进程大致为一个可以独立运行的程序单位
线程的集合为进程,一般有main线程、gc线程
Thread? ? ? ? 创建线程【1】
继承Thread | 重写run()方法 | 创建一个线程对象 调用start()方法开启线程 |
package hk.Thread.deom1;
//继承Thread ,重写run方法 ,调用start开启线程;
public class TextThread extends Thread{
@Override
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println("run()——"+i);
}
}
public static void main(String[] args) {
//创建一个线程对象,调用start方法;开启线程;
TextThread textThread = new TextThread();
textThread.start();
for (int i = 0; i < 5; i++) {
System.out.println("main()——"+i);
}
}
}
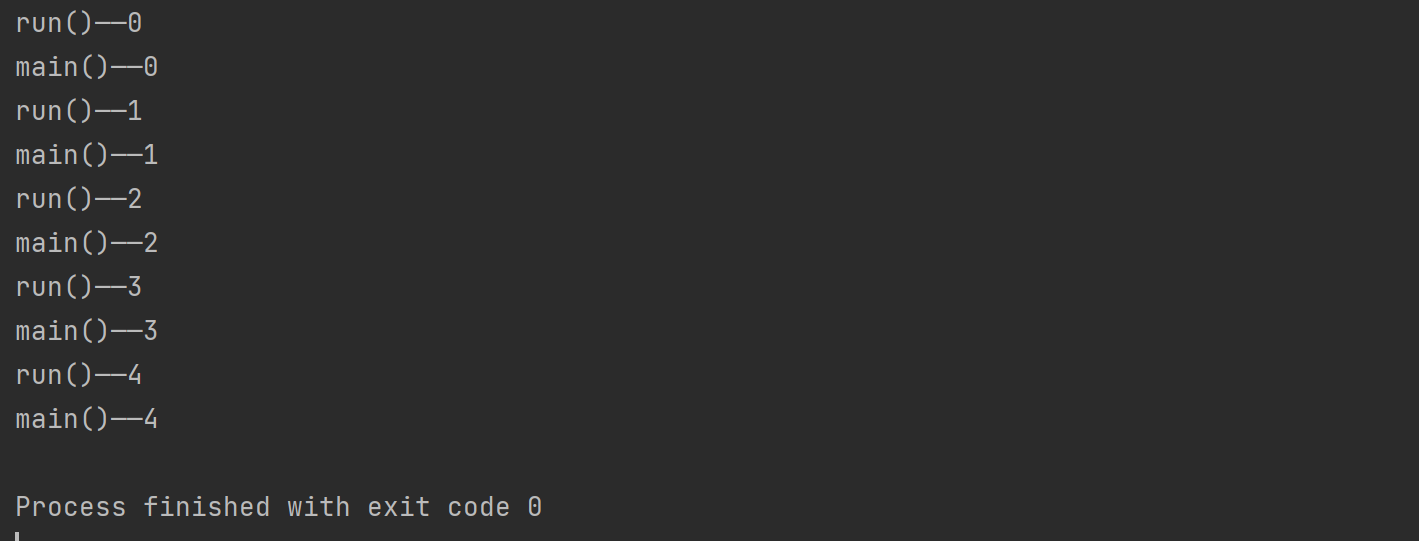
?main()for循环6次????????????????run()for循环3次
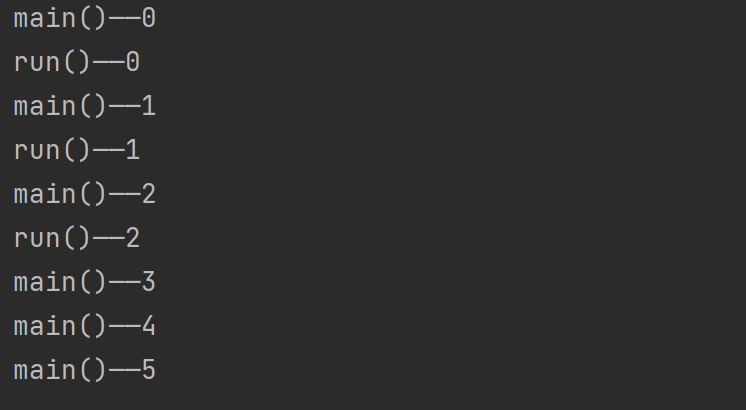
??main()for循环3次????????????????run()for循环6次
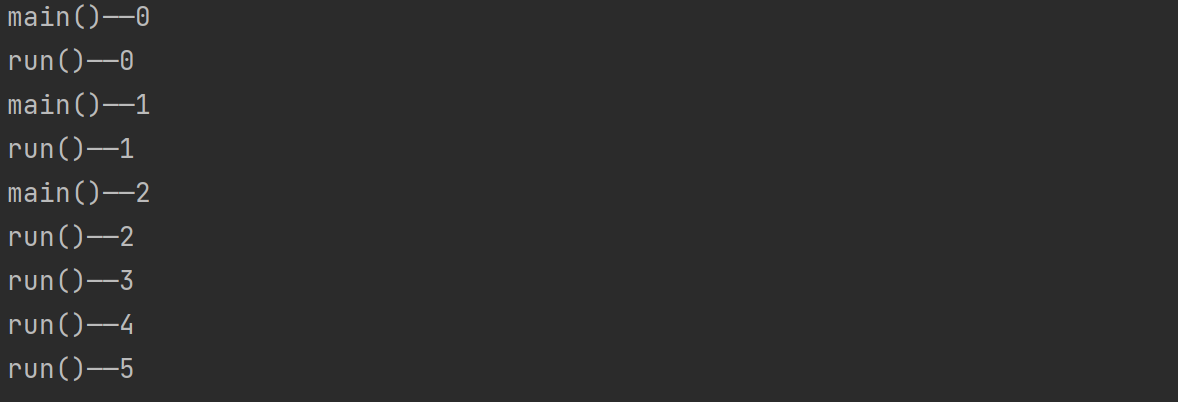
?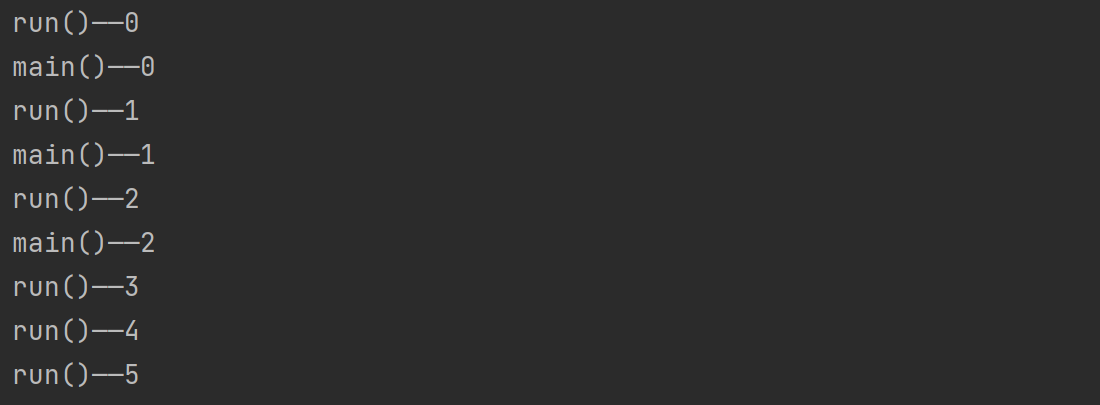
?线程开启不一定立即执行;由cpu调度执行;=》同一程序每次运行次序有可能不同
