JSOMNModel库的导入
- 终端输入:cd +项目地址
- 终端输入:touch PodFile
- 打开项目工程文件夹
- 在Pod文件内输入:
- platform :ios, ‘7.0’
target ‘你的项目工程名字’ do pod ‘JSONModel’ end - 保存并关闭Pod文件
- 终端输入:pod install
- 等待安装完成
- 打开后缀为.xcw的文件即可使用JSONModel库
在导入JSONModel库的过程中,可能会出现这种情况,我是重新创建了一个文件进行导入,就导入成功了。如果出现这种情况,可以试试换一个网或者重新创建一个工程进行导入。 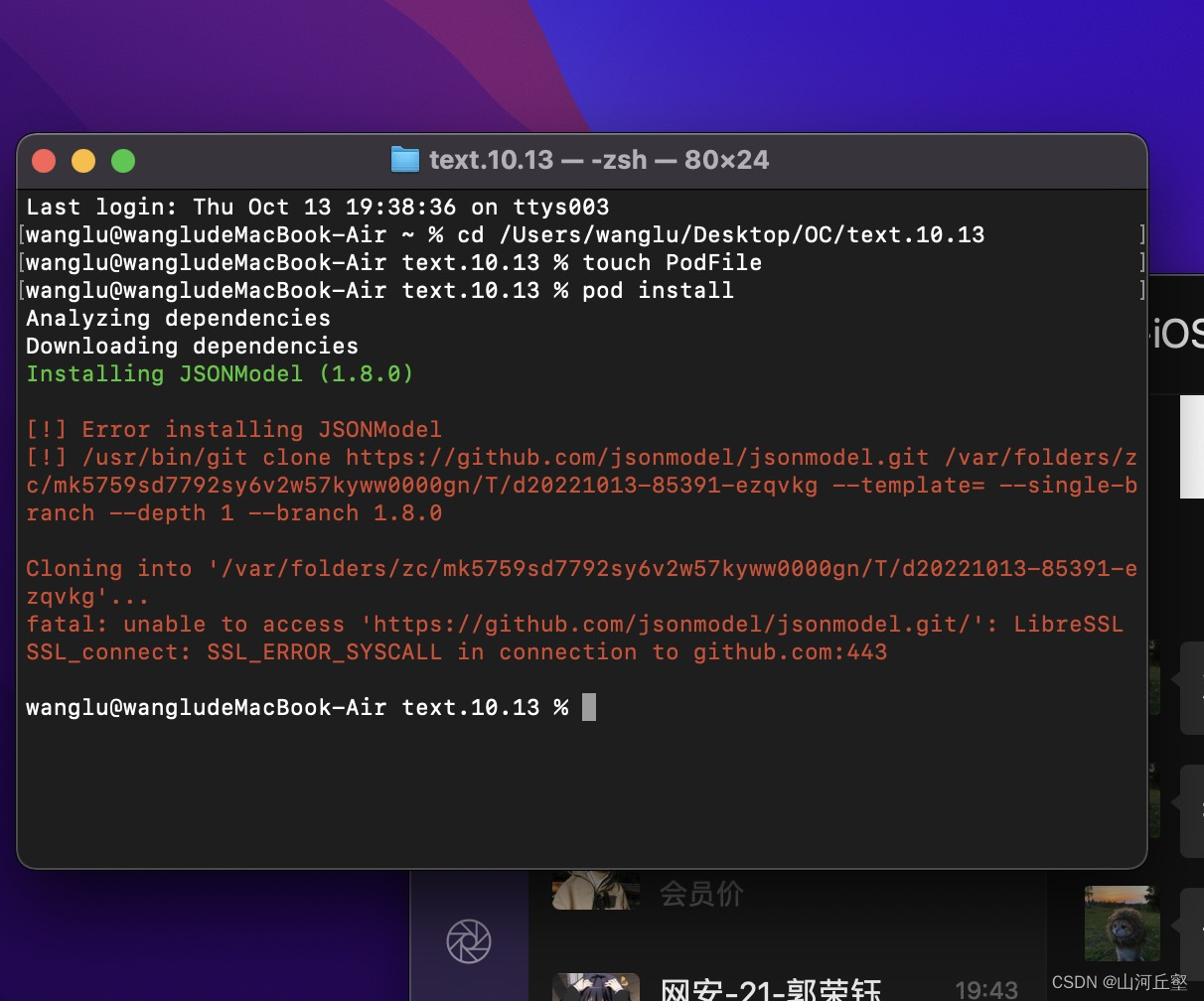
JSONModel的一些介绍
JSONModel是使用OC写的开源库,它包含了接受发送,解析JSON数据,用JSON数据驱动初始化类,检验JSON和嵌套模型等功能。 JSON是json转model的第三方开源库,当我们向服务器发送一个请求,可以通过JSONModel把数据转化成属性,使用会非常方便
JSONModel的使用
https://news-at.zhihu.com/api/4/news/latest
https://www.json.cn
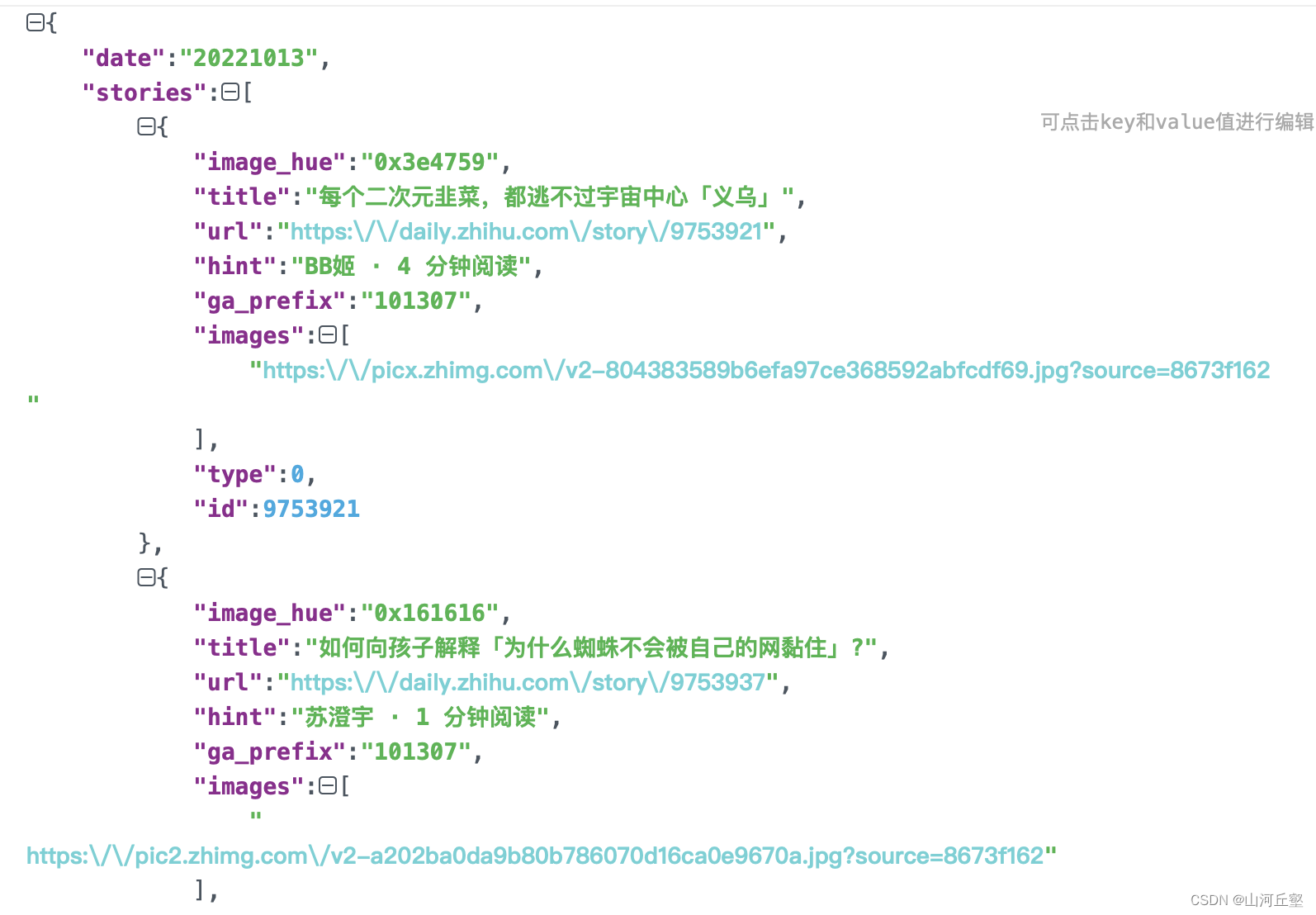 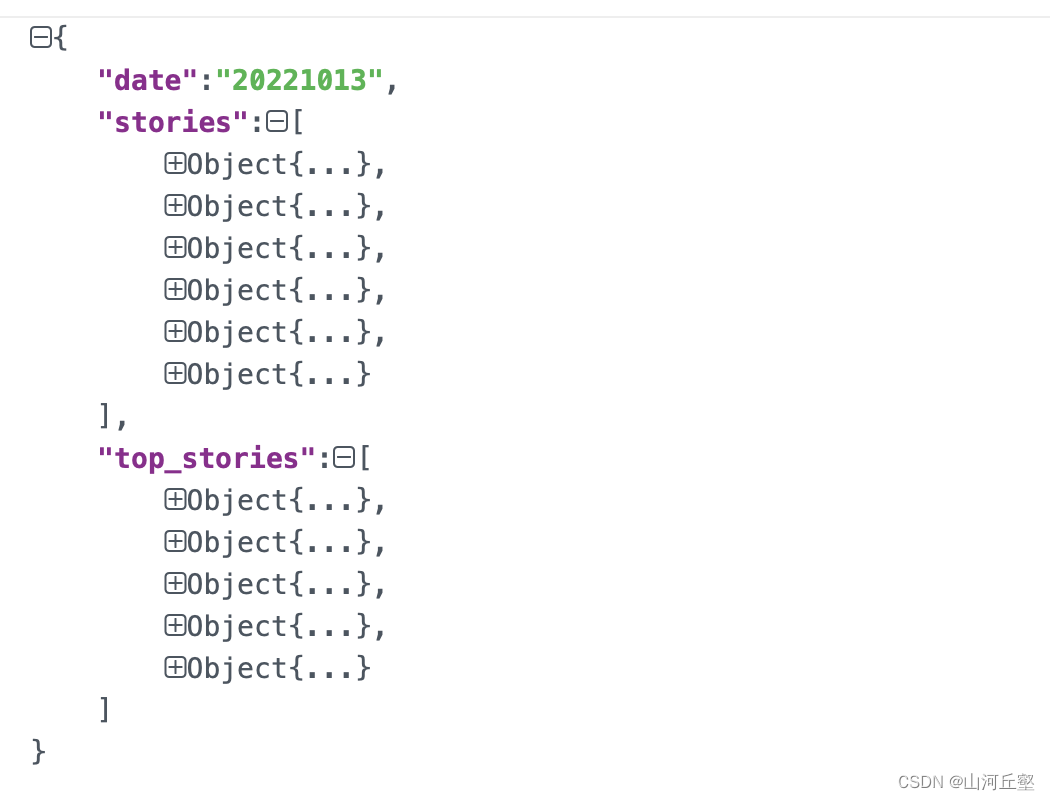
可以看到要解析的数据有一个data字符串,两个数组。
@protocol storiesModel
@end
@protocol top_StoriesModel
@end
#import "JSONModel.h"
NS_ASSUME_NONNULL_BEGIN
@interface storiesModel : JSONModel
@property (nonatomic, copy) NSString* image_hue;
@property (nonatomic, copy) NSString* title;
@property (nonatomic, copy) NSString* url;
@property (nonatomic, copy) NSString* hint;
@property (nonatomic, copy) NSString* ga_prefix;
@property (nonatomic, copy) NSArray* images;
@end
@interface top_StoriesModel : JSONModel
@property (nonatomic, copy) NSString* image_hue;
