一.设计目标:
1.完成基本的微信页面布局设计,包括顶部显示栏,和底部导航栏(四个tab页包括微信、朋友、发现、我)。
2.利用fragment(分段)、activity完善门户页面的框架设计,实现四个页面的切换,点击相应的导航键,便可以切换到该页面,相应的图标也发生改变,并对控件进行监听。
二.设计流程:
1.制作底部导航栏bottom.xml
?点击 res-layout 新建xml文件
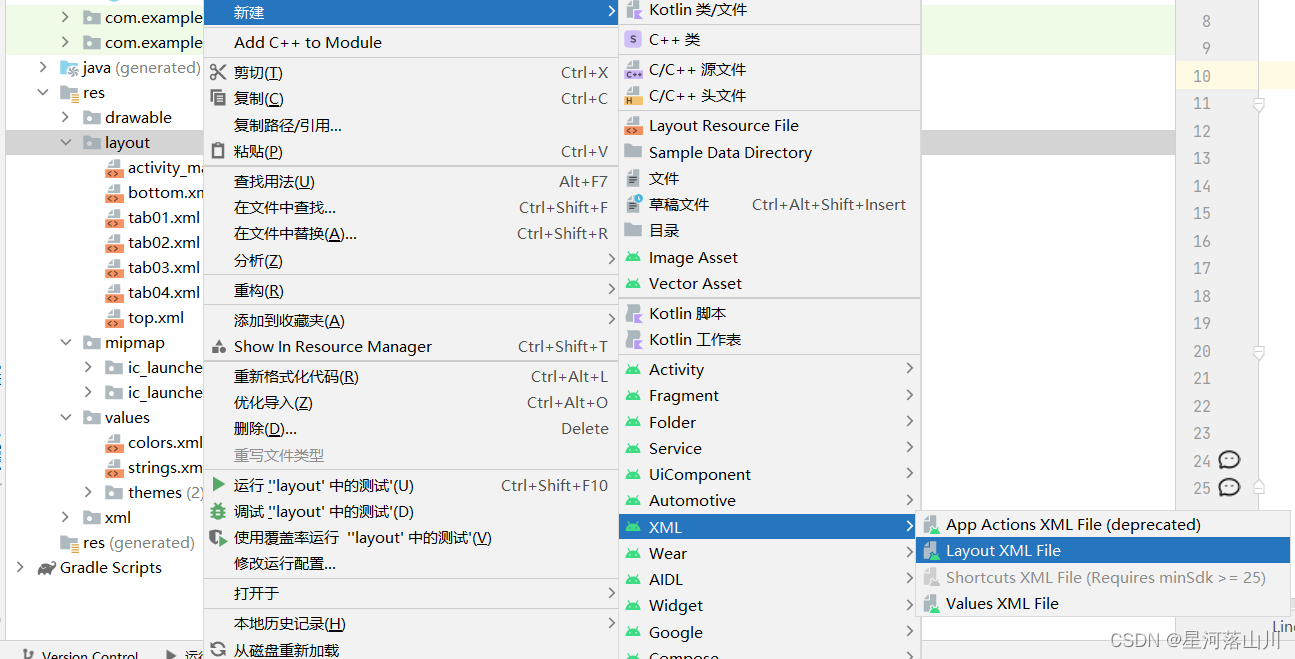
bottom平均分成四块,每块由一个图片和一个文字构成。可以用水平的LinearLayout中再包裹一个竖直的LinearLayout,被包裹的每个LinearLayout里包含一个ImageButton和TextView控件。
准备两组八张图标存放在res-drawable。
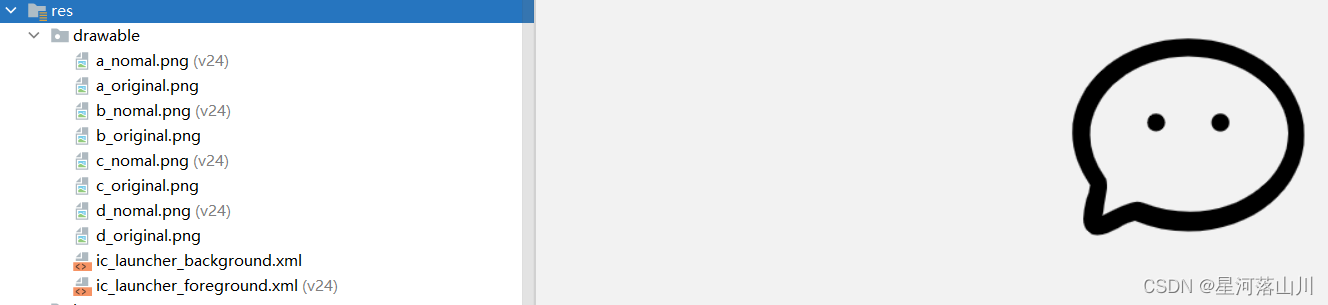
?添加bottom.xml配置代码:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="100dp"
android:background="@color/purple_200"
android:clickable="false"
android:orientation="horizontal">
<LinearLayout
android:id="@+id/tab_weixin"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:clickable="true"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="57dp"
android:src="@drawable/a_original"
tools:srcCompat="@drawable/a_original" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="微信"
android:textColor="@color/black"
android:textSize="23sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/tab_pengyou"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:clickable="true"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView1"
android:layout_width="103dp"
android:layout_height="60dp"
android:src="@drawable/b_original"
tools:srcCompat="@drawable/b_original" />
<TextView
android:id="@+id/textView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="朋友"
android:textColor="@color/black"