package hk.Thread.deom1;
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
import java.net.URL;
public class TextDownloader extends Thread{
private String url;
private String name;
public TextDownloader(String url ,String name){
this.name=name;
this.url=url;
}
@Override
public void run() {
Down down = new Down();
down.down(this.url,this.name);
System.out.println("下载结束 :"+name+"——:——"+url);
}
public static void main(String[] args) {
TextDownloader textDownloader = new TextDownloader("https://staticls.tianqistatic.com/static/img/yujing.png","img1.jpg");
TextDownloader textDownloader1 = new TextDownloader("https://content.pic.tianqistatic.com/contento/images/202204/05/e9aac8655667e3c4.jpg","img2.jpg");
TextDownloader textDownloader2 = new TextDownloader("https://staticls.tianqistatic.com/static/img/yujing.png","img3.jpg");
TextDownloader textDownloader0 = new TextDownloader("https://staticls.tianqistatic.com/static/img/logo_210.png","img0.jpg");
textDownloader0.start();
textDownloader.start();
textDownloader1.start();
textDownloader2.start();
}
}
class Down{
public void down(String url,String name){
try {
FileUtils.copyURLToFile(new URL(url),new File(name));
} catch (IOException e) {
e.printStackTrace();
System.out.println("Down方法异常..."+url);
}
}
}
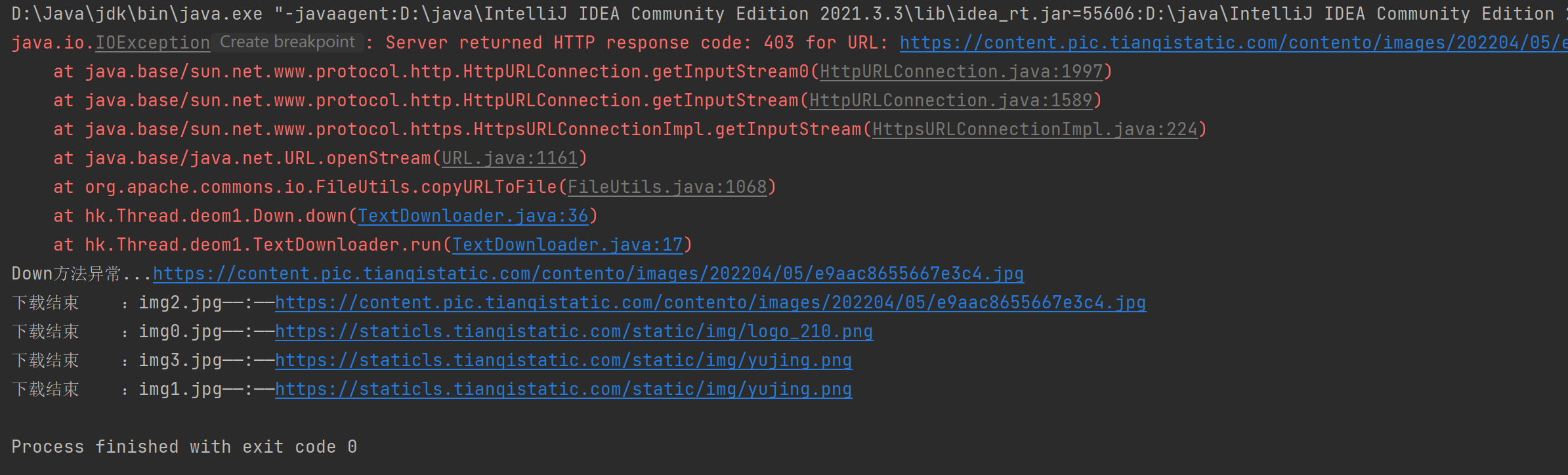
?多线程下载图片;没有ua伪装,有一个异常,其他三个成功多线程下载了
Runnable接口? ? ? ? 创建线程【2】? ? ? ? 【灵活方便? ? ? ? 推荐】
实现Runnable接口 | 重写run()方法 | 创建Runnable接口实现类对象 | 创建Thread对象,参数为runnable接口实现类对象 调用start()方法开启线程 |
package hk.Thread.deom2;
public class Text1 implements Runnable{
@Override