@property (nonatomic, copy) NSString* title;
@property (nonatomic, copy) NSString* url;
@property (nonatomic, copy) NSString* hint;
@property (nonatomic, copy) NSString* ga_prefix;
@property (nonatomic, copy) NSString* image;
@property (nonatomic, copy) NSString* type;
@end
@interface Model : JSONModel
@property (nonatomic, copy) NSString* date;
@property (nonatomic, copy) NSArray<storiesModel>* stories;
@property (nonatomic, copy) NSArray<top_StoriesModel>* top_stories;
@end
NS_ASSUME_NONNULL_END
#import "Model.h"
@implementation storiesModel
+ (BOOL)propertyIsOptional:(NSString *)propertyName {
return YES;
}
@end
@implementation top_StoriesModel
+ (BOOL)propertyIsOptional:(NSString *)propertyName {
return YES;
}
@end
@implementation Model
+ (BOOL)propertyIsOptional:(NSString *)propertyName {
return YES;
}
@end
#import <UIKit/UIKit.h>
#import "Model.h"
@interface ViewController : UIViewController
@property (nonatomic, strong) top_StoriesModel* Model;
@end
#import "ViewController.h"
#import "Model.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
NSString *json = @"https://news-at.zhihu.com/api/4/news/latest";
json = [json stringByAddingPercentEncodingWithAllowedCharacters:[NSCharacterSet URLQueryAllowedCharacterSet]];