android:textSize="23sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/tab_faxian"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:clickable="true"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView2"
android:layout_width="103dp"
android:layout_height="63dp"
android:src="@drawable/c_original"
tools:srcCompat="@drawable/c_original" />
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="发现"
android:textColor="@color/black"
android:textSize="23sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/tab_shezhi"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:clickable="true"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView3"
android:layout_width="match_parent"
android:layout_height="62dp"
android:src="@drawable/d_original"
tools:srcCompat="@drawable/d_original" />
<TextView
android:id="@+id/textView3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="我"
android:textColor="@color/black"
android:textSize="23sp" />
</LinearLayout>
</LinearLayout>
代码解析:
外部LinearLayout:android:orientation=“horizontal”,布局中组件的排列方式,有horizontal(水平)和vertical(垂直)两种方式。
内部ImageView:tools:srcCompat="@drawable/tab_weixin_normal",vector绘制图片的地址。 ?
功能说明(布局展示):
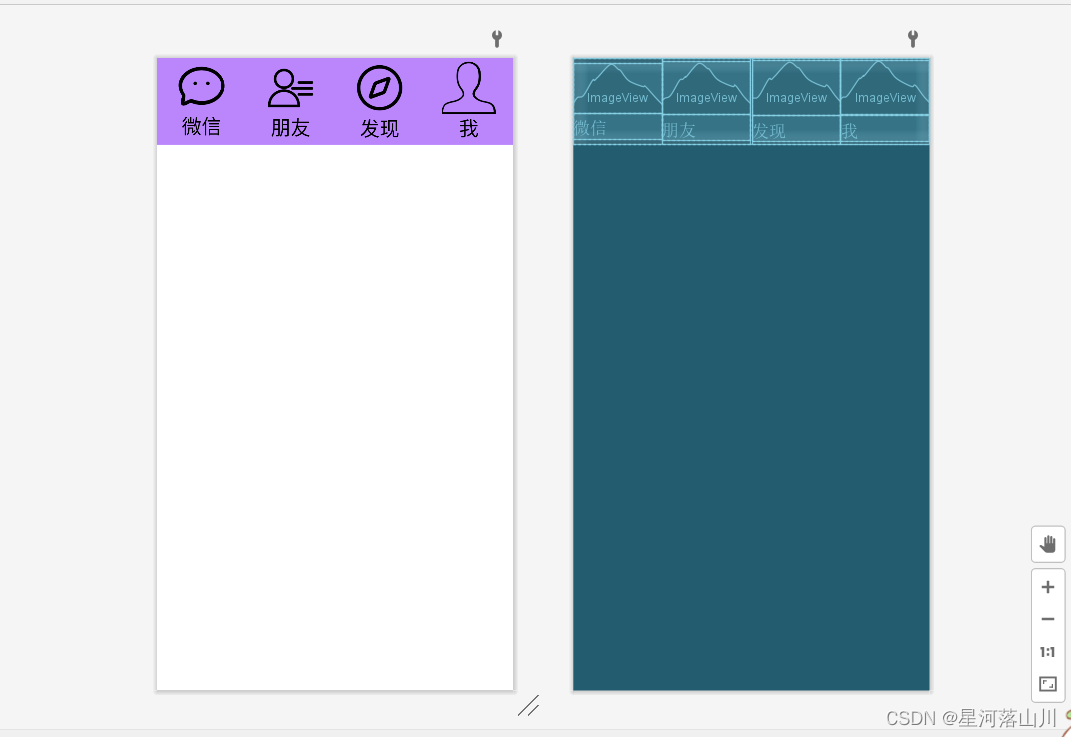
2.制作顶部的top.xml,在res-layout新建一个xml文件:
top.xml的配置代码:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/purple_200">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:text="微信"
android:textColor="@color/black"
android:textSize="30sp" />
