一、创建组件 1、在根目录下自定义一个components文件夹,用来存放自定义的组件。 2、再针对每一个组件创建一个文件夹,用来存放这个组件相关的文件。 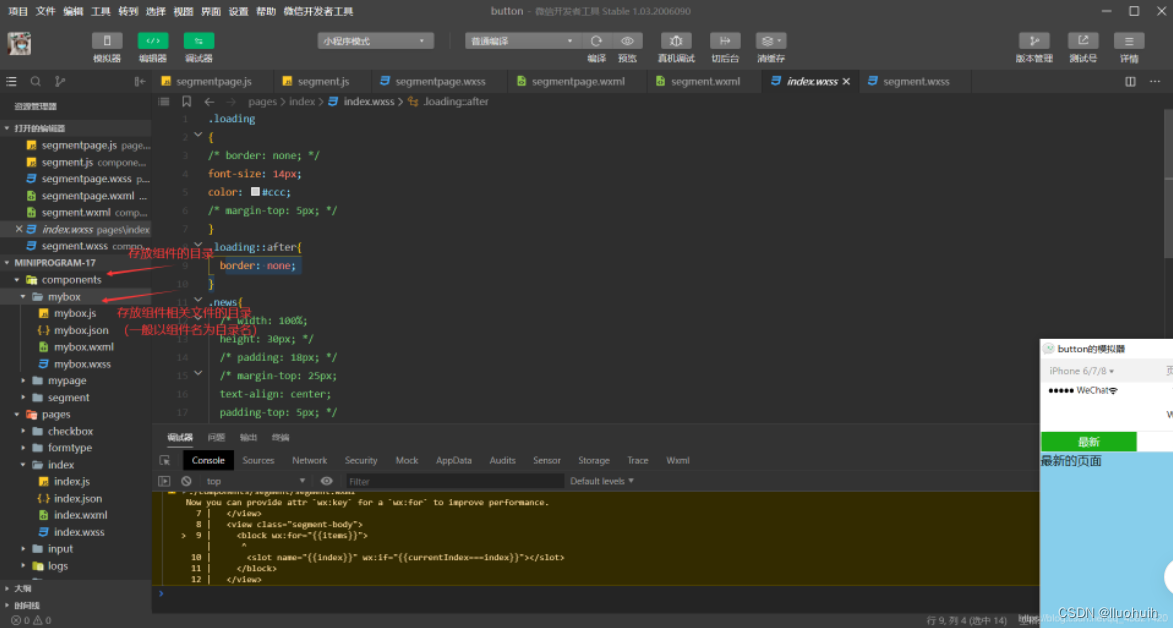 3、在指定组件的文件夹中右键->新建Component创建组件。这样创建的目的是在json文件中添加"component": true,将其声明为一个组件。 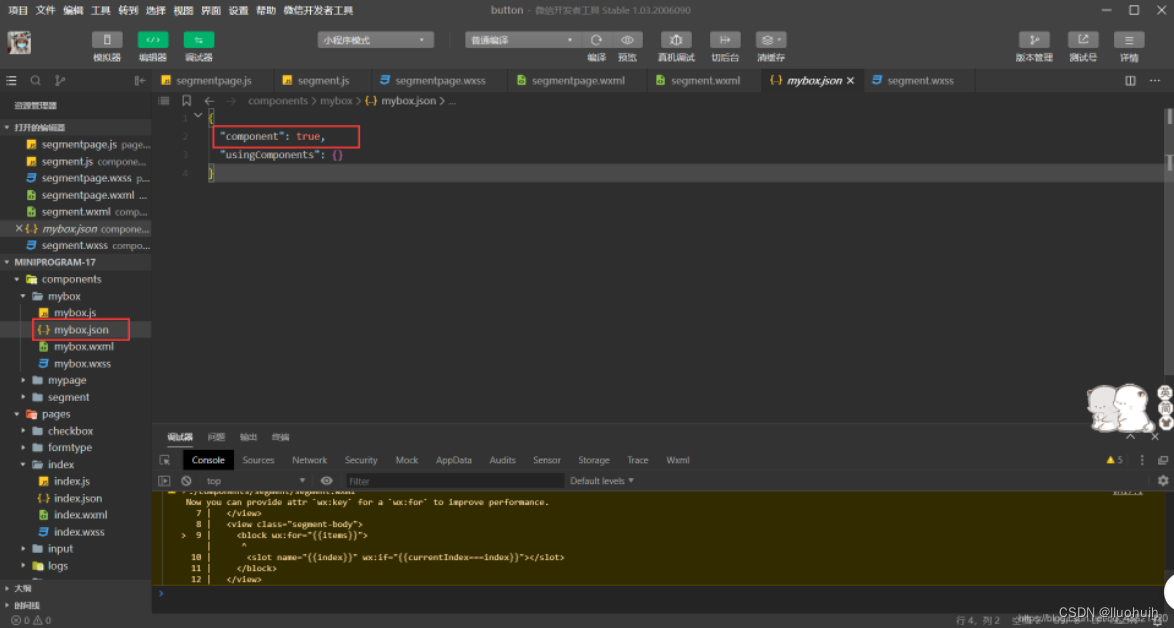 下面开始例子:
1、组件页面 index.wxml
<view class="components-modal-overlay-view" style="width: {{ windowWidth }}px; height: {{ windowHeight }}px; display: {{ show ? 'block' : 'none' }};"></view>
<view class="col-center" style="width: {{ windowWidth }}px; height: {{ windowHeight }}px; display: {{ show ? 'flex' : 'none' }};">
<view>
<view class="components-modal-close-view" style="display: {{ showCloseIcon ? 'block' : 'none' }};">
<image bindtouchend="hideCusModal" src="./images/close-white2x.png" style="width: 24px; height: 24px;"></image>
</view>
<view class="{{ showContentStyle ? 'components-modal-content-view' : '' }}">
<slot></slot>
</view>
</view>
</view>
2、组件样式 index.wxss
.components-modal-overlay-view {
background-color: #000000;
opacity: 0.5;
position: fixed;
z-index: 10;
}
.components-modal-close-view {
text-align: right;
margin-bottom: 5px;
}
.col-center {
position: fixed;
z-index: 11;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.components-modal-content-view {
background: #FFFFFF;
border-radius: 8px;
display: flex;
flex-direction: column;
justify-content: center;
padding: 20px;
}
3、组件json配置 index.json
{
"component": true,
"usingComponents": {}
}
4、组件页面的js index.js
Component({
options: {
styleIsolation: 'isolated'
},
data: {
