Android小实例-Activity之间数据交流
简介
活动(Activity)之间数据传递是相互的,介绍了上一页面将数据传递到下一个页面和上下一个页面将数据返回给上一页面。
向下传递数据
先需要两个活动(Activity)和对应的布局文件。 首先首页布局文件只需要一个按钮
第一个活动与布局
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<Button
android:id="@+id/send"
android:text="发送"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</androidx.constraintlayout.widget.ConstraintLayout>
java代码
package com.buxiaju.test3;
import android.content.Intent;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import com.buxiaju.test3.NewActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Intent intent = new Intent(this, NewActivity.class);
Bundle bundle = new Bundle();
bundle.putString("msg","今天很高兴");
intent.putExtras(bundle);
Button bt = findViewById(R.id.send); bt.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v){
startActivity(intent);
finish();
}
});
}
}
第二个活动与布局
布局文件
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
package com.buxiaju.test3;
import android.os.Bundle;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class NewActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_new);
Bundle bundle = getIntent().getExtras();
String msg = bundle.getString("msg");
TextView tv = findViewById(R.id.tv);
tv.setText(msg);
}
}
界面效果
首页 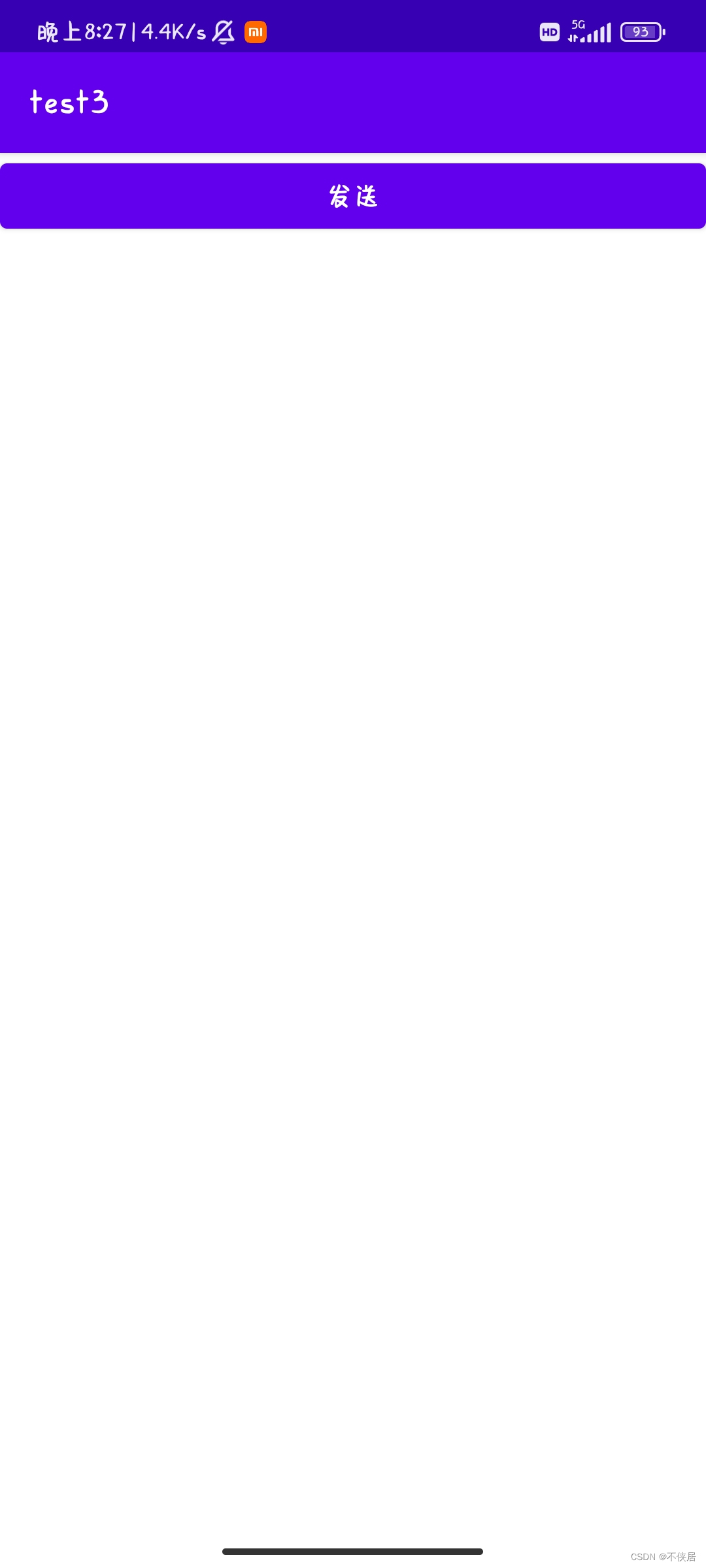
点击按钮后 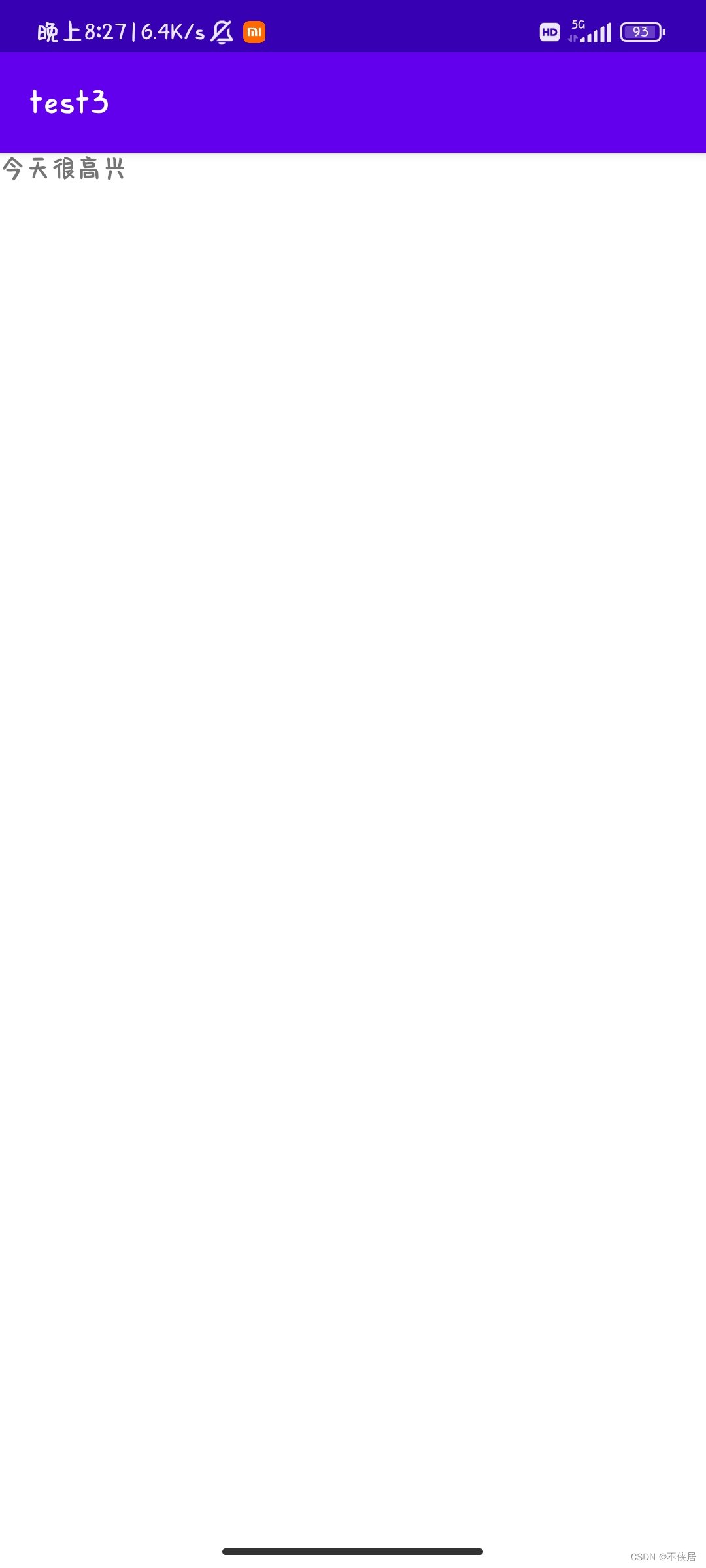
向上传递数据
也是两个活动,两个布局
第一个布局与活动
布局
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/tv1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/bt1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="跳转下一页"/></androidx.constraintlayout.widget.ConstraintLayout>
Java代码
package com.buxiaju.test4;
import android.app.Activity;import android.content.Intent;
import android.view.View;import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
private TextView tv;
private Button bt;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tv = findViewById(R.id.tv1);
