制作底部界面:下面的button分成四块,每块由一个图标和一段文本构成。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/linear_bottom"
android:layout_width="match_parent"
android:layout_gravity="bottom"
android:background="@color/blue"
android:layout_height="55dp">
<LinearLayout
android:id="@+id/wx_linear"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
app:srcCompat="@drawable/p1" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="2"
android:gravity="center"
android:textColor="#fff"
android:text="目录" />
</LinearLayout>
<LinearLayout
android:id="@+id/txl_linear"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
app:srcCompat="@drawable/p2" />
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="2"
android:gravity="center"
android:text="消息"
android:textColor="#fff" />
</LinearLayout>
<LinearLayout
android:id="@+id/fx_linear"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView3"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
app:srcCompat="@drawable/p3" />
<TextView
android:id="@+id/textView3"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="2"
android:gravity="center"
android:textColor="#fff"
android:text="设置" />
</LinearLayout>
<LinearLayout
android:id="@+id/w_linear"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView4"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
app:srcCompat="@drawable/p4" />
<TextView
android:id="@+id/textView4"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="2"
android:gravity="center"
android:textColor="#fff"
android:text="微信" />
</LinearLayout>
</LinearLayout>
制作主页面
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<include
layout="@layout/top"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<FrameLayout
android:id="@+id/id_content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1">
</FrameLayout>
<include
layout="@layout/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
</LinearLayout>
在与MainActivity.java相同的目录下新建四个Fragment,每个代表不同图标的界面
private Fragment mTab01 = new weixinFragment();
private Fragment mTab02 = new frdFragment();
