最近公司需要开发一款蓝牙app,从来没搞过蓝牙所以想写个demo试试手,于是按照文档就开始了,一口气把蓝牙的状态、扫描、扫描完成等广播一口气全都注册了
//蓝牙状态监听
IntentFilter filter = new IntentFilter(BluetoothAdapter.ACTION_STATE_CHANGED);
//扫描蓝牙设备
filter.addAction(BluetoothDevice.ACTION_FOUND);
//搜索完成
filter.addAction(BluetoothAdapter.ACTION_DISCOVERY_FINISHED);
BluetoothReceiver bluetoothReceiver = new BluetoothReceiver() ;
registerReceiver(bluetoothReceiver,filter);
//蓝牙适配器
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
showToast("设备不支持蓝牙");
}
if (!bluetoothAdapter.isEnabled()) {
//未打开蓝牙
Intent intent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(intent, REQUEST_ENBLE_BT);
}
//开启蓝牙扫描
bluetoothAdapter.startDiscovery();
自定义的广播接收器BluetoothReceiver如下:
package com.test.bluetooth;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import com.blankj.utilcode.util.LogUtils;
/**
* @CreateDate: 2022/5/5
* @Author:lp
* @Description:
*/
public class BluetoothReceiver extends BroadcastReceiver {
public int DEFAULT_VALUE_BLUETOOTH = 1000;
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
//蓝牙状态改变
if (BluetoothAdapter.ACTION_STATE_CHANGED.equals(action)) {
int state = intent.getIntExtra(BluetoothAdapter.EXTRA_STATE, DEFAULT_VALUE_BLUETOOTH);
switch (state) {
case BluetoothAdapter.STATE_ON:
LogUtils.i("onReceive: open");
break;
case BluetoothAdapter.STATE_OFF:
LogUtils.i("onReceive: off");
break;
case BluetoothAdapter.STATE_TURNING_ON:
LogUtils.i("onReceive: 正在打开");
break;
case BluetoothAdapter.STATE_TURNING_OFF:
LogUtils.i("onReceive: 正在关闭");
break;
}
}
//扫描其他设备
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
// 从intent对象中获取蓝牙设备的信息
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
Log.d("设备",device.getName() + "\t" + device.getAddress());
}
//蓝牙配对
if (BluetoothDevice.ACTION_BOND_STATE_CHANGED.equals(intent.getAction())) {
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
switch (device.getBondState()) {
case BluetoothDevice.BOND_BONDED:
LogUtils.i("onReceive: 配对完成");
break;
case BluetoothDevice.BOND_BONDING:
LogUtils.i("onReceive: 正在配对");
break;
case BluetoothDevice.BOND_NONE:
LogUtils.i("onReceive: 取消配对");
break;
}
}
if (BluetoothAdapter.ACTION_DISCOVERY_FINISHED.equals(intent.getAction())) {
LogUtils.i("扫描完成");
}
}
}
然后发现我注册的BluetoothDevice.ACTION_FOUND在广播接收器没有被触发,onReceive()方法没有被调用,查阅文档蓝牙6.0之后开启蓝牙搜索需要增加动态权限:android.permission.ACCESS_FINE_LOCATION
于是在AndroidManifest中添加权限
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
之前写的?bluetoothAdapter.startDiscovery();放到权限允许的方法中去做:(这里偷个懒,因为项目依赖了工具包com.blankj:utilcodex,这里就直接调用工具类的权限请求方法)
@SuppressLint("WrongConstant")
private void reqPermission(){
PermissionUtils.permissionGroup(Manifest.permission.ACCESS_FINE_LOCATION).callback(new PermissionUtils.FullCallback() {
@Override
public void onGranted(@NonNull @NotNull List<String> granted) {
//权限允许
bluetoothAdapter.startDiscovery();
}
@Override
public void onDenied(@NonNull @NotNull List<String> deniedForever, @NonNull @NotNull List<String> denied) {
//权限拒绝
showToast("权限拒绝");
}
}).request();
}
重新运行会弹出权限授权提示:
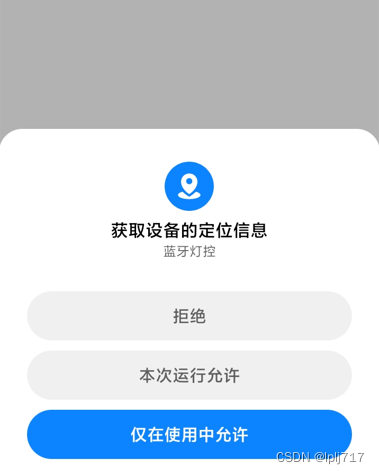
允许之后运行发现可以拿到周边蓝牙设备:
?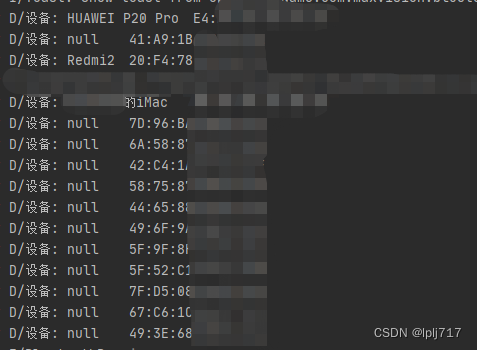
?
|