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println("run——"+i);
}
}
public static void main(String[] args) {
Text1 text1 = new Text1();
new Thread(text1).start();
for (int i = 0; i < 3; i++) {
System.out.println("main——"+i);
}
}
}
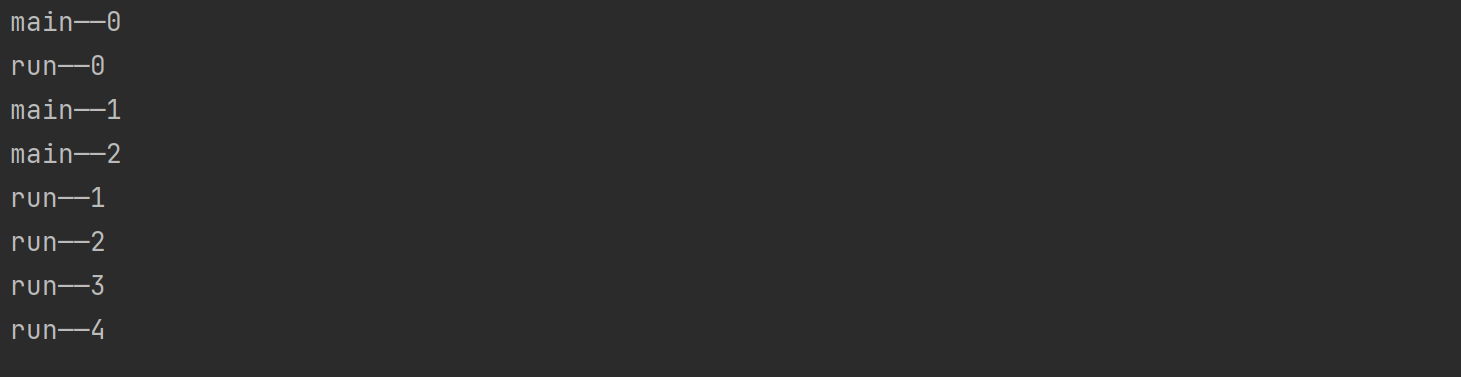
并发问题
多个线程同时操作同一资源,数据紊乱
package hk.Thread.deom3;
public class Text1 implements Runnable{
private int ticketsum=5;
@Override
public void run() {
while(true){
if (ticketsum<=0){
break;
}
try {
Thread.sleep(300);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+"ticket: "+ticketsum--);
}
}
public static void main(String[] args) {
Text1 text1 = new Text1();
new Thread(text1, "1").start();
new Thread(text1, "2").start();
new Thread(text1, "3").start();
}
}
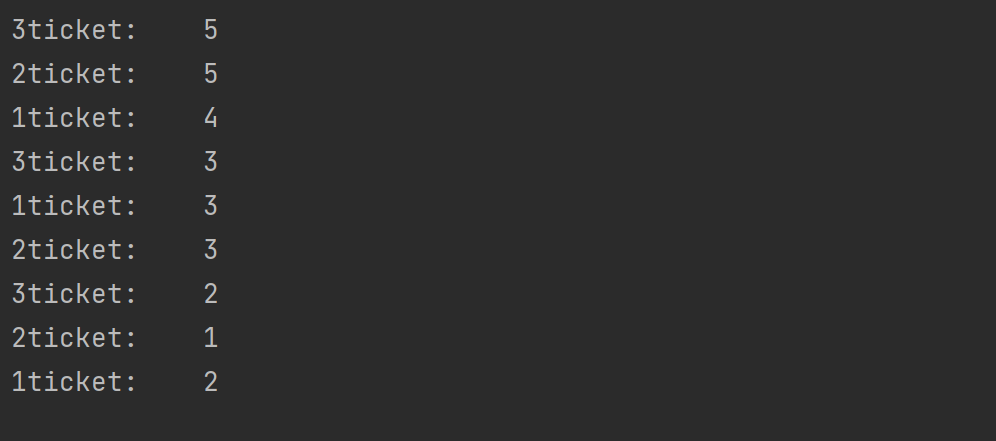
? Callable接口? ? ? ? 创建线程【3】? ? ? ? 可以定义返回值?
