代码均由IntelliJ IDEA 2020.1.4 x64运行 任务概述: 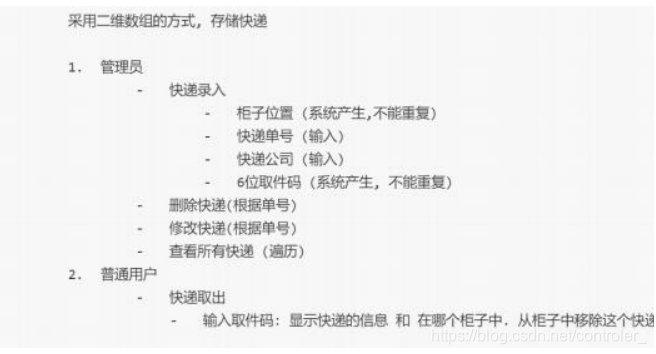 要求:运用面向对象,集合,IO流,实现快递管理系统
包视图: 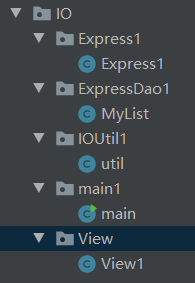
- Express1:快递信息存储类
- MyList:调度逻辑类
- View1:视图类
- util:序列化与反序列化
- main:调用实现类
注:导包可能出现问题,这里就不用我的包了,运用时看情况导包。
1.快递信息储存类:
public class Express1 implements Serializable {
private String number;
private String company;
private int code;
public Express1() {
}
public Express1(String number, String company, int code) {
this.number = number;
this.company = company;
this.code = code;
}
@Override
public String toString() {
return "Express1{" +
"number='" + number + '\'' +
", company='" + company + '\'' +
", code=" + code +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Express1 express1 = (Express1) o;
return Objects.equals(number, express1.number);
}
@Override
public int hashCode() {
return Objects.hash(number);
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
}
视图类:
public class View1 {
private Scanner input = new Scanner(System.in);
public void welcome(){
System.out.println("欢迎使用此快递管理系统");
}
public void bye(){
System.out.println("期待您的下次使用");
}
public int menu(){
System.out.println("请根据提示,输入功能序号:");
System.out.println("1. 快递员");
System.out.println("2. 用户");
System.out.println("0. 退出");
String text = input.nextLine();
int num = -1;
try {
num = Integer.parseInt(text);
}catch (Exception e){
}
if (num<0 || num>2){
System.out.println("输入有误,请重新输入:");
return menu();
}
return num;
}
public int Courier(){
System.out.println("请根据提示,输入功能序号:");
System.out.println("1. 快递录入");
System.out.println("2. 快递删除");
System.out.println("3. 快递修改");
System.out.println("4. 查看所有快递");
System.out.println("0. 返回上级目录");
String text = input.nextLine();
int num = -1;
try {
num = Integer.parseInt(text);
}catch (Exception e){
}
if (num<0 || num>4){
System.out.println("输入有误,请重新输入:");
return Courier();
}
return num;
}
public Express1 Insert(){
System.out.println("请根据提示,输入快递信息:");
System.out.println("请输入快递单号:");
String number = input.nextLine();
System.out.println("请输入快递公司:");
String company = input.nextLine();
Express1 e = new Express1();
e.setNumber(number);
e.setCompany(company);
return e;
}
public int delete() {
System.out.println("是否确认删除?");
System.out.println("1. 确认删除");
System.out.println("2. 取消操作");
System.out.println("0. 退出");
String text = input.nextLine();
int num = -1;
try {
num = Integer.parseInt(text);
} catch (Exception e) {
}
if (num < 0 || num > 2) {
System.out.println("输入错误,请重新输入:");
return delete();
}
return num;
}
public String findByNumber(){
System.out.println("请根据提示,输入快递信息:");
System.out.println("请输入要操作的快递单号:");
String number = input.nextLine();
return number;
}
public void printNull(){
System.out.println("没有找到该快递");
}
public void update(Express1 e){
System.out.println("请输入新的快递单号:");
String number = input.nextLine();
System.out.println("请输入新的快递公司:");
String company = input.nextLine();
e.setNumber(number);
e.setCompany(company);
}
public void printExpress(Express1 e){
System.out.println("快递信息如下:");
System.out.println("快递公司;"+e.getCompany()+",快递单号:"+e.getNumber()+",取件码:"+e.getCode());
}
public void printAll(ArrayList<Express1> list){
if (list.isEmpty()){
System.out.println("没有信息");
return;
}else {
System.out.println(list.toArray().toString());
}
}
public int User(){
System.out.println("请根据提示,输入快递信息:");
System.out.println("请输入取件码:");
String code = input.nextLine();
int num = -1;
try {
num = Integer.parseInt(code);
}catch (Exception e){
}
if (num<100000 || num>999999){
System.out.println("输入有误,请重新输入:");
return User();
}
return num;
}
public void expressExits(){
System.out.println("快递已存在,请勿重复存储:");
}
public void success(){
System.out.println("操作成功");
}
}
调度逻辑类:
public class MyList {
private ArrayList<Express1> list = new ArrayList<>();
private Random random = new Random();
private static final String FILE_NAME = "express.txt";
