Java 串口通信Demo
一、工具
1、虚拟串口工具:Virtual Serial Port Driver Pro
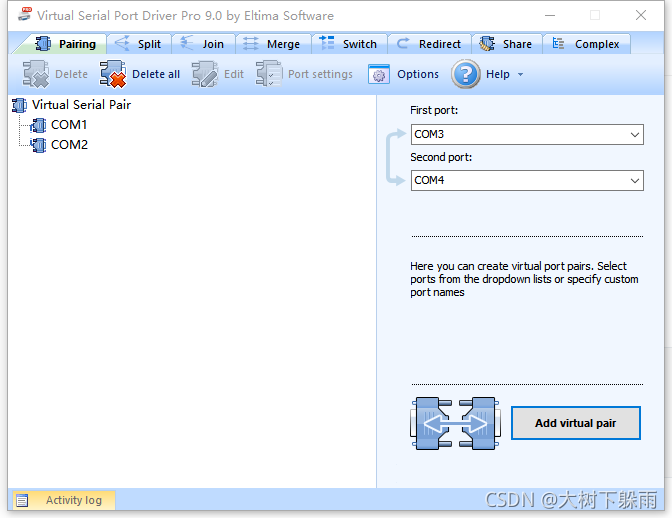
2、串口调试工具
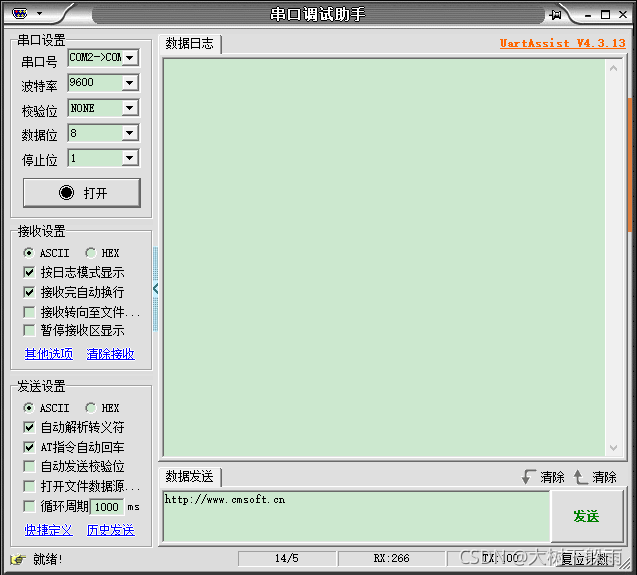
3、下载
链接:https://pan.baidu.com/s/1GSBlRECJ53WH6zHIn0vUWg 提取码:tknr
二、pom引入
<dependency>
<groupId>org.rxtx</groupId>
<artifactId>rxtx</artifactId>
<version>2.1.7</version>
</dependency>
三、Java串口通信demo
import com.google.common.util.concurrent.ThreadFactoryBuilder;
import gnu.io.CommPortIdentifier;
import gnu.io.SerialPort;
import lombok.SneakyThrows;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.charset.StandardCharsets;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.TimeUnit;
public class SerialPortCommunicationDemo {
private static byte[] cache = new byte[1024];
static SimpleDateFormat simpleDateFormat = new SimpleDateFormat("YYYY-MM-dd HH:mm:ss");
public static void main(String[] args) throws Exception {
String serialPortNo = "COM1";
List<String> serialPortList = new ArrayList<>();
Enumeration portIdentifiers = CommPortIdentifier.getPortIdentifiers();
System.out.printf("输出所有串口:");
while (portIdentifiers.hasMoreElements()) {
CommPortIdentifier portIdentifier = (CommPortIdentifier) portIdentifiers.nextElement();
serialPortList.add(portIdentifier.getName());
}
System.out.println(serialPortList);
if (!serialPortList.contains(serialPortNo)) {
System.out.println("串口不存在");
return;
}
CommPortIdentifier portIdentifier = CommPortIdentifier.getPortIdentifier(serialPortNo);
SerialPort serialPort = (SerialPort) portIdentifier.open(serialPortNo, 1000);
System.out.println("监听串口:" + serialPortNo);
ThreadFactory threadFactory = new ThreadFactoryBuilder().setNameFormat("thread-call-runner-%d").build();
ScheduledThreadPoolExecutor scheduledThreadPoolExecutor = new ScheduledThreadPoolExecutor(10, threadFactory);
scheduledThreadPoolExecutor.scheduleAtFixedRate(new Runnable() {
@SneakyThrows
@Override
public void run() {
readData(serialPort);
}
}, 1000, 3000, TimeUnit.MILLISECONDS);
scheduledThreadPoolExecutor.scheduleAtFixedRate(new Runnable() {
@SneakyThrows
@Override
public void run() {
writeData(serialPort);
}