bt = findViewById(R.id.bt1);
Intent intent = new Intent(this, NewActivity.class);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivityForResult(intent,0);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent intent){
super.onActivityResult(requestCode, resultCode, intent);
if (intent != null && requestCode == 0 && resultCode == Activity.RESULT_OK){
Bundle bundle = intent.getExtras();
String msg = bundle.getString("msg");
tv.setText(msg);
}
}
}
第二个活动与布局
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/bt2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="回到上一页"/>
</LinearLayout>
Java代码
package com.buxiaju.test4;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
public class NewActivity extends AppCompatActivity {
private Button bt;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_new);
bt = findViewById(R.id.bt2);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent();
Bundle bundle = new Bundle();
bundle.putString("msg", "你好陌生人");
intent.putExtras(bundle);
setResult(Activity.RESULT_OK, intent);
finish();
}
});
}
}
效果
第一个页面
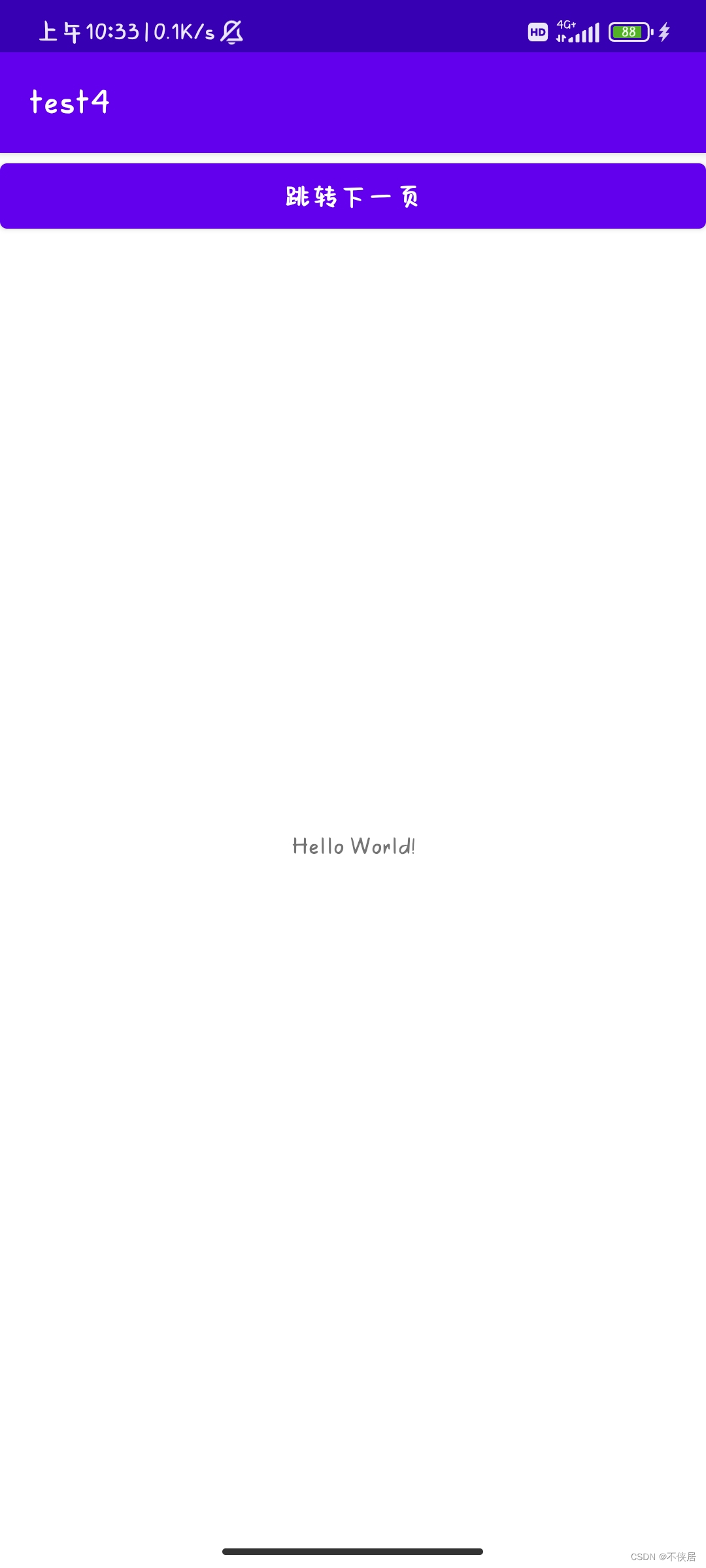 点击按钮
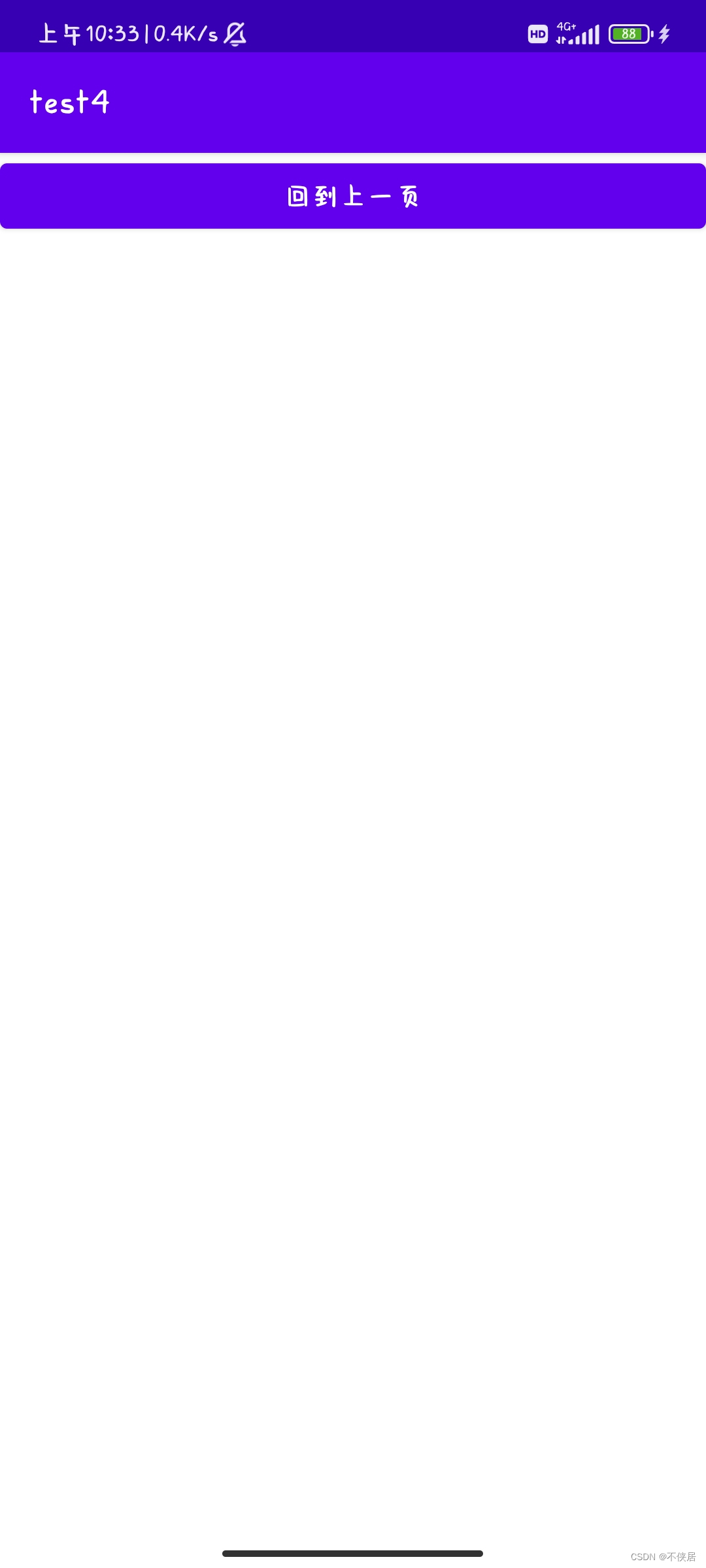
再次点击这个页面的按钮 会发现文本中的内容变了 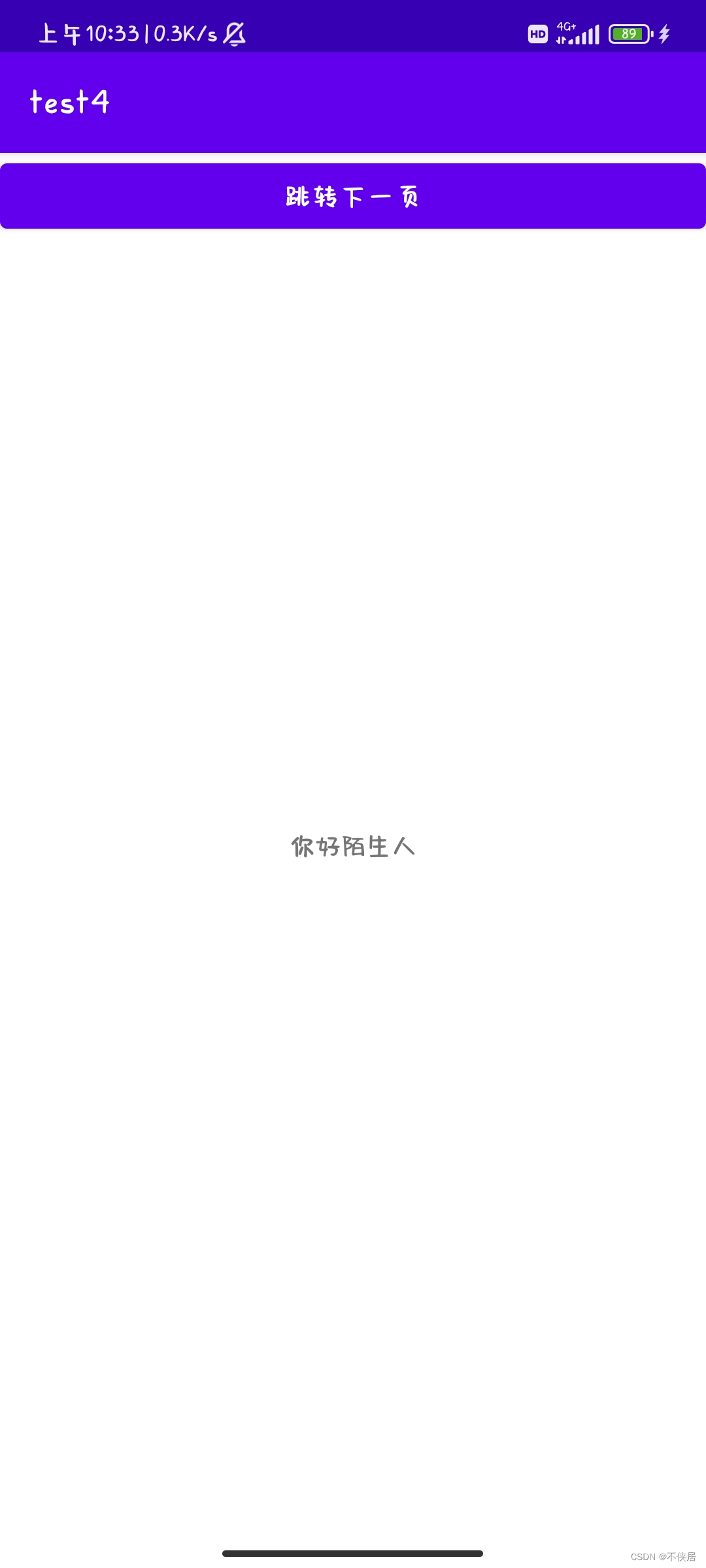
|