1、使用原生的方案,通过Bundle进行通信
private Button mReChange;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fragment);
mReChange = findViewById(R.id.fragment_rechange);
mReChange.setOnClickListener(view -> {
Bundle bundle = new Bundle();
bundle.putString("message","需要磨练,学习编码");
BlankFragment1 blankFragment1 = new BlankFragment1();
blankFragment1.setArguments(bundle);
replaceFragment(blankFragment1);
});
}
private void replaceFragment(Fragment fragment) {
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
transaction.replace(R.id.fragment_dynamic,fragment);
transaction.addToBackStack(null);
transaction.commit();
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
if (mInflate == null){
mInflate = inflater.inflate(R.layout.fragment_blank1, container, false);
}
Bundle arguments = getArguments();
mTextView = mInflate.findViewById(R.id.fragment_text);
mButton = mInflate.findViewById(R.id.fragment_btn);
mButton.setOnClickListener(view ->{
if (arguments == null){
mTextView.setText("好好学习");
}else {
mTextView.setText(arguments.getString("message"));
}
});
return mInflate;
}
效果如下: 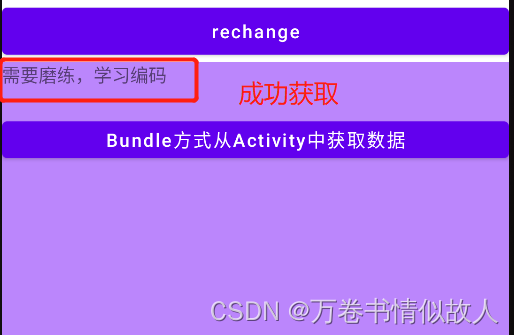
2、使用java语言中类与类通信常用方案:接口
package com.example.viewexample.fragment;
public interface IFragmentCallback {
void sendMsgToActivity(String msg);
String getMsgFromActivity();
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".fragment.CommunicationFragment">
<TextView
android:layout_width="match_parent"
android:layout_height="30dp"
android:id="@+id/communication_message"
android:textSize="20dp"
android:text="@string/hello_blank_fragment" />
<Button
android:id="@+id/getMsgFromActivity"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="从Activity获取信息" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/sendMsgToActivity"
android:text="给Activity发送信息"/>
</LinearLayout>
package com.example.viewexample.fragment;
import android.os.Bundle;
import androidx.fragment.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