}, 1000, 5000, TimeUnit.MILLISECONDS);
}
public static void readData(SerialPort serialPort) throws Exception {
InputStream inputStream = serialPort.getInputStream();
int availableBytes = inputStream.available();
while (availableBytes > 0) {
inputStream.read(cache);
for (int i = 0; i < cache.length && i < availableBytes; i++) {
System.out.print((char) cache[i]);
}
System.out.println();
availableBytes = inputStream.available();
}
}
public static void writeData(SerialPort serialPort) throws Exception {
OutputStream outputStream = serialPort.getOutputStream();
String date = simpleDateFormat.format(new Date());
outputStream.write(date.getBytes(StandardCharsets.UTF_8));
}
}
四、运行截图
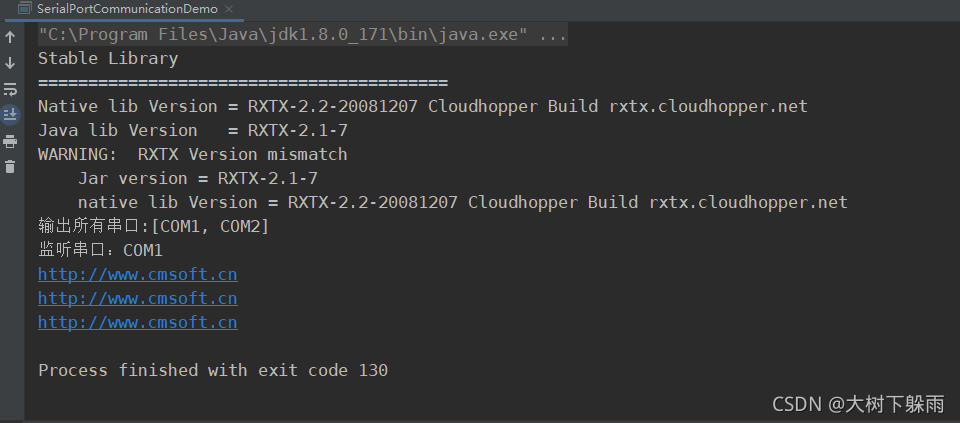
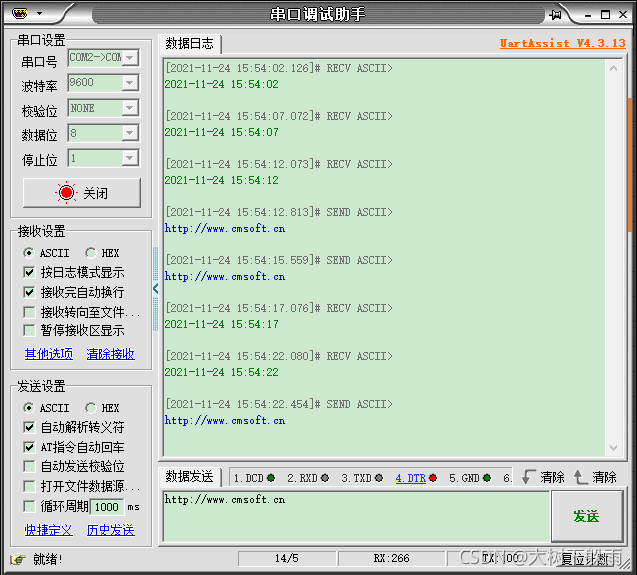
五、串口工具类
import gnu.io.*;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.TooManyListenersException;
@Slf4j
public class SerialPortUtil {
private static SerialPortUtil serialPortUtil = null;
static {
if (serialPortUtil == null) {
serialPortUtil = new SerialPortUtil();
}
}
private SerialPortUtil() {
}
public static SerialPortUtil getSerialPortUtil() {
if (serialPortUtil == null) {
serialPortUtil = new SerialPortUtil();
}
return serialPortUtil;
}
public ArrayList<String> findPort() {
Enumeration<CommPortIdentifier> portList = CommPortIdentifier.getPortIdentifiers();
ArrayList<String> portNameList = new ArrayList<>();
while (portList.hasMoreElements()) {
String portName = portList.nextElement().getName();
portNameList.add(portName);
}
return portNameList;
}
public SerialPort openPort(String portName, int baudrate, int databits, int stopbits, int parity) {
if (!findPort().contains(portName)) {
log.info("串口" + portName + "不存在...");
return null;
}
try {
CommPortIdentifier portIdentifier = CommPortIdentifier.getPortIdentifier(portName);
CommPort commPort = portIdentifier.open(portName, 2000);
if (commPort instanceof SerialPort) {
SerialPort serialPort = (SerialPort) commPort;
try {
serialPort.setSerialPortParams(baudrate, databits, stopbits, parity);
} catch (UnsupportedCommOperationException unsupportedCommOperationException) {
