ModelCategory == 2 && string.IsNullOrEmpty(dataModel.Description))
{
customValidation.AddTestError(nameof(dataModel.Description), "类型二必需输入描述字段!");
}
customValidation.DisplayErrors();
return;
}
}
?参考MSDN文档:ASP.NET Core Blazor forms and validation
|