该篇文章是《使用poi根据模版生成word文档并转换成PDF文件》后续解决传入文件为doc文档或docx的处理方法
public void getWord(String path, Map<String, Object> params, List<String[]> tableList, String fileName,
HttpServletResponse response) throws Exception {
String modelFilePath = PathConfig.temporaryFilePath+PathConfig.imgPath;
String temporaryFilePath = PathConfig.temporaryFilePath+PathConfig.temporaryPath;
String filePath = modelFilePath+path;
File file = new File(filePath);
InputStream is = new FileInputStream(file);
try{
CustomXWPFDocument docx = new CustomXWPFDocument(is);
docx(docx,params,tableList,fileName,temporaryFilePath,response);
}catch (Exception e){
is = new FileInputStream(file);
HWPFDocument doc = new HWPFDocument(is);
doc(doc,params,tableList,fileName,temporaryFilePath,response);
}
this.close(is);
}
public void doc(HWPFDocument doc, Map<String, Object> params, List<String[]> tableList, String fileName, String temporaryFilePath,
HttpServletResponse response) throws Exception{
Range range = doc.getRange();
for (Map.Entry<String, Object> entry : params.entrySet()) {
range.replaceText(entry.getKey(), entry.getValue()+"");
}
OutputStream os = null;
fileName = java.net.URLDecoder.decode(fileName, "UTF-8");
os = new FileOutputStream(temporaryFilePath + fileName);
doc.write(os);
try {
response.setContentType("application/xls");
response.addHeader("Content-Disposition",
"attachment;filename=" + new String(fileName.getBytes("UTF-8"), "iso-8859-1"));
InputStream input = new FileInputStream(temporaryFilePath + fileName);
byte buffBytes[] = new byte[1024];
OutputStream as = response.getOutputStream();
int read = 0;
while ((read = input.read(buffBytes)) != -1) {
as.write(buffBytes, 0, read);
}
as.flush();
as.close();
input.close();
} catch (IOException ex) {
ex.printStackTrace();
}
this.close(os);
File f = new File(temporaryFilePath + fileName);
f.delete();
}
public void docx(CustomXWPFDocument docx, Map<String, Object> params, List<String[]> tableList, String fileName, String temporaryFilePath,
HttpServletResponse response) throws Exception{
this.replaceInPara(docx, params);
this.replaceInTable(docx, params, tableList);
OutputStream os = null;
fileName = java.net.URLDecoder.decode(fileName, "UTF-8");
os = new FileOutputStream(temporaryFilePath + fileName);
docx.write(os);
try {
response.setContentType("application/xls");
response.addHeader("Content-Disposition",
"attachment;filename=" + new String(fileName.getBytes("UTF-8"), "iso-8859-1"));
InputStream input = new FileInputStream(temporaryFilePath + fileName);
byte buffBytes[] = new byte[1024];
OutputStream as = response.getOutputStream();
int read = 0;
while ((read = input.read(buffBytes)) != -1) {
as.write(buffBytes, 0, read);
}
as.flush();
as.close();
input.close();
} catch (IOException ex) {
ex.printStackTrace();
}
this.close(os);
File f = new File(temporaryFilePath + fileName);
f.delete();
}
参考链接:https://www.cnblogs.com/mr-wuxiansheng/p/6131494.html
主要参考方法: 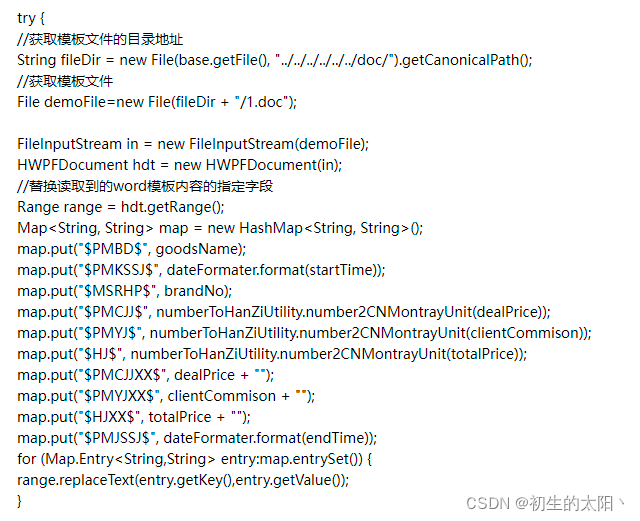
String fileDir = new File(base.getFile(), "../../../../../../doc/").getCanonicalPath();
File demoFile=new File(fileDir + "/1.doc");
FileInputStream in = new FileInputStream(demoFile);
HWPFDocument hdt = new HWPFDocument(in);
Range range = hdt.getRange();
Map<String, String> map = new HashMap<String, String>();
map.put("$PMBD$", goodsName);
map.put("$PMKSSJ$", dateFormater.format(startTime));
map.put("$MSRHP$", brandNo);
map.put("$PMCJJ$", numberToHanZiUtility.number2CNMontrayUnit(dealPrice));
map.put("$PMYJ$", numberToHanZiUtility.number2CNMontrayUnit(clientCommison));
map.put("$HJ$", numberToHanZiUtility.number2CNMontrayUnit(totalPrice));
map.put("$PMCJJXX$", dealPrice + "");
map.put("$PMYJXX$", clientCommison + "");
map.put("$HJXX$", totalPrice + "");
map.put("$PMJSSJ$", dateFormater.format(endTime));
for (Map.Entry<String,String> entry:map.entrySet()) {
range.replaceText(entry.getKey(),entry.getValue());
}
|