</LinearLayout>
?代码解析:
android:id="@+id/textView2",为组件设置一个资源id,在java文件中可以通过findViewById(id)找到该组件。
android:layout_width=“wrap_content”,布局的宽度,通常不直接写数字,用wrap_content(组件实际大小),fill_parent或者match_parent填满父容器。
android:layout_height=“wrap_content”,布局的高度,参数同上。
android:layout_weight=“1”,用来等比例地划分区域。
android:background="@color/purple_200",为组件设置一个背景图片,或者直接用颜色覆盖。
android:gravity=“center”,表示textView中的文字相对于TextView的对齐方式。
android:text=“微信”,要显示的文字。
android:textColor="@color/black",设置文字的颜色。
android:textSize=“30sp” />,设置文字的大小。
功能说明(布局展示):
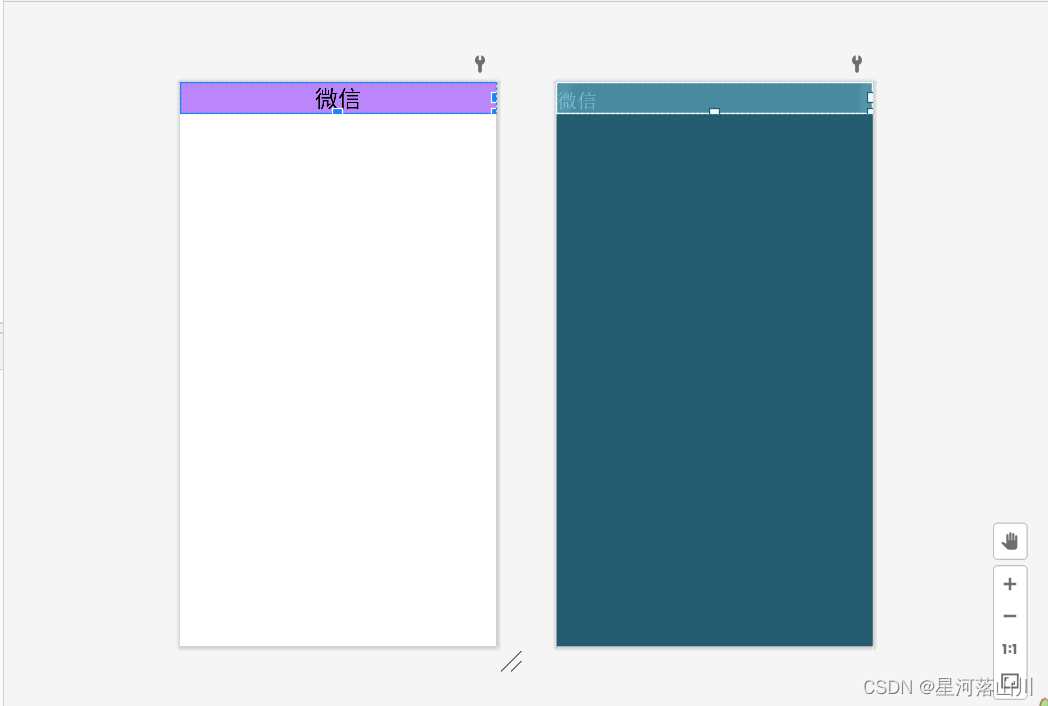
3.activity_main的配置
添加activity_main.xml代码:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<include layout="@layout/top"/>
<FrameLayout
android:id="@+id/content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1">
</FrameLayout>
<include layout="@layout/bottom" />
</LinearLayout>
?代码解析:
相关的属性作用和bottom.xml以及top.xml类似。
功能说明(布局展示):
对主页面显示的内容布局。整个主页面使用竖直的LinerLayout,将top.xml和bottom.xml导入,以及中间内容content(对应Fragment)。
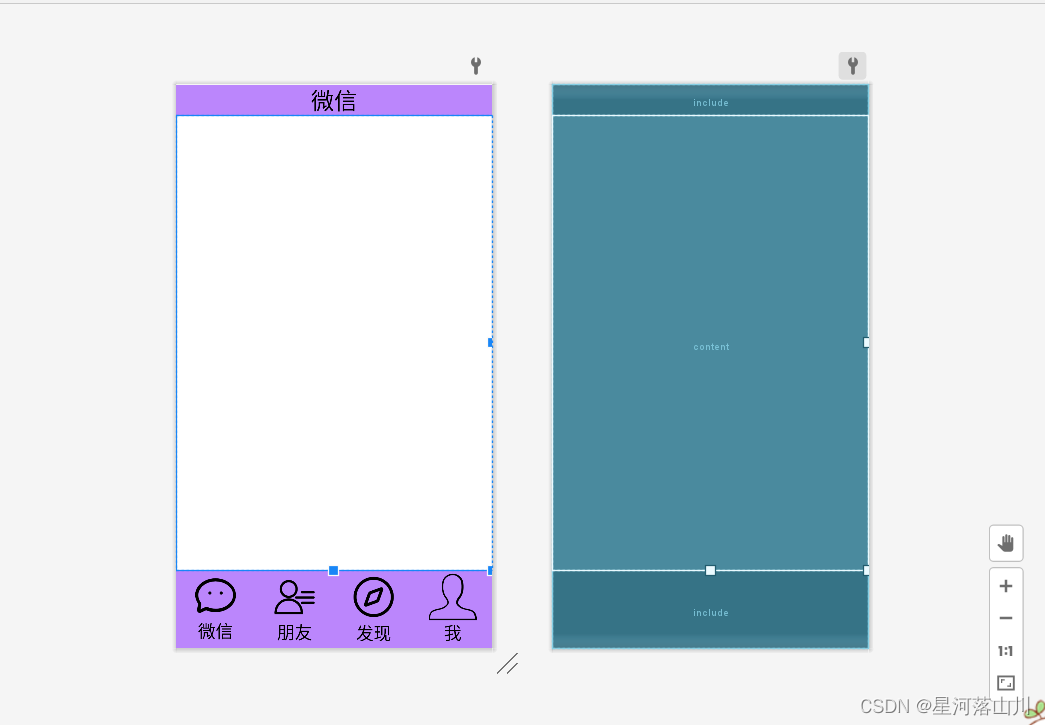
4.创建Fragment
在java目录下创建四个fragment类,WeChatBlankFragment(微信)、FriBlankFragment(朋友)、FoundBlankFragment(发现)、MeBlankFragment(我),系统会自动在res-layout目录下生成四个对应的xml文件(可自主命名),对应bottom的四个按钮。
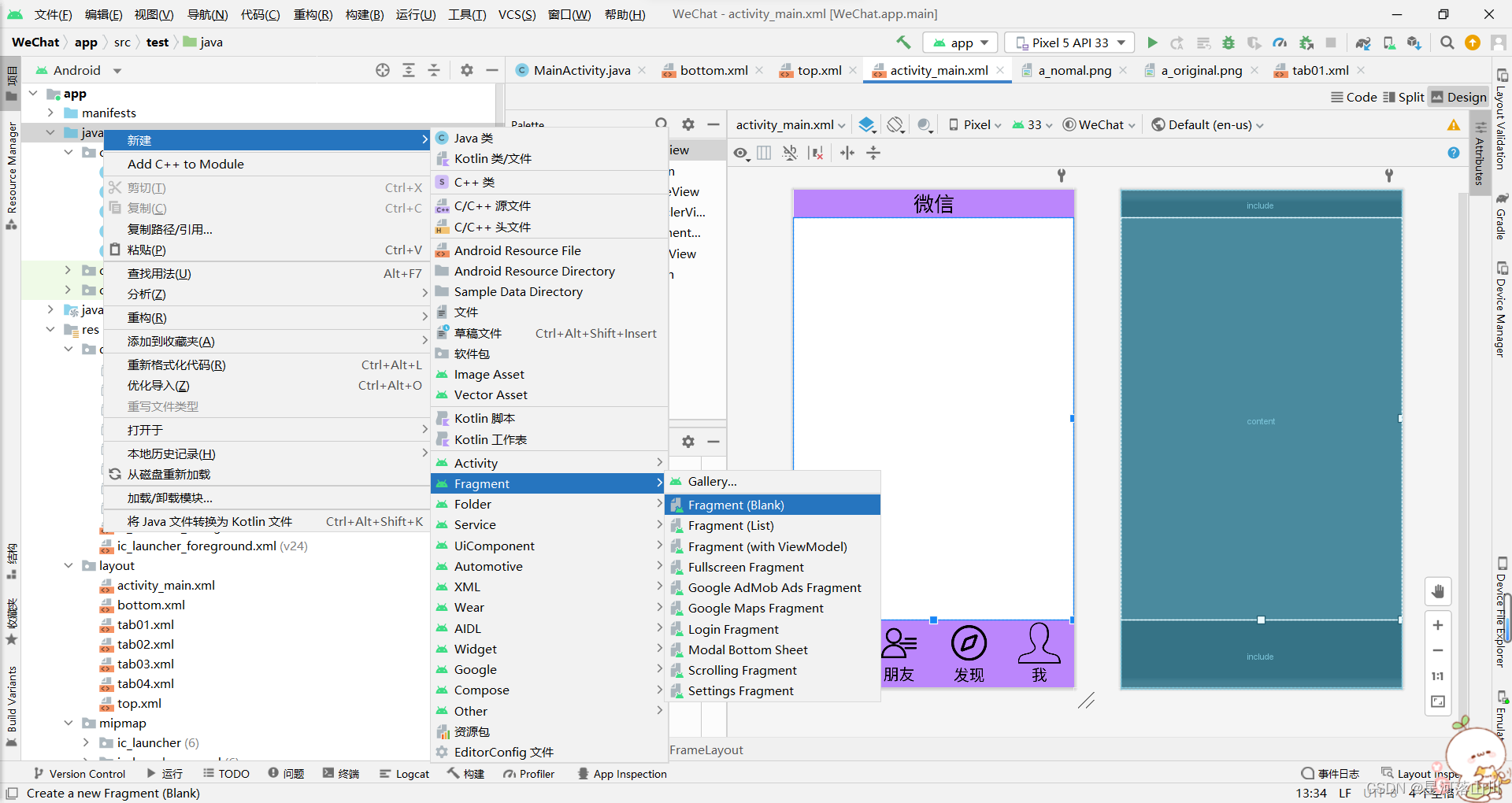
以FriBlankFragment(朋友)为例,配置相关代码
package com.example.wechat;
import android.os.Bundle;
import androidx.fragment.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class FriBlankFragment extends Fragment {
public FriBlankFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.tab02, container, false);
}
}
配置FriBlankFragment对应的xml文件代码
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".FriBlankFragment">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:text="朋友界面"
android:textSize="30sp"/>
</LinearLayout>
5.配置MainActivity
5.1声明所需要的变量
private Fragment hTab01 = new WeChatBlankFragment();
private Fragment hTab02 = new FriBlankFragment();
private Fragment hTab03 = new FoundBlankFragment();
private Fragment hTab04 = new MeBlankFragment();
private LinearLayout hTabWeChat;
private LinearLayout hTabFrd;
private LinearLayout hTabContact;
private LinearLayout hTabMe;
private ImageView imageWeixin,imagepengyou,imagefaxian, imagewo;
private FragmentManager fm;
private TextView textView;
代码解析:
Fragment变量:对应创建的Fragment? ,FragmentManager变量:管理Fragment的类 ImageView变量:对应之前创建的ImageView,LinearLayout变量:对应之前创建的LinearLayout
5.2 利用FragmentManager对Fragment进行管理,实现页面切换
将所有的Fragment添加进去:
private void initFragment() {
fm = getSupportFragmentManager();
FragmentTransaction transaction = fm.beginTransaction();
transaction.add(R.id.content, hTab01);
transaction.add(R.id.content, hTab02);
transaction.add(R.id.content, hTab03);
transaction.add(R.id.content, hTab04);
transaction.commit();
}
页面的切换(功能):
private void showfragment(int i){
FragmentTransaction transaction=fm.beginTransaction();
hideFragment(transaction);
switch (i){
