前言
当时刚学完单片机,参考了很多别人的代码,教程csdn上一搜就有,不过能做个东西觉得自己还挺厉害的,板子是学校发的散件,然后自己焊的,很好玩。买了一个底盘,两个l298n,其他不需要什么。
一、代码
前三个文件是一个单片机里的,最后一个文件是另一个单片机里面的。
car.h:
#ifndef __car_H
#define __car_H
#include <reg52.h>
#include <intrins.h>
sbit Left_moto_pwm1=P1^6 ;
sbit Right_moto_pwm1=P1^7;
sbit Left_moto_pwm2=P1^5 ;
sbit Right_moto_pwm2=P1^4;
sbit p20=P2^0;
sbit p21=P2^1;
sbit p22=P2^2;
sbit p23=P2^3;
sbit p24=P2^4;
sbit p25=P2^5;
sbit p26=P2^6;
sbit p27=P2^7;
sbit led0=P0^0;
sbit led1=P0^1;
sbit led2=P0^2;
sbit led3=P0^3;
sbit led4=P0^4;
sbit led5=P0^5;
sbit led6=P0^6;
sbit led7=P0^7;
void Left_moto_go() ;
void Left_moto_back() ;
void Left_moto_stp() ;
void Right_moto_go();
void Right_moto_back();
void Right_moto_stp();
void delay(unsigned int k) ;
void delay1s(void) ;
void pwm_out_left_moto(void) ;
void pwm_out_right_moto(void);
void run(void);
void back(void);
void left(void);
void right(void);
void stop(void);
void rotate(void);
void singel_front_right();
void singel_front_left();
void singel_behind_right();
void singel_behind_left();
void single_front_left_go();
void single_front_right_go();
void single_behind_left_go();
void single_behind_right_go();
#endif
motor_control.c
#include <car.h>
unsigned char pwm_val_left =0;
unsigned char push_val_left =0;
unsigned char pwm_val_right =0;
unsigned char push_val_right=0;
unsigned char Left_Speed_Ratio;
unsigned char Right_Speed_Ratio;
bit Left_moto_stop =1;
bit Right_moto_stop =1;
void Left_moto_go()
{p20=0;p21=1;p24=0;p25=1;}
void Left_moto_back()
{p20=1;p21=0;p24=1;p25=0;}
void Left_moto_stp()
{p20=1;p21=1;p24=1;p25=1;}
void Right_moto_go()
{p22=0;p23=1;p26=0;p27=1;}
void Right_moto_back()
