- 从腾讯云平台中申请短信调用服务,具体步骤见下方???????
- 创建短信签名,并填写相关申请信息,签名用途如果是个人的话,就选择自用,签名类型选择网站(需备案)或者已发布上线的小程序、公众号、APP,这里我选择的是小程序,签名内容就是小程序的名称,证明材料上传微信小程序后台管理的设置页面截图,剩下的就是上传自己和经办人的身份证照片信息,最后的申请说明填写的是微信小程序的全称,然后点击“确定”,会进行审核,一般半小时就会审核完成。
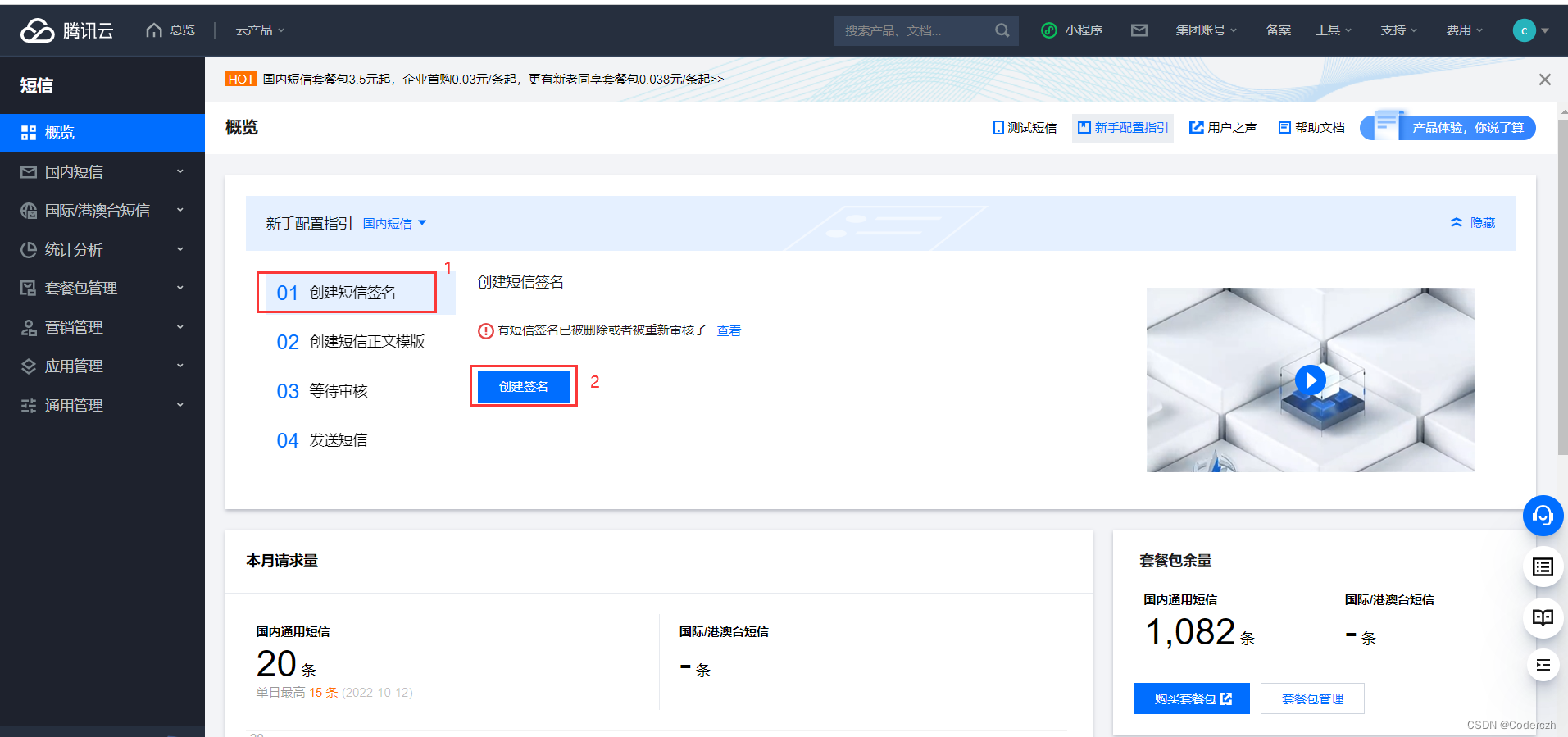 ???????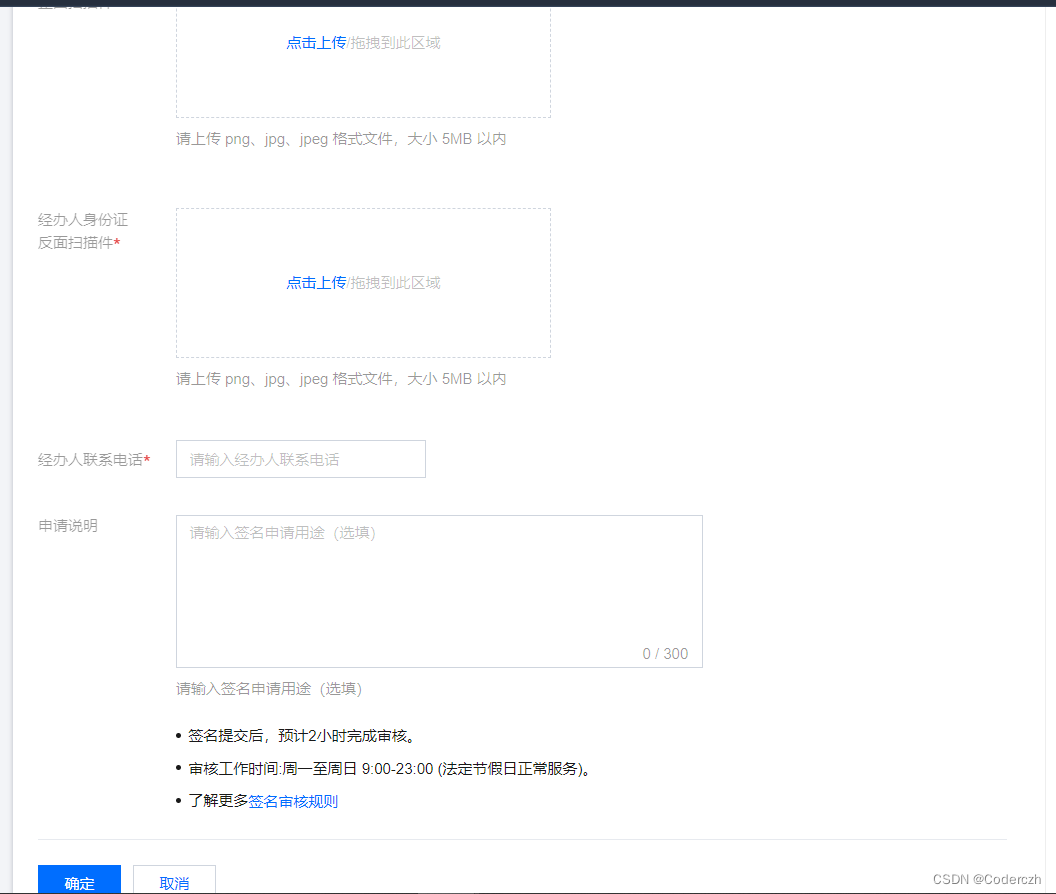
-
短信签名审核完成之后,就是短信正文模板的配置,点击“创建正文模板”,进行短信正文模板的配置,模板名称就是自己起的模板名称,短信类型因为是自用,所有只能选择普通短信,短信内容可以选择系统提供的模板,也可以自己自定义,其中的{1}表示占位符,可以动态添加数据,申请说明填写的是申请短信调用的理由,配置好之后,点击“确定”,会进行审核,一般半小时就会审核完成。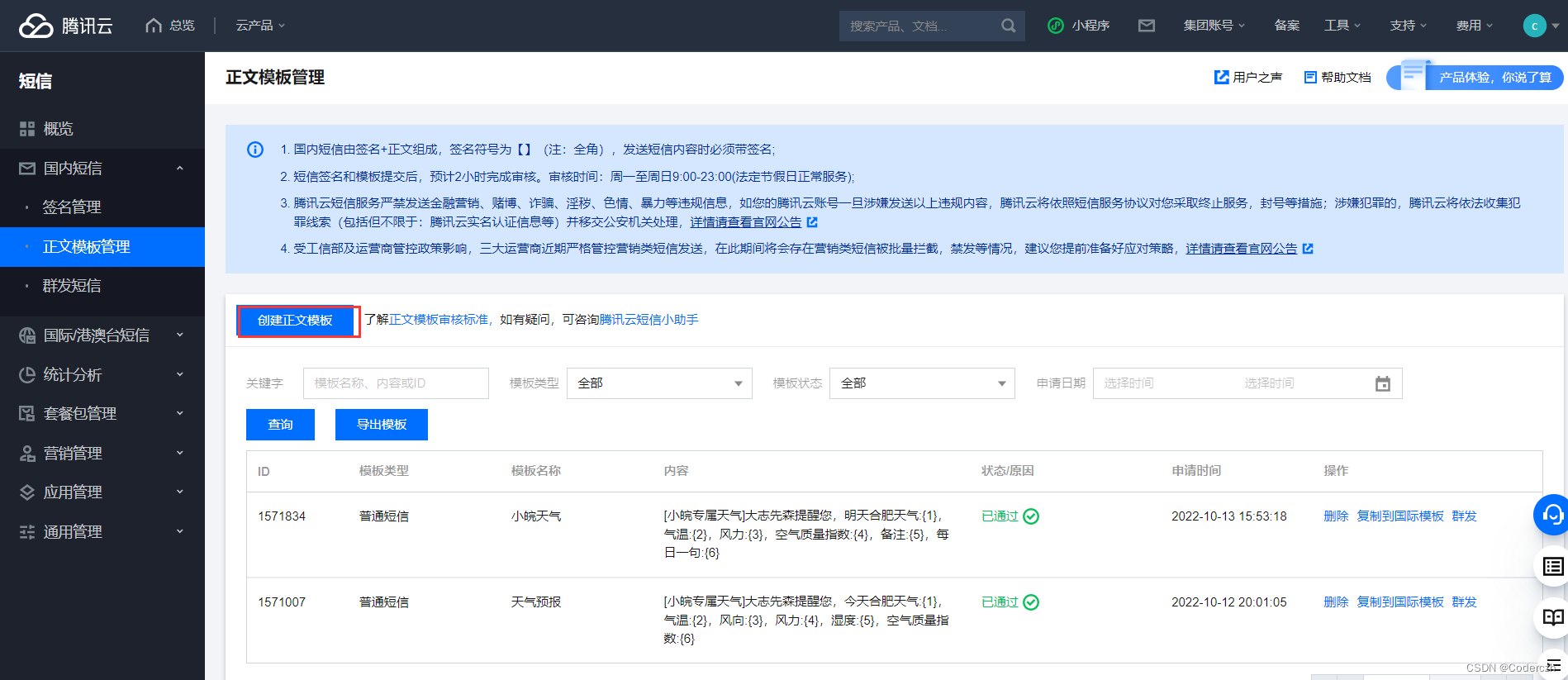 ?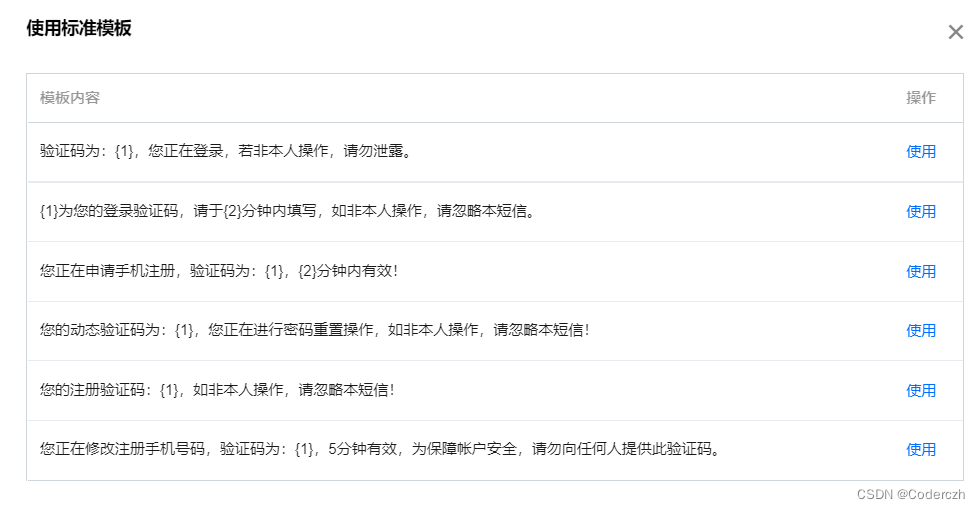 -
?到此为止,申请短信调用就完成了,接下来进行的是天气预报接口的API调用申请
2.?聚合数据天气预报API调用申请
- 在首页搜索天气预报,进行天气预报API的调用申请,每天有50次的免费调用额度
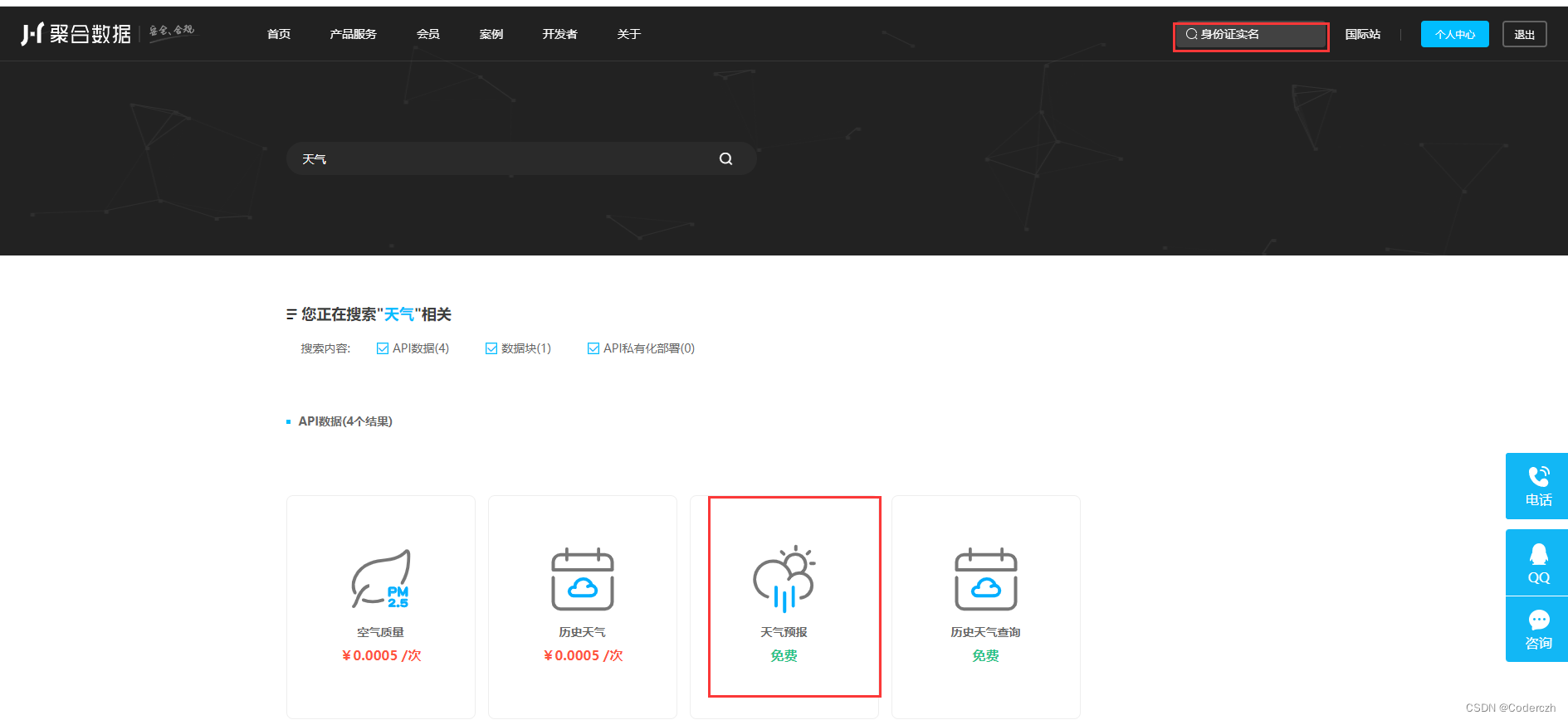 - 在个人中心->我的API中,可以看到当前申请的接口,其中请求Key每个人唯一,后面调用时需要使用
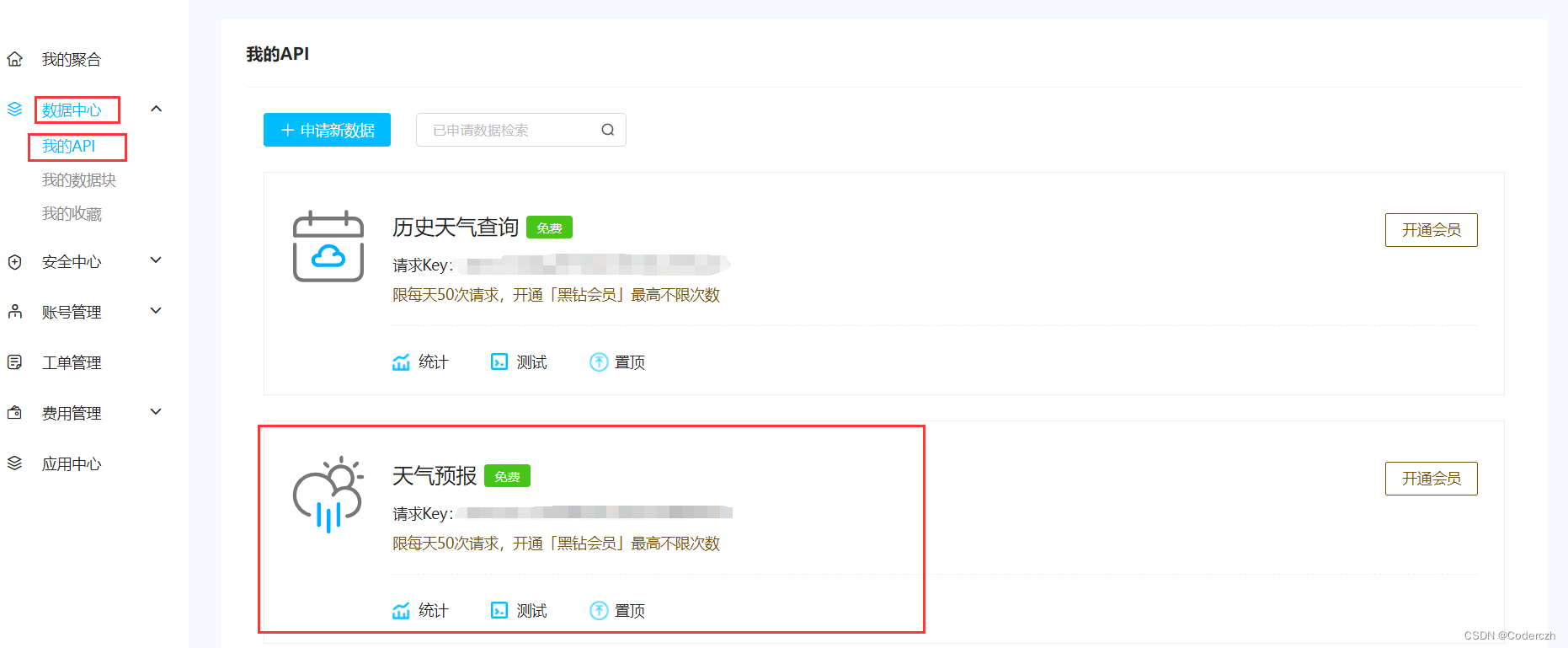 - 到此为止,天气接口的申请已完成
3.?后端代码开发
- 新建Spring Boot项目,目录接口如图所示
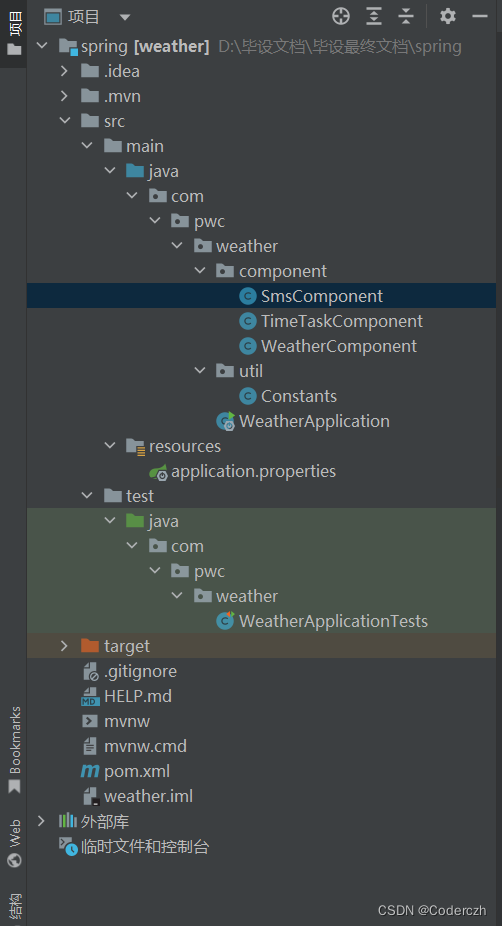 - maven中引入fastjson和tencentcloud-sdk的依赖包
<dependency>
<groupId>com.alibaba.fastjson2</groupId>
<artifactId>fastjson2</artifactId>
<version>2.0.15</version>
</dependency>
<dependency>
<groupId>com.tencentcloudapi</groupId>
<artifactId>tencentcloud-sdk-java</artifactId>
