效果图: 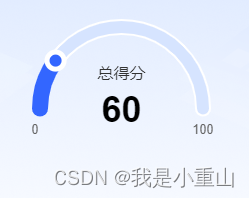
<canvas class="canvas" style="width:{{canvasWidth}}px;height:{{canvasHeight}}px;" canvas-id="circleBar"></canvas>
data: {
canvasWidth: 213, // 画布宽度
canvasHeight: 187, // 画布高度
value:60 , // 当前得分
total:100, // 总分值
},
// 初始化时调用即可
showCanvasRing() {
try {
//作画
var ctx = wx.createCanvasContext("circleBar", this); //canvas组建封装,需要后加个this
ctx.clearRect(0, 0, this.data.canvasWidth, this.data.canvasHeight); // 清除画布
var circle_r = this.data.canvasWidth / 2 - 10; //画布的一半,用来找中心点和半径
var scoreText = this.data.value; // 当前得分
var total = this.data.total;// 总分值
var score = (100 * scoreText) / total; // 满分为100时对应的值
let that = this;
//定义起始点
ctx.translate(this.data.canvasWidth / 2, this.data.canvasWidth / 2 + 5);
// 白边圆弧
ctx.beginPath();
ctx.setStrokeStyle("#FFFFFF");
ctx.setLineWidth(16);
ctx.setLineCap("round");
ctx.arc(0, 0, circle_r - 15, 1 * Math.PI, 0, false);
ctx.stroke();
ctx.closePath();
//灰色圆弧
ctx.beginPath();
ctx.setStrokeStyle("#D6E4FF");
ctx.setLineWidth(12);
ctx.setLineCap("round"); //线条结束端点样式 butt 平直 round 圆形 square 正方形
ctx.arc(0, 0, circle_r - 15, 1 * Math.PI, 0, false);
ctx.stroke();
ctx.closePath();
// 蓝色圆弧
ctx.beginPath();
ctx.setStrokeStyle("#3366FF");
ctx.setLineWidth(16);
ctx.arc(
0,
0,
circle_r - 15,
1 * Math.PI,
(score / 100 + 1) * Math.PI,
false
);
ctx.stroke();
ctx.closePath();
// 指示器-外层
let deg = 270 - score * 1.8;
const xAxis = Math.sin(((2 * Math.PI) / 360) * deg) * (circle_r - 15);
const yAxis = Math.cos(((2 * Math.PI) / 360) * deg) * (circle_r - 15);
ctx.beginPath();
ctx.arc(xAxis, yAxis, 11, 0, 2 * Math.PI);
ctx.setFillStyle("#FFFFFF");
ctx.fill();
// 指示器-内层
const xAxis2 = Math.sin(((2 * Math.PI) / 360) * deg) * (circle_r - 15);
const yAxis2 = Math.cos(((2 * Math.PI) / 360) * deg) * (circle_r - 15); // circle_r - 10
ctx.beginPath();
ctx.arc(xAxis2, yAxis2, 6, 0, 2 * Math.PI);
ctx.setFillStyle("#3366FF");
ctx.fill();
// 文字-总得分
ctx.setTextAlign("center"); // 字体位置
ctx.setFillStyle("#333333");
ctx.font = "normal normal 16px Arial,sans-serif";
ctx.fillText("总得分", 0, -30);
// 文字-具体分数
ctx.setTextAlign("center"); // 字体位置
ctx.setFillStyle("#000000");
ctx.font = "normal bold 36px Arial,sans-serif";
ctx.fillText(scoreText, 0, 13);
// 最低分
ctx.setFillStyle("#666666");
ctx.font = "normal normal 13px Arial,sans-serif";
ctx.fillText(0, -circle_r + 10, 25);
// 最高分
ctx.setFillStyle("#666666");
ctx.font = "normal normal 13px Arial,sans-serif";
ctx.fillText(total, circle_r - 15, 25);
// 绘图
ctx.draw(false, function () {
//将生成好的图片保存到本地
wx.canvasToTempFilePath(
{
canvasId: "circleBar",
success: function (res) {
var tempFilePath = res.tempFilePath;
that.setData({
loadImagePath: tempFilePath,
});
},
fail: function (res) {},
},
that
);
});
} catch (error) {
}
},
|