一、添加动态库
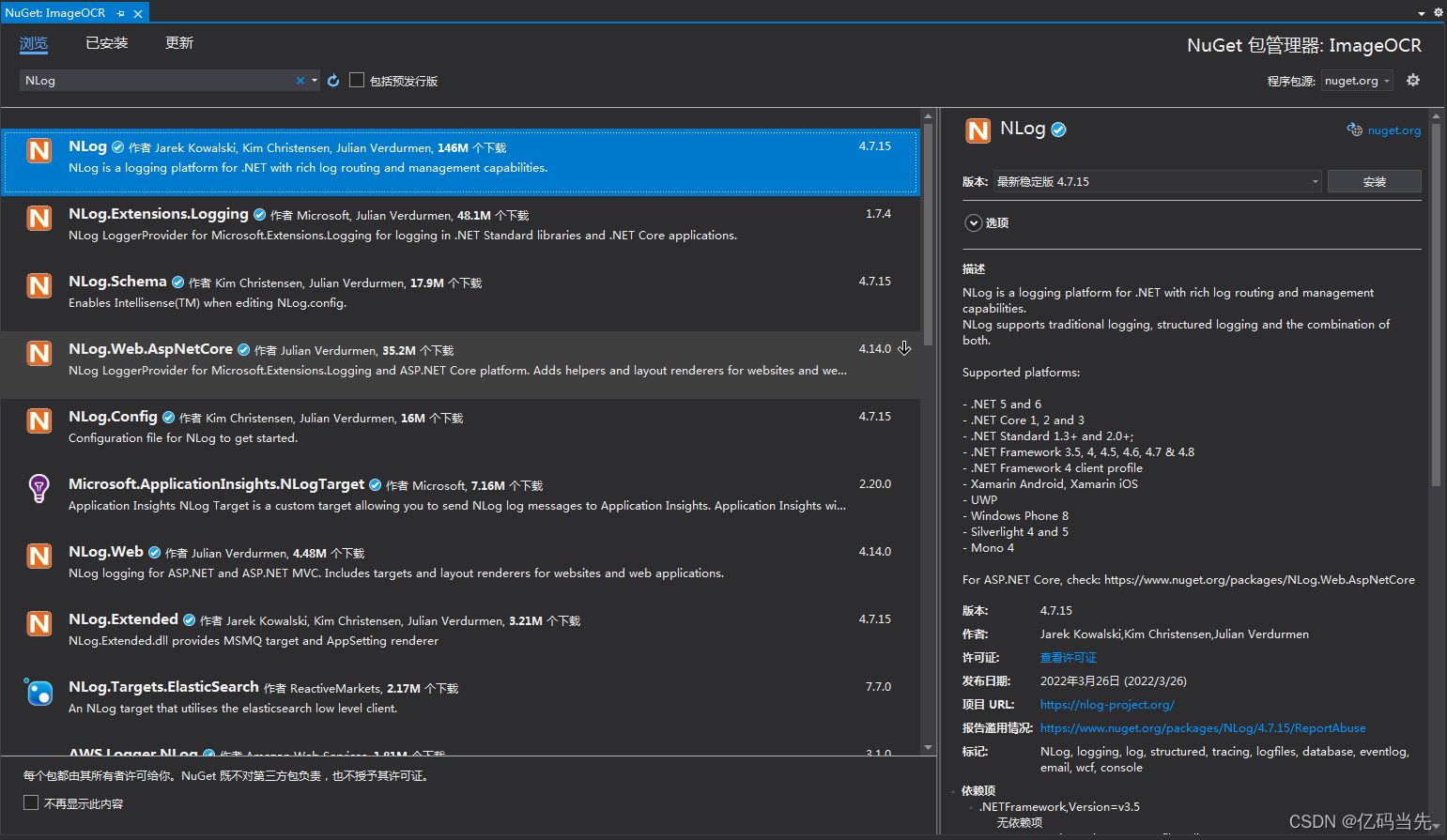
?PS:动态库版本>2.0.0,否则异常会无法输出
二、帮助类
public class Log
{
private static NLog.Logger logger = NLog.LogManager.GetCurrentClassLogger();
public static void Debug(string msg, params object[] args)
{
logger.Debug(msg, args);
}
public static void Debug(string msg, Exception err)
{
logger.Debug(msg, err);
}
public static void Info(string msg, params object[] args)
{
logger.Info(msg, args);
}
public static void Info(string msg, Exception err)
{
logger.Info(msg, err);
}
public static void Trace(string msg, params object[] args)
{
logger.Trace(msg, args);
}
public static void Trace(string msg, Exception err)
{
logger.Trace(msg, err);
}
public static void Error(string msg, params object[] args)
{
logger.Error(msg, args);
}
public static void Error(string msg, Exception err)
{
logger.Error(msg, err);
}
public static void Fatal(string msg, params object[] args)
{
logger.Fatal(msg, args);
}
public static void Fatal(string msg, Exception err)
{
logger.Fatal(msg, err);
}
/// <summary>
/// 删除指定日期前的日志
/// </summary>
/// <param name="time"></param>
public static void DelLogFile(DateTime time)
{
try
{
string directorypath = AppDomain.CurrentDomain.BaseDirectory + "Logs";
if (System.IO.Directory.Exists(directorypath))
{
DirectoryInfo thisOne = new DirectoryInfo(directorypath);
DirectoryInfo[] subDirectories = thisOne.GetDirectories(); //获得目录
//删除错误日志
if (subDirectories != null)
{
foreach (DirectoryInfo item in subDirectories)
{
DateTime filedate = DateTime.Now;
DateTime.TryParse(item.Name, out filedate);
if (time > filedate)
{
System.IO.Directory.Delete(item.FullName, true);
}
}
}
}
}
catch (Exception)
{
}
}
}
三、配置文件
<?xml version="1.0" encoding="utf-8" ?>
<nlog xmlns="http://www.nlog-project.org/schemas/NLog.xsd" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<targets>
<!--屏幕打印消息-->
