一般我们这样设置圆角
tempView.layer.cornerRadius = 10
tempView.layer.masksToBounds = true
当我们对UITableView做性能优化时,上面的方式容易触发离屏渲染给我们带来额外的性能损耗,所以这里还可以使用贝塞尔曲线UIBezierPath和Core Graphics框架画出一个圆角。
import Foundation
import UIKit
extension UIView {
func cornerRadius(radius: CGFloat, corners: UIRectCorner) {
let bezierPath = UIBezierPath(roundedRect: self.bounds, byRoundingCorners: corners, cornerRadii: CGSize(width: radius, height: radius))
let radiusLayer = CAShapeLayer()
radiusLayer.frame = self.bounds
radiusLayer.path = bezierPath.cgPath
self.layer.mask = radiusLayer
}
func cornerRadius(radius: CGFloat, corners: UIRectCorner, color: UIColor, lineWidth: CGFloat) {
let bezierPath = UIBezierPath(roundedRect: self.bounds, byRoundingCorners: corners, cornerRadii: CGSize(width: radius, height: radius))
let radiusLayer = CAShapeLayer()
radiusLayer.frame = self.bounds
radiusLayer.path = bezierPath.cgPath
self.layer.mask = radiusLayer
let borderLayer = CAShapeLayer()
borderLayer.frame = self.bounds
borderLayer.path = bezierPath.cgPath
borderLayer.lineWidth = lineWidth
borderLayer.strokeColor = color.cgColor
borderLayer.fillColor = UIColor.clear.cgColor
if let layers: NSArray = self.layer.sublayers as? NSArray {
if let last = layers.lastObject as? AnyObject {
if last.isKind(of: CAShapeLayer.self) {
last.removeFromSuperlayer()
}
}
}
self.layer.addSublayer(borderLayer)
}
func cornerRadius(radius: CGFloat, corners: UIRectCorner, bounds: CGRect) {
let bezierPath = UIBezierPath(roundedRect: bounds, byRoundingCorners: corners, cornerRadii: CGSize(width: radius, height: radius))
let radiusLayer = CAShapeLayer()
radiusLayer.frame = bounds
radiusLayer.path = bezierPath.cgPath
self.layer.mask = radiusLayer
}
}
具体使用
import Foundation
class LiveShopListCell: UITableViewCell {
var model: LiveDealsModel? {
didSet {
guard let vo = model else {
return
}
DispatchQueue.global().async {
DispatchQueue.main.async { [weak self] in
self?.bgView.cornerRadius(radius: 10, corners: .allCorners)
self?.shopIV.cornerRadius(radius: 6, corners: .allCorners)
self?.aBulkDiscountBut.cornerRadius(radius: 3, corners: .allCorners, color: R.color.themeColor()!, lineWidth: 0.5)
}
}
}
}
override func awakeFromNib() {
super.awakeFromNib()
self.selectionStyle = .none
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
}
示意图 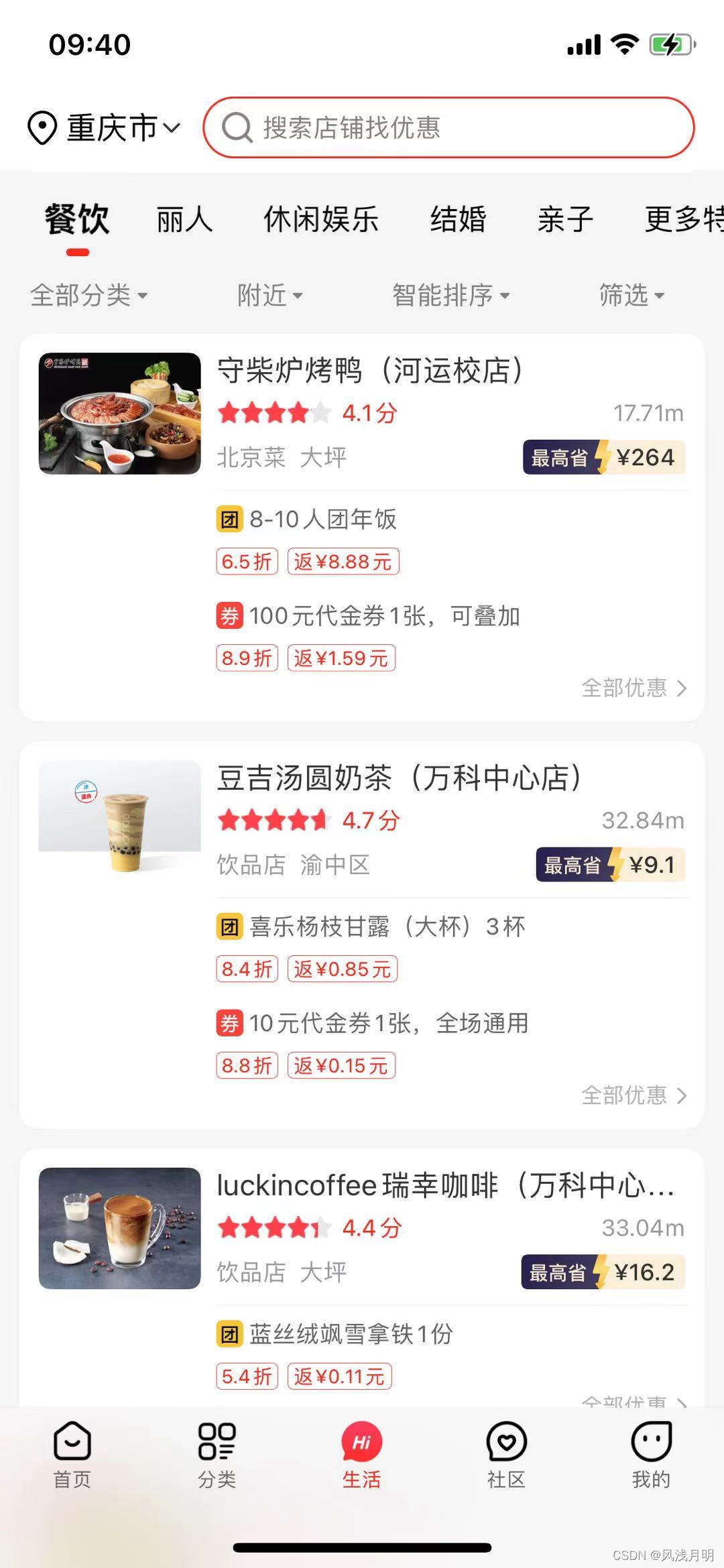
|