Java常用线程类和接口
名称 | 类型 | 特点 |
---|
Thread | class | 单继承 ,无返回值 | Runnalble | interface | 多实现,无返回值 | Callable | interface | 多实现,有返回值,带泛型,可抛出异常,Callable?般是配合线程池?具Executors来使?的 | Future | interface | 多实现,有返回值,带泛型,可取消(cancel方法) | FuturTask | class | 多实现,有返回值,实现的RunnableFuture接?,?RunnableFuture接?同时继承了Runnable 接?和Future接? |
Thread和Runnalble
JDK提供了 Thread 类和 Runnalble 接?来让我们 实现??的线程类。
- 继承 Thread 类,并重写 run ?法
- 实现 Runnable 接?的 run ?法
Thread类继承关系图: 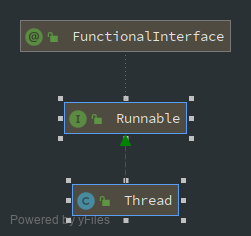 其实Thread也是实现了Runable接口。继承Thread类,Java代码实现:
public class Demo {
public static class MyThread extends Thread {
@Override
public void run() {
System.out.println("MyThread");
}
}
public static void main(String[] args) {
Thread myThread = new MyThread();
myThread.start();
}
}
Runable继承关系图: 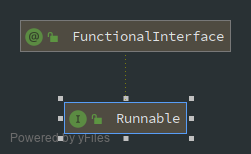
实现Runnable接?,Java代码实现:
public class Demo {
public static class MyThread implements Runnable {
@Override
public void run() {
System.out.println("MyThread");
}
}
public static void main(String[] args) {
new MyThread().start();
new Thread(() -> {
System.out.println("Java 8 匿名内部类");
}).start();
}
}
Thread类与Runnable接?的?较:
- 由于Java单继承,多实现的特性,Runnable接?使?起来?Thread更灵活
- Runnable接?出现更符合?向对象,将线程单独进?对象的封装
- Runnable接?出现降低了线程对象和线程任务的耦合性。 如果使?线程时不需要使?Thread类的诸多?法,显然使?Runnable接?更为轻量
Callable
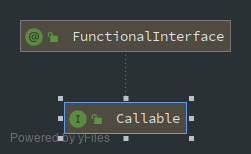
Callable接?
class Task implements Callable<Integer>{
@Override
public Integer call() throws Exception {
Thread.sleep(1000);
return 2;
}
public static void main(String args[]){
ExecutorService executor = Executors.newCachedThreadPool();
Task task = new Task();
Future<Integer> result = executor.submit(task);
System.out.println(result.get());
}
}
Future与FutureTask
Future接?部分方法:
public abstract interface Future<V> {
public abstract boolean cancel(boolean paramBoolean);
public abstract boolean isCancelled();
public abstract boolean isDone();
public abstract V get() throws InterruptedException, ExecutionException;
public abstract V get(long paramLong, TimeUnit paramTimeUnit)
throws InterruptedException, ExecutionException, TimeoutException; }
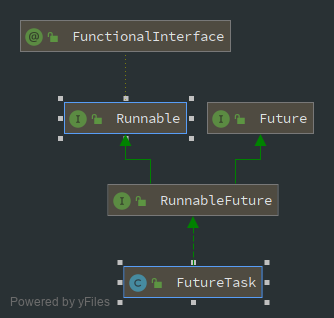
FuturTask类实现的RunnableFuture接?,?RunnableFuture接?同时继承了Runnable接?和Future接?
|