一、定义变量
#include <STC15F2K60S2.H>
#include <intrins.h>
#define uint unsigned int
#define uchar unsigned char
#define ulong unsigned long
#define cstUart2Ri 0x01
#define cstUart2Ti 0x02
#define cstNoneParity 0
#define PARITYBIT cstNoneParity
#define cstFosc 11059200L
#define cstBaud2 9600
#define cstT2HL (65536-(cstFosc/4/cstBaud2))
sbit sbtKey1 = P3 ^ 2 ;
sbit sbtKey2 = P3 ^ 3 ;
sbit sbtKey3 = P1 ^ 7 ;
sbit sbtSel0 = P2 ^ 0 ;
sbit sbtSel1 = P2 ^ 1 ;
sbit sbtSel2 = P2 ^ 2 ;
sbit sbtLedSel = P2 ^ 3 ;
sbit sbtM485_TRN = P3 ^ 7 ;
bit btSendBusy ;
uchar ucGetDataTmp ;
uchar ucPutDataTmp ;
uchar arrSegSelect[] = {0x3f, 0x06, 0x5b, 0x4f, 0x66,
0x6d, 0x7d, 0x07, 0x7f, 0x6f,
0x77, 0x7c, 0x39, 0x5e, 0x79,
0x71, 0x40, 0x00
};
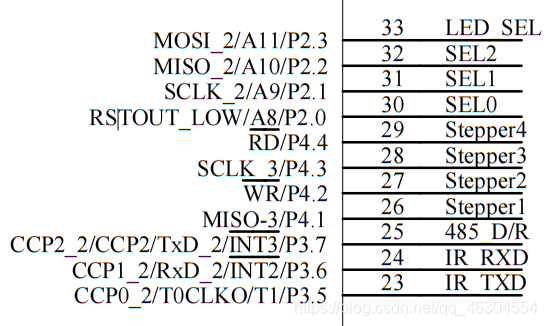 图1(定义中大部分引脚,其余的因为经常用就不展示了) 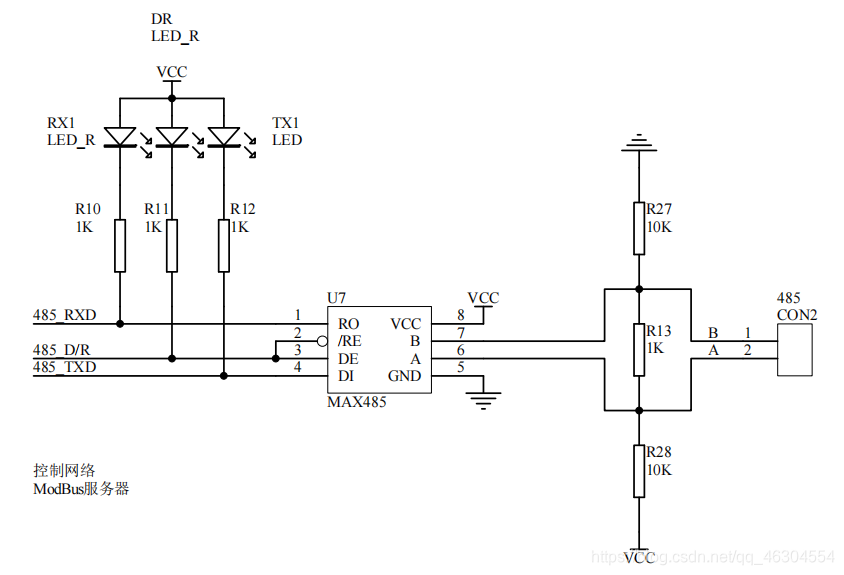 图2(通信的原理图,结合引脚图理解) 从这两张图可以看出sbtM485_TRN 所对应的P3 ^ 7 ;
二、与串口有关的函数
void Uart2Init( void )
{
S2CON = 0x10 ;
T2L = cstT2HL ;
T2H = cstT2HL >> 8 ;
AUXR |= 0x14 ;
}
void Uart2_Process( void ) interrupt 8 using 1
{
if( S2CON & cstUart2Ri )
{
ucGetDataTmp = S2BUF ;
ucPutDataTmp = ucGetDataTmp ;
S2CON &= ~cstUart2Ri;
}
if( S2CON & cstUart2Ti )
{
btSendBusy = 0 ;
S2CON &= ~cstUart2Ti ;
}
}
