一. 文件分类
① 文本文件
文件以文本的ASCII码 形式存储在计算机中
② 二进制文件
文件以文本的二进制的形式存储在计算机中,用户一般不能直接读懂它们.存放的都是0和1这些二进制数
二. 操作文件的三大类:
① ofstream
- 写操作 o-> out的意思, out针对的是内存,从内存输出到文件,所以是写操作.
- 继承自
ostream
② ifstream
- 读操作 i-> in的意思,in针对的是内存,从文件到内存,所以是读操作
- 继承自
istream
③ fstream
- out and in 读写操作
- 继承自
iostream
三. 写文件的操作步骤
① 写文件的步骤
- 包含头文件
#include <fstream> - 创建流对象
ofstream ofs ; 写是从内存输出到文件 - 打开文件
ofs.open("文件路径",打开方式) - 写数据,往输出流中写入数据
ofs << "写入的数据;" - 关闭文件
ofs.close();
② 文件打开的方式
打开方式 | 解释 |
---|
ios::in | 以只读的方式打开文件 | ios::out | 以只写的方式打开文件 | ios::ate | 初始位置: 文件末尾 | ios::app | 追加的方式写文件 | ios::trunc | 如果文件存在先删除,再创建 | ios::binary | 二进制方式 |
注意:
文件的打开方式可以配合使用,利用| 操作符 例如: 用二进制方式写文件 ios::binary | ios::out
③ 文本方式写文件的例子
#include <iostream>
#include <fstream>
using namespace std;
void write_test_01()
{
ofstream ofs;
ofs.open("test.txt", ios::out);
ofs << "姓名: 张三" << endl;
ofs << "性别: 男\n";
ofs << "年龄: 18" << endl;
}
int main()
{
write_test_01();
system("pause");
return 0;
}
结果: 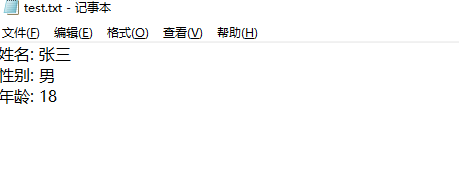
四. 读文件的操作
① 读文件的步骤
- 包含头文件
#include<fstream> - 创建流对象
ifstream 输入流,输入到内存 - 打开文件并判断文件是否打开成功
ifs.open("文件路径",打开方式) - 读取数据
- 关闭文件
② 读取文件的例子
读取文件到一个字符数组里面,有一个问题,就是遇到空格,换行符,或者是制表符会返回.
#include <iostream>
#include <fstream>
using namespace std;
void read_file_test(void)
{
ifstream ifs;
ifs.open("test.txt", ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
}
char buf[1024] = { 0 };
while (ifs >> buf)
{
cout << buf << endl;
}
ifs.close();
}
int main()
{
read_file_test();
system("pause");
return 0;
}
文件的内容: 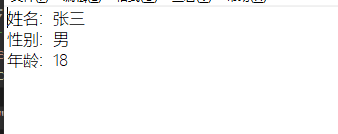 程序运行的结果: 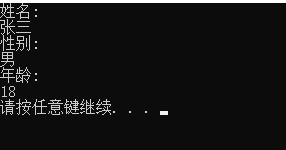 读取到buf中,使用ifstream里面的getline()函数,遇到换行符会返回
#include <iostream>
#include <fstream>
using namespace std;
void read_file_test(void)
{
fstream ifs;
ifs.open("test.txt", ios::in);
if (!ifs.is_open())
{
cout << "文件不存在,打开失败!" << endl;
}
char buf[1024] = { 0 };
while (ifs.getline(buf,sizeof(buf)))
{
cout << buf << endl;
}
ifs.close();
}
int main()
{
read_file_test();
system("pause");
return 0;
}
结果: 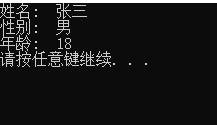
将数据读入到string中,使用全局的getline函数,需要输入流.也是一行一行的方式读取出来
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
void read_file_test(void)
{
ifstream ifs;
ifs.open("test.txt", ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败" << endl;
}
string buf;
while (getline(ifs, buf))
{
cout << buf << endl;
}
ifs.close();
}
int main()
{
read_file_test();
system("pause");
return 0;
}
结果: 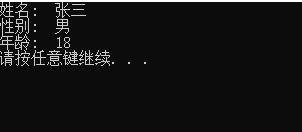 一个字符一个字符的读取,判断是否读取到文件尾, EOF 代表文件的尾部
#include <iostream>
#include <fstream>
using namespace std;
void read_file_test(void)
{
ifstream ifs;
ifs.open("test.txt", ios::in);
if (!ifs.is_open())
{
cout << "文件打开失败!" << endl;
return;
}
char c;
while ((c = ifs.get()) != EOF)
{
cout << c;
}
ifs.close();
}
int main()
{
read_file_test();
system("pause");
return 0;
}
注意,这里会把所有的字符读取出来,包括换行符,空格和制表符,但是这种效率太低,一般不建议使用!
五. 二进制写文件
- 二进制写文件主要利用流对象调用成员函数
write 原型 : ostream& write(const char* buffer,int len) - 参数解释: 字符指针
buffer 指向内存中一段存储空间.len 是读写的字节数
#include <iostream>
#include <fstream>
using namespace std;
class Person
{
public:
char mName[64];
int mAge;
};
void write_file_binary_test(void)
{
Person p = { "张三",18 };
ofstream ofs;
ofs.open("person.txt", ios::out | ios::binary);
ofs.write((const char *)&p, sizeof(p));
ofs.close();
}
int main()
{
write_file_binary_test();
system("pause");
return 0;
}
六. 二进制读文件
- 二进制方式读取文件主要利用流对象调用成员函数
read 原型: isttream& read(char* buffer,int len) - 字符指针buffer指向内存中的一段存储空间.len是读写的字节数
#include <iostream>
#include <fstream>
using namespace std;
class Person
{
public:
char mName[64];
int mAge;
};
void read_file_binary_test(void)
{
ifstream ifs;
ifs.open("person.txt", ios::in | ios::binary);
if (!ifs.is_open())
{
cout << "文件打开失败" << endl;
return;
}
Person p;
ifs.read((char *)&p, sizeof(Person));
cout << "姓名: " << p.mName << " 年龄: " << p.mAge << endl;
ifs.close();
}
int main()
{
read_file_binary_test();
system("pause");
return 0;
}
|