K027 基于51/STM32 SD_mini/Micro卡模块测试 串口打印读取值
一. 实现功能
上电后串口助手打印读取之前存储的数据
二. 硬件清单
- SD_mini/Micro卡模块
- STM32F103C8T6/STC89C52RC
- SWD或JLINK仿真器(直接用CH340串口模块烧录也行,不过注意配置BOOT)
- 杜邦线若干
三. 资料清单
程序代码 
文档资料 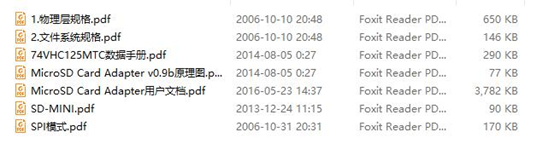
四. 模块简介
1.基本参数 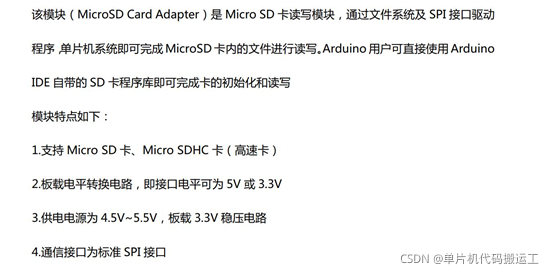 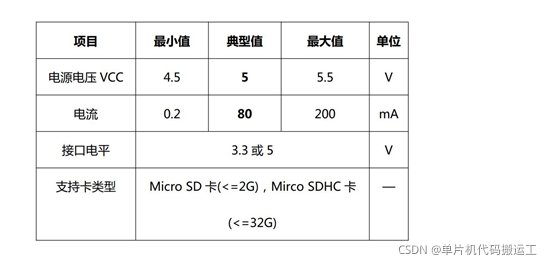
2.引脚说明 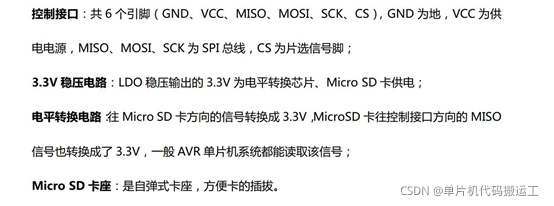
五. 接线
基于STM32 +模块接线
模块----------------------------------STM32
VCC-----------------------------------5V/3.3(有降压模块接5无降压模块接3.3)
GND-----------------------------------GND
OUT----------------- --------------- - GPIOB_5
SPI_CS --------------------------------GPIOB_6
SPI_SCK--------------------- ----------GPIOB_7
SPI_MISO------------------- ---------- GPIOB_8
SPI_MOSI------------------------------GPIOB_9
基于51 +SD_mini/Micro卡模块接线
模块-------- ----------------------STC89C52RC
VCC-------------------------------------5V/3.3(有降压模块接5无降压模块接3.3)
GND-------------------------------------GND
SPI_CS -------------------------------- P1.0
SPI_SCK--------------------- ---------- P1.2
SPI_MISO------------------- ---------- P1.3
SPI_MOSI------------------------------ P1.1
六.代码说明
以下以32代码为例, 串口函数直接移植过来用就行。
1. 模块引脚配置
void SPI_SD_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
SD_SPI_ALL_APBxClock_FUN ( SD_SPI_ALL_CLK, ENABLE );
GPIO_InitStructure.GPIO_Pin = SD_SPI_CS_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(SD_SPI_CS_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = SD_SPI_SCK_PIN;
GPIO_Init(SD_SPI_SCK_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = SD_SPI_MOSI_PIN;
GPIO_Init(SD_SPI_MOSI_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = SD_SPI_MISO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(SD_SPI_MISO_PORT, &GPIO_InitStructure);
SPI_SD_CS_1;
}
2. SPI读写函数
void SD_2Byte_Write(uint16_t IOData)
{
uint8_t BitCounter;
for (BitCounter=0;BitCounter<16;BitCounter++)
{
SD_SPI_SCK_0;
DelayUs(5);
if(IOData&0x8000)
SD_SPI_MOSI_1;
else
SD_SPI_MOSI_0;
SD_SPI_SCK_1;
DelayUs(5);
IOData=IOData<<1;
}
}
void SD_Write(uint16_t IOData)
{
uint8_t BitCounter;
IOData=IOData<<8;
for (BitCounter=0;BitCounter<8;BitCounter++)
{
SD_SPI_SCK_0;
DelayUs(5);
if(IOData&0x8000)
SD_SPI_MOSI_1;
else
SD_SPI_MOSI_0;
SD_SPI_SCK_1;
DelayUs(5);
IOData=IOData<<1;
}
}
uint16_t SD_2Byte_Read(void)
{
uint16_t Buffer;
uint8_t BitCounter;
Buffer=0;
for (BitCounter=0;BitCounter<16;BitCounter++)
