一.项目功能展示
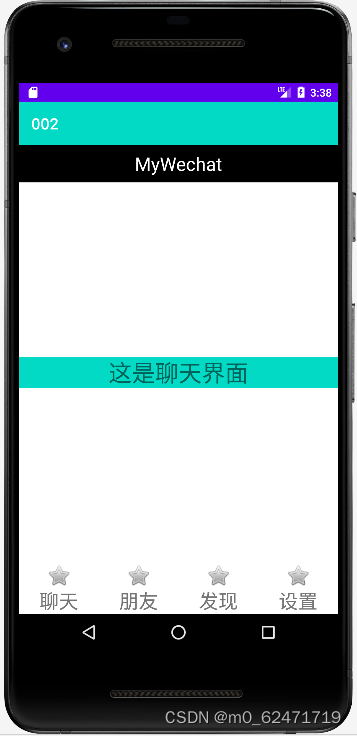
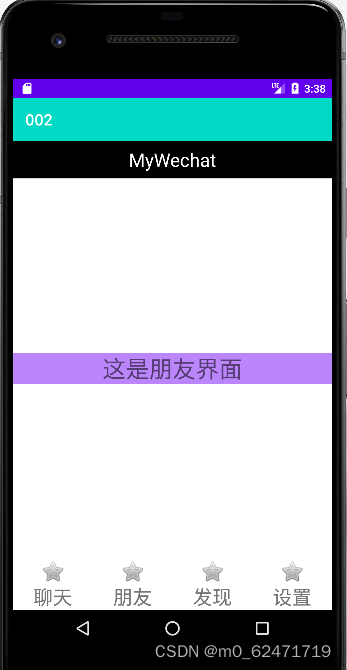
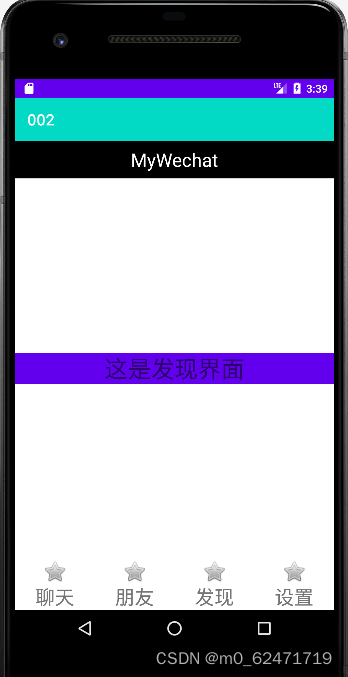
?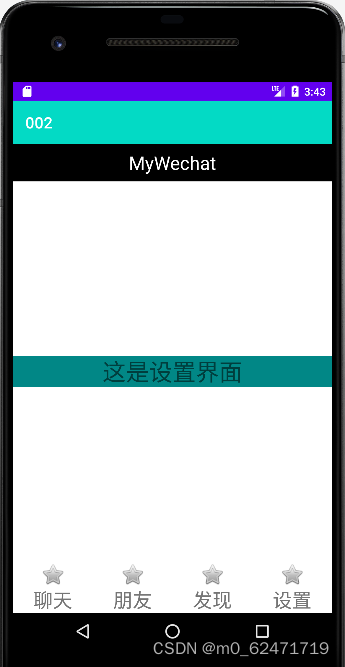
?二.项目实现具体方法
1.页面布局
?在layout中创建header.xml 文件实现顶部app标题
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="48dp"
android:layout_weight="1"
android:background="#000000"
android:gravity="center"
android:text="MyWechat"
android:textColor="@color/white"
android:textSize="24sp" />
</LinearLayout>
?