case 0:
textView.setText("微信");
transaction.show(hTab01);
imageWeixin.setImageResource(R.drawable.a_nomal);
break;
case 1:
textView.setText("朋友");
transaction.show(hTab02);
imagepengyou.setImageResource(R.drawable.b_nomal);
break;
case 2:
textView.setText("发现");
transaction.show(hTab03);
imagefaxian.setImageResource(R.drawable.c_nomal);
break;
case 3:
textView.setText("我");
transaction.show(hTab04);
imagewo.setImageResource(R.drawable.d_nomal);
break;
default:
break;
}
transaction.commit();
}
为了使所有Fragment不会发生重叠,将所有Fragment都隐藏(功能):
private void hideFragment(FragmentTransaction transaction) {
transaction.hide(hTab01);
transaction.hide(hTab02);
transaction.hide(hTab03);
transaction.hide(hTab04);
}
5.3 完成事件的触发
事件触发代码:
private void initEvent() {
hTabWeChat.setOnClickListener(this);
hTabFrd.setOnClickListener(this);
hTabContact.setOnClickListener(this);
hTabMe.setOnClickListener(this);
}
点击相应按钮的响应函数
public void onClick(View v) {
resetImage();
switch (v.getId()){
case R.id.tab_weixin:
showfragment(0);
break;
case R.id.tab_pengyou:
showfragment(1);
break;
case R.id.tab_faxian:
showfragment(2);
break;
case R.id.tab_shezhi:
showfragment(3);
break;
default:
break;
}
5.4 实现点击后的按钮切换
public void resetImage(){
imageWeixin.setImageResource(R.drawable.a_original);
imagepengyou.setImageResource(R.drawable.b_original);
imagefaxian.setImageResource(R.drawable.c_original);
imagewo.setImageResource(R.drawable.d_original);
}
5.5 主函数调用