<version>3.1.606</version>
</dependency> - ?编写一个常量类Constants,编写一个常量类Constants,appid和sdkAppid一样,见第二张图片,secretId和secretKey见第三张图片,sign见第四张图片,短信模板id见第五张图片
package com.pwc.weather.util;
/**
* 常量类
* @author chenzh
*/
public class Constants {
/**
* 短信 appid
*/
public static final String SMSAPPID="*****";
/**
* 腾讯云账户密钥对: secretId
*/
public static final String SMSSECREID="*****";
/**
* 腾讯云账户密钥对: secretKey
*/
public static final String SMSSECREKEY="*****";
/**
* SmsSdkAppid
*/
public static final String SMSSDKAPPID="*****";
/**
* sms sign : sms签名
*/
public static final String SMSSIGN="*****";
/**
* sms 短信模板id:
*/
public static final String SMSTEMPLATEID="*****";
}
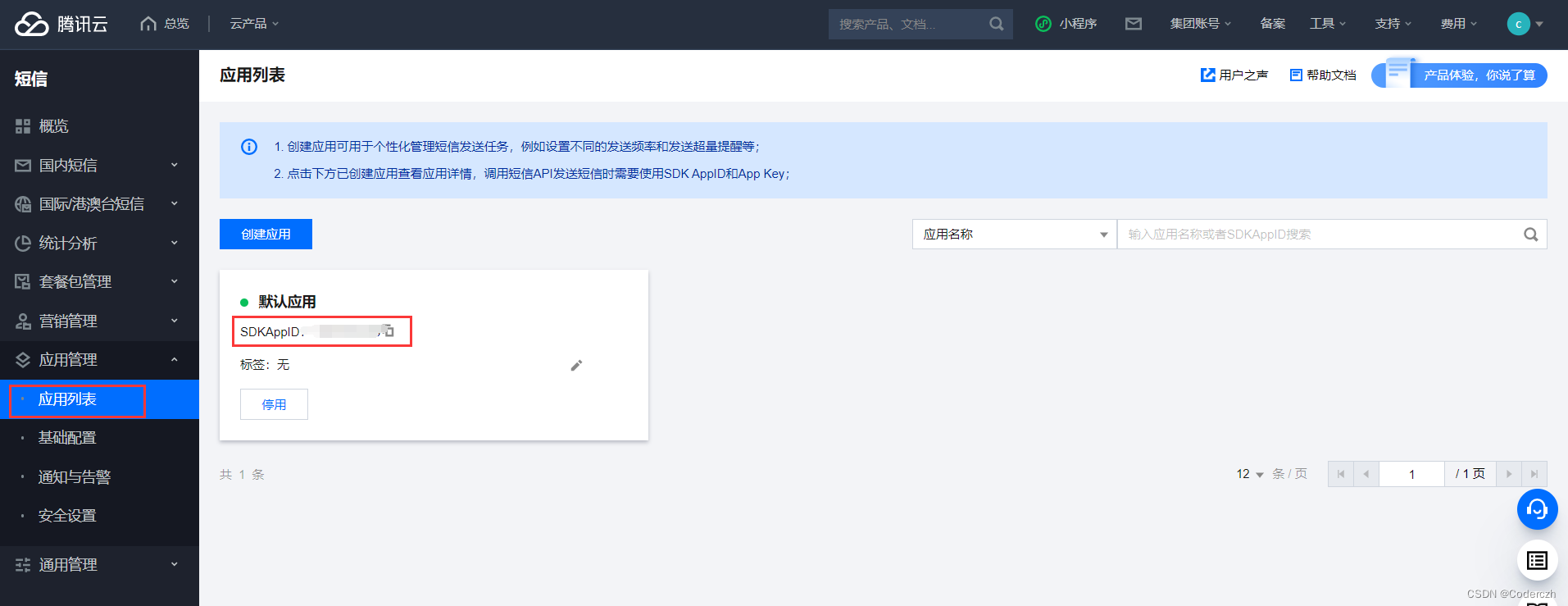 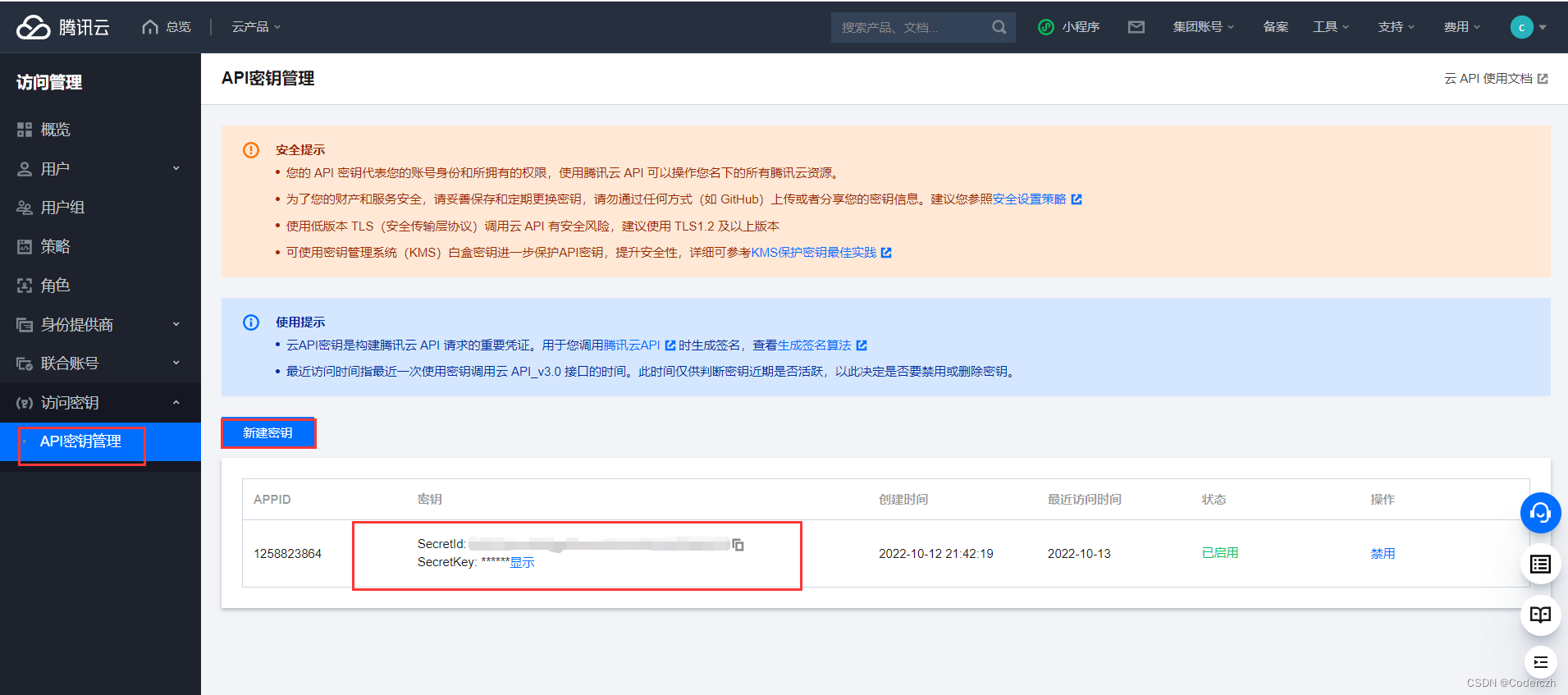 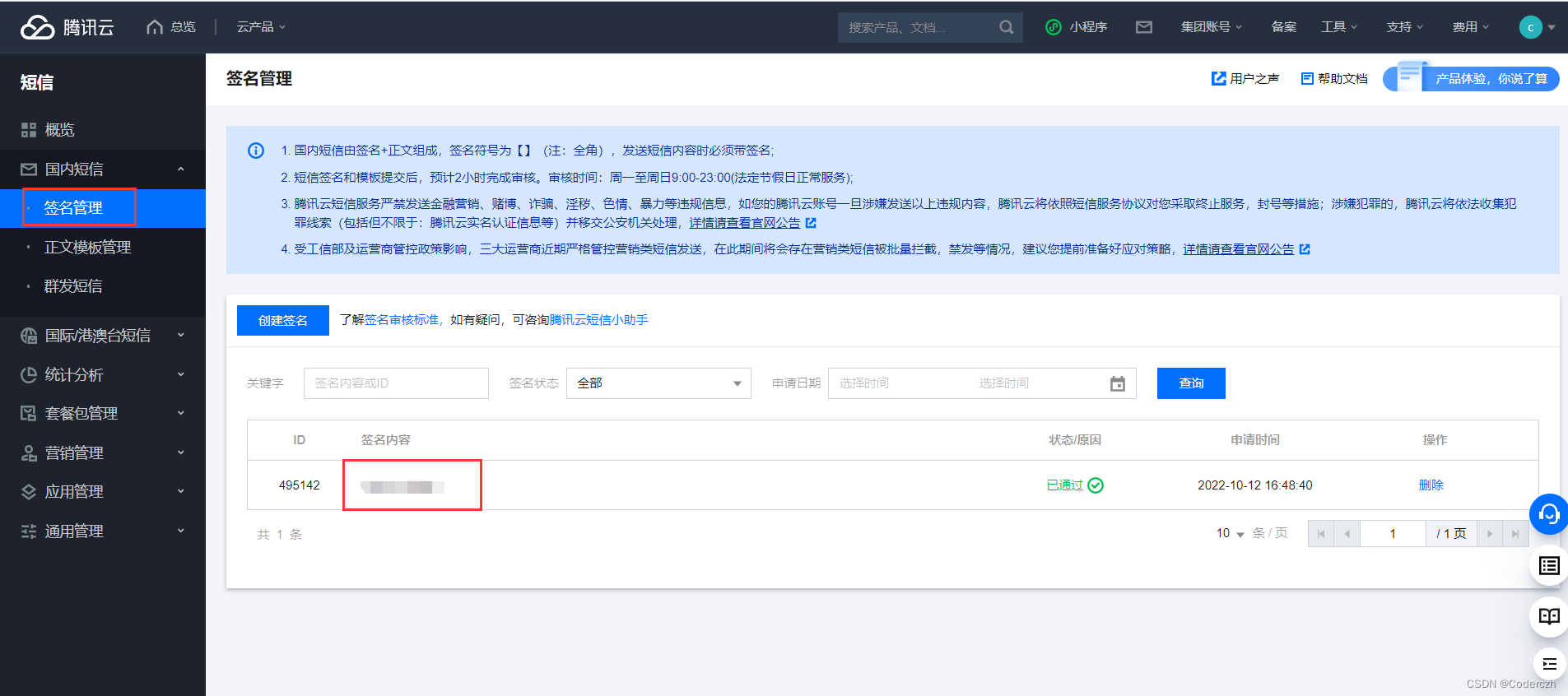 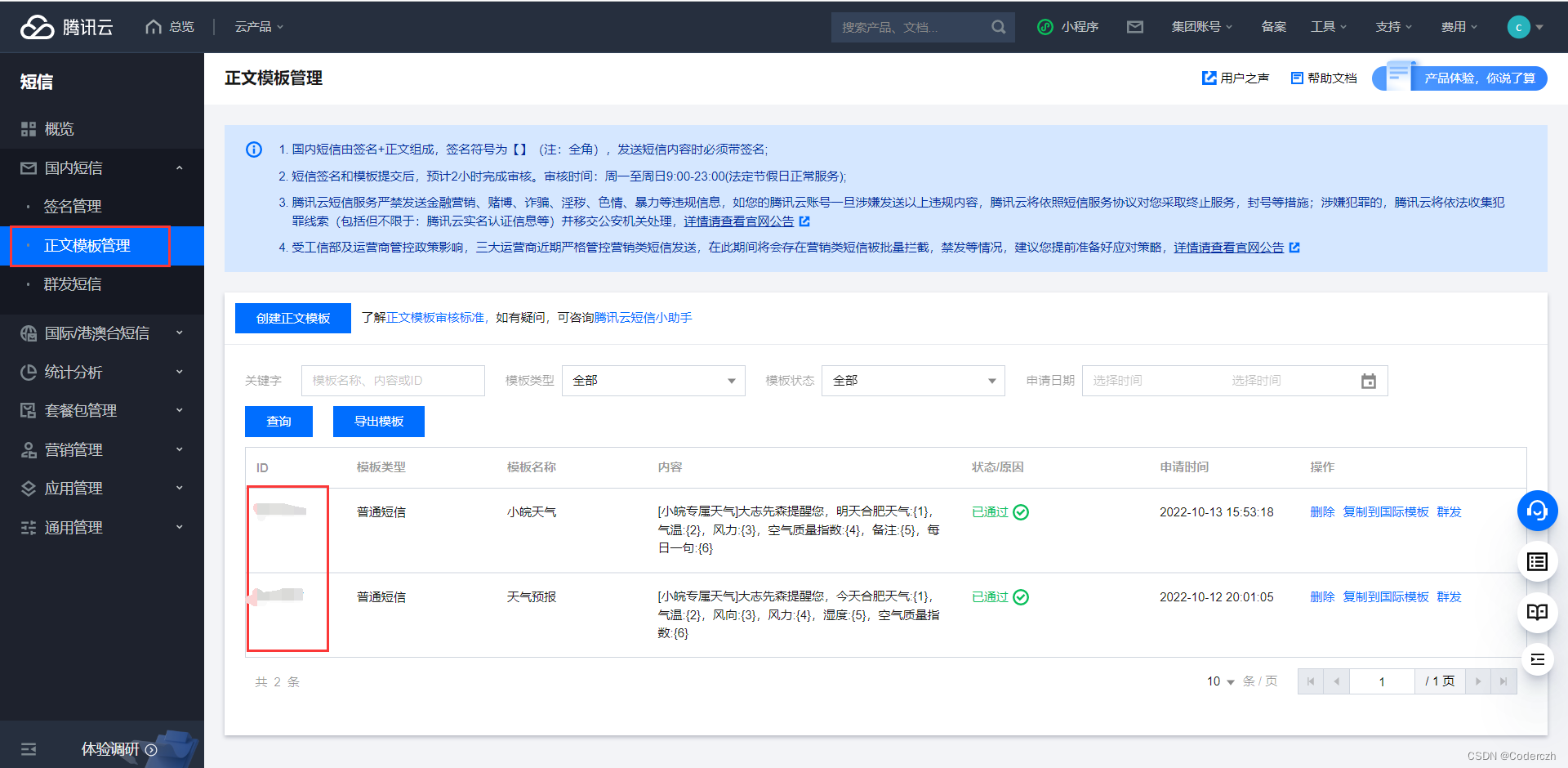 -
SmsComponent.java类,该类主要是进行短信的发送 package com.pwc.weather.component;
import com.pwc.weather.util.Constants;
import com.tencentcloudapi.common.Credential;
import com.tencentcloudapi.common.exception.TencentCloudSDKException;
import com.tencentcloudapi.common.profile.ClientProfile;
import com.tencentcloudapi.common.profile.HttpProfile;