{p22=1;p23=0;p26=1;p27=0;}
void Right_moto_stp()
{p22=1;p23=1;p26=1;p27=1;}
void singel_front_right()
{p26=0;p27=1;}
void singel_front_left()
{p24=0;p25=1;}
void singel_behind_right()
{p22=0;p23=1;}
void singel_behind_left()
{p20=0;p21=1;}
void pwm_out_left_moto(void) {
if(Left_moto_stop)
{
if(pwm_val_left<=push_val_left)
{
Left_moto_pwm1=1;
Left_moto_pwm2=1;}
else
{
Left_moto_pwm1=0;
Left_moto_pwm2=0;
}
if(pwm_val_left>=10)
{
pwm_val_left=0;
} }
else
{
Left_moto_pwm1=0;
Left_moto_pwm2=0;
}}
void pwm_out_right_moto(void)
{
if(Right_moto_stop)
{
if(pwm_val_right<=push_val_right)
{
Right_moto_pwm1=1;
Right_moto_pwm2=1;
}
else
{
Right_moto_pwm1=0;
Right_moto_pwm2=0;
}
if(pwm_val_right>=10)
{
pwm_val_right=0;
}
}
else
{
Right_moto_pwm1=0;
Right_moto_pwm2=0;
}
}
void run(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
Left_moto_go();
Right_moto_go();
}
void back(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
Left_moto_back();
Right_moto_back();
}
void left(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
Right_moto_go();
Left_moto_stp();
}
void right(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
Right_moto_stp();
Left_moto_go();
}
void stop(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
Left_moto_stp();
Right_moto_stp();
}
void rotate(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
Left_moto_back();
Right_moto_go();
}
void Speed_add(void)
{
if(Left_Speed_Ratio<10)
{
Left_Speed_Ratio++;
Right_Speed_Ratio++;
}
else
{
Left_Speed_Ratio=8;
Right_Speed_Ratio=8;
}
}
void Speed_reduce(void)
{
if(Left_Speed_Ratio>0)
{
Left_Speed_Ratio--;
Right_Speed_Ratio--;
}
else
{
Left_Speed_Ratio=3;
Right_Speed_Ratio=3;
