先展示页面样例: 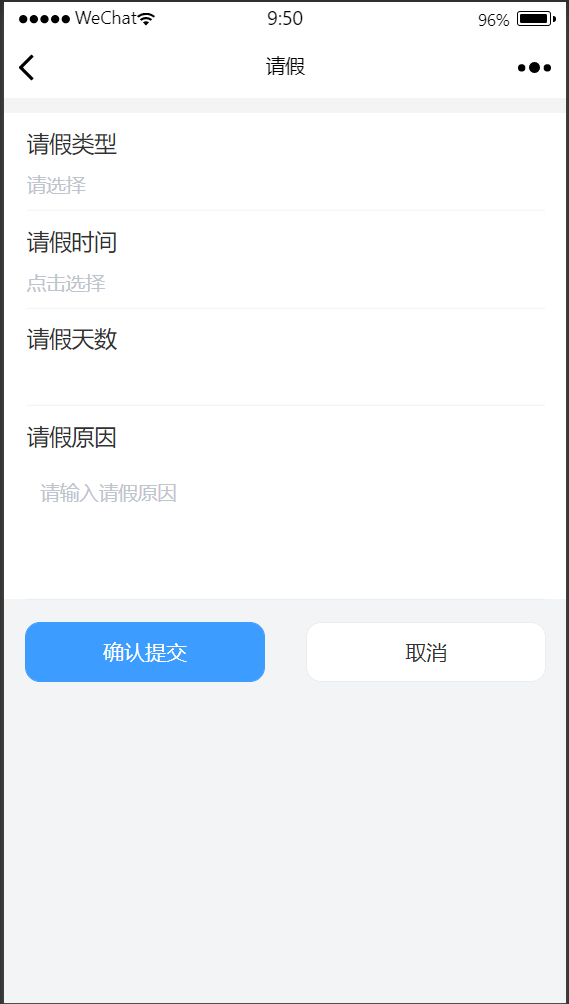 这是一个填写表单,在确认提交里写提交表单post接口。 api: 
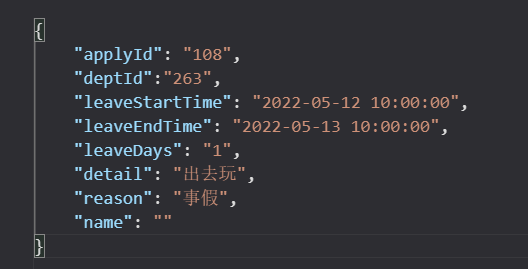 H5
<template>
<view class="app-container">
<u-gap height="10" bgColor="#f4f4f5"></u-gap>
<!-- 表单 -->
<view>
<!-- 注意,如果需要兼容微信小程序,最好通过setRules方法设置rules规则 -->
<u--form class="leave-form" labelPosition="left" :model="leaveInfo" :rules="rules" ref="form1">
<!-- 请假类型 -->
<u-form-item labelWidth="80" label="请假类型" prop="leaveInfo.reason" @click="showType = true" ref="item1">
</u-form-item>
<u--input v-model="leaveInfo.reason" disabled disabledColor="white" placeholder="请选择" border="none">
</u--input>
<u-line></u-line>
<!-- 请假时间 -->
<u-form-item labelWidth="80" label="请假时间" prop="leaveInfo.date" @click="showCalendar=true" ref="item1">
</u-form-item>
<u--input v-model="leaveInfo.date" disabled disabledColor="white" placeholder="点击选择" border="none">
</u--input>
<u-line></u-line>
<!-- 请假天数 -->
<u-form-item labelWidth="80" label="请假天数" prop="leaveInfo.number" ref="item1">
</u-form-item>
<u--input v-model="leaveInfo.number" disabled disabledColor="white" border="none" placeholder="">
</u--input>
<u-line></u-line>
<!-- 请假原因 -->
<u-form-item labelWidth="80" label="请假原因" prop="leaveInfo.detail" ref="item1" border="none">
</u-form-item>
<u--textarea v-model="leaveInfo.detail" border="none" placeholder="请输入请假原因"></u--textarea>
<u-line></u-line>
</u--form>
</view>
<!-- 提交按钮 -->
<view class="submit-content">
<u-button class="submit-button" type="primary" text="确认提交" @click="submit()"></u-button>
<u-button class="submit-button" text="取消" @click="cancelSubmit()"></u-button>
</view>
<!-- 选择类型 -->
<u-picker :show="showType" :columns="leaveType" title="请假类型" @cancel="cancel" @confirm="confirmType">
</u-picker>
<!-- 选择时间 -->
<u-calendar :show="showCalendar" :mode="mode" @confirm="confirmDate" @close="closeCalendar">
</u-calendar>
</view>
</template>
C3:
<style>
page {
background-color: #f3f4f6;
}
.uni-input-placeholder {
font-size: 26rpx;
line-height: 30rpx;
padding-bottom: 18rpx;
}
.uni-textarea-placeholder {
font-size: 26rpx;
}
.leave-form {
background-color: white;
padding: 0rpx 30rpx;
}
.submit-content {
display: flex;
align-items: center;