NSURL *testUrl = [NSURL URLWithString:json];
NSURLRequest *testRequest = [NSURLRequest requestWithURL:testUrl];
NSURLSession *testSession = [NSURLSession sharedSession];
NSURLSessionDataTask *testDataTask = [testSession dataTaskWithRequest:testRequest completionHandler:^(NSData * _Nullable data, NSURLResponse * _Nullable response, NSError * _Nullable error) {
Model* JsonModelX = [[Model alloc] initWithData:data error:nil];
NSLog(@"%@", JsonModelX.date);
}];
[testDataTask resume];
}
@end

#import <Foundation/Foundation.h>
#import "Model.h"
NS_ASSUME_NONNULL_BEGIN
typedef void (^TestSucceedBlock) (Model* ViewModel);
typedef void (^ErrorBlock) (NSError* error);
@interface Manager : NSObject
+ (instancetype) shareManger;
- (void) makeData:(TestSucceedBlock) succeedBlock error:(ErrorBlock) errorBlock;
@property (nonatomic, strong) top_StoriesModel* Model;
@end
NS_ASSUME_NONNULL_END
#import "Manager.h"
static Manager* manager = nil;
@implementation Manager
+ (instancetype) shareManger {
if (!manager) {
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
manager = [[Manager alloc] init];
});
}
return manager;
}
- (void)makeData:(TestSucceedBlock)succeedBlock error:(ErrorBlock)errorBlock {