import com.tencentcloudapi.sms.v20190711.SmsClient;
import com.tencentcloudapi.sms.v20190711.models.SendSmsRequest;
import com.tencentcloudapi.sms.v20190711.models.SendSmsResponse;
import org.springframework.stereotype.Component;
/**
* @author chenzh
*/
@Component
public class SmsComponent {
public String sendSms(String param1, String param2, String param3, String param4, String param5, String param6,
String[] phoneNumbers) {
try {
Credential cred = new Credential(Constants.SMSSECREID, Constants.SMSSECREKEY);
HttpProfile httpProfile = new HttpProfile();
httpProfile.setReqMethod("POST");
httpProfile.setConnTimeout(60);
httpProfile.setEndpoint("sms.tencentcloudapi.com");
ClientProfile clientProfile = new ClientProfile();
clientProfile.setSignMethod("HmacSHA256");
clientProfile.setHttpProfile(httpProfile);
SmsClient client = new SmsClient(cred, "ap-guangzhou", clientProfile);
SendSmsRequest req = new SendSmsRequest();
String appid = Constants.SMSAPPID;
req.setSmsSdkAppid(appid);
String sign = Constants.SMSSIGN;
req.setSign(sign);
String templateID = Constants.SMSTEMPLATEID;
req.setTemplateID(templateID);
req.setPhoneNumberSet(phoneNumbers);