<target name="console" xsi:type="ColoredConsole"
layout="${date:format=HH\:mm\:ss}> ${message}"/>
<!--vs输出窗口-->
<target name="vsdebugger" xsi:type="Debugger"
layout="[${date:format=HH\:mm\:ss}][${level:padding=5:uppercase=true}] ${message}"/>
<!--保存debug至文件-->
<target name="debugger" xsi:type="File" maxArchiveFiles="30"
fileName="${basedir}/Logs/${shortdate}/debug.txt"
layout="[${date:format=HH\:mm\:ss}][${level:padding=5:uppercase=true}] ${message}"/>
<!--保存erorr至文件-->
<target name="error_file" xsi:type="File" maxArchiveFiles="30"
fileName="${basedir}/Logs/${shortdate}/error.txt"
layout="[${longdate}][${level:uppercase=true:padding=5}] ${message} | ${onexception:${exception:format=message} ${newline} ${stacktrace}" />
<!--保存fatal至文件-->
<target name="fatal_file" xsi:type="File" maxArchiveFiles="30"
fileName="${basedir}/Logs/${shortdate}/fatal.txt"
layout="[${longdate}][${level:uppercase=true:padding=5}] ${message} | ${onexception:${exception:format=message} ${newline} ${stacktrace}" />
<!--保存info至文件-->
<target name="info_file" xsi:type="File" maxArchiveFiles="30"
fileName="${basedir}/Logs/${shortdate}/info.txt"
layout="[${longdate}][${level:uppercase=true:padding=5}] ${message} | ${exception}" />
</targets>
<rules>
<logger name="*" writeTo="console" />
<logger name="*" minlevel="Trace" writeTo="vsdebugger"/>
<logger name="*" levels="Debug" writeTo="debugger" />
<logger name="*" levels="Error" writeTo="error_file" />
<logger name="*" levels="Fatal" writeTo="fatal_file" />
<logger name="*" levels="Info" writeTo="info_file" />
</rules>
</nlog>
四、实例
public Main()
{
Log.Trace("Trace");
Log.Debug("Debug");
Log.Info("Info");
Log.Error("Error");
Log.Fatal("Info");
}
五、日志等级
Trace - 最常见的记录信息,一般用于普通输出 Debug - 调试信息 Info - 信息类型的消息 Warn - 警告信息,一般用于比较重要的场合 Error - 异常信息,捕捉的异常信息 Fatal - 致命信息。一般来讲,发生致命异常之后程序将无法继续执行。
|