其中用到的两个主要寄存器(串行口2的数据缓冲寄存器S2BUF、串行口2的控制寄存器S2CON)
S2CON的格式如下:  S2TI——发送中断请求标志位 , S2RI——接收中断请求标志位 S2BUF实际上是2个缓冲器,它可以进行两个操作(完成待发送数据的加载,获得已接收到的数据)。两个操作分别对应两个不同的寄存器,1个是只写寄存器,1个是只读寄存器。
三、外部中断函数
void ExInt0_Process() interrupt 0
{
sbtM485_TRN = 1 ;
S2BUF = ucPutDataTmp ;
while( btSendBusy ) ;
btSendBusy = 1 ;
sbtM485_TRN = 0 ;
}
这里为何MAX485使能引脚,为1时发送,为0时接收,具体可以看看图2,应该就可以理解了。 while( btSendBusy ) ; 这句话就是当btSendBusy=1时就不会进行下面的流程就会一直再循环这句。
四、初始化函数
void Init()
{
P0M0 = 0xff ;
P0M1 = 0x00 ;
P2M0 = 0x0f ;
P2M1 = 0x00 ;
P3M0 = 0x00 ;
P3M1 = 0x00 ;
P1M0 = 0x00 ;
P1M1 = 0x00 ;
IT0 = 1 ;
EX0 = 1 ;
PX0 = 0 ;
sbtM485_TRN = 0 ;
P_SW2 |= 0x01 ;
Uart2Init() ;
btSendBusy = 1 ;
IE2 |= 0x01 ;
IP2 |= 0x01 ;
EA = 1 ;
sbtLedSel = 0 ;
sbtSel0 = 1 ;
sbtSel1 = 1 ;
sbtSel2 = 1 ;
ucPutDataTmp = 0 ;
}
P_SW2寄存器的格式如下:  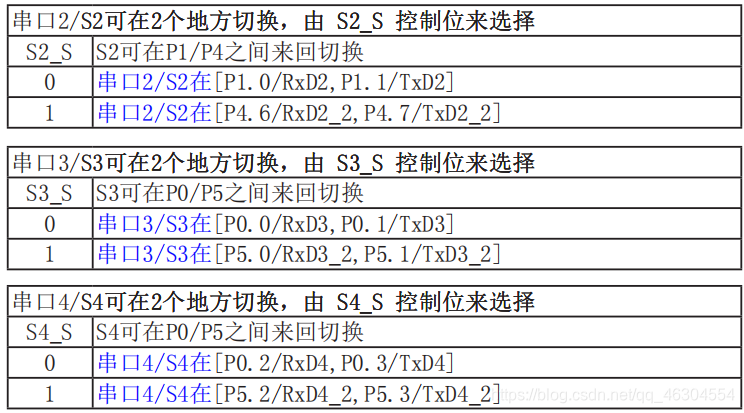
五、其他函数
void delay( void )
{
uchar i, j;
for( i = 0; i < 255; i++ )
for( j = 0; j < 255; j++ )
;
}
六、主函数
void main( void )
{
Init() ;
while( 1 )
{
ucPutDataTmp %= 16 ;
P0 = arrSegSelect[ucPutDataTmp] ;
if( sbtKey3 == 0 )
{
delay();
if( sbtKey3 == 0 )
{
while( !sbtKey3 );
ucPutDataTmp++ ;
}
}
if( sbtKey2 == 0 )
{
delay();
if( sbtKey2 == 0 )
{
while( !sbtKey2 );
ucPutDataTmp-- ;
}
}
}
}
|