3.16 haas506开发教程-example-通过子线程检测串口指令数据
1.简介
?本案例使用子线程对串口所发送的数据进行处理和执行相应的逻辑操作。
2.测试代码
?在main.py中,先初始化haas506开发板上的led灯,然后开启子线程对串口助手发送过来的数据进行处理,最后在主线程中循环打印helloworld。
import utime as time
import app_led
import app_input
def main():
app_led.led_init()
app_input.start_input_cmd_thread()
while True:
time.sleep(1)
print("hello world")
if __name__=="__main__":
main()
?在app_led.py中初始化led灯,以及执行点亮和熄灭操作。
from driver import GPIO
led1=GPIO()
led2=GPIO()
led3=GPIO()
led4=GPIO()
led5=GPIO()
led1.open('led1')
led2.open('led2')
led3.open('led3')
led4.open('led4')
led5.open("led5")
def led_init():
led1.write(0)
led2.write(0)
led3.write(0)
led4.write(0)
led5.write(0)
def write(key,value):
if key=="led1":
led1.write(value)
elif key=="led2":
led2.write(value)
elif key=="led3":
led3.write(value)
elif key=="led4":
led4.write(value)
elif key=="led5":
led5.write(value)
else:
print("please check cmd that you send")
pass
?在app_input.py中 ,先接收串口助手发送过来的json格式的数据,然后对该数据进行处理。
import _thread
import ujson
import app_led
def wait_input_cmd(id):
while True:
try:
inputcmd = input('please input cmd:')
except KeyboardInterrupt:
_thread.exit()
try:
inputcmd_dict = ujson.loads(inputcmd)
if isinstance(inputcmd_dict,dict):
if 'led_ctrl' in inputcmd_dict and isinstance(inputcmd_dict['led_ctrl'],dict):
for key,value in inputcmd_dict['led_ctrl'].items():
app_led.write(key,value)
else:
print("cmd is not a dict")
print(inputcmd_dict)