private Fragment mTab03 = new contactFragment();
private Fragment mTab04 = new settingFragment();
private FragmentManager fm;
private void initFragment() {
fm = getSupportFragmentManager();
FragmentTransaction transaction = fm.beginTransaction();
transaction.add(R.id.id_content,mTab01);
transaction.add(R.id.id_content,mTab02);
transaction.add(R.id.id_content,mTab03);
transaction.add(R.id.id_content,mTab04);
transaction.commit();
}
initView()函数,其中上面四个参数用来存储四个bottom图片底下的文字,下面四个参数用来存储四个图片
private void initView(){
mTabWeixin = (LinearLayout)findViewById(R.id.id_tab_weixin);
mTabFrd = (LinearLayout)findViewById(R.id.id_tab_frd);
mTabAddress = (LinearLayout)findViewById(R.id.id_tab_contact);
mTabSettings = (LinearLayout)findViewById(R.id.id_tab_settings);
mImgWeixin = (ImageButton)findViewById(R.id.id_tab_weixin_img);
mImgFrd = (ImageButton)findViewById(R.id.id_tab_frd_img);
mImgAddress = (ImageButton)findViewById(R.id.id_tab_contact_img);
mImgSettings = (ImageButton)findViewById(R.id.id_tab_settings_img);
}
对4个按钮进行监听
@Override
public void onClick(View v) {
resetimg();
switch (v.getId()){
case id.id_tab_weixin_img:
setSelect(0);
break;
case id.id_tab_frd_img:
setSelect(1);
break;
case id.id_tab_contact_img:
setSelect(2);
break;
case id.id_tab_settings_img:
setSelect(3);
break;
default:
break;
}
}
mainactivitiy.java文件
package com.example.homework1_xc;
import androidx.appcompat.app.AppCompatActivity;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import androidx.fragment.app.FragmentTransaction;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private LinearLayout linearWx,linearMF,linearBM,linearS;
private ImageView imgWx,imgTxl,imgBM,imgW,imgCur;
private TextView textWx,textTxl,textFx,textW,textCur;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
supportRequestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
initFun();
addFragment(new SetFlagment());
imgWx.setSelected(true);
textWx.setSelected(true);
}
private void initFun(){
linearWx = findViewById(R.id.wx_linear);
linearWx.setOnClickListener(this);