}
}
void Speed_reset(void)
{
Left_Speed_Ratio=5;
Right_Speed_Ratio=5;
}
void single_front_left_go(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
singel_front_left();
}
void single_front_right_go(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
singel_front_right();
}
void single_behind_left_go(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
singel_behind_left();
}
void single_behind_right_go(void)
{
push_val_left =Left_Speed_Ratio;
push_val_right =Right_Speed_Ratio;
singel_behind_right();
}
main.c:
#include <car.h>
#include <intrins.h>
#include <reg52.h>
extern unsigned char Left_Speed_Ratio;
extern unsigned char Right_Speed_Ratio;
unsigned int time=0;
unsigned char receive,receive_real_data;
extern unsigned char pwm_val_left;
extern unsigned char pwm_val_right;
void delay1s(void)
{
unsigned char a,b,c;
for(c=29;c>0;c--)
for(b=35;b>0;b--)
for(a=97;a>0;a--);
}
void delay10ms(void)
{
unsigned char b,c;
for(c=10;c>0;c--)
for(b=150;b>0;b--);
}
void TimerInit()
{
TMOD=0X21;
SCON=0x50;
PCON=0x80;
TH0=0XFC;
TL0=0X18;
TL1=TH1=0xF3;
ET0=1;
EA=1;
TR0=1;
TR1=1;
ES=1;
led7=0;
}
void timer0()interrupt 1 using 2
{
TH0=0XFC;
TL0=0X18;
time++;
pwm_val_left++;
pwm_val_right++;
pwm_out_left_moto();
pwm_out_right_moto();
}
void Com_Int(void) interrupt 4
{
EA = 0;
if(RI == 1)
{
led5=!led5;
RI = 0;
receive = SBUF;
if(receive!=0)
receive_real_data=receive;
switch(receive_real_data)
{
case 0x31: run();led0=!led0; break;
case 0x34: right();led1=!led1; break;
case 0x36: left(); led2=!led2;break;
case 0x39: back();led3=!led3; break;
case 0x35: stop(); led4=!led4;break;
case 0x32: rotate();break;
case 0x30: Speed_add(); led5=!led5;break;