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
supportRequestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
textView=findViewById(R.id.textView2);
hTabWeChat =findViewById(R.id.tab_weixin);
hTabFrd =findViewById(R.id.tab_pengyou);
hTabContact =findViewById(R.id.tab_faxian);
hTabMe =findViewById(R.id.tab_shezhi);
imageWeixin=findViewById(R.id.imageView);
imagepengyou=findViewById(R.id.imageView1);
imagefaxian=findViewById(R.id.imageView2);
imagewo =findViewById(R.id.imageView3);
initEvent();
initFragment();
showfragment(0);
}
5.6 完整的MainActivity代码
package com.example.wechat;
import androidx.appcompat.app.AppCompatActivity;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import androidx.fragment.app.FragmentTransaction;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private Fragment hTab01 = new WeChatBlankFragment();
private Fragment hTab02 = new FriBlankFragment();
private Fragment hTab03 = new FoundBlankFragment();
private Fragment hTab04 = new MeBlankFragment();
private LinearLayout hTabWeChat;
private LinearLayout hTabFrd;
private LinearLayout hTabContact;
private LinearLayout hTabMe;
private ImageView imageWeixin,imagepengyou,imagefaxian, imagewo;
private FragmentManager fm;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
supportRequestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
textView=findViewById(R.id.textView2);
hTabWeChat =findViewById(R.id.tab_weixin);
