一、前言
本文主要用于介绍指示器和文字边距的问题,其余用法可以参考文末的链接参考官方用法
二、依赖配置
implementation 'com.google.android.material:material:1.5.0'
三、TabLayout的简单使用
<com.google.android.material.tabs.TabLayout
android:id="@+id/tablayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
public class MainActivity extends AppCompatActivity {
private TabLayout tabLayout;
private String[] titles = new String[]{"最新","热门","我的"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tabLayout = (TabLayout) findViewById(R.id.tablayout);
}
private void initView(){
for(int i=0;i<titles.length;i++){
tabLayout.addTab(tabLayout.newTab());
}
for(int i=0;i<titles.length;i++){
tabLayout.getTabAt(i).setText(titles[i]);
}
}
}
效果如下: 
四、自定义Tab内容
TabLayout 允许自定义tab,这里我们模仿原来的UI,只是给tab增加个背景色 item.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@android:color/holo_blue_light"
android:gravity="center">
<TextView
android:id="@+id/textview"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="14sp"/>
</LinearLayout>
main.xml
<com.google.android.material.tabs.TabLayout
android:id="@+id/tablayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
private void setCustomIcon() {
tabLayout2 = (TabLayout) findViewById(R.id.tablayout2);
for(int i=0;i<titles.length;i++){
tabLayout2.addTab(tabLayout2.newTab());
}
for(int i=0;i<titles.length;i++){
tabLayout2.getTabAt(i).setCustomView(makeTabView(i));
}
}
private View makeTabView(int position){
View tabView = LayoutInflater.from(this).inflate(R.layout.item,null);
TextView textView = tabView.findViewById(R.id.textview);
textView.setText(titles[position]);
return tabView;
}
效果如下:  这里会发现,这个写的自定义的View是无法充满整个Tab的,另外把item.xml 的布局使用match_parent 也是不能的。
五、修改指示器离文本的距离
这里有两种方式修改,一种是修改item.xml 的高度,第二就是修改TabLayout ,这里采用第二种。思路都是一样的
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@android:color/holo_blue_light"
android:gravity="center"
android:paddingBottom="0dp"
>
<TextView
android:id="@+id/textview"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="14sp"
android:layout_marginLeft="8dp"/>
</LinearLayout>
<com.google.android.material.tabs.TabLayout
android:id="@+id/tablayout2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:background="@color/white"
app:tabPaddingTop="50dp"
app:tabPaddingBottom="50dp"/>
效果如下: 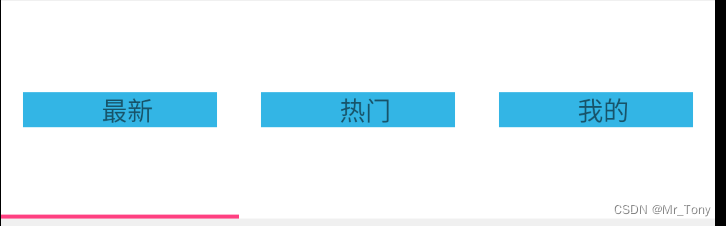
这里需要注意的是padding需要同时写app:tabPaddingTop 和app:tabPaddingBottom ,否则会出现另外一种效果,这里以只写app:tabPaddingBottom 为例 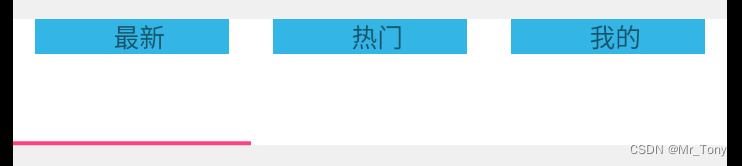 可以看到上面的默认边距也都没有了。说到这里假如想实现一种需求,就是上下边距都为0的效果,这种效果目前是没办法通过将app:tabPaddingTop 和app:tabPaddingBottom 置为0实现的。只能修改TabLayout 的android:layout_height="30dp" 这里需要写具体值。值的计算可以自己通过一次次的运行进行看那个值合适,也可以通过代码获取自定义View的高度进行赋值。这里就不进行详细说了。实现的效果如下:

六、修改指示器的宽度
上面可以看到指示器的宽度是铺满整个tab的,假设不想要铺满整个tab的话。需要进行特殊处理,示例如下: tab_indicator_layer.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:gravity="center">
<shape>
<size android:width="40dp" android:height="2dp"/>
</shape>
</item>
</layer-list>
<com.google.android.material.tabs.TabLayout
android:id="@+id/tablayout2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:background="@color/white"
app:tabIndicator="@drawable/tab_indicator_layer"
app:tabIndicatorColor="@android:color/holo_blue_light"
app:tabPaddingTop="20dp"
app:tabIndicatorFullWidth="false"
app:tabPaddingBottom="20dp"/>
效果如下 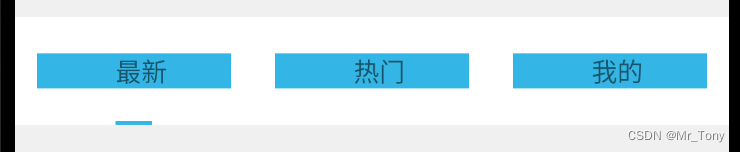
七、复杂的指示器效果
有时候不想使用这种指示器效果,那么需要设置app:tabBackground 。代码如下: tab_bg.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:gravity="center">
<shape>
<corners android:radius="5dp" />
<stroke
android:width="1dp"
android:color="@color/colorAccent" />
</shape>
</item>
</layer-list>
tab_indicator_selector.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:gravity="center">
<shape>
<size android:width="40dp" android:height="6dp"/>
</shape>
</item>
</layer-list>
<com.google.android.material.tabs.TabLayout
android:id="@+id/tablayout2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:background="@color/white"
app:tabBackground="@drawable/tab_indicator_selector"
app:tabIndicatorFullWidth="false"
app:tabIndicatorHeight="0dp"
app:tabPaddingBottom="20dp"
app:tabPaddingTop="20dp"
app:tabRippleColor="@android:color/transparent" />
效果如下: 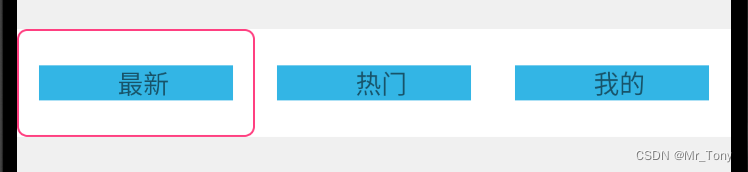
八、参考链接
- 【ANDROID】TABLAYOUT 自定义指示器 INDICATOR 样式
- Tabs
|