单片机系统一个非常实用的按键处理框架MultiButton
前言
在嵌入式开发中,对于输入设备有这几种,屏幕、按键、编码器等。 按键是我们使用最常见的一种。但是处理按键的时候也有一些麻烦之处,比方说我们对于一个按键进行程序消抖、区分按键单击、双击、长按、短按,如果没有一个按键驱动框架是很难实现的 ,在这里推荐一个非常好用的按键处理框架MultiButton 。
一、MultiButton 是什么?
MultiButton 就是这样一个按键管理框架,它不仅占用内存小,而且实现了按键操作的诸多功能(具体功能如下),使用回调函数进行业务的处理,是一个非常实用的按键框架。 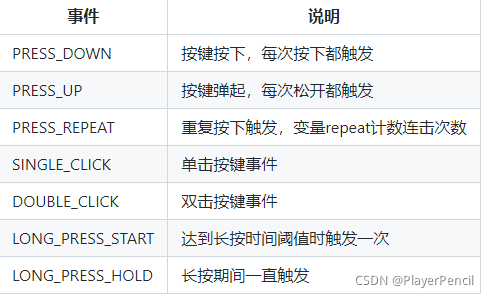 本篇文章对 MultiButton 进行简略的介绍,并且对业务处理代码进行了解读
GitHub仓库 https://github.com/0x1abin/MultiButton
2. 使用步骤和代码的问题
2.1 使用步骤
struct Button key2;
void button_init(struct Button* handle, uint8_t(*pin_level)(), uint8_t active_level)
button_init(&key2,Read_Key2_Pin , 1);
void button_attach(struct Button* handle, PressEvent event, BtnCallback cb)
button_start(&key2);
button_ticks();
2.2 按键库的问题
- 因为处理函数设计的问题,如果只有一个按键结构体,就会导致段错误
void button_ticks()
{
struct Button* target;
for(target=head_handle; target; target=target->next) {
button_handler(target);
}
}
2.3 按键库一些默认参数的配置
#define TICKS_INTERVAL 5
#define DEBOUNCE_TICKS 3
#define SHORT_TICKS (300 /TICKS_INTERVAL)
#define LONG_TICKS (1000 /TICKS_INTERVAL)
3. 代码解读
-
按键的处理流程为下图,按照下图看代码会更加清晰 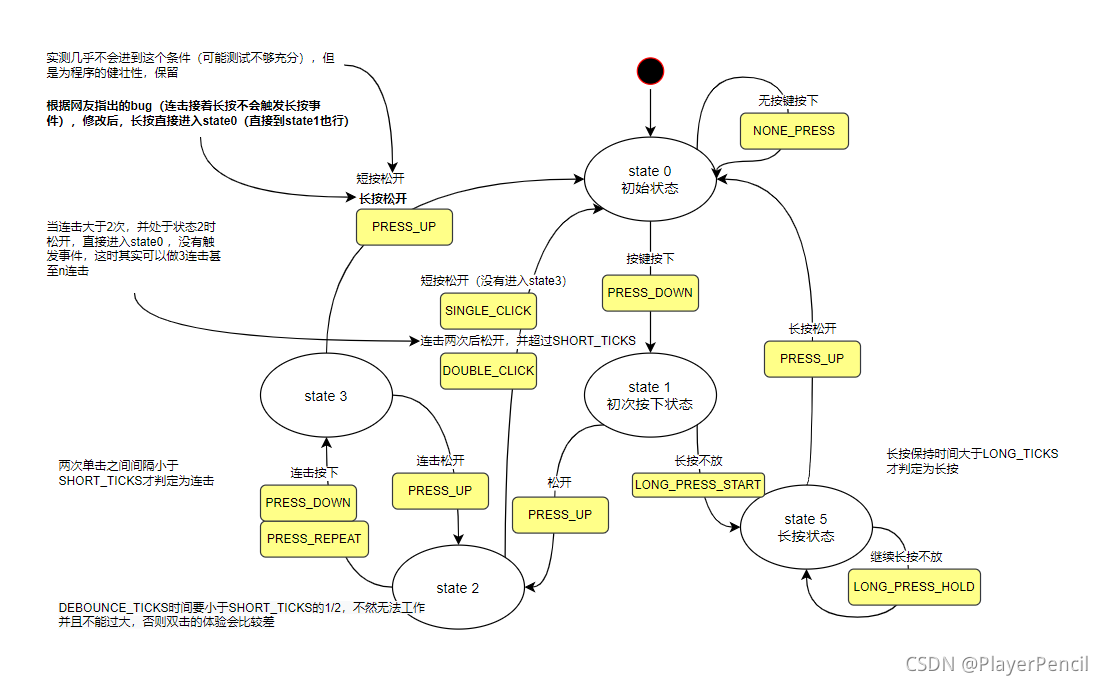 -
C文件
#include "multi_button.h"
#define EVENT_CB(ev) if(handle->cb[ev])handle->cb[ev]((Button*)handle)
static struct Button* head_handle = NULL;
void button_init(struct Button* handle, uint8_t(*pin_level)(), uint8_t active_level)
{
memset(handle, 0, sizeof(struct Button));
handle->event = (uint8_t)NONE_PRESS;
handle->hal_button_Level = pin_level;
handle->button_level = handle->hal_button_Level();
handle->active_level = active_level;
}
void button_attach(struct Button* handle, PressEvent event, BtnCallback cb)
{
handle->cb[event] = cb;
}
PressEvent get_button_event(struct Button* handle)
{
return (PressEvent)(handle->event);
}
void button_handler(struct Button* handle)
{
uint8_t read_gpio_level = handle->hal_button_Level();
if((handle->state) > 0) handle->ticks++;
if(read_gpio_level != handle->button_level) {
if(++(handle->debounce_cnt) >= DEBOUNCE_TICKS) {
handle->button_level = read_gpio_level;
handle->debounce_cnt = 0;
}
} else {
handle->debounce_cnt = 0;
}
switch (handle->state) {
case 0:
if(handle->button_level == handle->active_level) {
handle->event = (uint8_t)PRESS_DOWN;
EVENT_CB(PRESS_DOWN);
handle->ticks = 0;
handle->repeat = 1;
handle->state = 1;
} else {
handle->event = (uint8_t)NONE_PRESS;
}
break;
case 1:
if(handle->button_level != handle->active_level) {
handle->event = (uint8_t)PRESS_UP;
EVENT_CB(PRESS_UP);
handle->ticks = 0;
handle->state = 2;
} else if(handle->ticks > LONG_TICKS) {
handle->event = (uint8_t)LONG_PRESS_START;
EVENT_CB(LONG_PRESS_START);
handle->state = 5;
}
break;