hTabFrd =findViewById(R.id.tab_pengyou);
hTabContact =findViewById(R.id.tab_faxian);
hTabMe =findViewById(R.id.tab_shezhi);
imageWeixin=findViewById(R.id.imageView);
imagepengyou=findViewById(R.id.imageView1);
imagefaxian=findViewById(R.id.imageView2);
imagewo =findViewById(R.id.imageView3);
initEvent();
initFragment();
showfragment(0);
}
private void initFragment() {
fm = getSupportFragmentManager();
FragmentTransaction transaction = fm.beginTransaction();
transaction.add(R.id.content, hTab01);
transaction.add(R.id.content, hTab02);
transaction.add(R.id.content, hTab03);
transaction.add(R.id.content, hTab04);
transaction.commit();
}
private void initView() {
hTabWeChat = (LinearLayout) findViewById(R.id.tab_weixin);
hTabFrd = (LinearLayout) findViewById(R.id.tab_pengyou);
hTabContact = (LinearLayout) findViewById(R.id.tab_faxian);
hTabMe = (LinearLayout) findViewById(R.id.tab_shezhi);
}
private void showfragment(int i){
FragmentTransaction transaction=fm.beginTransaction();
hideFragment(transaction);
switch (i){
case 0:
textView.setText("微信");
transaction.show(hTab01);
imageWeixin.setImageResource(R.drawable.a_nomal);
break;
case 1:
textView.setText("朋友");
transaction.show(hTab02);
imagepengyou.setImageResource(R.drawable.b_nomal);
break;
case 2:
textView.setText("发现");
transaction.show(hTab03);
imagefaxian.setImageResource(R.drawable.c_nomal);
break;
case 3:
textView.setText("我");
transaction.show(hTab04);