import com.example.viewexample.R;
public class CommunicationFragment extends Fragment {
private View mInflate;
private Button mSendMsgToActivity;
private Button mGetMsgFromActivity;
private IFragmentCallback mIFragmentCallback;
private TextView mMessage;
private String mMsgFromActivity;
public void setIFragmentCallback(IFragmentCallback callback){
mIFragmentCallback = callback;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
if (inflater != null) {
mInflate = inflater.inflate(R.layout.fragment_communication, container, false);
}
mMessage = mInflate.findViewById(R.id.communication_message);
mSendMsgToActivity = mInflate.findViewById(R.id.sendMsgToActivity);
mSendMsgToActivity.setOnClickListener(View ->{
mIFragmentCallback.sendMsgToActivity("Fragment从这里给Activity发送了消息");
});
mGetMsgFromActivity = mInflate.findViewById(R.id.getMsgFromActivity);
mGetMsgFromActivity.setOnClickListener(View ->{
mMsgFromActivity = mIFragmentCallback.getMsgFromActivity();
mMessage.setText(mMsgFromActivity);
});
return mInflate;
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fragment);
mChange = findViewById(R.id.fragment_change);
mChange.setOnClickListener(view -> {
CommunicationFragment communicationFragment = new CommunicationFragment();
communicationFragment.setIFragmentCallback(new IFragmentCallback() {
@Override
public void sendMsgToActivity(String msg) {
Toast.makeText(FragmentActivity.this,msg,Toast.LENGTH_LONG).show();
}
@Override
public String getMsgFromActivity() {
String msg = "成功从Activity中传递了信息给Fragment";
return msg;
}
});
replaceFragment(communicationFragment);
});
}
private void replaceFragment(Fragment fragment) {
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
transaction.replace(R.id.fragment_dynamic,fragment);
transaction.addToBackStack(null);
transaction.commit();
}
效果如下: 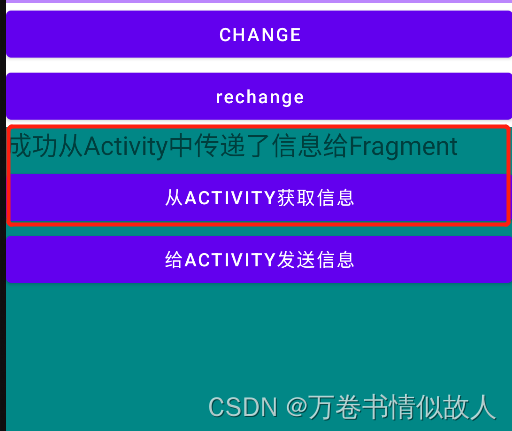 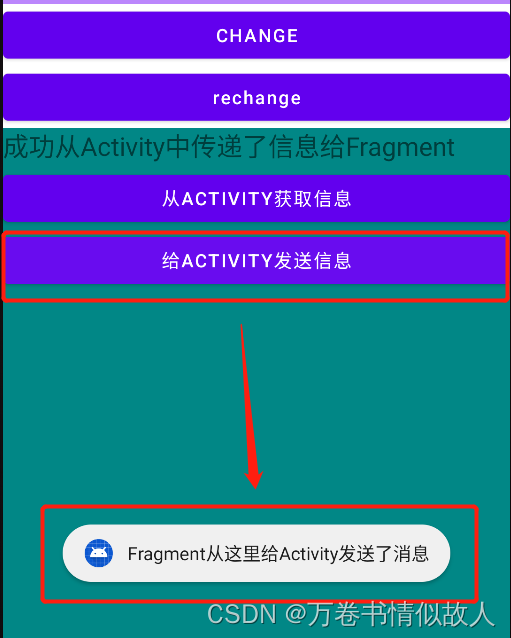
3、学习中的笔记(提醒自己需要查阅已经封装好的架构方案)
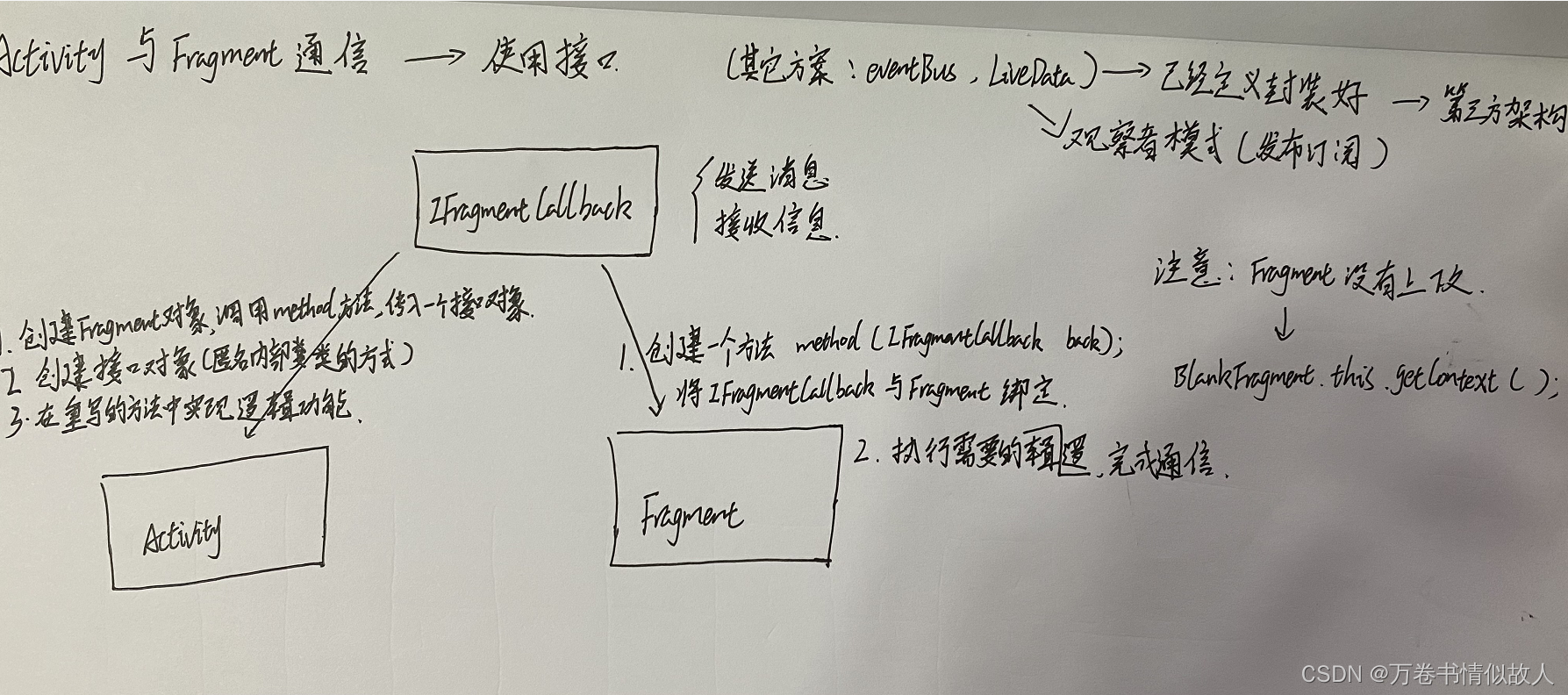
|