except ValueError:
'''other cmd process'''
print('input cmd is:' + inputcmd)
pass
finally:
pass
def start_input_cmd_thread():
_thread.start_new_thread(wait_input_cmd,(1,))
{
"name": "haas506",
"version": "1.0.0",
"io": {
"led1": {
"type": "GPIO",
"port": 0,
"dir": "output",
"pull": "pullup"
},
"led2": {
"type": "GPIO",
"port": 6,
"dir": "output",
"pull": "pullup"
},
"led3": {
"type": "GPIO",
"port": 7,
"dir": "output",
"pull": "pullup"
},
"led4": {
"type": "GPIO",
"port": 8,
"dir": "output",
"pull": "pullup"
},
"led5": {
"type": "GPIO",
"port": 9,
"dir": "output",
"pull": "pullup"
},
"serial1":{
"type":"UART",
"port":0,
"dataWidth":8,
"baudRate":115200,
"stopBits":1,
"flowControl":"disable",
"parity":"none"
},
"serial2":{
"type":"UART",
"port":1,
"dataWidth":8,
"baudRate":115200,
"stopBits":1,
"flowControl":"disable",
"parity":"none"
},
"serial3":{
"type":"UART",
"port":2,
"dataWidth":8,
"baudRate":115200,
"stopBits":1,
"flowControl":"disable",
"parity":"none"
}
},
"debugLevel": "ERROR",
"repl":"enable",
"replPort":0
}
3.测试结果
?代码烧录完毕后,打开串口助手。主线程循环打印"helloworld”,子线程等待数据的输入。
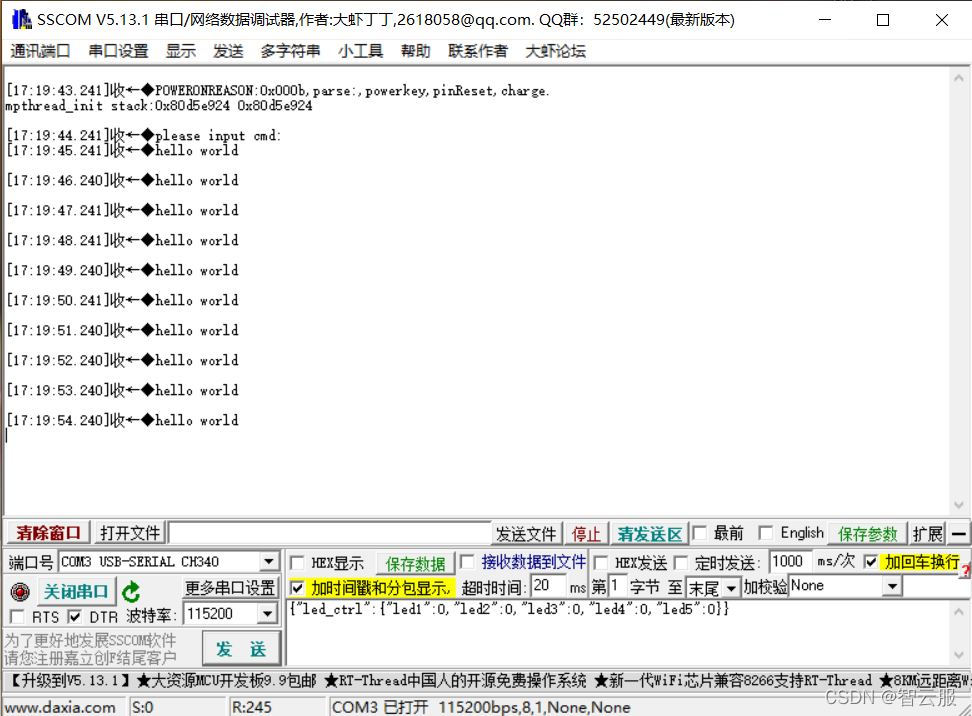
?先在串口助手的编辑框中输入{“led_ctrl”:{“led1”:1,“led2”:1,“led3”:1,“led4”:1,“led5”:1}},然后勾选“加回车换行”,最后点击“发送”。这样会点亮5个小灯。
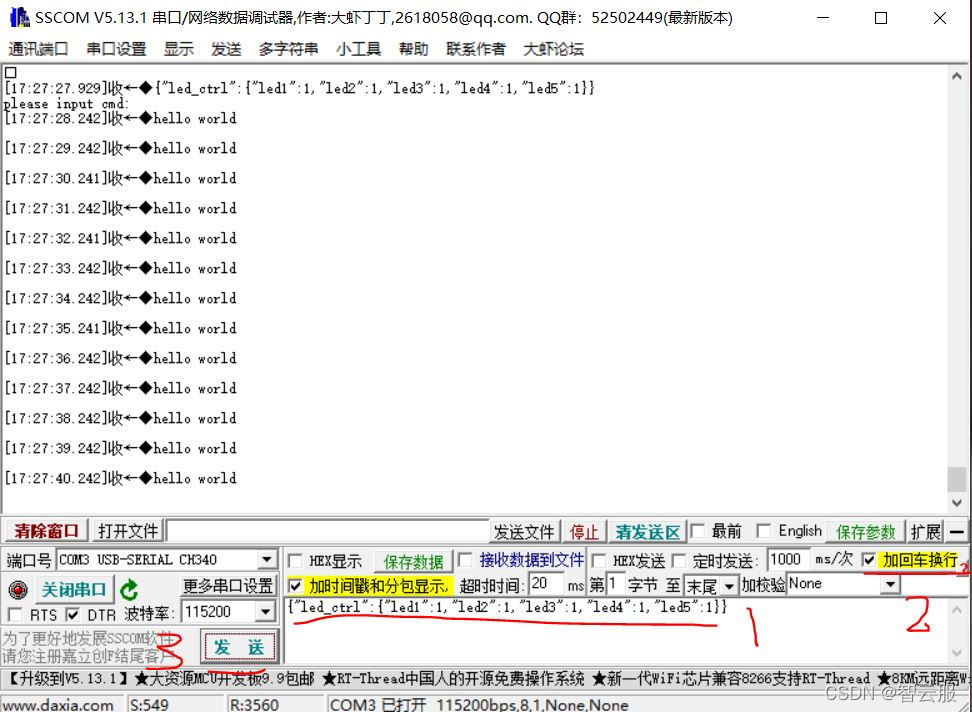
?也可以自定义点亮/熄灭某个小灯,如输入{“led_ctrl”:{“led1”:1,“led2”:0,“led3”:0,“led4”:0,“led5”:1}},点亮两个小灯和熄灭3个小灯。
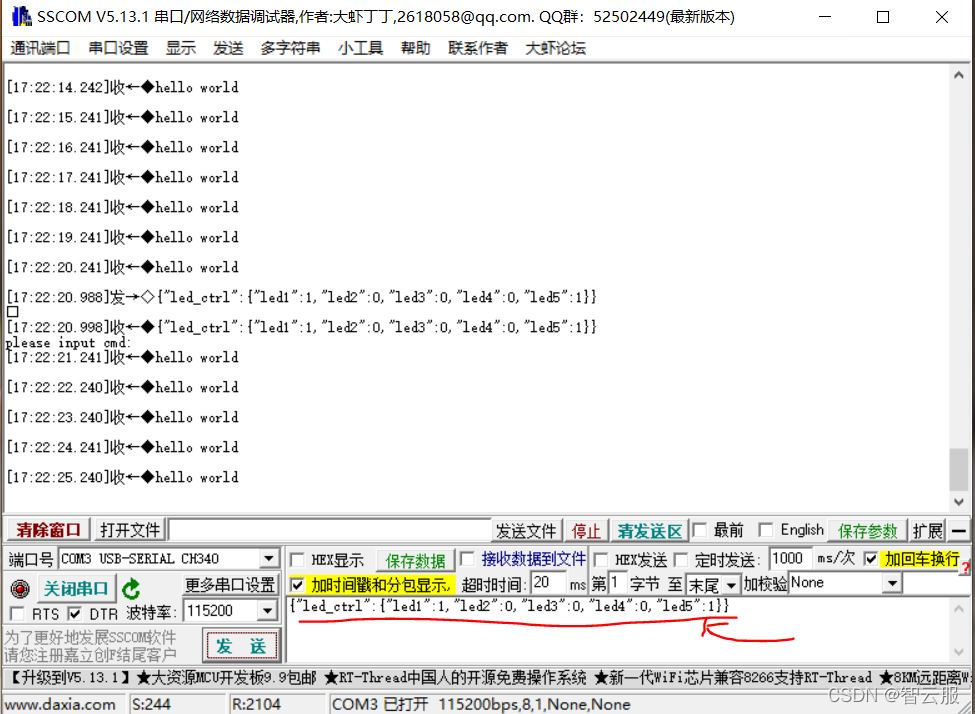
|