实现图片浏览器应用
效果图:
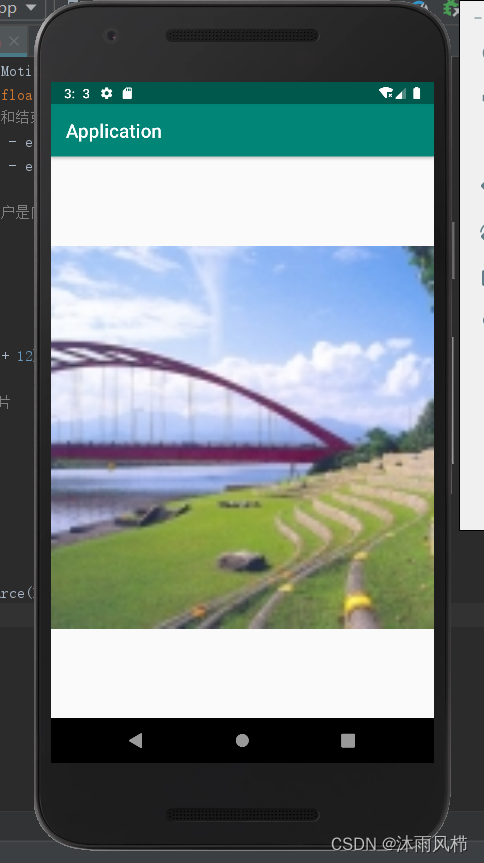 代码:
Activity
package com.example.application;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.GestureDetector;
import android.view.MotionEvent;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private GestureDetector gestureDetector;
private int[] ResId = new int[]{
R.drawable.picture0, R.drawable.picture1, R.drawable.picture2,
R.drawable.picture3, R.drawable.picture4, R.drawable.picture5,
R.drawable.picture6, R.drawable.picture7, R.drawable.picture8,
R.drawable.picture9, R.drawable.picture10, R.drawable.picture11,
R.drawable.picture12
};
private int count = 0;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
findView();
setListener();
}
private void setListener() {
gestureDetector = new GestureDetector(MainActivity.this,
onGestureListener);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
return gestureDetector.onTouchEvent(event);
}
private void findView() {
imageView = (ImageView) findViewById(R.id.imageView);
}
private GestureDetector.OnGestureListener onGestureListener
= new GestureDetector.SimpleOnGestureListener() {
@Override
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
float velocityY) {
float x = e2.getX() - e1.getX();
float y = e2.getY() - e1.getY();
if (x > 0) {
count++;
count %= 13;
} else if (x < 0) {
count--;
count = (count + 13) % 13;
}
changeImg();
return true;
}
};
public void changeImg() {
imageView.setImageResource(ResId[count]);
}
}
Layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:srcCompat="@drawable/picture0" />
</RelativeLayout>
|