{
SD_SPI_SCK_0;
DelayUs(5);
SD_SPI_SCK_1;
DelayUs(5);
Buffer=Buffer<<1;
if(READ_SD_SPI_MISO)
Buffer++;
}
return Buffer;
}
uint16_t SD_Read(void)
{
uint16_t Buffer;
uint8_t BitCounter;
Buffer=0xffff;
for (BitCounter=0;BitCounter<8;BitCounter++)
{
SD_SPI_SCK_0;
DelayUs(5);
SD_SPI_SCK_1;
DelayUs(5);
Buffer=Buffer<<1;
if(READ_SD_SPI_MISO)
Buffer++;
}
return Buffer;
}
3. 功能函数
比较多且有点负载,只展示部分,有需要直接加群私聊群主获取
uint16_t SD_Initiate_Card(void)
{
unsigned int temp,Response,MaximumTimes;
MaximumTimes=50;
for(temp=0;temp<MaximumTimes;temp++)
{
Response=SD_CMD_Write(0x0037,0x00000000,1,0);
Response=SD_CMD_Write(0x0029,0x00000000,1,0);
if (Response==0xff00)
temp=MaximumTimes;
}
return Response;
}
uint16_t SD_Get_CardInfo(void)
{
unsigned int temp,Response,MaximumTimes;
MaximumTimes=50;
for(temp=0;temp<MaximumTimes;temp++)
{
Response=SD_CMD_Write(9,0x00000000,1,1);
if (Response==0xff00)
temp=MaximumTimes;
}
for (temp=0;temp<8;temp++)
{
SD_SPI_SCK_0;
DelayUs(5);
SD_SPI_SCK_1;
DelayUs(5);
}
for (temp=0;temp<8;temp++) ReadBuffer[temp]=SD_2Byte_Read();
for (temp=0;temp<16;temp++)
{
SD_SPI_SCK_0;
DelayUs(5);
SD_SPI_SCK_1;
DelayUs(5);
}
SPI_SD_CS_1;
for (temp=0;temp<8;temp++)
{
SD_SPI_SCK_0;
DelayUs(5);
SD_SPI_SCK_1;
DelayUs(5);
}
BlockNR=((ReadBuffer[3]<<2)&0x0fff)+((ReadBuffer[4]>>14)&0x0003)+1;
BlockNR=BlockNR*(0x0002<<(((ReadBuffer[4]<<1)&0x0007)+((ReadBuffer[5]>>15)&0x0001)+1));
return Response;
}
uint16_t SD_Overall_Initiation(void)
{
unsigned int Response,Response_2;
Response=0x0000;
Response_2=0xff00;
SD_SPI_MOSI_1;
do
Response=SD_Reset_Card();
while (Response!=0xff01);
if (Response!=0xff01) Response_2+=8;
Response=SD_Initiate_Card();
if (Response==0xff00)
;
else
{
Response_2+=4;
;
}
do
Response=SD_Get_CardInfo();
while (Response!=0xff00);
if (Response==0xff01) Response_2+=2;
return Response_2;
}
4. 主函数
int main(void)
{
uint16_t M_Response=0;
uint16_t Data;
uint8_t i;
DelayInit();
USART_Config();
SPI_SD_Init();
for(i=0;i<128;i++)
{
if (i < 64)
{
WriteBuffer[i]=0x4141;
}
else
{
WriteBuffer[i]=0x4242;
}
}
while(1)
{
M_Response=SD_Overall_Initiation();
M_Response=SD_CMD_Write(16,512,1,0);
Data=SD_Get_CardID();
Write_Single_Block(0x0000);
Read_Single_Block(0x0000);
for(i=0; i<128; i++)
{
Usart_SendByte(DEBUG_USARTx, ReadBuffer[i]>>8);
Usart_SendByte(DEBUG_USARTx, ReadBuffer[i] );
}
}
}
七.资料获取
加群私聊群主可免费获得,也可加群学习交流 群号:1041406448。
|