log.error("串口" + portName + "参数配置错误...");
unsupportedCommOperationException.printStackTrace();
}
log.info("串口" + portName + "打开成功...");
return serialPort;
} else {
log.error(portName + "不是串口...");
}
} catch (PortInUseException portInUseException) {
log.error("串口" + portName + "被占用...");
portInUseException.printStackTrace();
} catch (Exception exception) {
exception.printStackTrace();
}
return null;
}
public void closePort(SerialPort serialPort) {
if (serialPort != null) {
serialPort.close();
log.info(SerialPortStringUtil.serialPortNameHandle(serialPort.getName())+"串口关闭成功...");
}
}
public void sendToPort(SerialPort serialPort, byte[] order) {
OutputStream out = null;
try {
out = serialPort.getOutputStream();
out.write(order);
out.flush();
log.info(SerialPortStringUtil.serialPortNameHandle(serialPort.getName())+"串口写入数据完毕...");
} catch (IOException e) {
e.printStackTrace();
} finally {
if (out != null) {
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public byte[] readFromPort(SerialPort serialPort) {
InputStream in = null;
byte[] bytes = null;
try {
in = serialPort.getInputStream();
int bufflenth = in.available();
while (bufflenth != 0) {
bytes = new byte[bufflenth];
in.read(bytes);
bufflenth = in.available();
}
log.info(SerialPortStringUtil.serialPortNameHandle(serialPort.getName())+"串口读取数据完毕...");
} catch (IOException e) {
e.printStackTrace();
} finally {
if (in != null) {
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return bytes;
}
public void addListener(SerialPort serialPort, SerialPortEventListener listener) {
try {
serialPort.addEventListener(listener);
serialPort.notifyOnDataAvailable(true);
serialPort.notifyOnBreakInterrupt(true);
log.info(SerialPortStringUtil.serialPortNameHandle(serialPort.getName())+"串口添加监听成功...");
} catch (TooManyListenersException e) {
log.error("监听器串口对象数量溢出...");
e.printStackTrace();
}
}
public void removeListener(SerialPort serialPort, SerialPortEventListener listener) {
serialPort.removeEventListener();
log.info(SerialPortStringUtil.serialPortNameHandle(serialPort.getName())+"串口移除监听成功...");
}
}
|