阅读源码目标
- 了解底层原理,有助于使用API,排查问题
- 学习设计模式
- 学习系统架构
- 面试
功能及原理
功能
- 同步请求
- 异步请求
- WebSocket
- 自定义拦截器处理缓存,重定向,请求体管理Bridge,链接管理
核心流程 RealCall 保存了拦截器List
@Throws(IOException::class)
internal fun getResponseWithInterceptorChain(): Response {
val interceptors = mutableListOf<Interceptor>()
interceptors += client.interceptors
interceptors += RetryAndFollowUpInterceptor(client)
interceptors += BridgeInterceptor(client.cookieJar)
interceptors += CacheInterceptor(client.cache)
interceptors += ConnectInterceptor
......
}
RealCall 发起请求后构建RealInterceptorChain对象,构造方法传入连接器List和当前访问的Index。
val chain = RealInterceptorChain(
call = this,
interceptors = interceptors,
index = 0,
)
执行val response = chain.proceed(originalRequest),该方法会进入到如下逻辑:
val next = copy(index = index + 1, request = request)
val interceptor = interceptors[index]
@Suppress("USELESS_ELVIS")
val response = interceptor.intercept(next) ?: throw NullPointerException(
"interceptor $interceptor returned null")
在提供的几种Intercept中会执行自身拦截处理逻辑,调用chain.proceed返回response,将责任链继续传导下去。 整体责任链其实是一个递归模式,假如拦截器List中是ABCD,先执行A的request的处理,再调用chain.proceed,继而执行B的request的处理,直到CD,接下来再从D一步步返回A,处理Response。 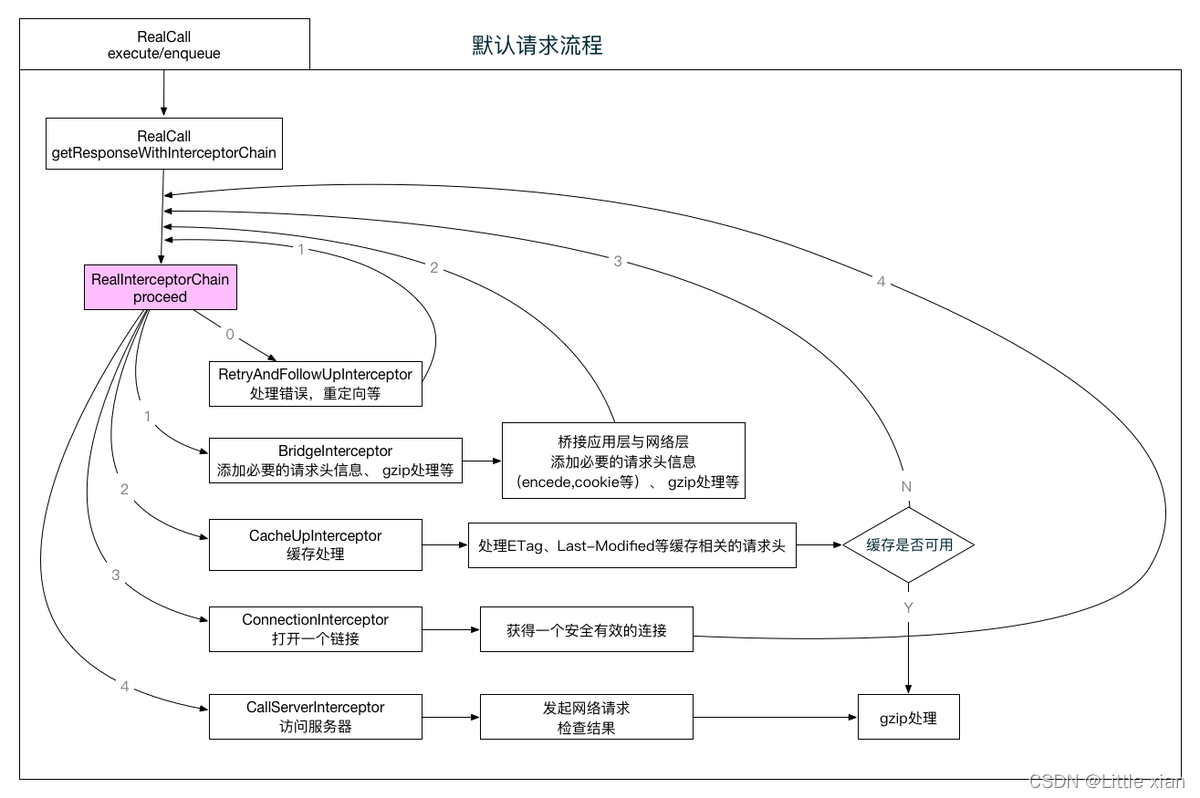 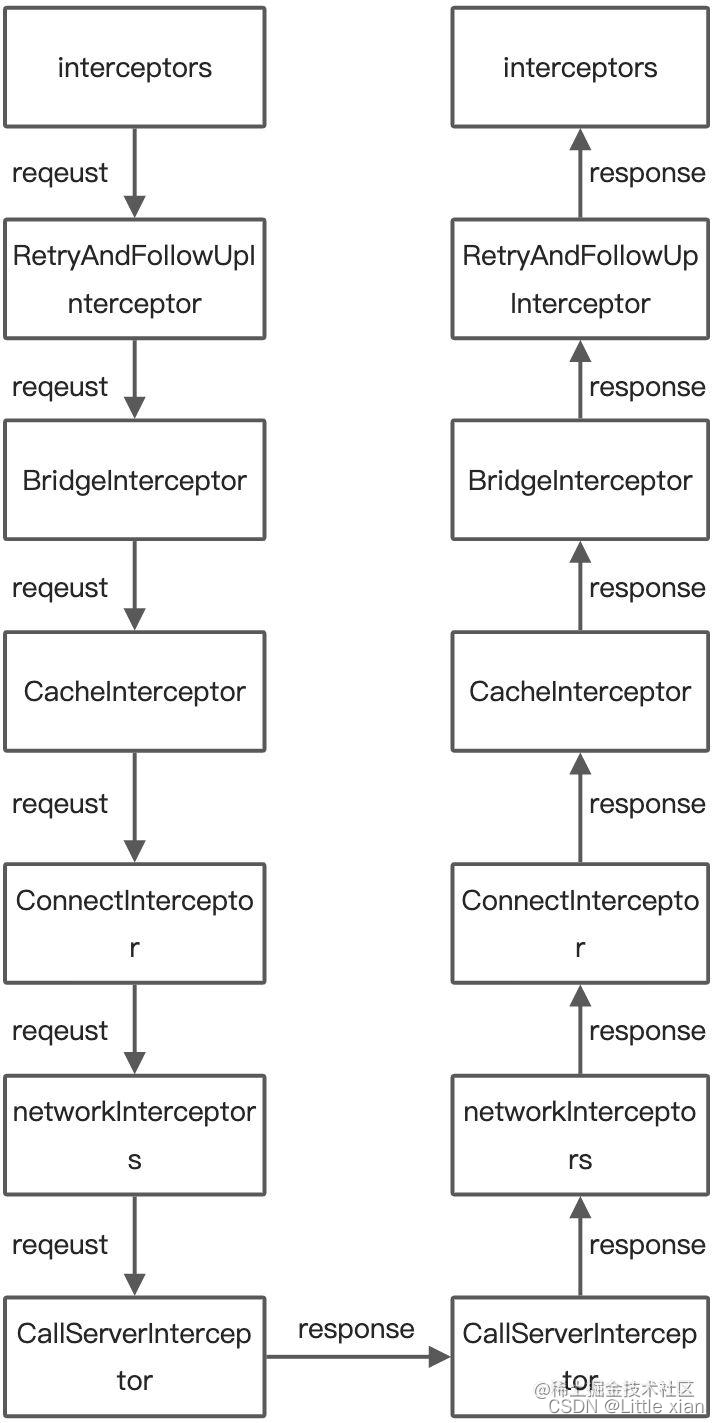
核心代码
Response getResponseWithInterceptorChain() throws IOException {
List<Interceptor> interceptors = new ArrayList<>();
interceptors.addAll(client.interceptors());
interceptors.add(new RetryAndFollowUpInterceptor(client));
interceptors.add(new BridgeInterceptor(client.cookieJar()));
interceptors.add(new CacheInterceptor(client.internalCache()));
interceptors.add(new ConnectInterceptor(client));
if (!forWebSocket) {
interceptors.addAll(client.networkInterceptors());
}
interceptors.add(new CallServerInterceptor(forWebSocket));