static {
System.out.println("静态代码块执行---------");
File file = new File(FILE_NAME);
if (!file.exists()){
try {
if (file.createNewFile()){
System.out.println("第一次启动项目,创建储存文件成功");
}
} catch (IOException e) {
System.out.println(e.getMessage());
}
}
}
public MyList() {
System.out.println("构造方法执行-------------");
try {
Object obj = util.readFile(FILE_NAME);
if (obj!=null && obj instanceof ArrayList){
list = (ArrayList<Express1>) obj;
}
} catch (IOException e) {
System.out.println(e.getMessage());
} catch (ClassNotFoundException e) {
System.out.println(e.getMessage());
}
}
public boolean add(Express1 e) throws IOException {
if (list.size() == 100){
return false;
}
int x = -1;
int y = -1;
while (true){
x = random.nextInt(10);
y = random.nextInt(10);
if (!(list.contains(x) && list.contains(y))){
break;
}
}
int code = randomCode();
e.setCode(code);
list.add(e);
util.writeFile(list,FILE_NAME);
return true;
}
public int randomCode(){
while (true){
int code = random.nextInt(900000)+100000;
Express1 e = findByCode(code);
if (e == null){
return code;
}
}
}
public Express1 findByCode(int code){
for (int i = 0; i < list.size(); i++) {
if (list.size()!=0 && list.get(i).getCode() == code){
return list.get(i);
}
}
return null;
}
public void delete(Express1 e) throws IOException {
p:for (int i = 0; i < list.size(); i++) {
if (e.equals(list.get(i))){
list.remove(i);
util.writeFile(list,FILE_NAME);
break p;
}
}
}
public Express1 findByNumber(String number){
Express1 e = new Express1();
e.setNumber(number);
for (int i = 0; i < list.size(); i++) {
if (e.getNumber().equals(list.get(i).getNumber())){
return list.get(i);
}
}
return null;
}
public void update(Express1 oldExpress,Express1 newExpress) throws IOException {
delete(oldExpress);
add(newExpress);
}
public void findAll(){
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i).toString());
}
}
}
IO序列化与反序列化:
public class util {
public static Object readFile(String fileName) throws IOException, ClassNotFoundException {
FileInputStream fileInputStream = new FileInputStream(fileName);
ObjectInputStream objectInputStream= new ObjectInputStream(fileInputStream);
return objectInputStream.readObject();
}
public static void writeFile(Object obj,String fileName) throws IOException {
FileOutputStream fileOutputStream = new FileOutputStream(fileName);
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
objectOutputStream.writeObject(obj);
}
}
调用实现类:
public class main {
private static View1 v = new View1();
private static MyList list = new MyList();
public static void main(String[] args) throws IOException {
v.welcome();
p:while (true){
int menu = v.menu();
switch (menu){
case 1:
cClient();
break;
case 2:
uClient();
break;
case 0:
break p;
}
}
v.bye();
}
public static void cClient() throws IOException {
p:while (true){
int menu = v.Courier();
switch (menu){
case 1:
Insert();
break;
case 2:
delete();
break;
case 3:
upadte();
break;
case 4:
All();
break;
case 0:
break p;
}
}
}
public static void Insert() throws IOException {
Express1 e = v.Insert();
list.add(e);
}
public static void delete() throws IOException {
String number = v.findByNumber();
Express1 e = list.findByNumber(number);
if (e == null){
v.printNull();
}else {
v.printExpress(e);
int type= v.delete();
if (type == 1){
list.delete(e);
v.success();
}
}
}
public static void upadte() throws IOException {
String number = v.findByNumber();
Express1 e = list.findByNumber(number);
Express1 e2 = e;
if (e == null){
v.printNull();
}else {
v.printExpress(e);
v.update(e2);
list.update(e,e2);
v.printExpress(e2);
}
}
public static void All(){
list.findAll();
}
public static void uClient() throws IOException {
int code = v.User();
Express1 e = list.findByCode(code);
if (e == null){
v.printNull();
}else {
v.success();
list.delete(e);
v.printExpress(e);
}
}
}
注:仅供参考,可以根据代码自己修改。
|