case 0x38: Speed_reduce(); led6=!led6;break;
case 0x41: Speed_reset();break;
case 0x44: single_behind_left_go();break;
case 0x43: single_behind_right_go();break;
default:stop();break;
}
}
EA = 1; }
void main(){
TimerInit();
Left_Speed_Ratio=5;
Right_Speed_Ratio=5;
while(1);}
FA.c:
#include<reg51.h>
#define uchar unsigned char
uchar KeyValue=0;
uchar math,send;
sbit led=P0^0;
sbit led1=P0^1;
sbit led2=P0^2;
sbit led3=P0^3;
sbit led4=P0^4;
void delay(unsigned int time){
unsigned int j;
for(;time>0;time--)
for(j=0;j<125;j++);}
char key_buf[]={
0xee,0xde,0xbe,0x7e,
0xed,0xdd,0xbd,0x7d,
0xeb,0xdb,0xbb,0x7b,
0xe7,0xd7,0xb7,0x77};
void getkey(void) {
char key_scan[]={0xef,0xdf,0xbf,0x7f};
char i=0,j=0;
for(i=0;i<4;i++){
P2=key_scan[i];
led1=!led1;
if((P2&0x0f)!=0x0f){
delay(75);
if((P2&0x0f)!=0x0f){
for(j=0;j<16;j++){
if(key_buf[j]==P2){
led2=!led2;
switch(j){
case 0:SBUF=0x30;break;
case 1:SBUF=0x31;break;
case 2:SBUF=0x32;break;
case 3:SBUF=0x33;break;
case 4:SBUF=0x34;break;
case 5:SBUF=0x35;break;
case 6:SBUF=0x36;break;
case 7:SBUF=0x37;break;
case 8:SBUF=0x38;break;
case 9:SBUF=0x39;break;
case 10:SBUF=0x41;break;
case 11:SBUF=0x42;break;
case 12:SBUF=0x43;break;
case 13:SBUF=0x44;break;
case 14:SBUF=0x45;break;
case 15:SBUF=0x46;break;
default:led4=!led4;break;}
while(TI==0);
TI=0;
break; }}}} }
while((P2&0x0f)!=0x0f);
delay(75);
while((P2&0x0f)!=0x0f);}
void main(void){
SCON=0x50;
PCON=0x80;
TMOD=0x20;
TH1=TL1=0xf3;
TR1=1;
while(1){
getkey();
led=!led;
}}
二、设计
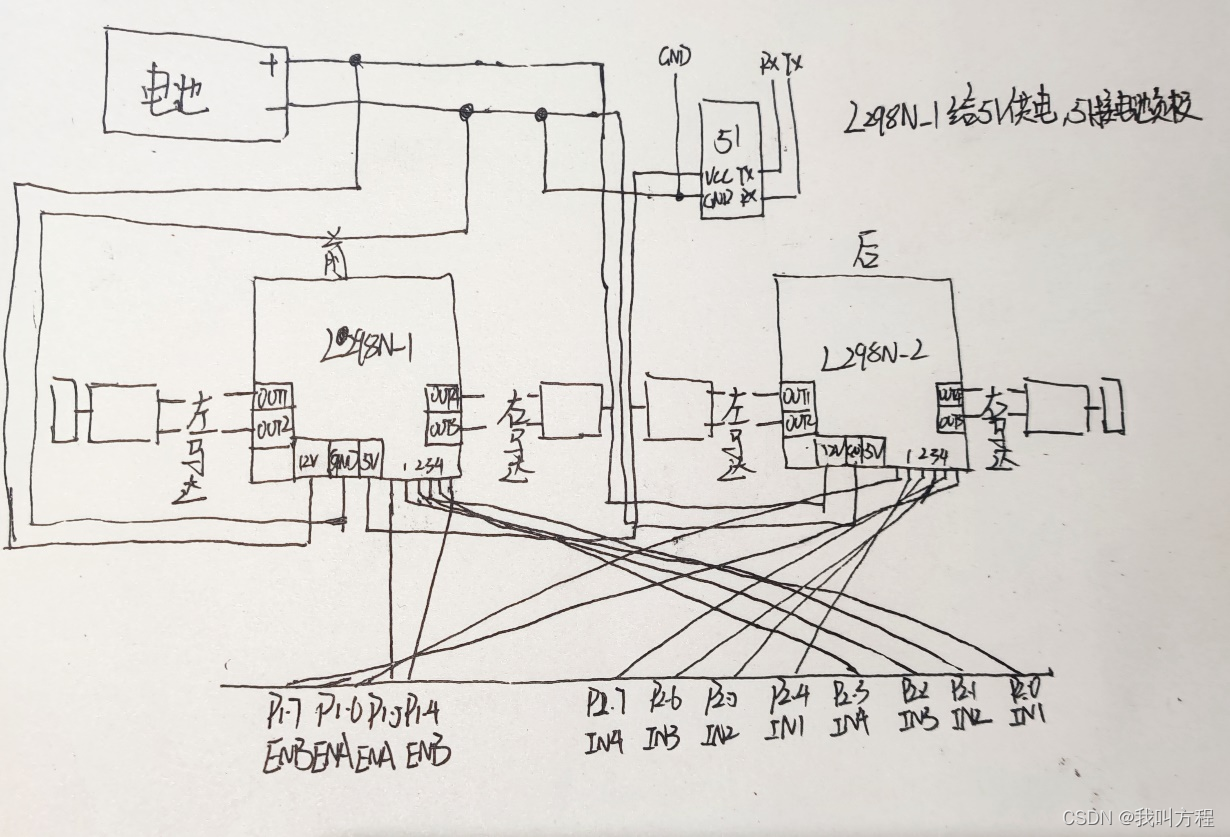 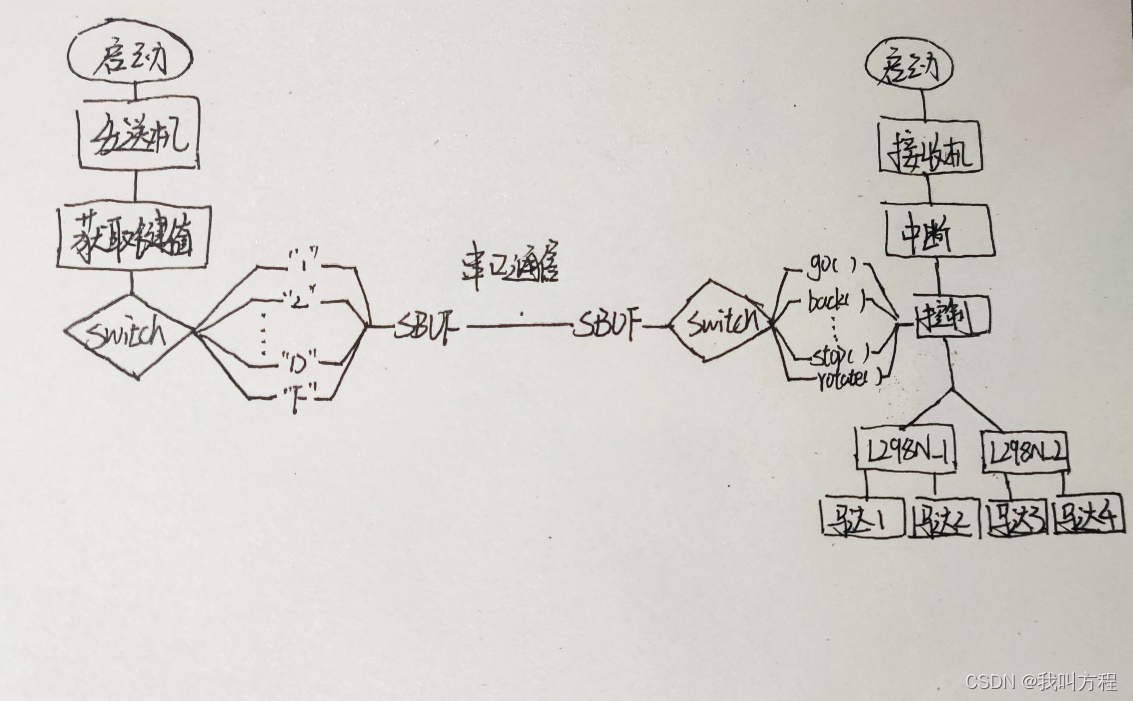 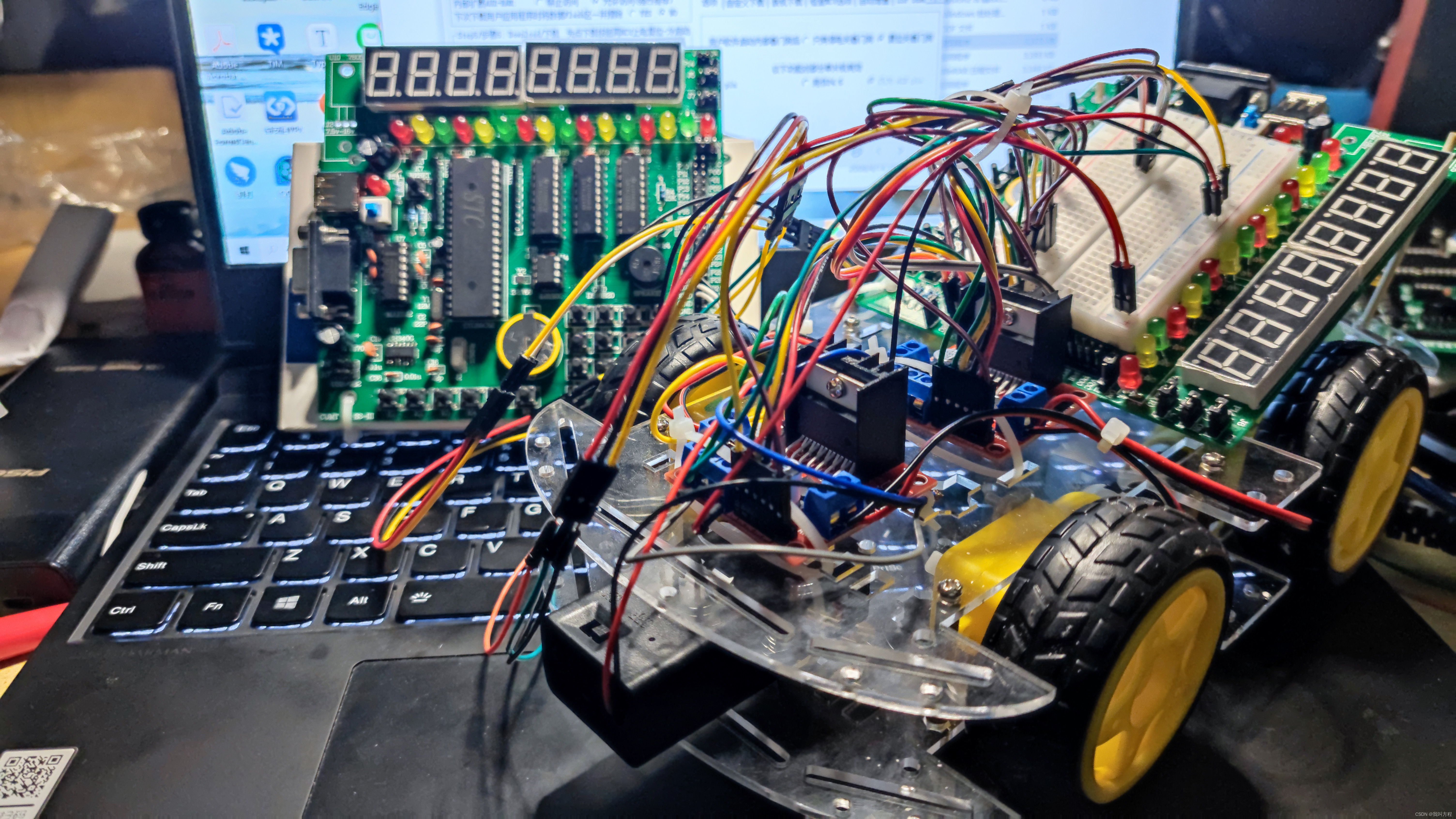
总结
考古一下这个最初的课设,虽然很简单,但是给我了很多思路。
|