NSThread是什么?
众所周知在iOS多线程开发主要有四种方式,NSThread就是其中一种。 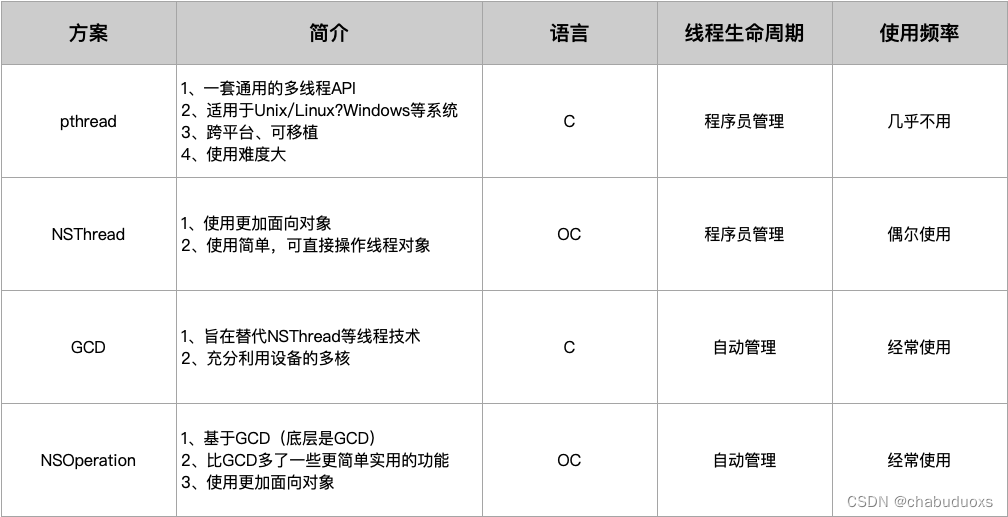 下面是apple官方给出的解释 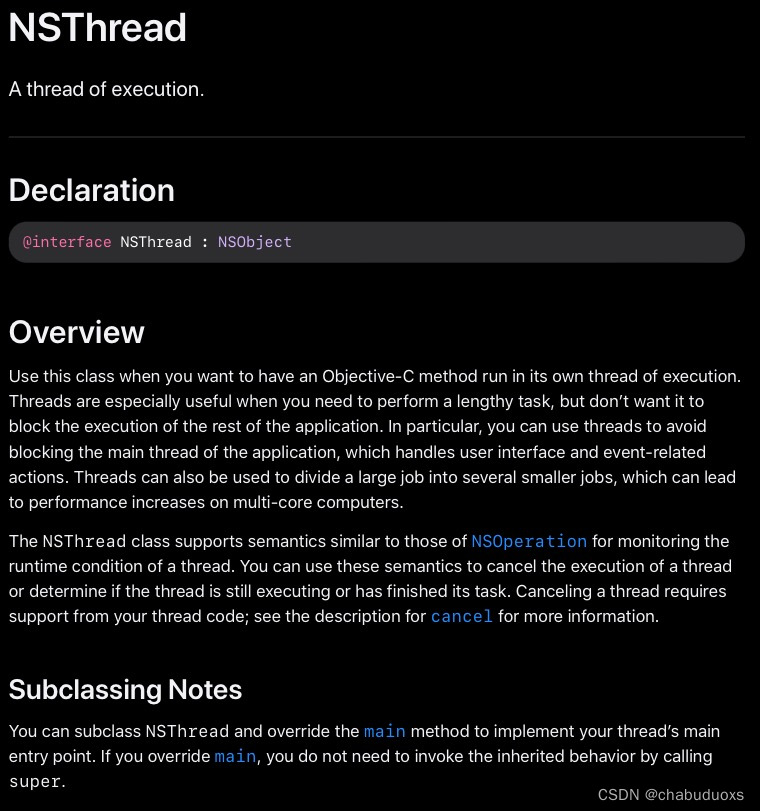 可以看出NSThread是apple封装的一个线程类,开发人员可以对线程进行操作,并且可以监控线程状态。 NSThread 是基于线程使用,轻量级的多线程编程方法(相对GCD 和NSOperation ),一个NSThread 对象代表一个线程
NSThread的使用
NSThread的创建
该类的创建方法比较简单,大致可以分为动态和静态两种方法。
- 1.可以动态创建(实例方法)初始化NSThread对象,需要自己调用- (void)start方法启动线程。
NSThread *firstThread = [[NSThread alloc] initWithTarget:self selector:@selector(sellTicket:) object:nil];
firstThread.name = @"thread1";
[firstThread start];
- 2.也可以通过NSThread的静态方法(类方法)快速创建并自动启动新线程
[NSThread detachNewThreadSelector:@selector(pressBack:) toTarget:self withObject:@"thread4"];
- 3.此外NSObject基类对象还提供了隐式快速创建performSelector,自动启动新线程
[self performSelectorInBackground:@selector(threadRun) withObject:@"thread5"];
一些线程通信
NSThread的一些属性
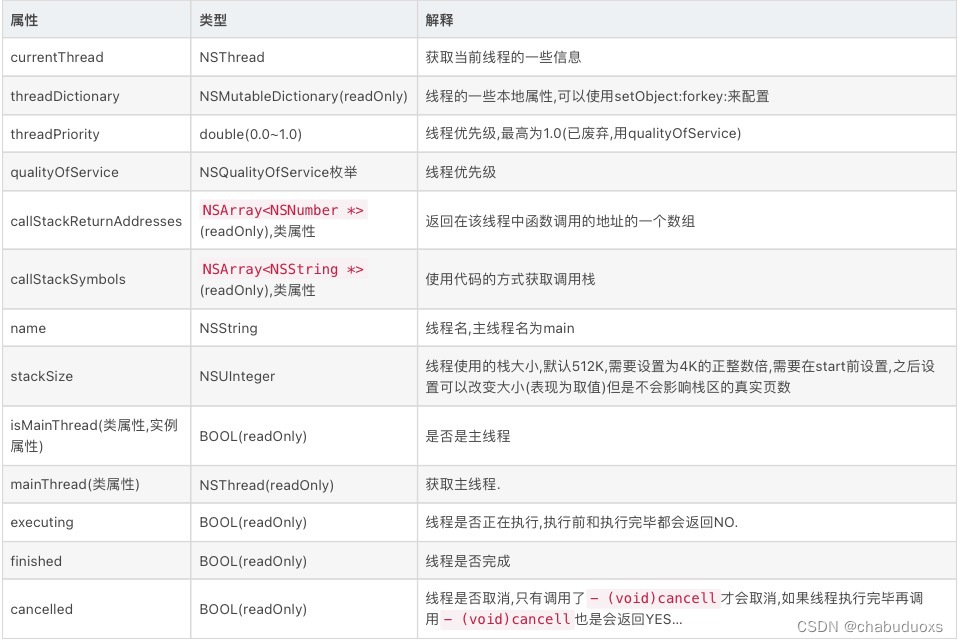