创建bottom.xml实现门户界面底部选择框
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<LinearLayout
android:id="@+id/linearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
app:srcCompat="@android:drawable/btn_star" />
<TextView
android:id="@+id/textView21"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="聊天"
android:layout_gravity="center"
android:textSize="25sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
app:srcCompat="@android:drawable/btn_star" />
<TextView
android:id="@+id/textView22"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="朋友"
android:layout_gravity="center"
android:textSize="25sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
app:srcCompat="@android:drawable/btn_star" />
<TextView
android:id="@+id/textView23"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="发现"
android:layout_gravity="center"
android:textSize="25sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
app:srcCompat="@android:drawable/btn_star" />
<TextView
android:id="@+id/textView24"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="设置"
android:layout_gravity="center"
android:textSize="25sp" />
</LinearLayout>
</LinearLayout>
同样创建 fragment_1.xml ,fragment_2.xml, fragment_3.xml, fragment_4.xml 来作为四个不同的中间内容框
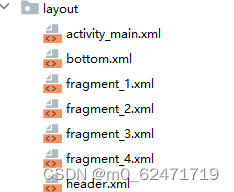
?
?以聊天界面为例展示中间内容框的部分代码
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Fragment1">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:background="#FF03DAC5"
android:gravity="center"
android:text="这是聊天界面"
android:textSize="30sp" />
</FrameLayout>
2.页面切换
创建四个fragment 布局文件对应四个中间内容文件实现界面布局的切换
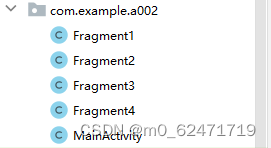
以聊天界面布局为例展示部分代码?
package com.example.a002;
import android.os.Bundle;
import androidx.fragment.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
/**
* A simple {@link Fragment} subclass.
* Use the {@link Fragment1#newInstance} factory method to
* create an instance of this fragment.
*/
public class Fragment1 extends Fragment {
// TODO: Rename parameter arguments, choose names that match
// the fragment initialization parameters, e.g. ARG_ITEM_NUMBER
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
// TODO: Rename and change types of parameters
private String mParam1;
private String mParam2;
public Fragment1() {
// Required empty public constructor
}
/**
* Use this factory method to create a new instance of
* this fragment using the provided parameters.
*
* @param param1 Parameter 1.
* @param param2 Parameter 2.
* @return A new instance of fragment Fragment1.
*/
// TODO: Rename and change types and number of parameters
public static Fragment1 newInstance(String param1, String param2) {
Fragment1 fragment = new Fragment1();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_1, container, false);
}
}
3.java文件完整对所有布局调用及控制
定义四个Fragment类对象,建立FragmentManager 类对象fragmentManager,
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
linearLayout1 = findViewById(R.id.linearLayout1);
linearLayout2 = findViewById(R.id.linearLayout2);
linearLayout3 = findViewById(R.id.linearLayout3);
linearLayout4 = findViewById(R.id.linearLayout4);
fragment1 =new Fragment1();
fragment2 =new Fragment2();
fragment3 =new Fragment3();
fragment4 =new Fragment4();
fragmentManager = getSupportFragmentManager();
initial();
hide();
linearLayout1.setOnClickListener(this);
linearLayout2.setOnClickListener(this);
linearLayout3.setOnClickListener(this);
linearLayout4.setOnClickListener(this);
showFragment(fragment1);
}
onclick()对四个控件进行监听 select()函数实现对于单个布局的显示
@Override
public void onClick(View view) {
int i = 0;
switch (view.getId()){
case R.id.linearLayout1:select(1);break;
case R.id.linearLayout2:select(2);break;
case R.id.linearLayout3:select(3);break;
case R.id.linearLayout4:select(4);break;
}
}
public void select(int i){
hide();
switch (i){
case 1:showFragment(fragment1);break;
case 2:showFragment(fragment2);break;
case 3:showFragment(fragment3);break;
case 4:showFragment(fragment4);break;
}
}
hide()函数,用于隐藏四个Fragement,达到隐藏中间内容框的效果避免四个内容框叠加显示
private void showFragment(Fragment fragment){
fragmentTransaction.show(fragment);
}
private void hide() {
fragmentTransaction = fragmentManager.beginTransaction().hide(fragment1)
.hide(fragment2).hide(fragment3).hide(fragment4);
fragmentTransaction.commit();
}
源码链接:
002: Android Studio微信界面设置
|