case 2:
if(handle->button_level == handle->active_level) {
handle->event = (uint8_t)PRESS_DOWN;
EVENT_CB(PRESS_DOWN);
handle->repeat++;
EVENT_CB(PRESS_REPEAT);
handle->ticks = 0;
handle->state = 3;
} else if(handle->ticks > SHORT_TICKS) {
if(handle->repeat == 1) {
handle->event = (uint8_t)SINGLE_CLICK;
EVENT_CB(SINGLE_CLICK);
} else if(handle->repeat == 2) {
handle->event = (uint8_t)DOUBLE_CLICK;
EVENT_CB(DOUBLE_CLICK);
}
handle->state = 0;
}
break;
case 3:
if(handle->button_level != handle->active_level) {
handle->event = (uint8_t)PRESS_UP;
EVENT_CB(PRESS_UP);
if(handle->ticks < SHORT_TICKS) {
handle->ticks = 0;
handle->state = 2;
} else {
handle->state = 0;
}
}else if(handle->ticks > SHORT_TICKS){
handle->state = 0;
}
break;
case 5:
if(handle->button_level == handle->active_level) {
handle->event = (uint8_t)LONG_PRESS_HOLD;
EVENT_CB(LONG_PRESS_HOLD);
} else {
handle->event = (uint8_t)PRESS_UP;
EVENT_CB(PRESS_UP);
handle->state = 0;
}
break;
}
}
int button_start(struct Button* handle)
{
struct Button* target = head_handle;
while(target) {
if(target == handle) return -1;
target = target->next;
}
handle->next = head_handle;
head_handle = handle;
return 0;
}
void button_stop(struct Button* handle)
{
struct Button** curr;
for(curr = &head_handle; *curr; ) {
struct Button* entry = *curr;
if (entry == handle) {
*curr = entry->next;
return;
} else
curr = &entry->next;
}
}
void button_ticks()
{
struct Button* target;
for(target=head_handle; target; target=target->next) {
button_handler(target);
}
}
-
头文件
#ifndef _MULTI_BUTTON_H_
#define _MULTI_BUTTON_H_
#include "stdint.h"
#include "string.h"
#define TICKS_INTERVAL 5
#define DEBOUNCE_TICKS 3
#define SHORT_TICKS (300 /TICKS_INTERVAL)
#define LONG_TICKS (1000 /TICKS_INTERVAL)
typedef void (*BtnCallback)(void*);
typedef enum {
PRESS_DOWN = 0,
PRESS_UP,
PRESS_REPEAT,
SINGLE_CLICK,
DOUBLE_CLICK,
LONG_PRESS_START,
LONG_PRESS_HOLD,
number_of_event,
NONE_PRESS
}PressEvent;
typedef struct Button {
uint16_t ticks;
uint8_t repeat : 4;
uint8_t event : 4;
uint8_t state : 3;
uint8_t debounce_cnt : 3;
uint8_t active_level : 1;
uint8_t button_level : 1;
uint8_t (*hal_button_Level)(void);
BtnCallback cb[number_of_event];
struct Button* next;
}Button;
#ifdef __cplusplus
extern "C" {
#endif
void button_init(struct Button* handle, uint8_t(*pin_level)(), uint8_t active_level);
void button_attach(struct Button* handle, PressEvent event, BtnCallback cb);
PressEvent get_button_event(struct Button* handle);
int button_start(struct Button* handle);
void button_stop(struct Button* handle);
void button_ticks(void);
#ifdef __cplusplus
}
#endif
#endif
|