String[] templateParams = {param1, param2, param3, param4, param5, param6};
req.setTemplateParamSet(templateParams);
SendSmsResponse res = client.SendSms(req);
System.out.println(SendSmsResponse.toJsonString(res));
} catch (TencentCloudSDKException e) {
e.printStackTrace();
}
return null;
}
}
-
WeatherComponent.java类,该类主要是调用天气接口,其中API_KEY属性的值见步骤2.2 package com.pwc.weather.component;
import com.alibaba.fastjson2.JSONArray;
import com.alibaba.fastjson2.JSONObject;
import org.springframework.stereotype.Component;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
/**
* @author chenzh
*/
@Component
public class WeatherComponent {
private static final String API_URL = "http://apis.juhe.cn/simpleWeather/query";
private static final String API_KEY = "******************";
private static final int RESULT_CODE = 200;
/**
* 根据城市名查询天气情况
*
* @param cityName 城市名称
*/
public Map<String, String> queryWeather(String cityName) {
Map<String, Object> params = new HashMap<>(4);
params.put("city", cityName);
params.put("key", API_KEY);
String queryParams = urlEncode(params);
String response = doGet(API_URL, queryParams);
Map<String, String> weatherMap = new HashMap<>(16);
try {
JSONObject jsonObject = JSONObject.parseObject(response);
int errorCode = jsonObject.getInteger("error_code");
if (errorCode == 0) {
System.out.println("调用接口成功");
JSONObject result = jsonObject.getJSONObject("result");
JSONArray future = result.getJSONArray("future");
weatherMap.put("weather", future.getJSONObject(1).getString("weather"));
weatherMap.put("temperature", future.getJSONObject(1).getString("temperature"));
weatherMap.put("direct", future.getJSONObject(1).getString("direct"));
weatherMap.put("wid", future.getJSONObject(1).getString("wid"));
} else {
System.out.println("调用接口失败:" + jsonObject.getString("reason"));
}
} catch (Exception e) {
e.printStackTrace();
}
return weatherMap;
}
/**
* get方式的http请求
*
* @param httpUrl 请求地址
* @return 返回结果
*/
public String doGet(String httpUrl, String queryParams) {
HttpURLConnection connection = null;
InputStream inputStream = null;
BufferedReader bufferedReader = null;
String result = null;
try {
// 创建远程url连接对象
URL url = new URL(httpUrl + "?" + queryParams);
// 通过远程url连接对象打开一个连接,强转成httpURLConnection类
connection = (HttpURLConnection) url.openConnection();
// 设置连接方式:get
connection.setRequestMethod("GET");
