类微信的门户页面框架设计
设计目标
根据课程教学内容完成类微信的门户页面框架设计,APP最少必须包含4个tab页面。框架设计需要使用fragment,activity,不得使用UNIAPP技术进行开发(H5或者小程序)
功能说明
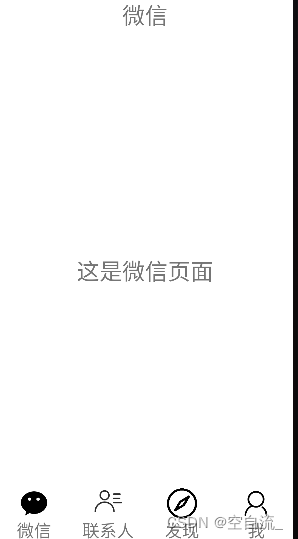
门户页面分为top、content、bottom三个部分,通过点击bottom的四个tab可以实现content的转换,同时点击的tab变为深色
代码解析
top.xml
用textview展示主页面的标题–微信,设置内容居中
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="微信"
android:gravity="center"
android:textSize="30sp"/>
</LinearLayout>
bottom.xml
一个水平LinearLayout嵌套四个垂直LinearLayout,四个垂直LinearLayout比例为1:1:1:1,每个垂直LinearLayout包含显示图片的ImageView和显示文字的TextView,并设置clickable为true、gravity为bottom
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="horizontal">
<LinearLayout
android:id="@+id/LinearLayoutWeixinFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="bottom"
android:clickable="true"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="40dp"
app:srcCompat="@drawable/image_wechat" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="微信"
android:textSize="23sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/LinearLayoutContactFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="bottom"
android:clickable="true"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView2"
android:layout_width="match_parent"
android:layout_height="45dp"
app:srcCompat="@drawable/image_contact" />
<TextView
android:id="@+id/textView3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="联系人"
android:textSize="23sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/LinearLayoutFindFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="bottom"
android:clickable="true"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView3"
android:layout_width="match_parent"
android:layout_height="40dp"
app:srcCompat="@drawable/image_find" />
<TextView
android:id="@+id/textView4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="发现"
android:textSize="23sp"/>
</LinearLayout>
<LinearLayout
android:id="@+id/LinearLayoutMineFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="bottom"
android:clickable="true"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView4"
android:layout_width="match_parent"
android:layout_height="40dp"
app:srcCompat="@drawable/image_mine" />
<TextView
android:id="@+id/textView5"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="我"
android:textSize="23sp"/>
</LinearLayout>
</LinearLayout>
fragment_wechat.xml(fragment_contact\fragment_find\fragment_mine与此类似)
设置TextView的layout_gravity和gravity均为center,layout_gravity是用来控制TextView在父控件FrameLayout中的位置
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".WechatFragment">
<TextView
android:id="@+id/textView6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_gravity="center"
android:gravity="center"
android:text="这是微信页面"
android:textSize="30sp"/>
</FrameLayout>
activity_main.xml
通过include引入top和bottom,FrameLayout用来动态展示具体的Fragment
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layout/top"/>
<FrameLayout
android:id="@+id/content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"/>
<include layout="@layout/bottom"/>
</androidx.constraintlayout.widget.ConstraintLayout>
WechatFragment类(ContactFragment\FindFragment\MineFragment与此类似)
定义一个继承Fragment的类WechatFragment,重写onCreateView方法,调用inflater.inflate加载fragment_wechat的布局文件
public class WechatFragment extends Fragment {
public WechatFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_wechat, container, false);
}
}
MainActivity
在MainActivity主类中实现View.OnClickListener接口
