AppBar 组件的结构
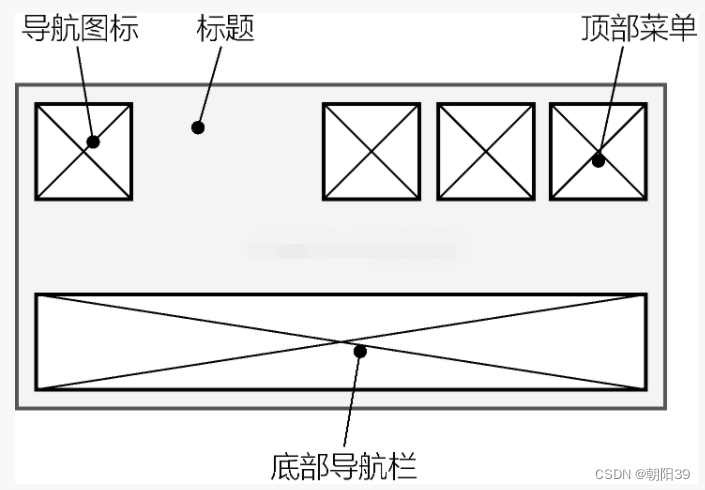
AppBar 组件的代码
AppBar(
leading: Icon(Icons.home),
title: Text('AppBar Title'),
actions: <Widget>[
IconButton(
onPressed: () {},
icon: Icon(Icons.build),
),
IconButton(
onPressed: () {},
icon: Icon(Icons.add),
)
],
)

顶部选项卡
class _AppBarSampleState extends State<AppBarSample>
with SingleTickerProviderStateMixin {
List<Item> items = const <Item>[
const Item(title: 'Item1', icon: Icons.directions_car),
const Item(title: 'Item2', icon: Icons.directions_bike),
const Item(title: 'Item3', icon: Icons.directions_boat),
const Item(title: 'Item4', icon: Icons.directions_walk),
];
TabController _tabController;
@override
void initState() {
super.initState();
_tabController = TabController(length: items.length, vsync: this);
}
@override
void dispose() {
_tabController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
bottom: TabBar(
isScrollable: true,
controller: _tabController,
tabs: items.map((Item item) {
return Tab(
text: item.title,
icon: Icon(item.icon),
);
}).toList(),
)
),
body: Center(
child: Text('body'),
),
);
}
}
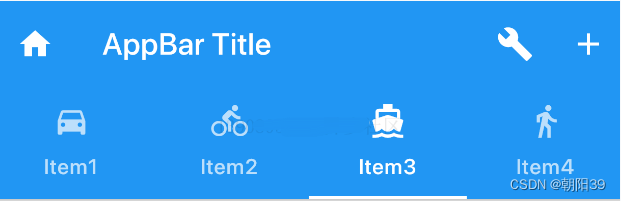 body中使用TabBarView组件对应各个选项卡所关联的主体视图,它的children属性接受一组组件来展示与每个选项卡对应的主体内容。
Scaffold(
appBar: AppBar(
bottom: TabBar(
controller: _tabController,
tabs: items.map((Item item) {
return Tab(
text: item.title,
icon: Icon(item.icon),
);
}).toList(),
)),
body: TabBarView(
controller: _tabController,
children: items.map((Item item) {
return Center(
child: Text(item.title, style: TextStyle(fontSize: 20.0),),
);
}).toList(),
),
)
- 选项卡和主体内容的控制器都是 _tabController
抽屉菜单
- 左抽屉菜单 drawer
- 右抽屉菜单 endDrawer
class _DrawerSampleState extends State<DrawerSample> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Drawer Demo'),
),
drawer: MyDrawer(),
);
}
}
class MyDrawer extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Drawer(
child: ListView(
padding: EdgeInsets.zero,
children: const <Widget>[
DrawerHeader(
decoration: BoxDecoration(
color: Colors.blue,
),
child: Text(
'菜单头部',
style: TextStyle(
color: Colors.white,
fontSize: 24,
),
),
),
ListTile(
leading: Icon(Icons.message),
title: Text('消息'),
),
ListTile(
leading: Icon(Icons.account_circle),
title: Text('我的'),
),
ListTile(
leading: Icon(Icons.settings),
title: Text('设置'),
),
],
),
);
}
}
- ListTile组件的基本结构
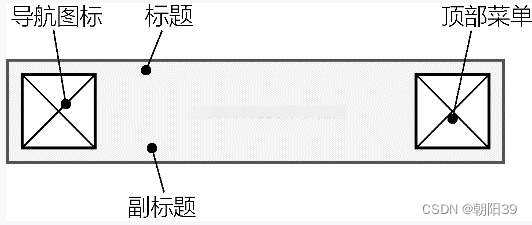 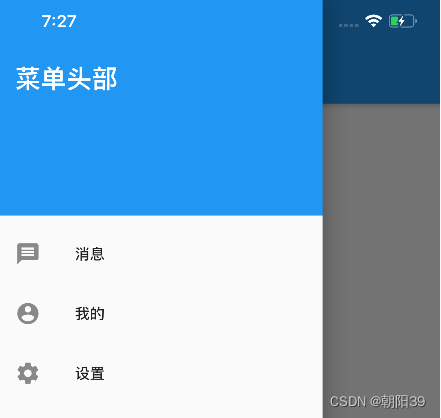 为Scaffold组件指定drawer属性后,AppBar就会自动生成一个导航图标,单击这个图标后抽屉栏就会弹出。 
自定义导航图标
- automaticallyImplyLeading 为 false 时,不显示导航图标
Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
title: const Text('Drawer Demo'),
),
drawer: MyDrawer(),
)
- AppBar里的 leading 可以自定义其他导航图标
AppBar(
leading: Icon(Icons.home),
……
)
|