Interceptor.Chain chain = new RealInterceptorChain(interceptors, transmitter, null, 0,
originalRequest, this, client.connectTimeoutMillis(),
client.readTimeoutMillis(), client.writeTimeoutMillis());
boolean calledNoMoreExchanges = false;
try {
Response response = chain.proceed(originalRequest);
if (transmitter.isCanceled()) {
closeQuietly(response);
throw new IOException("Canceled");
}
return response;
} catch (IOException e) {
calledNoMoreExchanges = true;
throw transmitter.noMoreExchanges(e);
} finally {
if (!calledNoMoreExchanges) {
transmitter.noMoreExchanges(null);
}
}
}
设计模式
建造者模式: client 的build 工厂模式:EventListener.Factory,SocketFactory 责任链模式:简单的说,就是让每个对象都能有机会处理这个请求,然后各自完成自己的事情,一直到事件被处理。Android中的事件分发机制也是用到了这种设计模式。
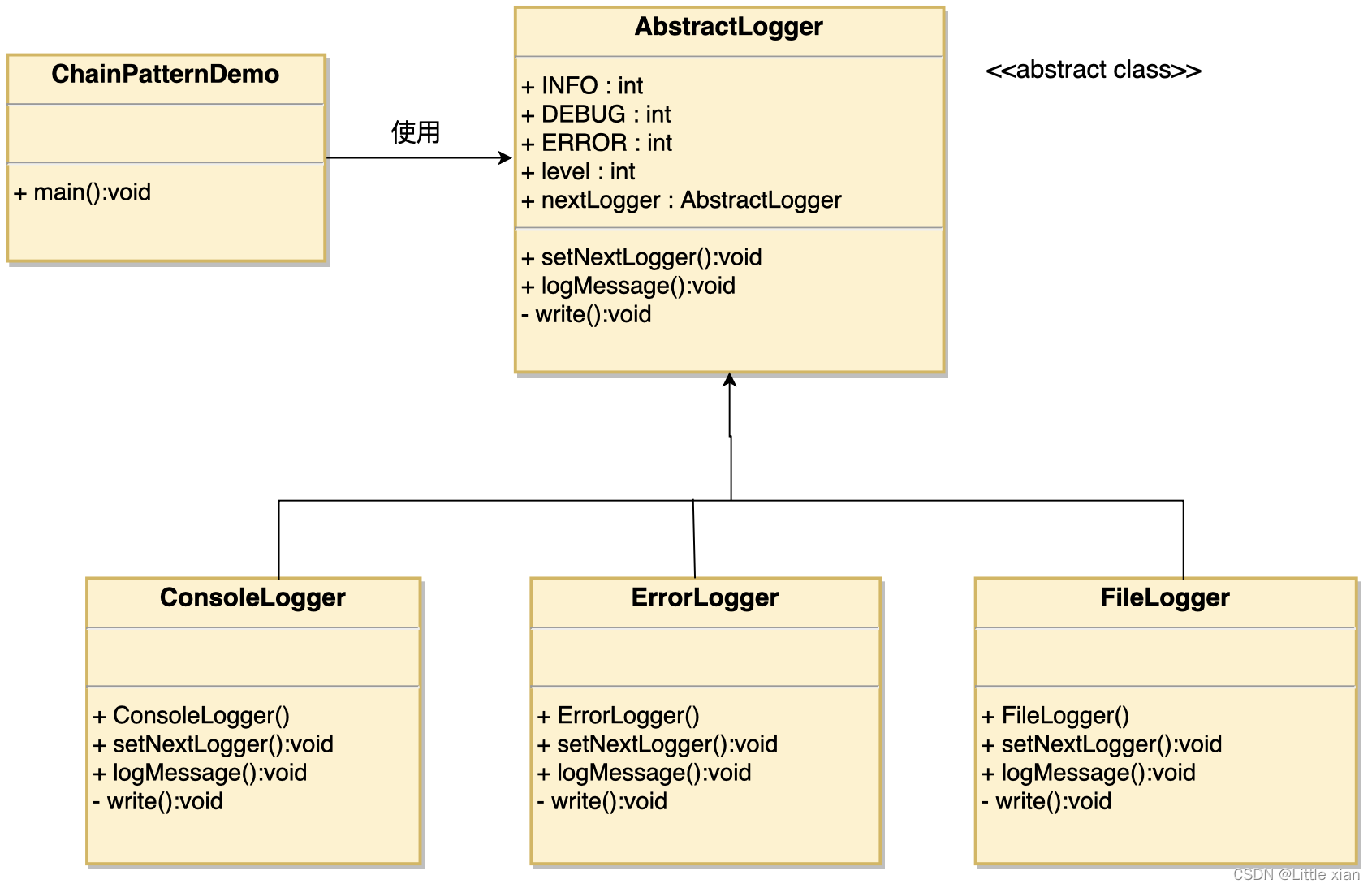 一般的责任链模式是ABCD,A保存B的引用,B保存C的,但是Okhttp是保存在list中。
参考
https://blog.csdn.net/heng615975867/article/details/105289872/ https://www.cnblogs.com/jimuzz/p/14536105.html 责任链模式
|