这个系列只是一个基于环信的demo。界面设计很潦草,主要是完成功能的实现。
首先结构分析:
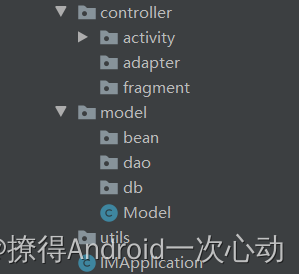
????????欢迎界面的功能是:延迟2s后跳转到指定界面(登录过就直接进入首页,没有登录就进入登录界面)
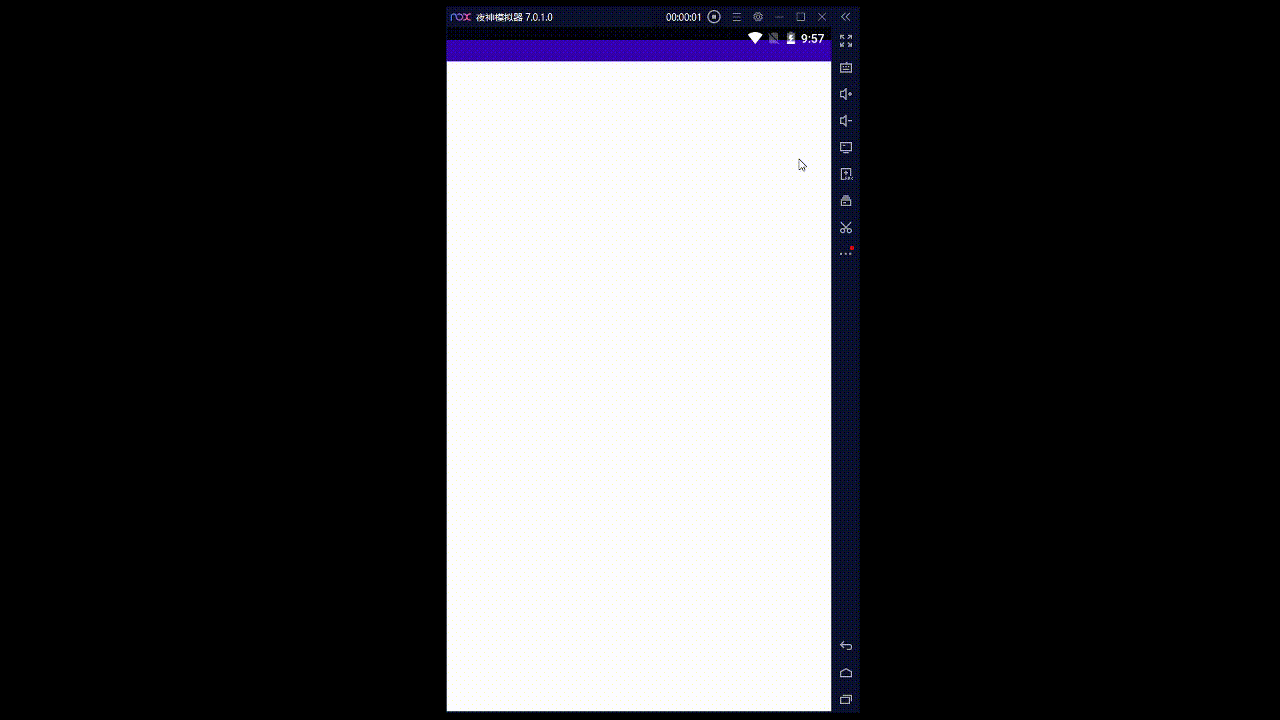
?
一、准备工作:
在集成环形的过程中需要创建一个IMApplication,用于初始化。
1.IMApplication.java
public class IMApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
EMOptions options = new EMOptions();
// 默认添加好友时,是不需要验证的,改成需要验证
options.setAcceptInvitationAlways(false);
// 是否自动将消息附件上传到环信服务器,默认为True是使用环信服务器上传下载,如果设为 false,需要开发者自己处理附件消息的上传和下载
options.setAutoTransferMessageAttachments(true);
// 是否自动下载附件类消息的缩略图等,默认为 true 这里和上边这个参数相关联
options.setAutoDownloadThumbnail(true);
//EaseIM初始化
if (EaseIM.getInstance().init(getApplicationContext(), options)) {
//在做打包混淆时,关闭debug模式,避免消耗不必要的资源
EMClient.getInstance().setDebugMode(true);
//EaseIM初始化成功之后再去调用注册消息监听的代码 ...
}
//初始化数据模型
Model.getInstance().init(this);
}
}
2.Model.java
在Model文件下创建一个Model模型(单例模式)
//创建一个数据模型层全局类
//来实现model层与controller层的交互,所有的数据都必须经过这个类才能访问到controller
public class Model {
private Context mContext;
//创建一个线程池
private ExecutorService executors = Executors.newCachedThreadPool();
//首先是一个全局单例模式
//创建私有对象
private static Model model = new Model();
//私有构造方法
private Model() {
}
//提供全局的访问方法,获取单例对象。
public static Model getInstance(){
return model;
}
//初始化方法
public void init(Context context){
mContext = context;
}
public ExecutorService getGlobalThreadPool(){
return executors;
}
}
二、欢迎界面
1.activity_welcome.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".controller.activity.WelcomeActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="欢迎进入基于环信开发的聊天demo"
android:textSize="20sp"
android:textStyle="bold"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
2.WelcomeActivity.java
public class WelcomeActivity extends Activity {
private Handler handler = new Handler(){
public void handleMessage(Message msg){
//如果当前activity已经退出,那么我就不处理handler中的消息
if(isFinishing()){
return;
}
//登录还是进入主界面
toMainOrLogin();
}
};
private void toMainOrLogin() {
//我们在这里new了一个匿名的线程,显然这种方法是不好的
//那应该怎么做呢,我们可以把线程放到线程池进行管理,随意的创建线程可能导致内存溢出OOM
// new Thread(){
// public void run(){
// //判断当前账号是否已经登录过
// if(EMClient.getInstance().isLoggedInBefore()){//登录过
//
// //获取当前用户信息
//
// //跳转到主界面
// Intent intent = new Intent(WelcomeActivity.this,MainActivity.class);
// startActivity(intent);
// }else{//没登录过
//
// //跳转到登录界面
// Intent intent = new Intent(WelcomeActivity.this,LoginActivity.class);
// startActivity(intent);
// }
// //结束当前界面
// finish();
// }
// }.start();
Model.getInstance().getGlobalThreadPool().execute(() -> {
//判断当前账号是否已经登录过
if(EMClient.getInstance().isLoggedInBefore()){//登录过
//获取当前用户信息
//跳转到主界面
Intent intent = new Intent(WelcomeActivity.this,MainActivity.class);
startActivity(intent);
}else{//没登录过
//跳转到登录界面
Intent intent = new Intent(WelcomeActivity.this,LoginActivity.class);
startActivity(intent);
}
//结束当前界面
finish();
});
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_welcome);
Toast.makeText(WelcomeActivity.this,"此为欢迎界面,等待2s后跳转",Toast.LENGTH_LONG).show();
//发送2s钟的延迟消息
handler.sendMessageDelayed(Message.obtain(),2000);
}
}
|