实现Callable接口 | 重写call()方法 | 创建Callable实现类对象 | 创建执行服务 | 提交执行 | 获取结果 | 关闭服务 |
package hk.Thread.deom3;
import java.util.concurrent.*;
public class Text0 implements Callable {
@Override
public Boolean call() {
for (int i = 0; i < 5; i++) {
System.out.println(Thread.currentThread().getName()+"call—— "+i);
}
return true;
}
public static void main(String[] args) throws ExecutionException, InterruptedException {
Text0 text00 = new Text0();
Text0 text01 = new Text0();
//创建执行服务[线程个数]
ExecutorService ser= Executors.newFixedThreadPool(2);
//提交执行
Future<Boolean> r0 =ser.submit(text00);
Future<Boolean> r1 =ser.submit(text01);
//获取结果
boolean rs0=r0.get();
boolean rs1=r1.get();
System.out.println(rs0);
System.out.println(rs1);
//关闭服务
ser.shutdown();
}
}
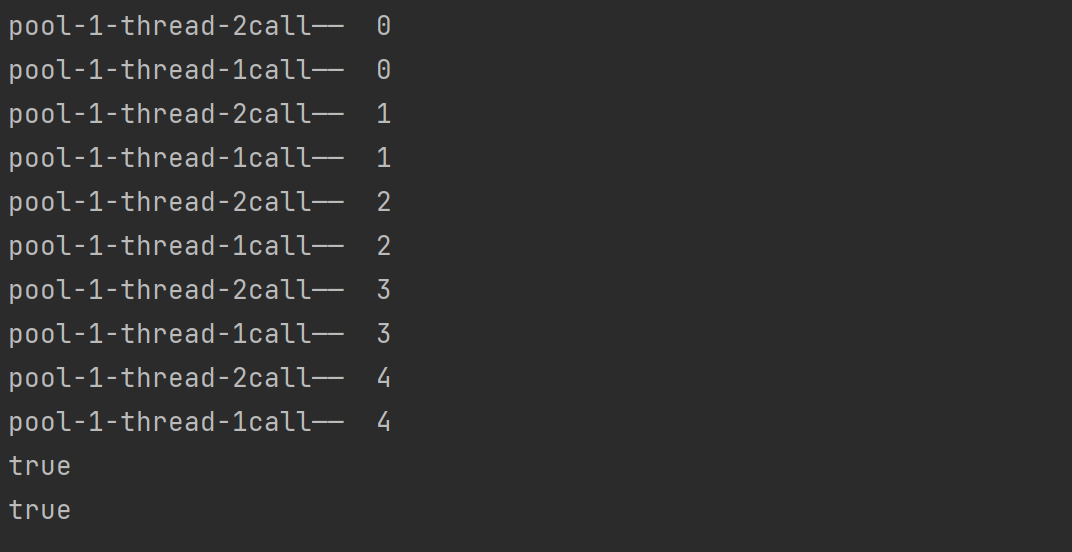
?lambda 表达式 (参数)——> ?具体函数?
package hk.Thread.deom4;
public class Lambda {
//2,静态内部类
static class A2 implements A{
@Override
public void Fun() {
System.out.println("A2——Fun()");
}
}
public static void main(String[] args) {
A a=new A1();
a.Fun();
a=new A2();
a.Fun();
//3,局部内部类
class A3 implements A{
@Override
public void Fun() {
System.out.println("A3——Fun()");
}
}
a=new A3();
a.Fun();
//4,匿名内部类,没有类的名称,必须借助接口或者父类
a=new A() {
@Override
public void Fun() {
System.out.println("A4——Fun()");
}
};
a.Fun();
//5,lambda
a=()-> {
System.out.println("A5——Fun()");
};
a.Fun();
}
}
//0.定义一个函数式接口
interface A{
void Fun();
}
//1,实现类
class A1 implements A{
@Override
public void Fun() {
System.out.println("A1——Fun()");
}
}
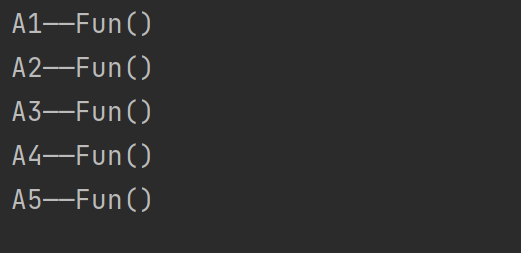
package hk.Thread.deom4;
public class Text {
public static void main(String[] args) {
B b=(int...nums)->{
int out=0;
for (int num : nums) {
out+=num;
}
System.out.println(out);
return out;
};
int out=b.fun(1,2,3,4,5,6,7,8,9);
System.out.println("out= "+out);
}
}
interface B{
int fun(int...nums);
}
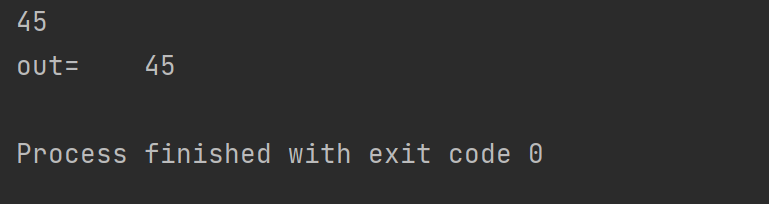
|