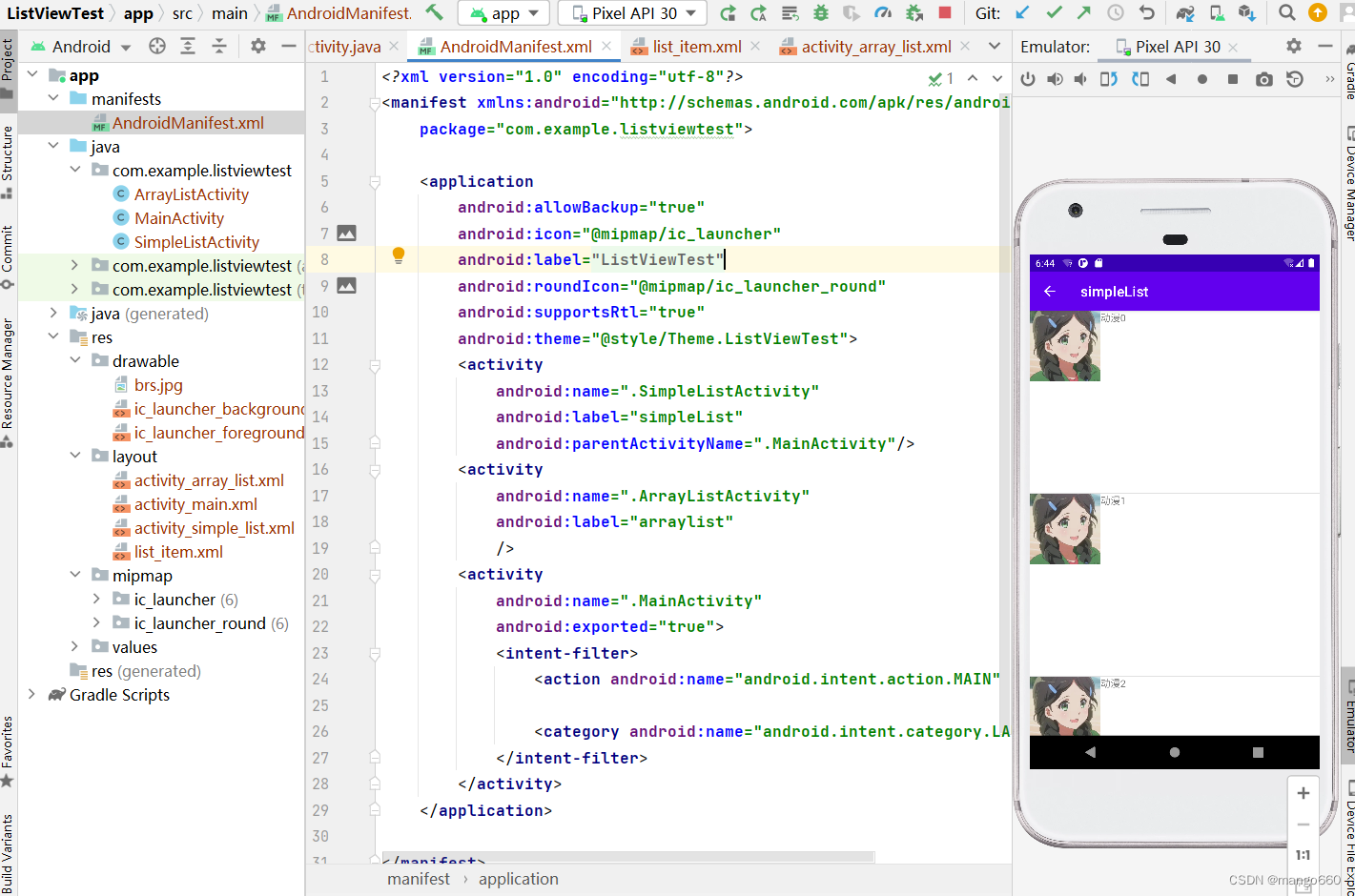
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="match_parent">
<ImageView
android:layout_width="100dp"
android:layout_height="100dp"
android:scaleType="centerCrop"
android:id="@+id/img"
/>
<TextView
android:id="@+id/title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/content"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".SimpleListActivity">
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/listview2" />
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="文本列表:ArrayAdapter"
android:textSize="40sp"
android:onClick="toArrayList"
android:layout_margin="20dp"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="图文列表"
android:textSize="40sp"
android:onClick="toSimpleList"
android:layout_margin="20dp"/>
</LinearLayout>
package com.example.listviewtest;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SimpleListActivity extends AppCompatActivity {
private ListView listView;
private SimpleAdapter simpleAdapter;
private List<Map<String, Object>> mapList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_simple_list);
listView = findViewById(R.id.listview2);
mapList = new ArrayList<>();
for (int i = 0; i < 30; i++) {
Map<String, Object> map = new HashMap<>();
map.put("img", R.drawable.brs);
map.put("keyword", "动漫" + i);
map.put("content", "离离原上草, 一岁一枯荣" + i);
mapList.add(map);
}
simpleAdapter = new SimpleAdapter(this, mapList,
R.layout.list_item,
new String[]{"img", "keyword", "content"},
new int[]{
R.id.img,
R.id.title,
R.id.content
});
listView.setAdapter(simpleAdapter);
}
}
|