windowWidth: 0,
windowHeight: 0,
},
ready: function() {
var _this = this;
wx.getSystemInfo({
success: function(res) {
_this.setData({
windowWidth: res.windowWidth,
windowHeight: res.windowHeight,
});
}
});
},
properties: {
showCloseIcon: {
type: Boolean,
value: true
},
showContentStyle: {
type: Boolean,
value: true
},
show: {
type: Boolean,
value: false
},
},
methods: {
hideCusModal: function(){
this.setData({
show: false,
});
}
}
})
5、组件js modal.js
const defaultOptions = {
show: false,
selector: '#cus-modal',
showCloseIcon: true,
showContentStyle: true,
};
let currentOptions = Object.assign({}, defaultOptions);
function getContext() {
const pages = getCurrentPages();
return pages[pages.length - 1];
}
const Modal = (options) => {
options = Object.assign(Object.assign({}, currentOptions), options);
const context = options.context || getContext();
const modal = context.selectComponent(options.selector);
delete options.context;
delete options.selector;
if (modal) {
modal.setData(options);
wx.nextTick(() => {
modal.setData({ show: true });
});
}
else {
console.warn('未找到 cus-modal 节点,请确认 selector 及 context 是否正确');
}
};
Modal.show = (options) => Modal(options);
export default Modal;
6、使用方法 需要用到modal的页面引入modal组件:
{
"usingComponents": {
"cus-modal": "../../components/modal/index"
}
}
页面加入modal节点:
<cus-modal id="testModal">
<view style="text-align: center;">
</view>
</cus-modal>
在页面的js中弹出modal窗口:
import Modal from '../../components/modal/modal';
Modal.show({
selector: '#testModal'
});
|