justify-content: center;
width: 100%;
padding: 30rpx 0;
}
.submit-button {
padding: 20rpx;
width: 320rpx;
border-radius: 20rpx;
}
</style>
JS
<script>
import config from '../../config'
export default {
data() {
return {
leaveInfo: {
reason: '',
date: '',
number: '',
detail: '',
},
rules: {},
showType: false,
leaveType: [
['事假', '休假', '病假']
],
showCalendar: false,
mode: 'range',
leaveStartTime: "",
leaveEndTime: "",
leaveNumber: "",
applyId: '',
}
},
onShow() {
uni.setStorageSync('applyId', '108')
this.applyId = uni.getStorageSync('applyId')
},
methods: {
cancel() {
this.showType = false;
},
confirmType(e) {
this.leaveInfo.reason = e.value[0];
this.showType = false;
},
confirmDate(e) {
this.leaveStartTime = e[0];
this.leaveEndTime = e[e.length - 1];
this.leaveInfo.date = this.leaveStartTime + "到" + this.leaveEndTime;
let startDate = Date.parse(this.leaveStartTime);
let endDate = Date.parse(this.leaveEndTime);
let days = (endDate - startDate) / (1 * 24 * 60 * 60 * 1000);
this.leaveNumber = days;
this.leaveInfo.number = days + "天";
this.showCalendar = false;
},
closeCalendar() {
this.showCalendar = false;
},
submit() {
let that = this;
let param = {};
param.applyId = that.applyId;
param.reason = that.leaveInfo.reason;
param.leaveStartTime = that.leaveStartTime;
param.leaveEndTime = that.leaveEndTime;
param.leaveDays = that.leaveNumber;
param.detail = that.leaveInfo.detail;
console.log(param);
if (param.reason === undefined || param.reason === "") {
uni.showToast({
icon: 'none',
title: '请假类型不能为空'
})
return;
}
if (param.leaveDays === undefined || param.leaveDays === "") {
uni.showToast({
icon: 'none',
title: '请假时间不能为空'
})
return;
}
if (param.detail === undefined || param.detail === "") {
uni.showToast({
icon: 'none',
title: '请假原因不能为空'
})
return;
}
uni.request({
url: config.baseUrl + "/app/attendance/leave/add",
method: 'POST',
data: param,
success: (res) => {
if (res.data.code === 200) {
uni.showToast({
icon: 'success',
title: '提交成功'
})
setTimeout(function() {
uni.redirectTo({
url: '/pages/process/attendance'
})
}, 1000);
} else {
uni.showToast({
title: '请重试',
icon: 'none'
});
}
}
})
},
cancelSubmit() {
this.$router.push('/pages/process/attendance');
}
}
}
</script>
|