简单例子,售票处理
假设我们需要卖一百张电影票,我们在售票处同时开了三个窗口售票
{
NSThread *firstThread = [[NSThread alloc] initWithTarget:self selector:@selector(sellTicket:) object:nil];
firstThread.name = @"thread1";
NSThread *secondThread = [[NSThread alloc] initWithTarget:self selector:@selector(sellTicket:) object:nil];
secondThread.name = @"thread2";
NSThread *thirdThread = [[NSThread alloc] initWithTarget:self selector:@selector(sellTicket:) object:nil];
thirdThread.name=@"thread3";
[firstThread start];
[secondThread start];
[thirdThread start];
[NSThread currentThread];
}
- (void)sellTicket:(NSThread*) thread {
while (self.ticketsCount > 0) {
NSThread *thread = [NSThread currentThread];
[NSThread sleepForTimeInterval:2];
self.ticketsCount -- ;
NSLog(@"当前线程:%@\n剩余票数为:%d ",thread.name, self.ticketsCount);
}
}
看一下运行结果,发现数据会混乱,这是因为我们没有实现线程同步,造成了资源竞争。 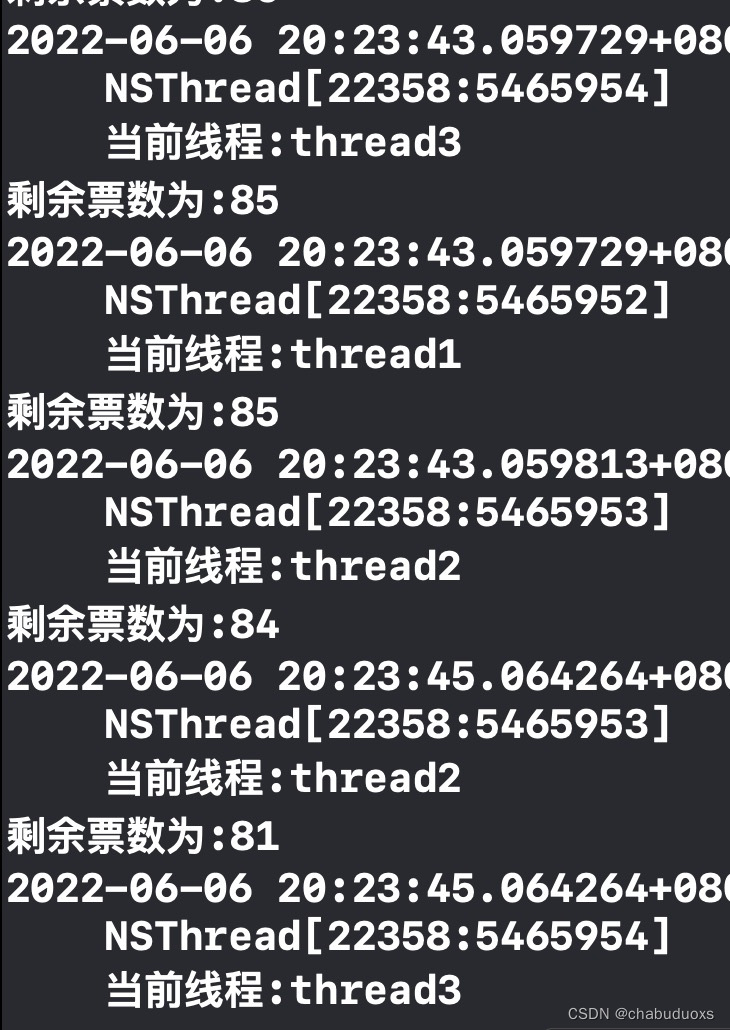 下面我们可以实现线程同步:
- 第一种方式
@synchronized(对象) 关键字也就是互斥锁 ,在新的线程访问时,如果发现其他线程正在执行锁定的代码,新线程进入休眠 .
- (void)sellTicket:(NSThread*) thread {
while (self.ticketsCount > 0) {
@synchronized(self) {
NSThread *thread = [NSThread currentThread];
[NSThread sleepForTimeInterval:2];
self.ticketsCount -- ;
NSLog(@"当前线程:%@\n剩余票数为:%d ",thread.name, self.ticketsCount);
}
}
}
- 还可以使用NSLock
NSLock 是 Cocoa 提供给我们最基本的锁对象,也是经常使用的,除 lock 和 unlock 方法外,NSLock 还提供了 tryLock 和 lockBeforeDate: 两个方法,前一个方法会尝试加锁,如果锁不可用(已经被锁住),并不会阻塞线程,直接返回 NO。lockBeforeDate: 方法会在所指定 Date 之前尝试加锁,如果在指定时间之前都不能加锁,则返回 NO。
self.threadLock = [[NSLock alloc]init];
while (self.ticketsCount > 0) {
[self.threadLock lock];
NSThread *thread = [NSThread currentThread];
[NSThread sleepForTimeInterval:2];
self.ticketsCount -- ;
NSLog(@"当前线程:%@\n剩余票数为:%zd ",thread.name, self.ticketsCount);
[self.threadLock unlock];
}
|