// 设置连接主机服务器的超时时间:15000毫秒
connection.setConnectTimeout(5000);
// 设置读取远程返回的数据时间:60000毫秒
connection.setReadTimeout(6000);
// 发送请求
connection.connect();
// 通过connection连接,获取输入流
if (connection.getResponseCode() == RESULT_CODE) {
inputStream = connection.getInputStream();
// 封装输入流,并指定字符集
bufferedReader = new BufferedReader(new InputStreamReader(inputStream, StandardCharsets.UTF_8));
// 存放数据
StringBuilder sbf = new StringBuilder();
String temp;
while ((temp = bufferedReader.readLine()) != null) {
sbf.append(temp);
sbf.append(System.getProperty("line.separator"));
}
result = sbf.toString();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// 关闭资源
if (null != bufferedReader) {
try {
bufferedReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (null != inputStream) {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (connection != null) {
// 关闭远程连接
connection.disconnect();
}
}
return result;
}
/**
* 将map型转为请求参数型
*
* @param data 请求数据
* @return Map数据
*/
public String urlEncode(Map<String, ?> data) {
StringBuilder sb = new StringBuilder();
for (Map.Entry<String, ?> i : data.entrySet()) {
sb.append(i.getKey()).append("=").append(URLEncoder.encode(i.getValue() + "", StandardCharsets.UTF_8)).append("&");
