Flutter自定义视频播放器
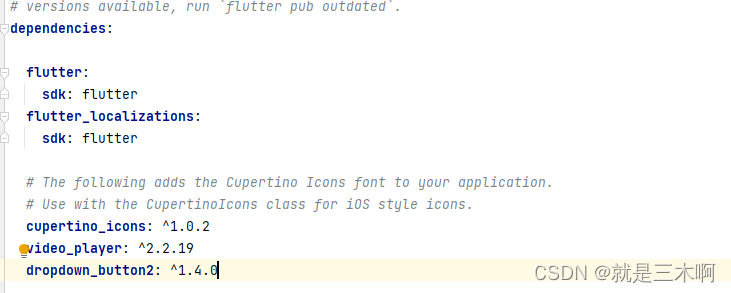
添加完成后在需要视频的dart文件引入
import 'package:video_player/video_player.dart';
import 'package:dropdown_button2/dropdown_button2.dart';
具体代码
//定义一个VideoPlayerController
var _videoPlayerController;
//监听视频是否在播放
bool _isPlaying = false;
//视频地址
String videoUrl="";
//显示隐藏功能栏
bool _isShow = true;
//显示倍速
String _selectedValue = '倍速';
List<String> items = ['1.0', '0.75', '0.5', '0.25'];
//在initstate中初始化
@override
void initState() {
_videoPlayerController =
VideoPlayerController.network(videoUrl)
..initialize().then((_) {
// 确保在初始化视频后显示第一帧,直至在按下播放按钮。
setState(() {});
});
//执行监听,只要有内容就会刷新
_videoPlayerController.addListener(() {
setState(() {
_isPlaying = _videoPlayerController.value.isPlaying;
});
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Video Demo',
home: Scaffold(
body:Container(
color: Color(0XFF000000),
child: Center(
child: GestureDetector(
child: Container(
width: double.infinity,
height: double.infinity,
color: Color(0XFF000000),
child: new Stack(
alignment: Alignment.center,
children: [
viDeoPlayer(),
_isPlaying && _isShow
? new Positioned(
bottom: 0,
left: 0,
right: 0,
child: Container(
padding: const EdgeInsets.only(
right: 10, left: 10),
color:
Color.fromRGBO(0, 0, 0, 0.5),
height: 88,
child: new Row(
mainAxisAlignment:
MainAxisAlignment
.spaceEvenly,
children: [
//暂停按钮
InkWell(
child: Icon(
Icons.pause,
size: 36,
color: Color(0xffefefef),
),
onTap: () {
_videoPlayerController
.pause();
},
),
//当前播放进度
new Text(
formatString(
_videoPlayerController
.value.position),
style: TextStyle(
fontSize: 18,
color:
Color(0xffefefef)),
),
//进度条
new Expanded(
child: Slider(
activeColor:
Color(0xffFFFFFF),
max: _videoPlayerController
.value
.duration
.inMilliseconds
.truncateToDouble(),
value:
_videoPlayerController
.value
.position
.inMilliseconds
.truncateToDouble(),
onChanged: (newRating) {
_videoPlayerController
.seekTo(Duration(
milliseconds:
newRating
.truncate()));
},
),
flex: 1,
),
//总视频进度
new Text(
formatString(
_videoPlayerController
.value.duration),
style: TextStyle(
fontSize: 18,
color:
Color(0xffefefef)),
),
//倍速下拉菜单
DropdownButtonHideUnderline(
child: DropdownButton2(
hint: Text(
_selectedValue,
style: TextStyle(
fontSize: 18,
color:
Color(0xffefefef),
),
),
items: items
.map((item) =>
DropdownMenuItem<
String>(
value: item,
child: Text(
'${item}x',
style:
const TextStyle(
fontSize:
16,
),
),
))
.toList(),
// value: _selectedValue,
icon: Visibility(
visible: false,
child: Icon(Icons
.arrow_downward)),
onChanged: (value) async {
double val =
double.parse(value
as String);
setState(() {
if (val == 1.0) {
_selectedValue =
'倍速';
} else {
_selectedValue =
'${value as String}x';
}
});
},
dropdownWidth: 100,
buttonWidth: 60,
iconSize: 36,
iconEnabledColor:Colors.white,
buttonPadding:const EdgeInsets.only(left: 10),
),
),
],
),
),
)
: new Container(),
_isPlaying
? new Container() :
InkWell(
child: Icon(
Icons.play,
size: 72,
color: Color(0xffefefef),
),
onTap: () {
_videoPlayerController
.play();
},
),
],
),
),
onTap: () {
setState(() {
//点击显示隐藏功能栏
_isShow = !_isShow;
});
},
),
),
),
),
);
}
//******************建议视频播放控件封装成类或函数************************
Widget viDeoPlayer() {
return Container(
width: double.infinity,
height: double.infinity,
color: Color(0xff000000),
child: Center(
// _controller.value.initialized表示视频是否已加载并准备好播放,
// 如果是true则返回AspectRatio(固定宽高比的组件,宽高比为视频本身的宽高比)
// VideoPlayer为视频插放器,对像就是前面定义的_controller
child: _videoPlayerController.value.isInitialized
? AspectRatio(
aspectRatio: _videoPlayerController.value.aspectRatio,
child: VideoPlayer(_videoPlayerController),
)
//如果视频没有加载好或者因网络原因加载不出来则返回Container 组件
//一般用于放置过渡画面
: Container(
child: Text("没有要播放的视频"),
),
),
);
}
//销毁videoPlayerController
@override
void dispose() {
// TODO: implement dispose
_videoPlayerController.dispose();
super.dispose();
}
|