NSString *json = @"https://news-at.zhihu.com/api/4/news/latest";
json = [json stringByAddingPercentEncodingWithAllowedCharacters:[NSCharacterSet URLQueryAllowedCharacterSet]];
NSURL *testUrl = [NSURL URLWithString:json];
NSURLRequest *testRequest = [NSURLRequest requestWithURL:testUrl];
NSURLSession *testSession = [NSURLSession sharedSession];
NSURLSessionDataTask *testDataTask = [testSession dataTaskWithRequest:testRequest completionHandler:^(NSData * _Nullable data, NSURLResponse * _Nullable response, NSError * _Nullable error) {
if (error == nil) {
Model* JsonModelX = [[Model alloc] initWithData:data error:nil];
succeedBlock(JsonModelX);
} else {
errorBlock(error);
}
}];
[testDataTask resume];
}
@end
#import <UIKit/UIKit.h>
#import "Model.h"
@interface ViewController : UIViewController
@property (nonatomic, strong) top_StoriesModel* Model;
@end
#import "ViewController.h"
#import "Model.h"
#import "Manager.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
[[Manager shareManger]makeData:^(Model * _Nonnull ViewModel) {
NSLog(@"%@", ViewModel.stories[1]);
} error:^(NSError * _Nonnull error) {
NSLog(@"请求失败!");
}];
}
@end
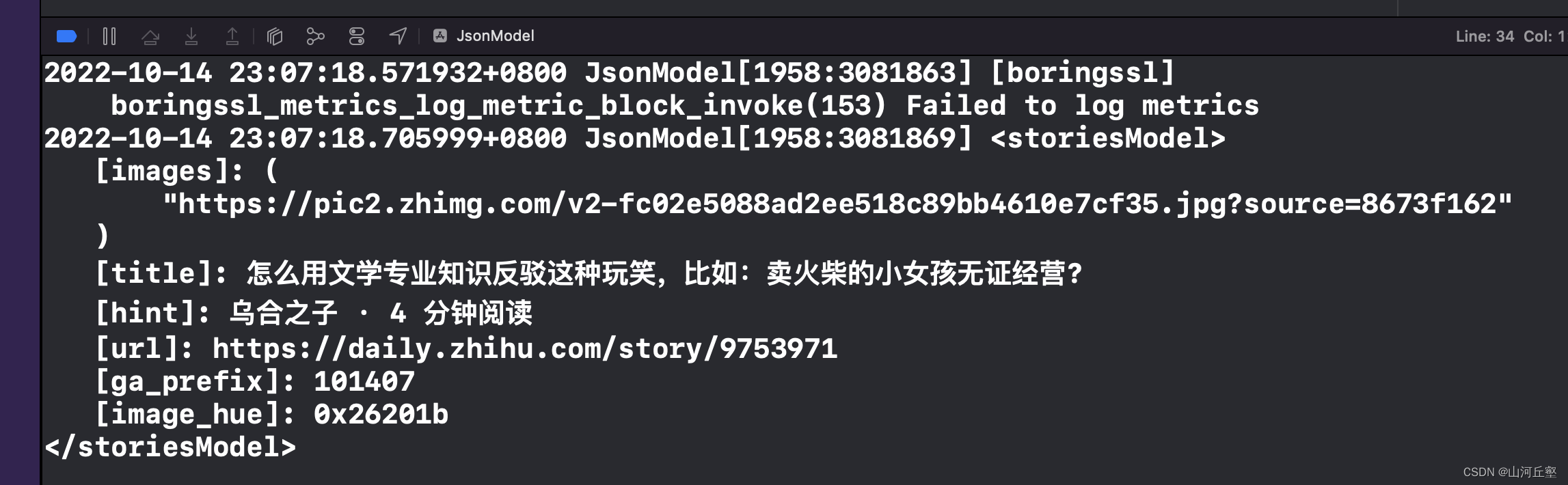
|