imagewo.setImageResource(R.drawable.d_nomal);
break;
default:
break;
}
transaction.commit();
}
private void hideFragment(FragmentTransaction transaction) {
transaction.hide(hTab01);
transaction.hide(hTab02);
transaction.hide(hTab03);
transaction.hide(hTab04);
}
private void initEvent() {
hTabWeChat.setOnClickListener(this);
hTabFrd.setOnClickListener(this);
hTabContact.setOnClickListener(this);
hTabMe.setOnClickListener(this);
}
public void onClick(View v) {
resetImage();
switch (v.getId()){
case R.id.tab_weixin:
showfragment(0);
break;
case R.id.tab_pengyou:
showfragment(1);
break;
case R.id.tab_faxian:
showfragment(2);
break;
case R.id.tab_shezhi:
showfragment(3);
break;
default:
break;
}
}
public void resetImage(){
imageWeixin.setImageResource(R.drawable.a_original);
imagepengyou.setImageResource(R.drawable.b_original);
imagefaxian.setImageResource(R.drawable.c_original);
imagewo.setImageResource(R.drawable.d_original);
}
}
三 运行结果
3.1运行环境:
计算机型号:联想拯救者 R7000P 2020H
处理器:AMD Ryzen 7 4800H with Radeon Graphics
内存:16.0 GB (15.9 GB 可用)
操作系统:Windows 11 家庭中文版
浏览器:Microsoft Edge 102.0.1245.30(64-bit)
编译器:Android Studio 2021.2.0.0
3.2 运行结果:
运行后点击微信、朋友、发现、我的效果,可以看到界面和图标都发生了相应的切换。
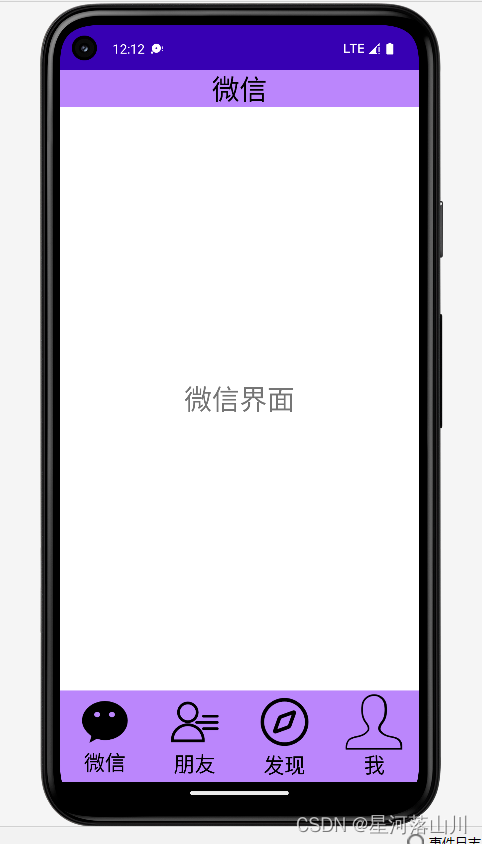 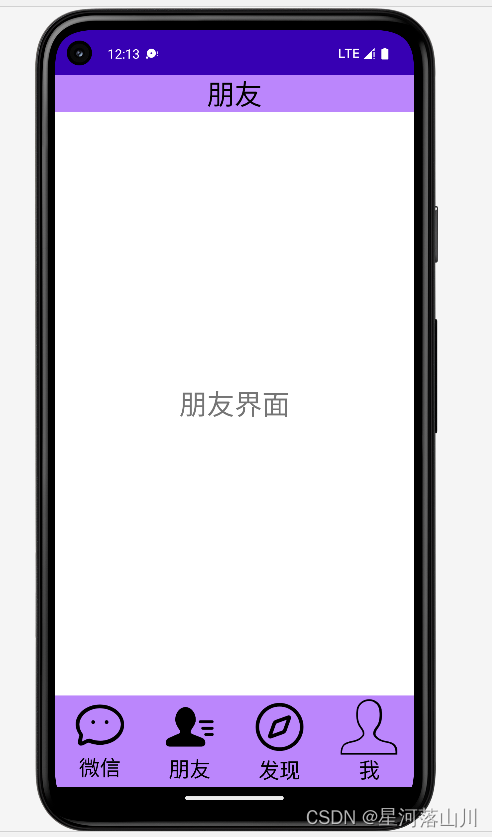 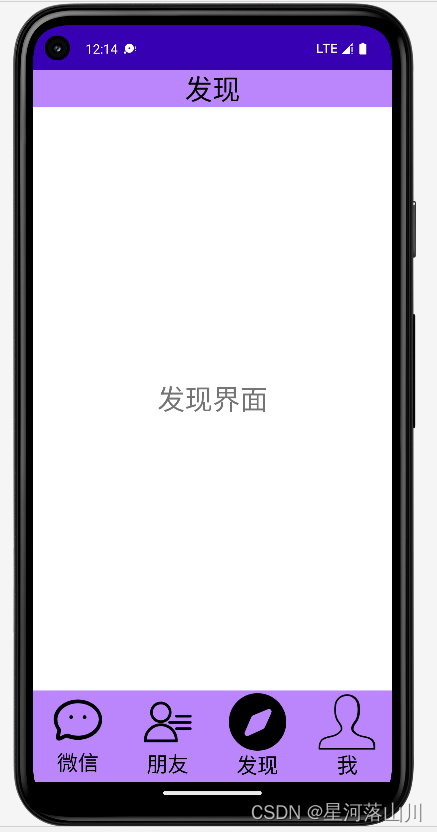 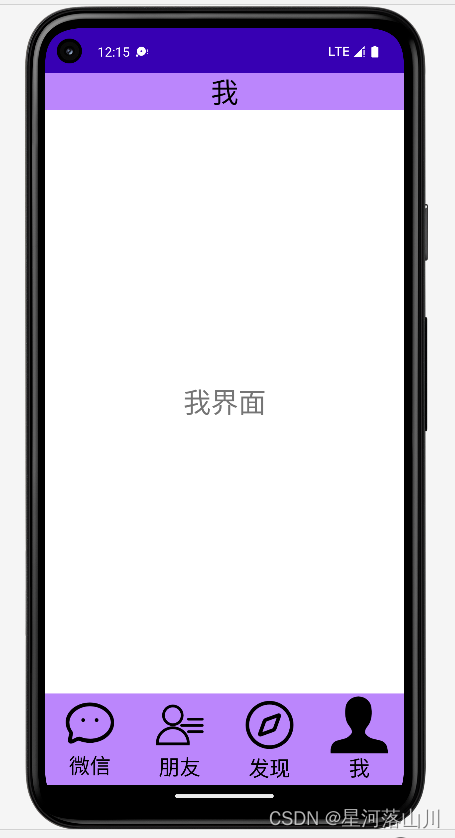
?四 源码仓库
WeChat: 微信门户页面设计(第一次作业) (gitee.com) https://gitee.com/xiaohutongxue80/WeChat
?
?
?
?
?
|