linearMF = findViewById(R.id.txl_linear);
linearMF.setOnClickListener(this);
linearBM = findViewById(R.id.fx_linear);
linearBM.setOnClickListener(this);
linearS = findViewById(R.id.w_linear);
linearS.setOnClickListener(this);
imgWx = findViewById(R.id.imageView);
imgTxl = findViewById(R.id.imageView2);
imgBM = findViewById(R.id.imageView3);
imgW = findViewById(R.id.imageView4);
imgCur = findViewById(R.id.imageView);
textWx = findViewById(R.id.textView);
textTxl = findViewById(R.id.textView2);
textFx = findViewById(R.id.textView3);
textW = findViewById(R.id.textView4);
textCur = findViewById(R.id.textView);
}
@Override
public void onClick(View view) {
switch (view.getId()){
case R.id.wx_linear:
changeFragment(new BookmarkFragment());
colorChange(1);
break;
case R.id.txl_linear:
changeFragment(new MessageFlagment());
colorChange(2);
break;
case R.id.fx_linear:
changeFragment(new WechatFlagment());
colorChange(3);
break;
case R.id.w_linear:
changeFragment(new SetFlagment());
colorChange(4);
break;
default:
break;
}
}
private void colorChange(int id){
textCur.setSelected(false);
imgCur.setSelected(false);
switch (id){
case 1:
imgWx.setSelected(true);
imgCur=imgWx;
textWx.setSelected(true);
textCur=textWx;
break;
case 2:
imgTxl.setSelected(true);
imgCur=imgTxl;
textTxl.setSelected(true);
textCur=textTxl;
break;
case 3:
imgBM.setSelected(true);
imgCur=imgBM;
textFx.setSelected(true);
textCur=textFx;
break;
case 4:
imgW.setSelected(true);
imgCur=imgW;
textW.setSelected(true);
textCur=textW;
break;
default:
break;
}
}
private void changeFragment(Fragment fragment){
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
transaction.replace(R.id.body, fragment);
transaction.commit();
}
private void addFragment(Fragment fragment){
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
transaction.add(R.id.body, fragment);
transaction.commit();
}
}
运行截图
?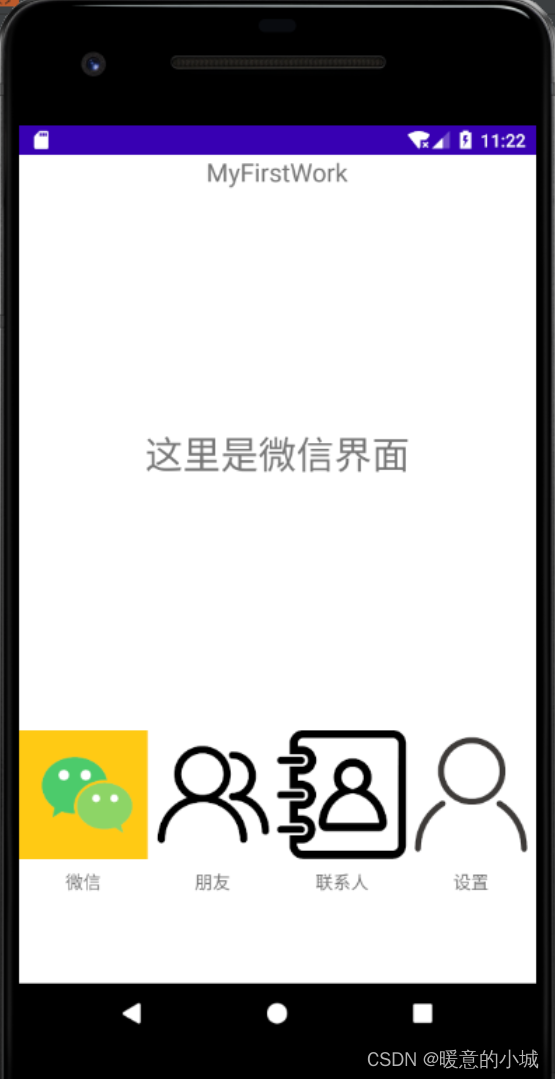
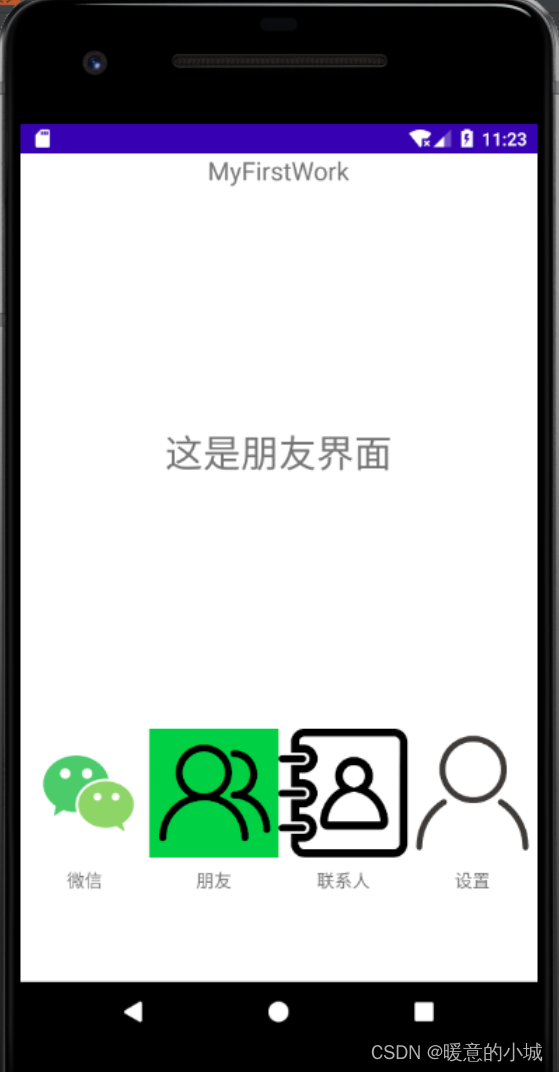
?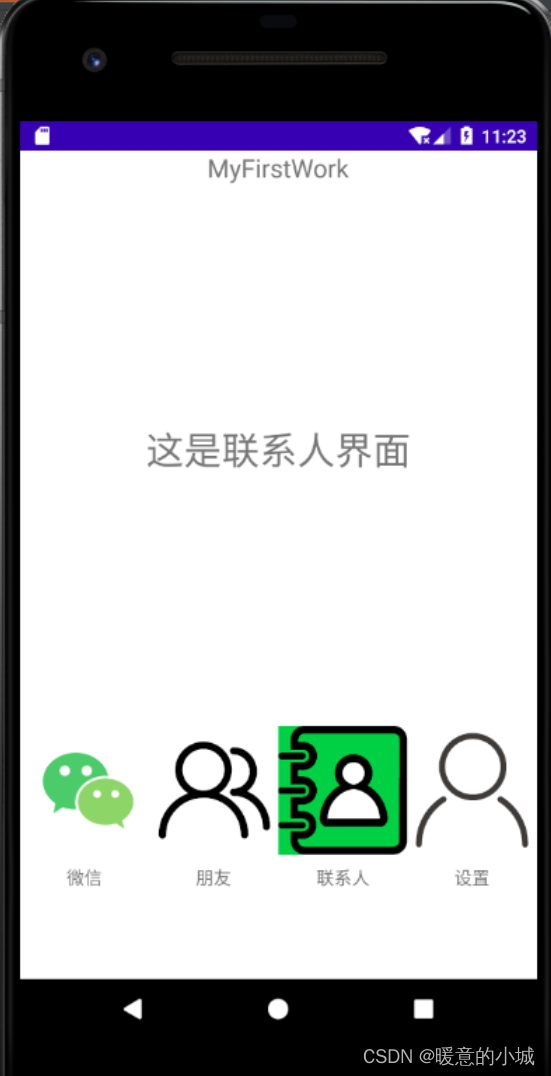
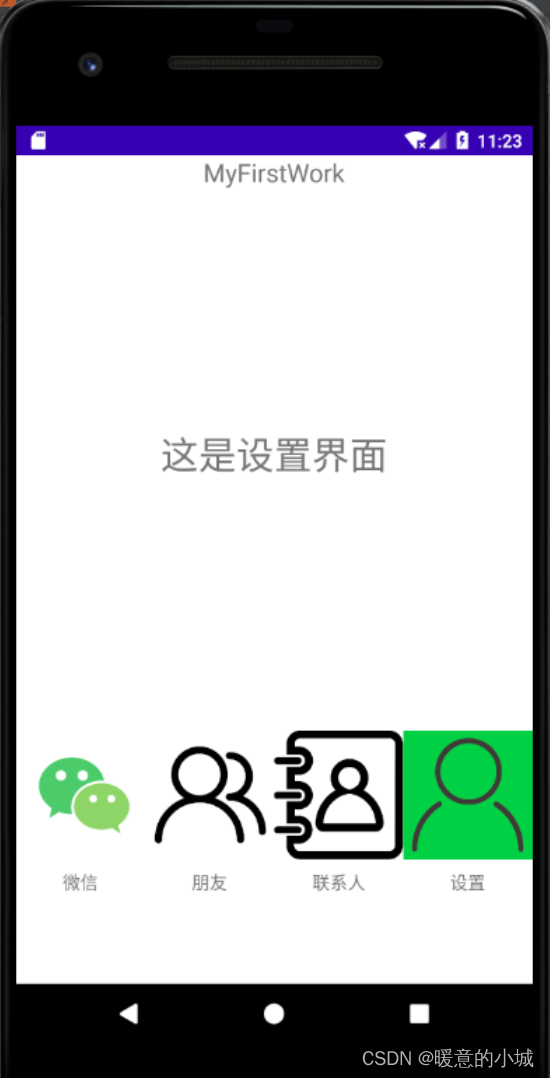
?geek地址:我的工作台 - Gitee.com