}
String result = sb.toString();
result = result.substring(0, result.lastIndexOf("&"));
return result;
}
}
-
TimeTaskComponent.java类,定时任务执行,到某一时间点定时推送 package com.pwc.weather.component;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
import java.util.Map;
/**
* @author chenzh
*/
@Component
public class TimeTaskComponent {
@Autowired
private WeatherComponent weatherComponent;
@Autowired
private SmsComponent smsComponent;
@Scheduled(cron = "0 50 06 * * ?")
public void doTask(){
Map<String, String> weatherInfo = weatherComponent.queryWeather("合肥");
String remark = "";
String wid = weatherInfo.get("wid").substring(8, 10);
weatherInfo.put("wid", wid);
String judgeWid = judgeWid(wid);
String judgeWeather = judgeWeather(weatherInfo.get("weather"));
String judgeTemperature = judgeTemperature(weatherInfo.get("temperature"));
if ("".equals(judgeWid) && "".equals(judgeWeather) && "".equals(judgeTemperature)) {
remark = "明日天气不错!";
} else {
remark = judgeWeather + judgeTemperature + judgeWid;
}
String weather = weatherInfo.get("weather");
String temperature = weatherInfo.get("temperature");
String language = "你是我的克莱因蓝";
String[] phoneNum = {"***********", "**********"};
smsComponent.sendSms(weather, temperature, wid + "级", "4", remark, language, phoneNum);
}
/**
* 判断风力
*
* @param wid 风力
* @return 提示语
*/
private String judgeWid(String wid) {
String remindWid = "";
int widLevel = Integer.parseInt(wid);
if (widLevel > 5) {
remindWid = "明日风比较大,注意安全哦!";
}
return remindWid;
}
private String judgeWeather(String weather) {
String remindWeather = "";
if (weather.contains("雨")) {
remindWeather = "明日有雨,记得带伞哦!";
}
return remindWeather;
}
private String judgeTemperature(String temperature) {
String remindTemperature = "";
int start = temperature.indexOf("/");
int end = temperature.indexOf("℃");
String temperatureHigh = temperature.substring(start + 1, end);
int high = Integer.parseInt(temperatureHigh);
if (high > 28) {
remindTemperature = "明日温度挺高,注意防晒哦!";
}
return remindTemperature;
}
}
4. 项目部署
- 使用maven达成jar包
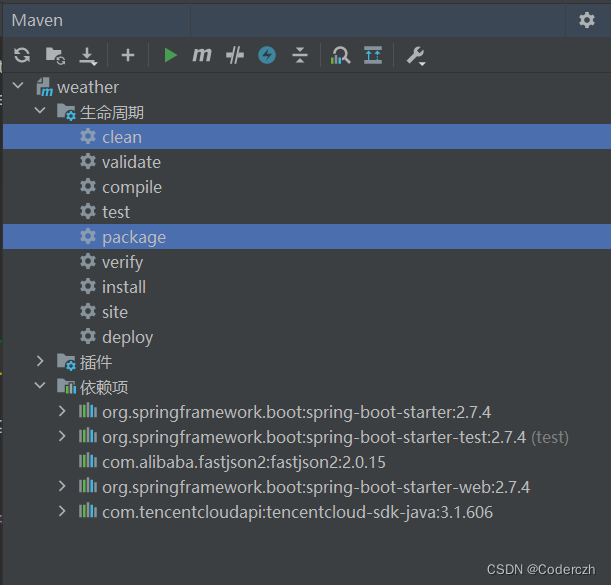 - ?将jar包推送到公网服务器中,此处我使用的是阿里云的云服务器,如果没有安装jdk,则需要先安装linux版本的jdk
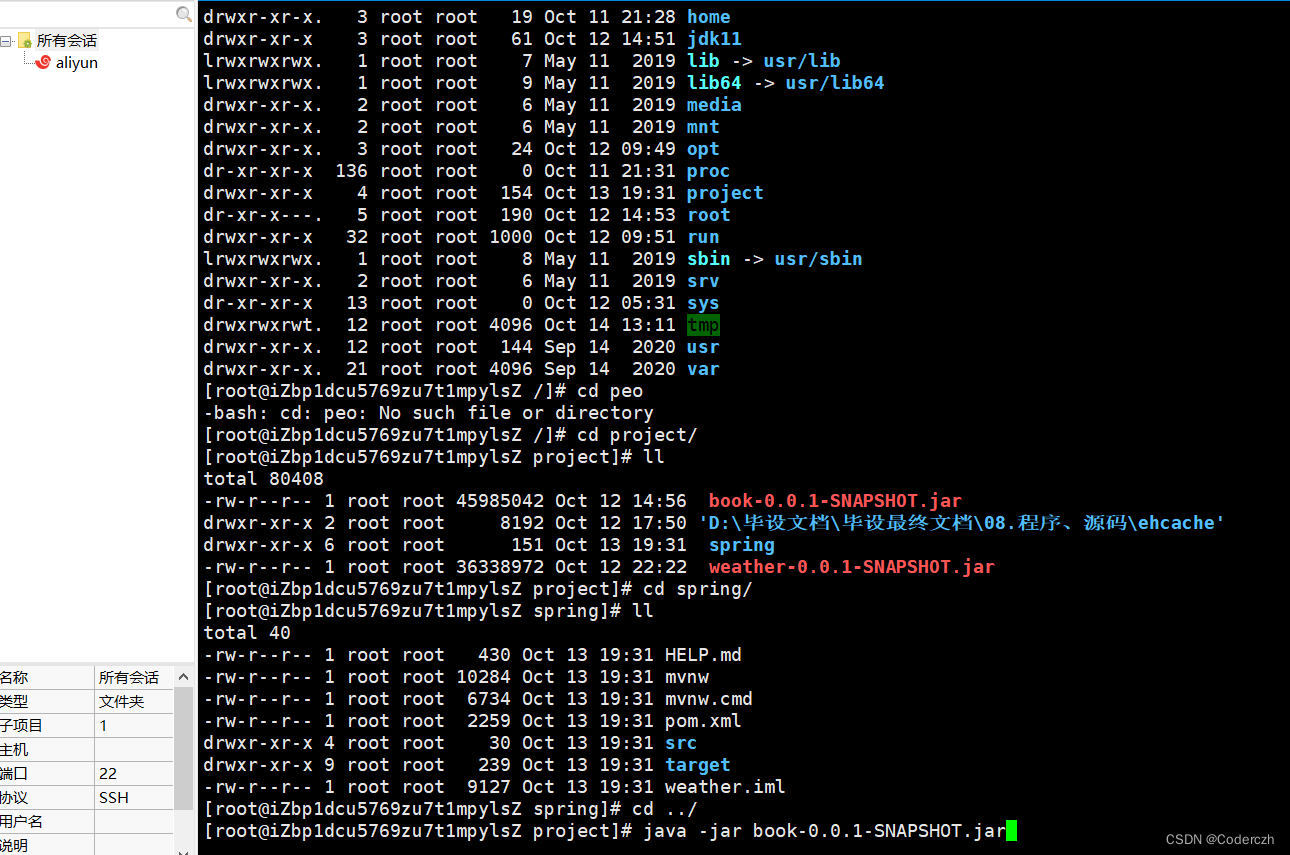 - ?运行结果如图所示
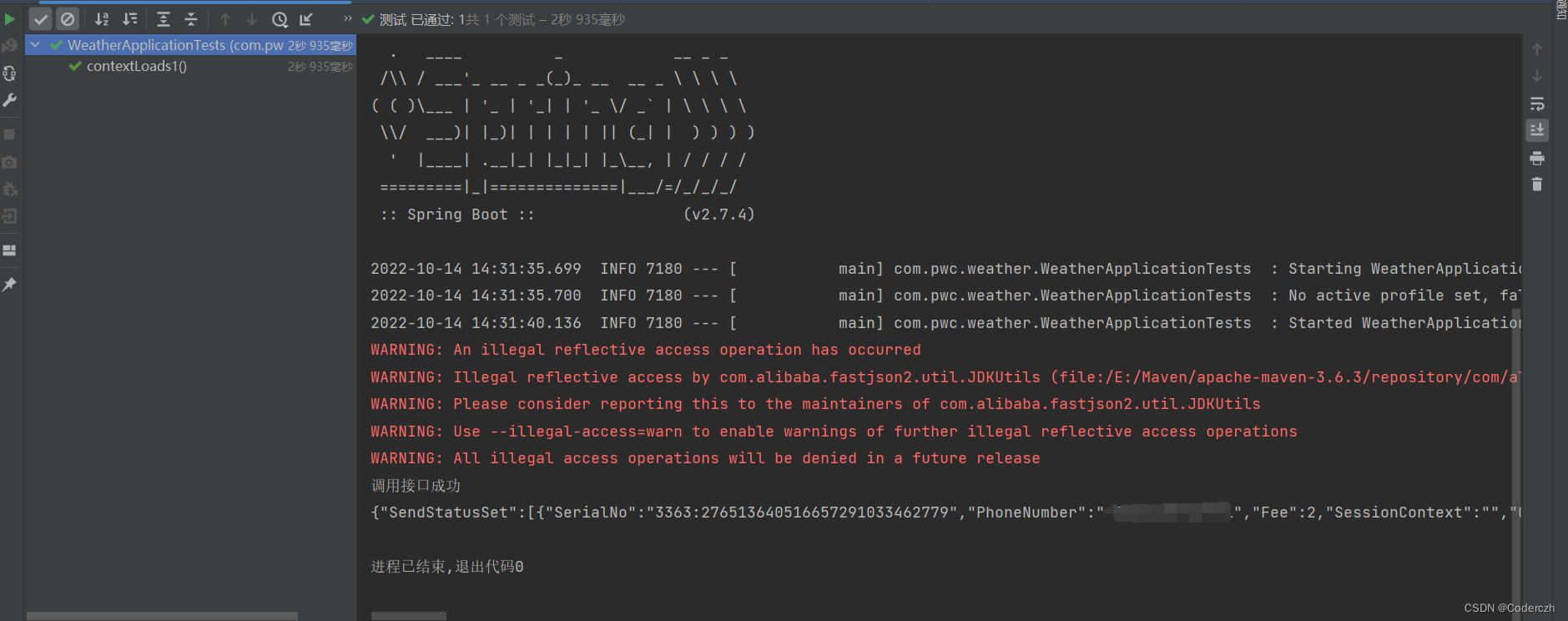
5.? 结果展示
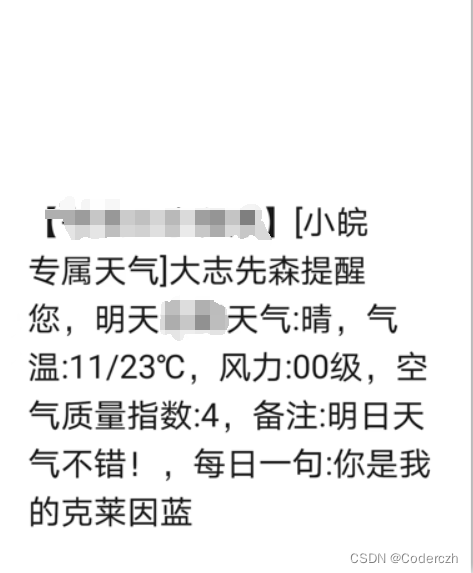
????????
|