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private View LinearLayout1,LinearLayout2,LinearLayout3,LinearLayout4;
private FragmentManager fragmentManager;
private ImageView image1,image2,image3,image4;
private Fragment wechatFragment = new WechatFragment();
private Fragment contactFragment = new ContactFragment();
private Fragment findFragment = new FindFragment();
private Fragment mineFragment = new MineFragment();
onCreate(),初始化四个LinearLayout并设置监听,初始化四个ImageView,调用initFragment(),showFragment()(参数为0,默认展示第一个tab的页面)
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
supportRequestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
LinearLayout1 = findViewById(R.id.LinearLayoutWeixinFragment);
LinearLayout2 = findViewById(R.id.LinearLayoutContactFragment);
LinearLayout3 = findViewById(R.id.LinearLayoutFindFragment);
LinearLayout4 = findViewById(R.id.LinearLayoutMineFragment);
LinearLayout1.setOnClickListener(this);
LinearLayout2.setOnClickListener(this);
LinearLayout3.setOnClickListener(this);
LinearLayout4.setOnClickListener(this);
image1=findViewById(R.id.imageView1);
image2=findViewById(R.id.imageView2);
image3=findViewById(R.id.imageView3);
image4=findViewById(R.id.imageView4);
initFragment();
showFragment(0);
}
initFragment(),首先获取一个fragmentManager实例用来管理fragment,通过FragmentTransaction向Activity state中添加所有Fragment,最后调用commit()提交
private void initFragment(){
fragmentManager = getSupportFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
transaction.add(R.id.content,wechatFragment)
.add(R.id.content,contactFragment)
.add(R.id.content,findFragment)
.add(R.id.content,mineFragment);
transaction.commit();
}
hideFragment()用于隐藏所有的Fragment
public void hideFragment(FragmentTransaction transaction){
transaction.hide(wechatFragment)
.hide(contactFragment)
.hide(findFragment)
.hide(mineFragment);
}
showFragment(),通过FragmentManager获取FragmentTransaction,隐藏所有的fragment,根据传入参数i选择显示相应的fragment,并将对应的drawable中的image切换为深色的image
private void showFragment(int i){
FragmentTransaction transaction = fragmentManager.beginTransaction();
hideFragment(transaction);
switch (i){
case 0:
transaction.show(wechatFragment);
image1.setImageResource(R.drawable.image_wechat2);
break;
case 1:
transaction.show(contactFragment);
image2.setImageResource(R.drawable.image_contact2);
break;
case 2:
transaction.show(findFragment);
image3.setImageResource(R.drawable.image_find2);
break;
case 3:
transaction.show(mineFragment);
image4.setImageResource(R.drawable.image_mine2);
break;
default:
break;
}
transaction.commit();
}
resetImgs()用于将所有tab的图标置为初始状态白色,实质上是切换了一个image
private void resetImgs(){
image1.setImageResource(R.drawable.image_wechat);
image2.setImageResource(R.drawable.image_contact);
image3.setImageResource(R.drawable.image_find);
image4.setImageResource(R.drawable.image_mine);
}
onClick(),首先将所有tab的图标变换为白色,通过siwtch分支响应点击事件,展示对应的fragment
@Override
public void onClick(View v) {
resetImgs();
switch (v.getId()){
case R.id.LinearLayoutWeixinFragment:
showFragment(0);
break;
case R.id.LinearLayoutContactFragment:
showFragment(1);
break;
case R.id.LinearLayoutFindFragment:
showFragment(2);
break;
case R.id.LinearLayoutMineFragment:
showFragment(3);
break;
default:
break;
}
}
}
运行展示截图
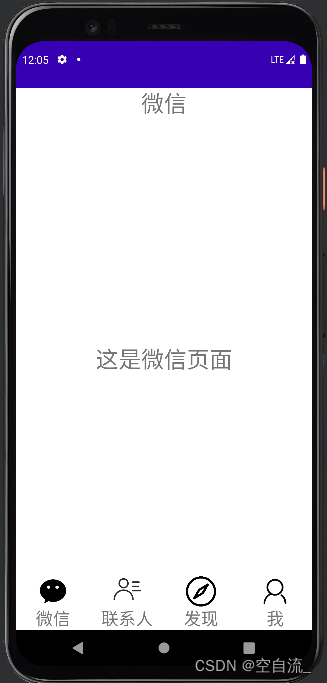 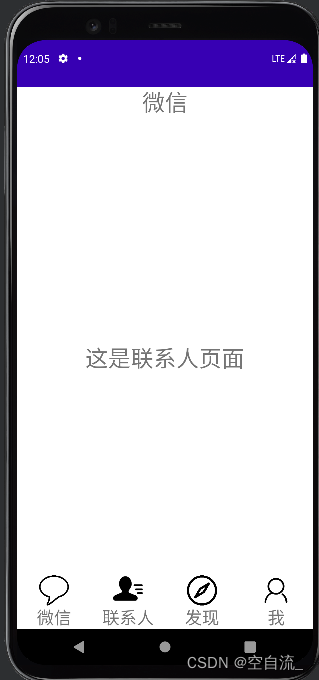 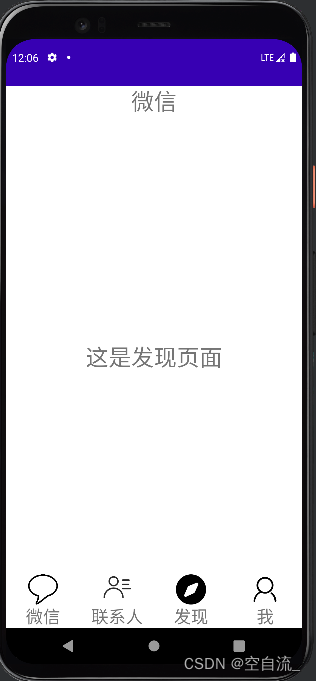 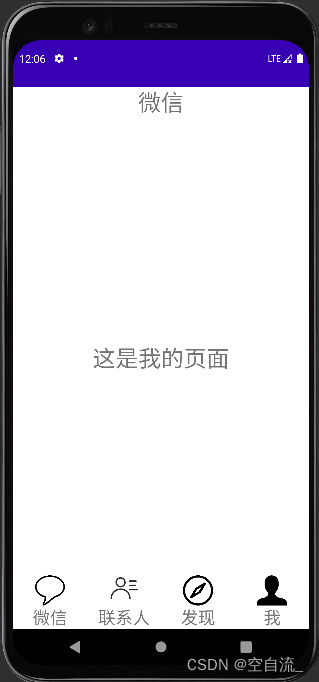
源码仓库地址
https://gitee.com/xia-minzhe/AS-Work1.git
|