Mediator Mode 中介者模式
提起中介,我们常想到,中介是使用自己手上的人脉资源,构建起被介绍人之间一对一的协作的;同时他手上的许多人脉之间通常是不能直接沟通的,想要沟通,是找中介来帮忙,把自己需求告诉中介,让中介找合适的人来做事情。同样的,设计模式中的中介者模式有类似的性质。
对于中介者模式,有以下的描述:
The mediator constrains the interaction between the component, the components can only notify the mediator and the mediator can manage the action of all the components it contains.
中介者限制了组件之间的交互,组件只能通知中介一些指令,然后中介者管理它内部拥有的所有组件的数据操作与行为。
中介者持有多个发送者的引用(预先注册好),如下图的 a,b,c,发送者(在需要的时候)只调用相应的通知方法来通知中介者,中介者协调各方关系从而执行动作。 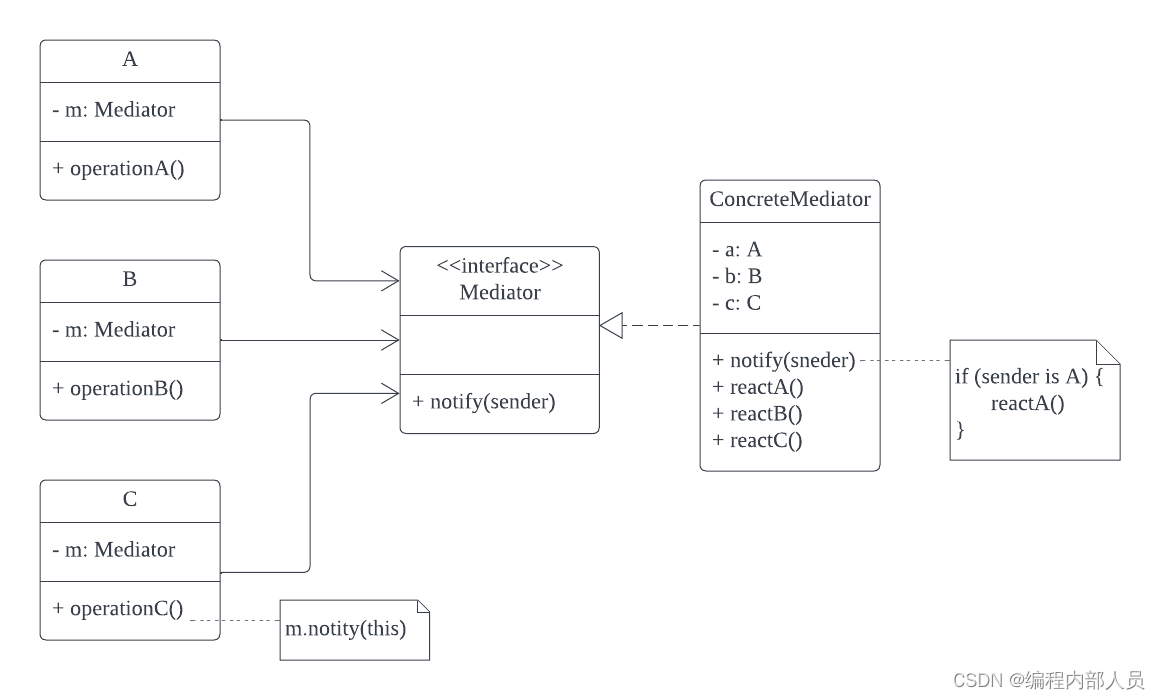
class Component {
var m: Mediator
init(mediator: Mediator) {
self.m = mediator
m.notify(component: self)
}
}
class Button: Component {
func click() {
m.notify(component: self)
}
}
class CheckBox: Component {
var check = false
func isCheck() -> Bool {
return check
}
}
class PasswordText: Component {
var text: String = ""
func getText() -> String{
return text
}
}
class UserNameText: Component {
var text: String = ""
func getText() -> String{
return text
}
}
protocol Mediator {
func notify(component: Component)
}
class LoginMediator: Mediator {
var btnLogin: Button?
var tickSavePassword: CheckBox?
var password: PasswordText?
var userName: UserNameText?
func notify(component: Component) {
if component is Button {
var btn = component as! Button
if self.btnLogin == nil {
self.btnLogin = btn
return
}
if (canLogin(userName: userName?.getText(), password: password?.getText())) {
print("Login success")
if let wantSave = tickSavePassword?.isCheck() {
}
} else {
print("Login failed")
}
} else if component is CheckBox {
var box = component as! CheckBox
self.tickSavePassword = box
} else if component is UserNameText {
var userName = component as! UserNameText
self.userName = userName
} else if component is PasswordText {
var password = component as! PasswordText
self.password = password
}
}
}
func canLogin(userName: String?, password: String?) -> Bool {
if userName == nil || password == nil {
return false
}
if let name = userName, let word = password{
if name == "hello" && word == "Mike" {
return true
} else {
return false
}
}
return false
}
let mediator = LoginMediator()
let userName = UserNameText(mediator: mediator)
let password = PasswordText(mediator: mediator)
let tickSavePassword = CheckBox(mediator: mediator)
let btnLogin = Button(mediator: mediator)
userName.text = "hello"
password.text = "Mike"
tickSavePassword.check = true
btnLogin.click()
以上代码中,多个组件都可能向中介者发送通知,中介者会判断通知的来源,并且做出相应的动作。登录按钮所需要的数据分散在各个组件中,为了集中这些消息,又要不让组件之间互相沟通(这会使得组件之间产生耦合),所以使用中介者,统一收集信息,然后登陆按钮才会发挥作用。
命令模式是一对一的命令,中介者模式是中介者对多个发送者负责并协调各方关系。
中介者的作用:收集各个组件的信息,同时不让组件之间耦合。耦合的点,集中在中介者上。
|